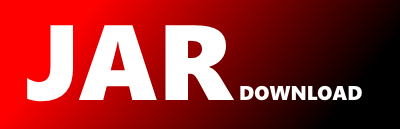
com.stripe.model.PaymentMethod Maven / Gradle / Ivy
// Generated by delombok at Fri Nov 19 19:06:04 EST 2021
// File generated from our OpenAPI spec
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.PaymentMethodAttachParams;
import com.stripe.param.PaymentMethodCreateParams;
import com.stripe.param.PaymentMethodDetachParams;
import com.stripe.param.PaymentMethodListParams;
import com.stripe.param.PaymentMethodRetrieveParams;
import com.stripe.param.PaymentMethodUpdateParams;
import java.util.List;
import java.util.Map;
public class PaymentMethod extends ApiResource implements HasId, MetadataStore {
@SerializedName("acss_debit")
AcssDebit acssDebit;
@SerializedName("afterpay_clearpay")
AfterpayClearpay afterpayClearpay;
@SerializedName("alipay")
Alipay alipay;
@SerializedName("au_becs_debit")
AuBecsDebit auBecsDebit;
@SerializedName("bacs_debit")
BacsDebit bacsDebit;
@SerializedName("bancontact")
Bancontact bancontact;
@SerializedName("billing_details")
BillingDetails billingDetails;
@SerializedName("boleto")
Boleto boleto;
@SerializedName("card")
Card card;
@SerializedName("card_present")
CardPresent cardPresent;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The ID of the Customer to which this PaymentMethod is saved. This will not be set when the
* PaymentMethod has not been saved to a Customer.
*/
@SerializedName("customer")
ExpandableField customer;
@SerializedName("eps")
Eps eps;
@SerializedName("fpx")
Fpx fpx;
@SerializedName("giropay")
Giropay giropay;
@SerializedName("grabpay")
Grabpay grabpay;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
@SerializedName("ideal")
Ideal ideal;
@SerializedName("interac_present")
InteracPresent interacPresent;
@SerializedName("klarna")
Klarna klarna;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code payment_method}.
*/
@SerializedName("object")
String object;
@SerializedName("oxxo")
Oxxo oxxo;
@SerializedName("p24")
P24 p24;
@SerializedName("sepa_debit")
SepaDebit sepaDebit;
@SerializedName("sofort")
Sofort sofort;
/**
* The type of the PaymentMethod. An additional hash is included on the PaymentMethod with a name
* matching this value. It contains additional information specific to the PaymentMethod type.
*
*
One of {@code acss_debit}, {@code afterpay_clearpay}, {@code alipay}, {@code au_becs_debit},
* {@code bacs_debit}, {@code bancontact}, {@code boleto}, {@code card}, {@code card_present},
* {@code eps}, {@code fpx}, {@code giropay}, {@code grabpay}, {@code ideal}, {@code
* interac_present}, {@code klarna}, {@code oxxo}, {@code p24}, {@code sepa_debit}, {@code
* sofort}, or {@code wechat_pay}.
*/
@SerializedName("type")
String type;
@SerializedName("wechat_pay")
WechatPay wechatPay;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Creates a PaymentMethod object. Read the Stripe.js
* reference to learn how to create PaymentMethods via Stripe.js.
*
* Instead of creating a PaymentMethod directly, we recommend using the PaymentIntents API to accept a
* payment immediately or the SetupIntent API to collect payment
* method details ahead of a future payment.
*/
public static PaymentMethod create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a PaymentMethod object. Read the Stripe.js
* reference to learn how to create PaymentMethods via Stripe.js.
*
* Instead of creating a PaymentMethod directly, we recommend using the PaymentIntents API to accept a
* payment immediately or the SetupIntent API to collect payment
* method details ahead of a future payment.
*/
public static PaymentMethod create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/payment_methods");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Creates a PaymentMethod object. Read the Stripe.js
* reference to learn how to create PaymentMethods via Stripe.js.
*
* Instead of creating a PaymentMethod directly, we recommend using the PaymentIntents API to accept a
* payment immediately or the SetupIntent API to collect payment
* method details ahead of a future payment.
*/
public static PaymentMethod create(PaymentMethodCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a PaymentMethod object. Read the Stripe.js
* reference to learn how to create PaymentMethods via Stripe.js.
*
*
Instead of creating a PaymentMethod directly, we recommend using the PaymentIntents API to accept a
* payment immediately or the SetupIntent API to collect payment
* method details ahead of a future payment.
*/
public static PaymentMethod create(PaymentMethodCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/payment_methods");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Retrieves a PaymentMethod object.
*/
public static PaymentMethod retrieve(String paymentMethod) throws StripeException {
return retrieve(paymentMethod, (Map) null, (RequestOptions) null);
}
/**
* Retrieves a PaymentMethod object.
*/
public static PaymentMethod retrieve(String paymentMethod, RequestOptions options) throws StripeException {
return retrieve(paymentMethod, (Map) null, options);
}
/**
* Retrieves a PaymentMethod object.
*/
public static PaymentMethod retrieve(String paymentMethod, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s", ApiResource.urlEncodeId(paymentMethod)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, PaymentMethod.class, options);
}
/**
* Retrieves a PaymentMethod object.
*/
public static PaymentMethod retrieve(String paymentMethod, PaymentMethodRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s", ApiResource.urlEncodeId(paymentMethod)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, PaymentMethod.class, options);
}
/**
* Updates a PaymentMethod object. A PaymentMethod must be attached a customer to be updated.
*/
@Override
public PaymentMethod update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a PaymentMethod object. A PaymentMethod must be attached a customer to be updated.
*/
@Override
public PaymentMethod update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Updates a PaymentMethod object. A PaymentMethod must be attached a customer to be updated.
*/
public PaymentMethod update(PaymentMethodUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates a PaymentMethod object. A PaymentMethod must be attached a customer to be updated.
*/
public PaymentMethod update(PaymentMethodUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Returns a list of PaymentMethods. For listing a customer’s payment methods, you should use List a Customer’s
* PaymentMethods
*/
public static PaymentMethodCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of PaymentMethods. For listing a customer’s payment methods, you should use List a Customer’s
* PaymentMethods
*/
public static PaymentMethodCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/payment_methods");
return ApiResource.requestCollection(url, params, PaymentMethodCollection.class, options);
}
/**
* Returns a list of PaymentMethods. For listing a customer’s payment methods, you should use List a Customer’s
* PaymentMethods
*/
public static PaymentMethodCollection list(PaymentMethodListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of PaymentMethods. For listing a customer’s payment methods, you should use List a Customer’s
* PaymentMethods
*/
public static PaymentMethodCollection list(PaymentMethodListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/payment_methods");
return ApiResource.requestCollection(url, params, PaymentMethodCollection.class, options);
}
/**
* Attaches a PaymentMethod object to a Customer.
*
* To attach a new PaymentMethod to a customer for future payments, we recommend you use a SetupIntent or a PaymentIntent with setup_future_usage.
* These approaches will perform any necessary steps to ensure that the PaymentMethod can be used
* in a future payment. Using the /v1/payment_methods/:id/attach
endpoint does not
* ensure that future payments can be made with the attached PaymentMethod. See Optimizing cards for
* future payments for more information about setting up future payments.
*
*
To use this PaymentMethod as the default for invoice or subscription payments, set
* invoice_settings.default_payment_method
, on the Customer to the
* PaymentMethod’s ID.
*/
public PaymentMethod attach(Map params) throws StripeException {
return attach(params, (RequestOptions) null);
}
/**
* Attaches a PaymentMethod object to a Customer.
*
* To attach a new PaymentMethod to a customer for future payments, we recommend you use a SetupIntent or a PaymentIntent with setup_future_usage.
* These approaches will perform any necessary steps to ensure that the PaymentMethod can be used
* in a future payment. Using the /v1/payment_methods/:id/attach
endpoint does not
* ensure that future payments can be made with the attached PaymentMethod. See Optimizing cards for
* future payments for more information about setting up future payments.
*
*
To use this PaymentMethod as the default for invoice or subscription payments, set
* invoice_settings.default_payment_method
, on the Customer to the
* PaymentMethod’s ID.
*/
public PaymentMethod attach(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s/attach", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Attaches a PaymentMethod object to a Customer.
*
* To attach a new PaymentMethod to a customer for future payments, we recommend you use a SetupIntent or a PaymentIntent with setup_future_usage.
* These approaches will perform any necessary steps to ensure that the PaymentMethod can be used
* in a future payment. Using the /v1/payment_methods/:id/attach
endpoint does not
* ensure that future payments can be made with the attached PaymentMethod. See Optimizing cards for
* future payments for more information about setting up future payments.
*
*
To use this PaymentMethod as the default for invoice or subscription payments, set
* invoice_settings.default_payment_method
, on the Customer to the
* PaymentMethod’s ID.
*/
public PaymentMethod attach(PaymentMethodAttachParams params) throws StripeException {
return attach(params, (RequestOptions) null);
}
/**
* Attaches a PaymentMethod object to a Customer.
*
*
To attach a new PaymentMethod to a customer for future payments, we recommend you use a SetupIntent or a PaymentIntent with setup_future_usage.
* These approaches will perform any necessary steps to ensure that the PaymentMethod can be used
* in a future payment. Using the /v1/payment_methods/:id/attach
endpoint does not
* ensure that future payments can be made with the attached PaymentMethod. See Optimizing cards for
* future payments for more information about setting up future payments.
*
*
To use this PaymentMethod as the default for invoice or subscription payments, set
* invoice_settings.default_payment_method
, on the Customer to the
* PaymentMethod’s ID.
*/
public PaymentMethod attach(PaymentMethodAttachParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s/attach", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Detaches a PaymentMethod object from a Customer.
*/
public PaymentMethod detach() throws StripeException {
return detach((Map) null, (RequestOptions) null);
}
/**
* Detaches a PaymentMethod object from a Customer.
*/
public PaymentMethod detach(RequestOptions options) throws StripeException {
return detach((Map) null, options);
}
/**
* Detaches a PaymentMethod object from a Customer.
*/
public PaymentMethod detach(Map params) throws StripeException {
return detach(params, (RequestOptions) null);
}
/**
* Detaches a PaymentMethod object from a Customer.
*/
public PaymentMethod detach(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s/detach", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
/**
* Detaches a PaymentMethod object from a Customer.
*/
public PaymentMethod detach(PaymentMethodDetachParams params) throws StripeException {
return detach(params, (RequestOptions) null);
}
/**
* Detaches a PaymentMethod object from a Customer.
*/
public PaymentMethod detach(PaymentMethodDetachParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/payment_methods/%s/detach", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, PaymentMethod.class, options);
}
public static class AcssDebit extends StripeObject {
/**
* Name of the bank associated with the bank account.
*/
@SerializedName("bank_name")
String bankName;
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@SerializedName("fingerprint")
String fingerprint;
/**
* Institution number of the bank account.
*/
@SerializedName("institution_number")
String institutionNumber;
/**
* Last four digits of the bank account number.
*/
@SerializedName("last4")
String last4;
/**
* Transit number of the bank account.
*/
@SerializedName("transit_number")
String transitNumber;
/**
* Name of the bank associated with the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBankName() {
return this.bankName;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFingerprint() {
return this.fingerprint;
}
/**
* Institution number of the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInstitutionNumber() {
return this.institutionNumber;
}
/**
* Last four digits of the bank account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLast4() {
return this.last4;
}
/**
* Transit number of the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTransitNumber() {
return this.transitNumber;
}
/**
* Name of the bank associated with the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankName(final String bankName) {
this.bankName = bankName;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFingerprint(final String fingerprint) {
this.fingerprint = fingerprint;
}
/**
* Institution number of the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInstitutionNumber(final String institutionNumber) {
this.institutionNumber = institutionNumber;
}
/**
* Last four digits of the bank account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLast4(final String last4) {
this.last4 = last4;
}
/**
* Transit number of the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransitNumber(final String transitNumber) {
this.transitNumber = transitNumber;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.AcssDebit)) return false;
final PaymentMethod.AcssDebit other = (PaymentMethod.AcssDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bankName = this.getBankName();
final java.lang.Object other$bankName = other.getBankName();
if (this$bankName == null ? other$bankName != null : !this$bankName.equals(other$bankName)) return false;
final java.lang.Object this$fingerprint = this.getFingerprint();
final java.lang.Object other$fingerprint = other.getFingerprint();
if (this$fingerprint == null ? other$fingerprint != null : !this$fingerprint.equals(other$fingerprint)) return false;
final java.lang.Object this$institutionNumber = this.getInstitutionNumber();
final java.lang.Object other$institutionNumber = other.getInstitutionNumber();
if (this$institutionNumber == null ? other$institutionNumber != null : !this$institutionNumber.equals(other$institutionNumber)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
final java.lang.Object this$transitNumber = this.getTransitNumber();
final java.lang.Object other$transitNumber = other.getTransitNumber();
if (this$transitNumber == null ? other$transitNumber != null : !this$transitNumber.equals(other$transitNumber)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.AcssDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bankName = this.getBankName();
result = result * PRIME + ($bankName == null ? 43 : $bankName.hashCode());
final java.lang.Object $fingerprint = this.getFingerprint();
result = result * PRIME + ($fingerprint == null ? 43 : $fingerprint.hashCode());
final java.lang.Object $institutionNumber = this.getInstitutionNumber();
result = result * PRIME + ($institutionNumber == null ? 43 : $institutionNumber.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
final java.lang.Object $transitNumber = this.getTransitNumber();
result = result * PRIME + ($transitNumber == null ? 43 : $transitNumber.hashCode());
return result;
}
}
public static class AfterpayClearpay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.AfterpayClearpay)) return false;
final PaymentMethod.AfterpayClearpay other = (PaymentMethod.AfterpayClearpay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.AfterpayClearpay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Alipay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Alipay)) return false;
final PaymentMethod.Alipay other = (PaymentMethod.Alipay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Alipay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class AuBecsDebit extends StripeObject {
/**
* Six-digit number identifying bank and branch associated with this bank account.
*/
@SerializedName("bsb_number")
String bsbNumber;
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@SerializedName("fingerprint")
String fingerprint;
/**
* Last four digits of the bank account number.
*/
@SerializedName("last4")
String last4;
/**
* Six-digit number identifying bank and branch associated with this bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBsbNumber() {
return this.bsbNumber;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFingerprint() {
return this.fingerprint;
}
/**
* Last four digits of the bank account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLast4() {
return this.last4;
}
/**
* Six-digit number identifying bank and branch associated with this bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBsbNumber(final String bsbNumber) {
this.bsbNumber = bsbNumber;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFingerprint(final String fingerprint) {
this.fingerprint = fingerprint;
}
/**
* Last four digits of the bank account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLast4(final String last4) {
this.last4 = last4;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.AuBecsDebit)) return false;
final PaymentMethod.AuBecsDebit other = (PaymentMethod.AuBecsDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bsbNumber = this.getBsbNumber();
final java.lang.Object other$bsbNumber = other.getBsbNumber();
if (this$bsbNumber == null ? other$bsbNumber != null : !this$bsbNumber.equals(other$bsbNumber)) return false;
final java.lang.Object this$fingerprint = this.getFingerprint();
final java.lang.Object other$fingerprint = other.getFingerprint();
if (this$fingerprint == null ? other$fingerprint != null : !this$fingerprint.equals(other$fingerprint)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.AuBecsDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bsbNumber = this.getBsbNumber();
result = result * PRIME + ($bsbNumber == null ? 43 : $bsbNumber.hashCode());
final java.lang.Object $fingerprint = this.getFingerprint();
result = result * PRIME + ($fingerprint == null ? 43 : $fingerprint.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
return result;
}
}
public static class BacsDebit extends StripeObject {
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@SerializedName("fingerprint")
String fingerprint;
/**
* Last four digits of the bank account number.
*/
@SerializedName("last4")
String last4;
/**
* Sort code of the bank account. (e.g., {@code 10-20-30})
*/
@SerializedName("sort_code")
String sortCode;
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFingerprint() {
return this.fingerprint;
}
/**
* Last four digits of the bank account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLast4() {
return this.last4;
}
/**
* Sort code of the bank account. (e.g., {@code 10-20-30})
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSortCode() {
return this.sortCode;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFingerprint(final String fingerprint) {
this.fingerprint = fingerprint;
}
/**
* Last four digits of the bank account number.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLast4(final String last4) {
this.last4 = last4;
}
/**
* Sort code of the bank account. (e.g., {@code 10-20-30})
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSortCode(final String sortCode) {
this.sortCode = sortCode;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.BacsDebit)) return false;
final PaymentMethod.BacsDebit other = (PaymentMethod.BacsDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$fingerprint = this.getFingerprint();
final java.lang.Object other$fingerprint = other.getFingerprint();
if (this$fingerprint == null ? other$fingerprint != null : !this$fingerprint.equals(other$fingerprint)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
final java.lang.Object this$sortCode = this.getSortCode();
final java.lang.Object other$sortCode = other.getSortCode();
if (this$sortCode == null ? other$sortCode != null : !this$sortCode.equals(other$sortCode)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.BacsDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $fingerprint = this.getFingerprint();
result = result * PRIME + ($fingerprint == null ? 43 : $fingerprint.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
final java.lang.Object $sortCode = this.getSortCode();
result = result * PRIME + ($sortCode == null ? 43 : $sortCode.hashCode());
return result;
}
}
public static class Bancontact extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Bancontact)) return false;
final PaymentMethod.Bancontact other = (PaymentMethod.Bancontact) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Bancontact;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class BillingDetails extends StripeObject {
/**
* Billing address.
*/
@SerializedName("address")
Address address;
/**
* Email address.
*/
@SerializedName("email")
String email;
/**
* Full name.
*/
@SerializedName("name")
String name;
/**
* Billing phone number (including extension).
*/
@SerializedName("phone")
String phone;
/**
* Billing address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
/**
* Email address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
/**
* Full name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* Billing phone number (including extension).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhone() {
return this.phone;
}
/**
* Billing address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddress(final Address address) {
this.address = address;
}
/**
* Email address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
/**
* Full name.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
/**
* Billing phone number (including extension).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPhone(final String phone) {
this.phone = phone;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.BillingDetails)) return false;
final PaymentMethod.BillingDetails other = (PaymentMethod.BillingDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$phone = this.getPhone();
final java.lang.Object other$phone = other.getPhone();
if (this$phone == null ? other$phone != null : !this$phone.equals(other$phone)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.BillingDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $phone = this.getPhone();
result = result * PRIME + ($phone == null ? 43 : $phone.hashCode());
return result;
}
}
public static class Boleto extends StripeObject {
/**
* Uniquely identifies the customer tax id (CNPJ or CPF).
*/
@SerializedName("tax_id")
String taxId;
/**
* Uniquely identifies the customer tax id (CNPJ or CPF).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTaxId() {
return this.taxId;
}
/**
* Uniquely identifies the customer tax id (CNPJ or CPF).
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxId(final String taxId) {
this.taxId = taxId;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Boleto)) return false;
final PaymentMethod.Boleto other = (PaymentMethod.Boleto) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$taxId = this.getTaxId();
final java.lang.Object other$taxId = other.getTaxId();
if (this$taxId == null ? other$taxId != null : !this$taxId.equals(other$taxId)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Boleto;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $taxId = this.getTaxId();
result = result * PRIME + ($taxId == null ? 43 : $taxId.hashCode());
return result;
}
}
public static class Card extends StripeObject {
/**
* Card brand. Can be {@code amex}, {@code diners}, {@code discover}, {@code jcb}, {@code
* mastercard}, {@code unionpay}, {@code visa}, or {@code unknown}.
*/
@SerializedName("brand")
String brand;
/**
* Checks on Card address and CVC if provided.
*/
@SerializedName("checks")
Checks checks;
/**
* Two-letter ISO code representing the country of the card. You could use this attribute to get
* a sense of the international breakdown of cards you've collected.
*/
@SerializedName("country")
String country;
/**
* A high-level description of the type of cards issued in this range. (For internal use only
* and not typically available in standard API requests.)
*/
@SerializedName("description")
String description;
/**
* Two-digit number representing the card's expiration month.
*/
@SerializedName("exp_month")
Long expMonth;
/**
* Four-digit number representing the card's expiration year.
*/
@SerializedName("exp_year")
Long expYear;
/**
* Uniquely identifies this particular card number. You can use this attribute to check whether
* two customers who’ve signed up with you are using the same card number, for example. For
* payment methods that tokenize card information (Apple Pay, Google Pay), the tokenized number
* might be provided instead of the underlying card number.
*
* Starting May 1, 2021, card fingerprint in India for Connect will change to allow two
* fingerprints for the same card --- one for India and one for the rest of the world.
*/
@SerializedName("fingerprint")
String fingerprint;
/**
* Card funding type. Can be {@code credit}, {@code debit}, {@code prepaid}, or {@code unknown}.
*/
@SerializedName("funding")
String funding;
/**
* Issuer identification number of the card. (For internal use only and not typically available
* in standard API requests.)
*/
@SerializedName("iin")
String iin;
/**
* The name of the card's issuing bank. (For internal use only and not typically available in
* standard API requests.)
*/
@SerializedName("issuer")
String issuer;
/**
* The last four digits of the card.
*/
@SerializedName("last4")
String last4;
/**
* Contains information about card networks that can be used to process the payment.
*/
@SerializedName("networks")
Networks networks;
/**
* Contains details on how this Card maybe be used for 3D Secure authentication.
*/
@SerializedName("three_d_secure_usage")
ThreeDSecureUsage threeDSecureUsage;
/**
* If this Card is part of a card wallet, this contains the details of the card wallet.
*/
@SerializedName("wallet")
Wallet wallet;
public static class Checks extends StripeObject {
/**
* If a address line1 was provided, results of the check, one of {@code pass}, {@code fail},
* {@code unavailable}, or {@code unchecked}.
*/
@SerializedName("address_line1_check")
String addressLine1Check;
/**
* If a address postal code was provided, results of the check, one of {@code pass}, {@code
* fail}, {@code unavailable}, or {@code unchecked}.
*/
@SerializedName("address_postal_code_check")
String addressPostalCodeCheck;
/**
* If a CVC was provided, results of the check, one of {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@SerializedName("cvc_check")
String cvcCheck;
/**
* If a address line1 was provided, results of the check, one of {@code pass}, {@code fail},
* {@code unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressLine1Check() {
return this.addressLine1Check;
}
/**
* If a address postal code was provided, results of the check, one of {@code pass}, {@code
* fail}, {@code unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddressPostalCodeCheck() {
return this.addressPostalCodeCheck;
}
/**
* If a CVC was provided, results of the check, one of {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCvcCheck() {
return this.cvcCheck;
}
/**
* If a address line1 was provided, results of the check, one of {@code pass}, {@code fail},
* {@code unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressLine1Check(final String addressLine1Check) {
this.addressLine1Check = addressLine1Check;
}
/**
* If a address postal code was provided, results of the check, one of {@code pass}, {@code
* fail}, {@code unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddressPostalCodeCheck(final String addressPostalCodeCheck) {
this.addressPostalCodeCheck = addressPostalCodeCheck;
}
/**
* If a CVC was provided, results of the check, one of {@code pass}, {@code fail}, {@code
* unavailable}, or {@code unchecked}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCvcCheck(final String cvcCheck) {
this.cvcCheck = cvcCheck;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Checks)) return false;
final PaymentMethod.Card.Checks other = (PaymentMethod.Card.Checks) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$addressLine1Check = this.getAddressLine1Check();
final java.lang.Object other$addressLine1Check = other.getAddressLine1Check();
if (this$addressLine1Check == null ? other$addressLine1Check != null : !this$addressLine1Check.equals(other$addressLine1Check)) return false;
final java.lang.Object this$addressPostalCodeCheck = this.getAddressPostalCodeCheck();
final java.lang.Object other$addressPostalCodeCheck = other.getAddressPostalCodeCheck();
if (this$addressPostalCodeCheck == null ? other$addressPostalCodeCheck != null : !this$addressPostalCodeCheck.equals(other$addressPostalCodeCheck)) return false;
final java.lang.Object this$cvcCheck = this.getCvcCheck();
final java.lang.Object other$cvcCheck = other.getCvcCheck();
if (this$cvcCheck == null ? other$cvcCheck != null : !this$cvcCheck.equals(other$cvcCheck)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Checks;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $addressLine1Check = this.getAddressLine1Check();
result = result * PRIME + ($addressLine1Check == null ? 43 : $addressLine1Check.hashCode());
final java.lang.Object $addressPostalCodeCheck = this.getAddressPostalCodeCheck();
result = result * PRIME + ($addressPostalCodeCheck == null ? 43 : $addressPostalCodeCheck.hashCode());
final java.lang.Object $cvcCheck = this.getCvcCheck();
result = result * PRIME + ($cvcCheck == null ? 43 : $cvcCheck.hashCode());
return result;
}
}
public static class Networks extends StripeObject {
/**
* All available networks for the card.
*/
@SerializedName("available")
List available;
/**
* The preferred network for the card.
*/
@SerializedName("preferred")
String preferred;
/**
* All available networks for the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAvailable() {
return this.available;
}
/**
* The preferred network for the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPreferred() {
return this.preferred;
}
/**
* All available networks for the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAvailable(final List available) {
this.available = available;
}
/**
* The preferred network for the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPreferred(final String preferred) {
this.preferred = preferred;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Networks)) return false;
final PaymentMethod.Card.Networks other = (PaymentMethod.Card.Networks) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$available = this.getAvailable();
final java.lang.Object other$available = other.getAvailable();
if (this$available == null ? other$available != null : !this$available.equals(other$available)) return false;
final java.lang.Object this$preferred = this.getPreferred();
final java.lang.Object other$preferred = other.getPreferred();
if (this$preferred == null ? other$preferred != null : !this$preferred.equals(other$preferred)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Networks;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $available = this.getAvailable();
result = result * PRIME + ($available == null ? 43 : $available.hashCode());
final java.lang.Object $preferred = this.getPreferred();
result = result * PRIME + ($preferred == null ? 43 : $preferred.hashCode());
return result;
}
}
public static class ThreeDSecureUsage extends StripeObject {
/**
* Whether 3D Secure is supported on this card.
*/
@SerializedName("supported")
Boolean supported;
/**
* Whether 3D Secure is supported on this card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getSupported() {
return this.supported;
}
/**
* Whether 3D Secure is supported on this card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSupported(final Boolean supported) {
this.supported = supported;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.ThreeDSecureUsage)) return false;
final PaymentMethod.Card.ThreeDSecureUsage other = (PaymentMethod.Card.ThreeDSecureUsage) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$supported = this.getSupported();
final java.lang.Object other$supported = other.getSupported();
if (this$supported == null ? other$supported != null : !this$supported.equals(other$supported)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.ThreeDSecureUsage;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $supported = this.getSupported();
result = result * PRIME + ($supported == null ? 43 : $supported.hashCode());
return result;
}
}
public static class Wallet extends StripeObject {
@SerializedName("amex_express_checkout")
AmexExpressCheckout amexExpressCheckout;
@SerializedName("apple_pay")
ApplePay applePay;
/** (For tokenized numbers only.) The last four digits of the device account number. */
@SerializedName("dynamic_last4")
String dynamicLast4;
@SerializedName("google_pay")
GooglePay googlePay;
@SerializedName("masterpass")
Masterpass masterpass;
@SerializedName("samsung_pay")
SamsungPay samsungPay;
/**
* The type of the card wallet, one of {@code amex_express_checkout}, {@code apple_pay},
* {@code google_pay}, {@code masterpass}, {@code samsung_pay}, or {@code visa_checkout}. An
* additional hash is included on the Wallet subhash with a name matching this value. It
* contains additional information specific to the card wallet type.
*/
@SerializedName("type")
String type;
@SerializedName("visa_checkout")
VisaCheckout visaCheckout;
public static class AmexExpressCheckout extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet.AmexExpressCheckout)) return false;
final PaymentMethod.Card.Wallet.AmexExpressCheckout other = (PaymentMethod.Card.Wallet.AmexExpressCheckout) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet.AmexExpressCheckout;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class ApplePay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet.ApplePay)) return false;
final PaymentMethod.Card.Wallet.ApplePay other = (PaymentMethod.Card.Wallet.ApplePay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet.ApplePay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class GooglePay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet.GooglePay)) return false;
final PaymentMethod.Card.Wallet.GooglePay other = (PaymentMethod.Card.Wallet.GooglePay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet.GooglePay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Masterpass extends StripeObject {
/**
* Owner's verified billing address. Values are verified or provided by the wallet directly
* (if supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("billing_address")
Address billingAddress;
/**
* Owner's verified email. Values are verified or provided by the wallet directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("email")
String email;
/**
* Owner's verified full name. Values are verified or provided by the wallet directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("name")
String name;
/**
* Owner's verified shipping address. Values are verified or provided by the wallet directly
* (if supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("shipping_address")
Address shippingAddress;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getBillingAddress() {
return this.billingAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getShippingAddress() {
return this.shippingAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingAddress(final Address billingAddress) {
this.billingAddress = billingAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippingAddress(final Address shippingAddress) {
this.shippingAddress = shippingAddress;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet.Masterpass)) return false;
final PaymentMethod.Card.Wallet.Masterpass other = (PaymentMethod.Card.Wallet.Masterpass) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$billingAddress = this.getBillingAddress();
final java.lang.Object other$billingAddress = other.getBillingAddress();
if (this$billingAddress == null ? other$billingAddress != null : !this$billingAddress.equals(other$billingAddress)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$shippingAddress = this.getShippingAddress();
final java.lang.Object other$shippingAddress = other.getShippingAddress();
if (this$shippingAddress == null ? other$shippingAddress != null : !this$shippingAddress.equals(other$shippingAddress)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet.Masterpass;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $billingAddress = this.getBillingAddress();
result = result * PRIME + ($billingAddress == null ? 43 : $billingAddress.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $shippingAddress = this.getShippingAddress();
result = result * PRIME + ($shippingAddress == null ? 43 : $shippingAddress.hashCode());
return result;
}
}
public static class SamsungPay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet.SamsungPay)) return false;
final PaymentMethod.Card.Wallet.SamsungPay other = (PaymentMethod.Card.Wallet.SamsungPay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet.SamsungPay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class VisaCheckout extends StripeObject {
/**
* Owner's verified billing address. Values are verified or provided by the wallet directly
* (if supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("billing_address")
Address billingAddress;
/**
* Owner's verified email. Values are verified or provided by the wallet directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("email")
String email;
/**
* Owner's verified full name. Values are verified or provided by the wallet directly (if
* supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
@SerializedName("name")
String name;
@SerializedName("shipping_address")
Address shippingAddress;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getBillingAddress() {
return this.billingAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getShippingAddress() {
return this.shippingAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingAddress(final Address billingAddress) {
this.billingAddress = billingAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippingAddress(final Address shippingAddress) {
this.shippingAddress = shippingAddress;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet.VisaCheckout)) return false;
final PaymentMethod.Card.Wallet.VisaCheckout other = (PaymentMethod.Card.Wallet.VisaCheckout) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$billingAddress = this.getBillingAddress();
final java.lang.Object other$billingAddress = other.getBillingAddress();
if (this$billingAddress == null ? other$billingAddress != null : !this$billingAddress.equals(other$billingAddress)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$shippingAddress = this.getShippingAddress();
final java.lang.Object other$shippingAddress = other.getShippingAddress();
if (this$shippingAddress == null ? other$shippingAddress != null : !this$shippingAddress.equals(other$shippingAddress)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet.VisaCheckout;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $billingAddress = this.getBillingAddress();
result = result * PRIME + ($billingAddress == null ? 43 : $billingAddress.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $shippingAddress = this.getShippingAddress();
result = result * PRIME + ($shippingAddress == null ? 43 : $shippingAddress.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AmexExpressCheckout getAmexExpressCheckout() {
return this.amexExpressCheckout;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ApplePay getApplePay() {
return this.applePay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDynamicLast4() {
return this.dynamicLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public GooglePay getGooglePay() {
return this.googlePay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Masterpass getMasterpass() {
return this.masterpass;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SamsungPay getSamsungPay() {
return this.samsungPay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public VisaCheckout getVisaCheckout() {
return this.visaCheckout;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmexExpressCheckout(final AmexExpressCheckout amexExpressCheckout) {
this.amexExpressCheckout = amexExpressCheckout;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplePay(final ApplePay applePay) {
this.applePay = applePay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDynamicLast4(final String dynamicLast4) {
this.dynamicLast4 = dynamicLast4;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setGooglePay(final GooglePay googlePay) {
this.googlePay = googlePay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMasterpass(final Masterpass masterpass) {
this.masterpass = masterpass;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSamsungPay(final SamsungPay samsungPay) {
this.samsungPay = samsungPay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVisaCheckout(final VisaCheckout visaCheckout) {
this.visaCheckout = visaCheckout;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card.Wallet)) return false;
final PaymentMethod.Card.Wallet other = (PaymentMethod.Card.Wallet) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amexExpressCheckout = this.getAmexExpressCheckout();
final java.lang.Object other$amexExpressCheckout = other.getAmexExpressCheckout();
if (this$amexExpressCheckout == null ? other$amexExpressCheckout != null : !this$amexExpressCheckout.equals(other$amexExpressCheckout)) return false;
final java.lang.Object this$applePay = this.getApplePay();
final java.lang.Object other$applePay = other.getApplePay();
if (this$applePay == null ? other$applePay != null : !this$applePay.equals(other$applePay)) return false;
final java.lang.Object this$dynamicLast4 = this.getDynamicLast4();
final java.lang.Object other$dynamicLast4 = other.getDynamicLast4();
if (this$dynamicLast4 == null ? other$dynamicLast4 != null : !this$dynamicLast4.equals(other$dynamicLast4)) return false;
final java.lang.Object this$googlePay = this.getGooglePay();
final java.lang.Object other$googlePay = other.getGooglePay();
if (this$googlePay == null ? other$googlePay != null : !this$googlePay.equals(other$googlePay)) return false;
final java.lang.Object this$masterpass = this.getMasterpass();
final java.lang.Object other$masterpass = other.getMasterpass();
if (this$masterpass == null ? other$masterpass != null : !this$masterpass.equals(other$masterpass)) return false;
final java.lang.Object this$samsungPay = this.getSamsungPay();
final java.lang.Object other$samsungPay = other.getSamsungPay();
if (this$samsungPay == null ? other$samsungPay != null : !this$samsungPay.equals(other$samsungPay)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$visaCheckout = this.getVisaCheckout();
final java.lang.Object other$visaCheckout = other.getVisaCheckout();
if (this$visaCheckout == null ? other$visaCheckout != null : !this$visaCheckout.equals(other$visaCheckout)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card.Wallet;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amexExpressCheckout = this.getAmexExpressCheckout();
result = result * PRIME + ($amexExpressCheckout == null ? 43 : $amexExpressCheckout.hashCode());
final java.lang.Object $applePay = this.getApplePay();
result = result * PRIME + ($applePay == null ? 43 : $applePay.hashCode());
final java.lang.Object $dynamicLast4 = this.getDynamicLast4();
result = result * PRIME + ($dynamicLast4 == null ? 43 : $dynamicLast4.hashCode());
final java.lang.Object $googlePay = this.getGooglePay();
result = result * PRIME + ($googlePay == null ? 43 : $googlePay.hashCode());
final java.lang.Object $masterpass = this.getMasterpass();
result = result * PRIME + ($masterpass == null ? 43 : $masterpass.hashCode());
final java.lang.Object $samsungPay = this.getSamsungPay();
result = result * PRIME + ($samsungPay == null ? 43 : $samsungPay.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $visaCheckout = this.getVisaCheckout();
result = result * PRIME + ($visaCheckout == null ? 43 : $visaCheckout.hashCode());
return result;
}
}
/**
* Card brand. Can be {@code amex}, {@code diners}, {@code discover}, {@code jcb}, {@code
* mastercard}, {@code unionpay}, {@code visa}, or {@code unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBrand() {
return this.brand;
}
/**
* Checks on Card address and CVC if provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Checks getChecks() {
return this.checks;
}
/**
* Two-letter ISO code representing the country of the card. You could use this attribute to get
* a sense of the international breakdown of cards you've collected.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* A high-level description of the type of cards issued in this range. (For internal use only
* and not typically available in standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* Two-digit number representing the card's expiration month.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpMonth() {
return this.expMonth;
}
/**
* Four-digit number representing the card's expiration year.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpYear() {
return this.expYear;
}
/**
* Uniquely identifies this particular card number. You can use this attribute to check whether
* two customers who’ve signed up with you are using the same card number, for example. For
* payment methods that tokenize card information (Apple Pay, Google Pay), the tokenized number
* might be provided instead of the underlying card number.
*
* Starting May 1, 2021, card fingerprint in India for Connect will change to allow two
* fingerprints for the same card --- one for India and one for the rest of the world.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFingerprint() {
return this.fingerprint;
}
/**
* Card funding type. Can be {@code credit}, {@code debit}, {@code prepaid}, or {@code unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFunding() {
return this.funding;
}
/**
* Issuer identification number of the card. (For internal use only and not typically available
* in standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIin() {
return this.iin;
}
/**
* The name of the card's issuing bank. (For internal use only and not typically available in
* standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getIssuer() {
return this.issuer;
}
/**
* The last four digits of the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLast4() {
return this.last4;
}
/**
* Contains information about card networks that can be used to process the payment.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Networks getNetworks() {
return this.networks;
}
/**
* Contains details on how this Card maybe be used for 3D Secure authentication.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ThreeDSecureUsage getThreeDSecureUsage() {
return this.threeDSecureUsage;
}
/**
* If this Card is part of a card wallet, this contains the details of the card wallet.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Wallet getWallet() {
return this.wallet;
}
/**
* Card brand. Can be {@code amex}, {@code diners}, {@code discover}, {@code jcb}, {@code
* mastercard}, {@code unionpay}, {@code visa}, or {@code unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBrand(final String brand) {
this.brand = brand;
}
/**
* Checks on Card address and CVC if provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setChecks(final Checks checks) {
this.checks = checks;
}
/**
* Two-letter ISO code representing the country of the card. You could use this attribute to get
* a sense of the international breakdown of cards you've collected.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
/**
* A high-level description of the type of cards issued in this range. (For internal use only
* and not typically available in standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
/**
* Two-digit number representing the card's expiration month.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpMonth(final Long expMonth) {
this.expMonth = expMonth;
}
/**
* Four-digit number representing the card's expiration year.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpYear(final Long expYear) {
this.expYear = expYear;
}
/**
* Uniquely identifies this particular card number. You can use this attribute to check whether
* two customers who’ve signed up with you are using the same card number, for example. For
* payment methods that tokenize card information (Apple Pay, Google Pay), the tokenized number
* might be provided instead of the underlying card number.
*
*
Starting May 1, 2021, card fingerprint in India for Connect will change to allow two
* fingerprints for the same card --- one for India and one for the rest of the world.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFingerprint(final String fingerprint) {
this.fingerprint = fingerprint;
}
/**
* Card funding type. Can be {@code credit}, {@code debit}, {@code prepaid}, or {@code unknown}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFunding(final String funding) {
this.funding = funding;
}
/**
* Issuer identification number of the card. (For internal use only and not typically available
* in standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIin(final String iin) {
this.iin = iin;
}
/**
* The name of the card's issuing bank. (For internal use only and not typically available in
* standard API requests.)
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIssuer(final String issuer) {
this.issuer = issuer;
}
/**
* The last four digits of the card.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLast4(final String last4) {
this.last4 = last4;
}
/**
* Contains information about card networks that can be used to process the payment.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNetworks(final Networks networks) {
this.networks = networks;
}
/**
* Contains details on how this Card maybe be used for 3D Secure authentication.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setThreeDSecureUsage(final ThreeDSecureUsage threeDSecureUsage) {
this.threeDSecureUsage = threeDSecureUsage;
}
/**
* If this Card is part of a card wallet, this contains the details of the card wallet.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWallet(final Wallet wallet) {
this.wallet = wallet;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Card)) return false;
final PaymentMethod.Card other = (PaymentMethod.Card) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$expMonth = this.getExpMonth();
final java.lang.Object other$expMonth = other.getExpMonth();
if (this$expMonth == null ? other$expMonth != null : !this$expMonth.equals(other$expMonth)) return false;
final java.lang.Object this$expYear = this.getExpYear();
final java.lang.Object other$expYear = other.getExpYear();
if (this$expYear == null ? other$expYear != null : !this$expYear.equals(other$expYear)) return false;
final java.lang.Object this$brand = this.getBrand();
final java.lang.Object other$brand = other.getBrand();
if (this$brand == null ? other$brand != null : !this$brand.equals(other$brand)) return false;
final java.lang.Object this$checks = this.getChecks();
final java.lang.Object other$checks = other.getChecks();
if (this$checks == null ? other$checks != null : !this$checks.equals(other$checks)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$fingerprint = this.getFingerprint();
final java.lang.Object other$fingerprint = other.getFingerprint();
if (this$fingerprint == null ? other$fingerprint != null : !this$fingerprint.equals(other$fingerprint)) return false;
final java.lang.Object this$funding = this.getFunding();
final java.lang.Object other$funding = other.getFunding();
if (this$funding == null ? other$funding != null : !this$funding.equals(other$funding)) return false;
final java.lang.Object this$iin = this.getIin();
final java.lang.Object other$iin = other.getIin();
if (this$iin == null ? other$iin != null : !this$iin.equals(other$iin)) return false;
final java.lang.Object this$issuer = this.getIssuer();
final java.lang.Object other$issuer = other.getIssuer();
if (this$issuer == null ? other$issuer != null : !this$issuer.equals(other$issuer)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
final java.lang.Object this$networks = this.getNetworks();
final java.lang.Object other$networks = other.getNetworks();
if (this$networks == null ? other$networks != null : !this$networks.equals(other$networks)) return false;
final java.lang.Object this$threeDSecureUsage = this.getThreeDSecureUsage();
final java.lang.Object other$threeDSecureUsage = other.getThreeDSecureUsage();
if (this$threeDSecureUsage == null ? other$threeDSecureUsage != null : !this$threeDSecureUsage.equals(other$threeDSecureUsage)) return false;
final java.lang.Object this$wallet = this.getWallet();
final java.lang.Object other$wallet = other.getWallet();
if (this$wallet == null ? other$wallet != null : !this$wallet.equals(other$wallet)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Card;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $expMonth = this.getExpMonth();
result = result * PRIME + ($expMonth == null ? 43 : $expMonth.hashCode());
final java.lang.Object $expYear = this.getExpYear();
result = result * PRIME + ($expYear == null ? 43 : $expYear.hashCode());
final java.lang.Object $brand = this.getBrand();
result = result * PRIME + ($brand == null ? 43 : $brand.hashCode());
final java.lang.Object $checks = this.getChecks();
result = result * PRIME + ($checks == null ? 43 : $checks.hashCode());
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $fingerprint = this.getFingerprint();
result = result * PRIME + ($fingerprint == null ? 43 : $fingerprint.hashCode());
final java.lang.Object $funding = this.getFunding();
result = result * PRIME + ($funding == null ? 43 : $funding.hashCode());
final java.lang.Object $iin = this.getIin();
result = result * PRIME + ($iin == null ? 43 : $iin.hashCode());
final java.lang.Object $issuer = this.getIssuer();
result = result * PRIME + ($issuer == null ? 43 : $issuer.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
final java.lang.Object $networks = this.getNetworks();
result = result * PRIME + ($networks == null ? 43 : $networks.hashCode());
final java.lang.Object $threeDSecureUsage = this.getThreeDSecureUsage();
result = result * PRIME + ($threeDSecureUsage == null ? 43 : $threeDSecureUsage.hashCode());
final java.lang.Object $wallet = this.getWallet();
result = result * PRIME + ($wallet == null ? 43 : $wallet.hashCode());
return result;
}
}
/**
* Owner's verified shipping address. Values are verified or provided by the wallet directly
* (if supported) at the time of authorization or settlement. They cannot be set or mutated.
*/
public static class CardPresent extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.CardPresent)) return false;
final PaymentMethod.CardPresent other = (PaymentMethod.CardPresent) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.CardPresent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Eps extends StripeObject {
/**
* The customer's bank. Should be one of {@code arzte_und_apotheker_bank}, {@code
* austrian_anadi_bank_ag}, {@code bank_austria}, {@code bankhaus_carl_spangler}, {@code
* bankhaus_schelhammer_und_schattera_ag}, {@code bawag_psk_ag}, {@code bks_bank_ag}, {@code
* brull_kallmus_bank_ag}, {@code btv_vier_lander_bank}, {@code capital_bank_grawe_gruppe_ag},
* {@code dolomitenbank}, {@code easybank_ag}, {@code erste_bank_und_sparkassen}, {@code
* hypo_alpeadriabank_international_ag}, {@code hypo_noe_lb_fur_niederosterreich_u_wien}, {@code
* hypo_oberosterreich_salzburg_steiermark}, {@code hypo_tirol_bank_ag}, {@code
* hypo_vorarlberg_bank_ag}, {@code hypo_bank_burgenland_aktiengesellschaft}, {@code
* marchfelder_bank}, {@code oberbank_ag}, {@code raiffeisen_bankengruppe_osterreich}, {@code
* schoellerbank_ag}, {@code sparda_bank_wien}, {@code volksbank_gruppe}, {@code
* volkskreditbank_ag}, or {@code vr_bank_braunau}.
*/
@SerializedName("bank")
String bank;
/**
* The customer's bank. Should be one of {@code arzte_und_apotheker_bank}, {@code
* austrian_anadi_bank_ag}, {@code bank_austria}, {@code bankhaus_carl_spangler}, {@code
* bankhaus_schelhammer_und_schattera_ag}, {@code bawag_psk_ag}, {@code bks_bank_ag}, {@code
* brull_kallmus_bank_ag}, {@code btv_vier_lander_bank}, {@code capital_bank_grawe_gruppe_ag},
* {@code dolomitenbank}, {@code easybank_ag}, {@code erste_bank_und_sparkassen}, {@code
* hypo_alpeadriabank_international_ag}, {@code hypo_noe_lb_fur_niederosterreich_u_wien}, {@code
* hypo_oberosterreich_salzburg_steiermark}, {@code hypo_tirol_bank_ag}, {@code
* hypo_vorarlberg_bank_ag}, {@code hypo_bank_burgenland_aktiengesellschaft}, {@code
* marchfelder_bank}, {@code oberbank_ag}, {@code raiffeisen_bankengruppe_osterreich}, {@code
* schoellerbank_ag}, {@code sparda_bank_wien}, {@code volksbank_gruppe}, {@code
* volkskreditbank_ag}, or {@code vr_bank_braunau}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBank() {
return this.bank;
}
/**
* The customer's bank. Should be one of {@code arzte_und_apotheker_bank}, {@code
* austrian_anadi_bank_ag}, {@code bank_austria}, {@code bankhaus_carl_spangler}, {@code
* bankhaus_schelhammer_und_schattera_ag}, {@code bawag_psk_ag}, {@code bks_bank_ag}, {@code
* brull_kallmus_bank_ag}, {@code btv_vier_lander_bank}, {@code capital_bank_grawe_gruppe_ag},
* {@code dolomitenbank}, {@code easybank_ag}, {@code erste_bank_und_sparkassen}, {@code
* hypo_alpeadriabank_international_ag}, {@code hypo_noe_lb_fur_niederosterreich_u_wien}, {@code
* hypo_oberosterreich_salzburg_steiermark}, {@code hypo_tirol_bank_ag}, {@code
* hypo_vorarlberg_bank_ag}, {@code hypo_bank_burgenland_aktiengesellschaft}, {@code
* marchfelder_bank}, {@code oberbank_ag}, {@code raiffeisen_bankengruppe_osterreich}, {@code
* schoellerbank_ag}, {@code sparda_bank_wien}, {@code volksbank_gruppe}, {@code
* volkskreditbank_ag}, or {@code vr_bank_braunau}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBank(final String bank) {
this.bank = bank;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Eps)) return false;
final PaymentMethod.Eps other = (PaymentMethod.Eps) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bank = this.getBank();
final java.lang.Object other$bank = other.getBank();
if (this$bank == null ? other$bank != null : !this$bank.equals(other$bank)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Eps;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bank = this.getBank();
result = result * PRIME + ($bank == null ? 43 : $bank.hashCode());
return result;
}
}
public static class Fpx extends StripeObject {
/**
* Account holder type, if provided. Can be one of {@code individual} or {@code company}.
*/
@SerializedName("account_holder_type")
String accountHolderType;
/**
* The customer's bank, if provided. Can be one of {@code affin_bank}, {@code agrobank}, {@code
* alliance_bank}, {@code ambank}, {@code bank_islam}, {@code bank_muamalat}, {@code
* bank_rakyat}, {@code bsn}, {@code cimb}, {@code hong_leong_bank}, {@code hsbc}, {@code kfh},
* {@code maybank2u}, {@code ocbc}, {@code public_bank}, {@code rhb}, {@code
* standard_chartered}, {@code uob}, {@code deutsche_bank}, {@code maybank2e}, or {@code
* pb_enterprise}.
*/
@SerializedName("bank")
String bank;
/**
* Account holder type, if provided. Can be one of {@code individual} or {@code company}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAccountHolderType() {
return this.accountHolderType;
}
/**
* The customer's bank, if provided. Can be one of {@code affin_bank}, {@code agrobank}, {@code
* alliance_bank}, {@code ambank}, {@code bank_islam}, {@code bank_muamalat}, {@code
* bank_rakyat}, {@code bsn}, {@code cimb}, {@code hong_leong_bank}, {@code hsbc}, {@code kfh},
* {@code maybank2u}, {@code ocbc}, {@code public_bank}, {@code rhb}, {@code
* standard_chartered}, {@code uob}, {@code deutsche_bank}, {@code maybank2e}, or {@code
* pb_enterprise}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBank() {
return this.bank;
}
/**
* Account holder type, if provided. Can be one of {@code individual} or {@code company}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccountHolderType(final String accountHolderType) {
this.accountHolderType = accountHolderType;
}
/**
* The customer's bank, if provided. Can be one of {@code affin_bank}, {@code agrobank}, {@code
* alliance_bank}, {@code ambank}, {@code bank_islam}, {@code bank_muamalat}, {@code
* bank_rakyat}, {@code bsn}, {@code cimb}, {@code hong_leong_bank}, {@code hsbc}, {@code kfh},
* {@code maybank2u}, {@code ocbc}, {@code public_bank}, {@code rhb}, {@code
* standard_chartered}, {@code uob}, {@code deutsche_bank}, {@code maybank2e}, or {@code
* pb_enterprise}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBank(final String bank) {
this.bank = bank;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Fpx)) return false;
final PaymentMethod.Fpx other = (PaymentMethod.Fpx) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$accountHolderType = this.getAccountHolderType();
final java.lang.Object other$accountHolderType = other.getAccountHolderType();
if (this$accountHolderType == null ? other$accountHolderType != null : !this$accountHolderType.equals(other$accountHolderType)) return false;
final java.lang.Object this$bank = this.getBank();
final java.lang.Object other$bank = other.getBank();
if (this$bank == null ? other$bank != null : !this$bank.equals(other$bank)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Fpx;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $accountHolderType = this.getAccountHolderType();
result = result * PRIME + ($accountHolderType == null ? 43 : $accountHolderType.hashCode());
final java.lang.Object $bank = this.getBank();
result = result * PRIME + ($bank == null ? 43 : $bank.hashCode());
return result;
}
}
public static class Giropay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Giropay)) return false;
final PaymentMethod.Giropay other = (PaymentMethod.Giropay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Giropay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Grabpay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Grabpay)) return false;
final PaymentMethod.Grabpay other = (PaymentMethod.Grabpay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Grabpay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Ideal extends StripeObject {
/**
* The customer's bank, if provided. Can be one of {@code abn_amro}, {@code asn_bank}, {@code
* bunq}, {@code handelsbanken}, {@code ing}, {@code knab}, {@code moneyou}, {@code rabobank},
* {@code regiobank}, {@code revolut}, {@code sns_bank}, {@code triodos_bank}, or {@code
* van_lanschot}.
*/
@SerializedName("bank")
String bank;
/**
* The Bank Identifier Code of the customer's bank, if the bank was provided.
*
*
One of {@code ABNANL2A}, {@code ASNBNL21}, {@code BUNQNL2A}, {@code FVLBNL22}, {@code
* HANDNL2A}, {@code INGBNL2A}, {@code KNABNL2H}, {@code MOYONL21}, {@code RABONL2U}, {@code
* RBRBNL21}, {@code REVOLT21}, {@code SNSBNL2A}, or {@code TRIONL2U}.
*/
@SerializedName("bic")
String bic;
/**
* The customer's bank, if provided. Can be one of {@code abn_amro}, {@code asn_bank}, {@code
* bunq}, {@code handelsbanken}, {@code ing}, {@code knab}, {@code moneyou}, {@code rabobank},
* {@code regiobank}, {@code revolut}, {@code sns_bank}, {@code triodos_bank}, or {@code
* van_lanschot}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBank() {
return this.bank;
}
/**
* The Bank Identifier Code of the customer's bank, if the bank was provided.
*
*
One of {@code ABNANL2A}, {@code ASNBNL21}, {@code BUNQNL2A}, {@code FVLBNL22}, {@code
* HANDNL2A}, {@code INGBNL2A}, {@code KNABNL2H}, {@code MOYONL21}, {@code RABONL2U}, {@code
* RBRBNL21}, {@code REVOLT21}, {@code SNSBNL2A}, or {@code TRIONL2U}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBic() {
return this.bic;
}
/**
* The customer's bank, if provided. Can be one of {@code abn_amro}, {@code asn_bank}, {@code
* bunq}, {@code handelsbanken}, {@code ing}, {@code knab}, {@code moneyou}, {@code rabobank},
* {@code regiobank}, {@code revolut}, {@code sns_bank}, {@code triodos_bank}, or {@code
* van_lanschot}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBank(final String bank) {
this.bank = bank;
}
/**
* The Bank Identifier Code of the customer's bank, if the bank was provided.
*
*
One of {@code ABNANL2A}, {@code ASNBNL21}, {@code BUNQNL2A}, {@code FVLBNL22}, {@code
* HANDNL2A}, {@code INGBNL2A}, {@code KNABNL2H}, {@code MOYONL21}, {@code RABONL2U}, {@code
* RBRBNL21}, {@code REVOLT21}, {@code SNSBNL2A}, or {@code TRIONL2U}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBic(final String bic) {
this.bic = bic;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Ideal)) return false;
final PaymentMethod.Ideal other = (PaymentMethod.Ideal) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bank = this.getBank();
final java.lang.Object other$bank = other.getBank();
if (this$bank == null ? other$bank != null : !this$bank.equals(other$bank)) return false;
final java.lang.Object this$bic = this.getBic();
final java.lang.Object other$bic = other.getBic();
if (this$bic == null ? other$bic != null : !this$bic.equals(other$bic)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Ideal;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bank = this.getBank();
result = result * PRIME + ($bank == null ? 43 : $bank.hashCode());
final java.lang.Object $bic = this.getBic();
result = result * PRIME + ($bic == null ? 43 : $bic.hashCode());
return result;
}
}
public static class InteracPresent extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.InteracPresent)) return false;
final PaymentMethod.InteracPresent other = (PaymentMethod.InteracPresent) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.InteracPresent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class Klarna extends StripeObject {
/**
* The customer's date of birth, if provided.
*/
@SerializedName("dob")
DateOfBirth dob;
public static class DateOfBirth extends StripeObject {
/** The day of birth, between 1 and 31. */
@SerializedName("day")
Long day;
/** The month of birth, between 1 and 12. */
@SerializedName("month")
Long month;
@SerializedName("year")
Long year;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDay() {
return this.day;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMonth() {
return this.month;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getYear() {
return this.year;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDay(final Long day) {
this.day = day;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMonth(final Long month) {
this.month = month;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setYear(final Long year) {
this.year = year;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Klarna.DateOfBirth)) return false;
final PaymentMethod.Klarna.DateOfBirth other = (PaymentMethod.Klarna.DateOfBirth) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$day = this.getDay();
final java.lang.Object other$day = other.getDay();
if (this$day == null ? other$day != null : !this$day.equals(other$day)) return false;
final java.lang.Object this$month = this.getMonth();
final java.lang.Object other$month = other.getMonth();
if (this$month == null ? other$month != null : !this$month.equals(other$month)) return false;
final java.lang.Object this$year = this.getYear();
final java.lang.Object other$year = other.getYear();
if (this$year == null ? other$year != null : !this$year.equals(other$year)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Klarna.DateOfBirth;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $day = this.getDay();
result = result * PRIME + ($day == null ? 43 : $day.hashCode());
final java.lang.Object $month = this.getMonth();
result = result * PRIME + ($month == null ? 43 : $month.hashCode());
final java.lang.Object $year = this.getYear();
result = result * PRIME + ($year == null ? 43 : $year.hashCode());
return result;
}
}
/**
* The customer's date of birth, if provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public DateOfBirth getDob() {
return this.dob;
}
/**
* The customer's date of birth, if provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDob(final DateOfBirth dob) {
this.dob = dob;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Klarna)) return false;
final PaymentMethod.Klarna other = (PaymentMethod.Klarna) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$dob = this.getDob();
final java.lang.Object other$dob = other.getDob();
if (this$dob == null ? other$dob != null : !this$dob.equals(other$dob)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Klarna;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $dob = this.getDob();
result = result * PRIME + ($dob == null ? 43 : $dob.hashCode());
return result;
}
}
/**
* The four-digit year of birth.
*/
public static class Oxxo extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Oxxo)) return false;
final PaymentMethod.Oxxo other = (PaymentMethod.Oxxo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Oxxo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
public static class P24 extends StripeObject {
/**
* The customer's bank, if provided.
*
*
One of {@code alior_bank}, {@code bank_millennium}, {@code bank_nowy_bfg_sa}, {@code
* bank_pekao_sa}, {@code banki_spbdzielcze}, {@code blik}, {@code bnp_paribas}, {@code boz},
* {@code citi_handlowy}, {@code credit_agricole}, {@code envelobank}, {@code
* etransfer_pocztowy24}, {@code getin_bank}, {@code ideabank}, {@code ing}, {@code inteligo},
* {@code mbank_mtransfer}, {@code nest_przelew}, {@code noble_pay}, {@code pbac_z_ipko}, {@code
* plus_bank}, {@code santander_przelew24}, {@code tmobile_usbugi_bankowe}, {@code toyota_bank},
* or {@code volkswagen_bank}.
*/
@SerializedName("bank")
String bank;
/**
* The customer's bank, if provided.
*
*
One of {@code alior_bank}, {@code bank_millennium}, {@code bank_nowy_bfg_sa}, {@code
* bank_pekao_sa}, {@code banki_spbdzielcze}, {@code blik}, {@code bnp_paribas}, {@code boz},
* {@code citi_handlowy}, {@code credit_agricole}, {@code envelobank}, {@code
* etransfer_pocztowy24}, {@code getin_bank}, {@code ideabank}, {@code ing}, {@code inteligo},
* {@code mbank_mtransfer}, {@code nest_przelew}, {@code noble_pay}, {@code pbac_z_ipko}, {@code
* plus_bank}, {@code santander_przelew24}, {@code tmobile_usbugi_bankowe}, {@code toyota_bank},
* or {@code volkswagen_bank}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBank() {
return this.bank;
}
/**
* The customer's bank, if provided.
*
*
One of {@code alior_bank}, {@code bank_millennium}, {@code bank_nowy_bfg_sa}, {@code
* bank_pekao_sa}, {@code banki_spbdzielcze}, {@code blik}, {@code bnp_paribas}, {@code boz},
* {@code citi_handlowy}, {@code credit_agricole}, {@code envelobank}, {@code
* etransfer_pocztowy24}, {@code getin_bank}, {@code ideabank}, {@code ing}, {@code inteligo},
* {@code mbank_mtransfer}, {@code nest_przelew}, {@code noble_pay}, {@code pbac_z_ipko}, {@code
* plus_bank}, {@code santander_przelew24}, {@code tmobile_usbugi_bankowe}, {@code toyota_bank},
* or {@code volkswagen_bank}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBank(final String bank) {
this.bank = bank;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.P24)) return false;
final PaymentMethod.P24 other = (PaymentMethod.P24) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bank = this.getBank();
final java.lang.Object other$bank = other.getBank();
if (this$bank == null ? other$bank != null : !this$bank.equals(other$bank)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.P24;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bank = this.getBank();
result = result * PRIME + ($bank == null ? 43 : $bank.hashCode());
return result;
}
}
public static class SepaDebit extends StripeObject {
/**
* Bank code of bank associated with the bank account.
*/
@SerializedName("bank_code")
String bankCode;
/**
* Branch code of bank associated with the bank account.
*/
@SerializedName("branch_code")
String branchCode;
/**
* Two-letter ISO code representing the country the bank account is located in.
*/
@SerializedName("country")
String country;
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@SerializedName("fingerprint")
String fingerprint;
/**
* Information about the object that generated this PaymentMethod.
*/
@SerializedName("generated_from")
GeneratedFrom generatedFrom;
/**
* Last four characters of the IBAN.
*/
@SerializedName("last4")
String last4;
public static class GeneratedFrom extends StripeObject {
/** The ID of the Charge that generated this PaymentMethod, if any. */
@SerializedName("charge")
ExpandableField charge;
/** The ID of the SetupAttempt that generated this PaymentMethod, if any. */
@SerializedName("setup_attempt")
ExpandableField setupAttempt;
/** Get ID of expandable {@code charge} object. */
public String getCharge() {
return (this.charge != null) ? this.charge.getId() : null;
}
public void setCharge(String id) {
this.charge = ApiResource.setExpandableFieldId(id, this.charge);
}
/** Get expanded {@code charge}. */
public Charge getChargeObject() {
return (this.charge != null) ? this.charge.getExpanded() : null;
}
public void setChargeObject(Charge expandableObject) {
this.charge = new ExpandableField(expandableObject.getId(), expandableObject);
}
/** Get ID of expandable {@code setupAttempt} object. */
public String getSetupAttempt() {
return (this.setupAttempt != null) ? this.setupAttempt.getId() : null;
}
public void setSetupAttempt(String id) {
this.setupAttempt = ApiResource.setExpandableFieldId(id, this.setupAttempt);
}
public SetupAttempt getSetupAttemptObject() {
return (this.setupAttempt != null) ? this.setupAttempt.getExpanded() : null;
}
public void setSetupAttemptObject(SetupAttempt expandableObject) {
this.setupAttempt = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.SepaDebit.GeneratedFrom)) return false;
final PaymentMethod.SepaDebit.GeneratedFrom other = (PaymentMethod.SepaDebit.GeneratedFrom) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$charge = this.getCharge();
final java.lang.Object other$charge = other.getCharge();
if (this$charge == null ? other$charge != null : !this$charge.equals(other$charge)) return false;
final java.lang.Object this$setupAttempt = this.getSetupAttempt();
final java.lang.Object other$setupAttempt = other.getSetupAttempt();
if (this$setupAttempt == null ? other$setupAttempt != null : !this$setupAttempt.equals(other$setupAttempt)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.SepaDebit.GeneratedFrom;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $charge = this.getCharge();
result = result * PRIME + ($charge == null ? 43 : $charge.hashCode());
final java.lang.Object $setupAttempt = this.getSetupAttempt();
result = result * PRIME + ($setupAttempt == null ? 43 : $setupAttempt.hashCode());
return result;
}
}
/**
* Bank code of bank associated with the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBankCode() {
return this.bankCode;
}
/**
* Branch code of bank associated with the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBranchCode() {
return this.branchCode;
}
/**
* Two-letter ISO code representing the country the bank account is located in.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFingerprint() {
return this.fingerprint;
}
/**
* Information about the object that generated this PaymentMethod.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public GeneratedFrom getGeneratedFrom() {
return this.generatedFrom;
}
/**
* Last four characters of the IBAN.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLast4() {
return this.last4;
}
/**
* Bank code of bank associated with the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankCode(final String bankCode) {
this.bankCode = bankCode;
}
/**
* Branch code of bank associated with the bank account.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBranchCode(final String branchCode) {
this.branchCode = branchCode;
}
/**
* Two-letter ISO code representing the country the bank account is located in.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
/**
* Uniquely identifies this particular bank account. You can use this attribute to check whether
* two bank accounts are the same.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFingerprint(final String fingerprint) {
this.fingerprint = fingerprint;
}
/**
* Information about the object that generated this PaymentMethod.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setGeneratedFrom(final GeneratedFrom generatedFrom) {
this.generatedFrom = generatedFrom;
}
/**
* Last four characters of the IBAN.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLast4(final String last4) {
this.last4 = last4;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.SepaDebit)) return false;
final PaymentMethod.SepaDebit other = (PaymentMethod.SepaDebit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$bankCode = this.getBankCode();
final java.lang.Object other$bankCode = other.getBankCode();
if (this$bankCode == null ? other$bankCode != null : !this$bankCode.equals(other$bankCode)) return false;
final java.lang.Object this$branchCode = this.getBranchCode();
final java.lang.Object other$branchCode = other.getBranchCode();
if (this$branchCode == null ? other$branchCode != null : !this$branchCode.equals(other$branchCode)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$fingerprint = this.getFingerprint();
final java.lang.Object other$fingerprint = other.getFingerprint();
if (this$fingerprint == null ? other$fingerprint != null : !this$fingerprint.equals(other$fingerprint)) return false;
final java.lang.Object this$generatedFrom = this.getGeneratedFrom();
final java.lang.Object other$generatedFrom = other.getGeneratedFrom();
if (this$generatedFrom == null ? other$generatedFrom != null : !this$generatedFrom.equals(other$generatedFrom)) return false;
final java.lang.Object this$last4 = this.getLast4();
final java.lang.Object other$last4 = other.getLast4();
if (this$last4 == null ? other$last4 != null : !this$last4.equals(other$last4)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.SepaDebit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $bankCode = this.getBankCode();
result = result * PRIME + ($bankCode == null ? 43 : $bankCode.hashCode());
final java.lang.Object $branchCode = this.getBranchCode();
result = result * PRIME + ($branchCode == null ? 43 : $branchCode.hashCode());
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $fingerprint = this.getFingerprint();
result = result * PRIME + ($fingerprint == null ? 43 : $fingerprint.hashCode());
final java.lang.Object $generatedFrom = this.getGeneratedFrom();
result = result * PRIME + ($generatedFrom == null ? 43 : $generatedFrom.hashCode());
final java.lang.Object $last4 = this.getLast4();
result = result * PRIME + ($last4 == null ? 43 : $last4.hashCode());
return result;
}
}
/**
* Get expanded {@code setupAttempt}.
*/
public static class Sofort extends StripeObject {
/**
* Two-letter ISO code representing the country the bank account is located in.
*/
@SerializedName("country")
String country;
/**
* Two-letter ISO code representing the country the bank account is located in.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
/**
* Two-letter ISO code representing the country the bank account is located in.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.Sofort)) return false;
final PaymentMethod.Sofort other = (PaymentMethod.Sofort) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.Sofort;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
return result;
}
}
public static class WechatPay extends StripeObject {
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod.WechatPay)) return false;
final PaymentMethod.WechatPay other = (PaymentMethod.WechatPay) o;
if (!other.canEqual((java.lang.Object) this)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod.WechatPay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int result = 1;
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AcssDebit getAcssDebit() {
return this.acssDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AfterpayClearpay getAfterpayClearpay() {
return this.afterpayClearpay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Alipay getAlipay() {
return this.alipay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AuBecsDebit getAuBecsDebit() {
return this.auBecsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BacsDebit getBacsDebit() {
return this.bacsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Bancontact getBancontact() {
return this.bancontact;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BillingDetails getBillingDetails() {
return this.billingDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boleto getBoleto() {
return this.boleto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CardPresent getCardPresent() {
return this.cardPresent;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Eps getEps() {
return this.eps;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Fpx getFpx() {
return this.fpx;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Giropay getGiropay() {
return this.giropay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Grabpay getGrabpay() {
return this.grabpay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Ideal getIdeal() {
return this.ideal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InteracPresent getInteracPresent() {
return this.interacPresent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Klarna getKlarna() {
return this.klarna;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code payment_method}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Oxxo getOxxo() {
return this.oxxo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public P24 getP24() {
return this.p24;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SepaDebit getSepaDebit() {
return this.sepaDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Sofort getSofort() {
return this.sofort;
}
/**
* The type of the PaymentMethod. An additional hash is included on the PaymentMethod with a name
* matching this value. It contains additional information specific to the PaymentMethod type.
*
*
One of {@code acss_debit}, {@code afterpay_clearpay}, {@code alipay}, {@code au_becs_debit},
* {@code bacs_debit}, {@code bancontact}, {@code boleto}, {@code card}, {@code card_present},
* {@code eps}, {@code fpx}, {@code giropay}, {@code grabpay}, {@code ideal}, {@code
* interac_present}, {@code klarna}, {@code oxxo}, {@code p24}, {@code sepa_debit}, {@code
* sofort}, or {@code wechat_pay}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public WechatPay getWechatPay() {
return this.wechatPay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAcssDebit(final AcssDebit acssDebit) {
this.acssDebit = acssDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAfterpayClearpay(final AfterpayClearpay afterpayClearpay) {
this.afterpayClearpay = afterpayClearpay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAlipay(final Alipay alipay) {
this.alipay = alipay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuBecsDebit(final AuBecsDebit auBecsDebit) {
this.auBecsDebit = auBecsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBacsDebit(final BacsDebit bacsDebit) {
this.bacsDebit = bacsDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBancontact(final Bancontact bancontact) {
this.bancontact = bancontact;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingDetails(final BillingDetails billingDetails) {
this.billingDetails = billingDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBoleto(final Boleto boleto) {
this.boleto = boleto;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Card card) {
this.card = card;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCardPresent(final CardPresent cardPresent) {
this.cardPresent = cardPresent;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEps(final Eps eps) {
this.eps = eps;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFpx(final Fpx fpx) {
this.fpx = fpx;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setGiropay(final Giropay giropay) {
this.giropay = giropay;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setGrabpay(final Grabpay grabpay) {
this.grabpay = grabpay;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIdeal(final Ideal ideal) {
this.ideal = ideal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInteracPresent(final InteracPresent interacPresent) {
this.interacPresent = interacPresent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setKlarna(final Klarna klarna) {
this.klarna = klarna;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code payment_method}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOxxo(final Oxxo oxxo) {
this.oxxo = oxxo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setP24(final P24 p24) {
this.p24 = p24;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSepaDebit(final SepaDebit sepaDebit) {
this.sepaDebit = sepaDebit;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSofort(final Sofort sofort) {
this.sofort = sofort;
}
/**
* The type of the PaymentMethod. An additional hash is included on the PaymentMethod with a name
* matching this value. It contains additional information specific to the PaymentMethod type.
*
*
One of {@code acss_debit}, {@code afterpay_clearpay}, {@code alipay}, {@code au_becs_debit},
* {@code bacs_debit}, {@code bancontact}, {@code boleto}, {@code card}, {@code card_present},
* {@code eps}, {@code fpx}, {@code giropay}, {@code grabpay}, {@code ideal}, {@code
* interac_present}, {@code klarna}, {@code oxxo}, {@code p24}, {@code sepa_debit}, {@code
* sofort}, or {@code wechat_pay}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWechatPay(final WechatPay wechatPay) {
this.wechatPay = wechatPay;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentMethod)) return false;
final PaymentMethod other = (PaymentMethod) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$acssDebit = this.getAcssDebit();
final java.lang.Object other$acssDebit = other.getAcssDebit();
if (this$acssDebit == null ? other$acssDebit != null : !this$acssDebit.equals(other$acssDebit)) return false;
final java.lang.Object this$afterpayClearpay = this.getAfterpayClearpay();
final java.lang.Object other$afterpayClearpay = other.getAfterpayClearpay();
if (this$afterpayClearpay == null ? other$afterpayClearpay != null : !this$afterpayClearpay.equals(other$afterpayClearpay)) return false;
final java.lang.Object this$alipay = this.getAlipay();
final java.lang.Object other$alipay = other.getAlipay();
if (this$alipay == null ? other$alipay != null : !this$alipay.equals(other$alipay)) return false;
final java.lang.Object this$auBecsDebit = this.getAuBecsDebit();
final java.lang.Object other$auBecsDebit = other.getAuBecsDebit();
if (this$auBecsDebit == null ? other$auBecsDebit != null : !this$auBecsDebit.equals(other$auBecsDebit)) return false;
final java.lang.Object this$bacsDebit = this.getBacsDebit();
final java.lang.Object other$bacsDebit = other.getBacsDebit();
if (this$bacsDebit == null ? other$bacsDebit != null : !this$bacsDebit.equals(other$bacsDebit)) return false;
final java.lang.Object this$bancontact = this.getBancontact();
final java.lang.Object other$bancontact = other.getBancontact();
if (this$bancontact == null ? other$bancontact != null : !this$bancontact.equals(other$bancontact)) return false;
final java.lang.Object this$billingDetails = this.getBillingDetails();
final java.lang.Object other$billingDetails = other.getBillingDetails();
if (this$billingDetails == null ? other$billingDetails != null : !this$billingDetails.equals(other$billingDetails)) return false;
final java.lang.Object this$boleto = this.getBoleto();
final java.lang.Object other$boleto = other.getBoleto();
if (this$boleto == null ? other$boleto != null : !this$boleto.equals(other$boleto)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$cardPresent = this.getCardPresent();
final java.lang.Object other$cardPresent = other.getCardPresent();
if (this$cardPresent == null ? other$cardPresent != null : !this$cardPresent.equals(other$cardPresent)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$eps = this.getEps();
final java.lang.Object other$eps = other.getEps();
if (this$eps == null ? other$eps != null : !this$eps.equals(other$eps)) return false;
final java.lang.Object this$fpx = this.getFpx();
final java.lang.Object other$fpx = other.getFpx();
if (this$fpx == null ? other$fpx != null : !this$fpx.equals(other$fpx)) return false;
final java.lang.Object this$giropay = this.getGiropay();
final java.lang.Object other$giropay = other.getGiropay();
if (this$giropay == null ? other$giropay != null : !this$giropay.equals(other$giropay)) return false;
final java.lang.Object this$grabpay = this.getGrabpay();
final java.lang.Object other$grabpay = other.getGrabpay();
if (this$grabpay == null ? other$grabpay != null : !this$grabpay.equals(other$grabpay)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$ideal = this.getIdeal();
final java.lang.Object other$ideal = other.getIdeal();
if (this$ideal == null ? other$ideal != null : !this$ideal.equals(other$ideal)) return false;
final java.lang.Object this$interacPresent = this.getInteracPresent();
final java.lang.Object other$interacPresent = other.getInteracPresent();
if (this$interacPresent == null ? other$interacPresent != null : !this$interacPresent.equals(other$interacPresent)) return false;
final java.lang.Object this$klarna = this.getKlarna();
final java.lang.Object other$klarna = other.getKlarna();
if (this$klarna == null ? other$klarna != null : !this$klarna.equals(other$klarna)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$oxxo = this.getOxxo();
final java.lang.Object other$oxxo = other.getOxxo();
if (this$oxxo == null ? other$oxxo != null : !this$oxxo.equals(other$oxxo)) return false;
final java.lang.Object this$p24 = this.getP24();
final java.lang.Object other$p24 = other.getP24();
if (this$p24 == null ? other$p24 != null : !this$p24.equals(other$p24)) return false;
final java.lang.Object this$sepaDebit = this.getSepaDebit();
final java.lang.Object other$sepaDebit = other.getSepaDebit();
if (this$sepaDebit == null ? other$sepaDebit != null : !this$sepaDebit.equals(other$sepaDebit)) return false;
final java.lang.Object this$sofort = this.getSofort();
final java.lang.Object other$sofort = other.getSofort();
if (this$sofort == null ? other$sofort != null : !this$sofort.equals(other$sofort)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$wechatPay = this.getWechatPay();
final java.lang.Object other$wechatPay = other.getWechatPay();
if (this$wechatPay == null ? other$wechatPay != null : !this$wechatPay.equals(other$wechatPay)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentMethod;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $acssDebit = this.getAcssDebit();
result = result * PRIME + ($acssDebit == null ? 43 : $acssDebit.hashCode());
final java.lang.Object $afterpayClearpay = this.getAfterpayClearpay();
result = result * PRIME + ($afterpayClearpay == null ? 43 : $afterpayClearpay.hashCode());
final java.lang.Object $alipay = this.getAlipay();
result = result * PRIME + ($alipay == null ? 43 : $alipay.hashCode());
final java.lang.Object $auBecsDebit = this.getAuBecsDebit();
result = result * PRIME + ($auBecsDebit == null ? 43 : $auBecsDebit.hashCode());
final java.lang.Object $bacsDebit = this.getBacsDebit();
result = result * PRIME + ($bacsDebit == null ? 43 : $bacsDebit.hashCode());
final java.lang.Object $bancontact = this.getBancontact();
result = result * PRIME + ($bancontact == null ? 43 : $bancontact.hashCode());
final java.lang.Object $billingDetails = this.getBillingDetails();
result = result * PRIME + ($billingDetails == null ? 43 : $billingDetails.hashCode());
final java.lang.Object $boleto = this.getBoleto();
result = result * PRIME + ($boleto == null ? 43 : $boleto.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $cardPresent = this.getCardPresent();
result = result * PRIME + ($cardPresent == null ? 43 : $cardPresent.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $eps = this.getEps();
result = result * PRIME + ($eps == null ? 43 : $eps.hashCode());
final java.lang.Object $fpx = this.getFpx();
result = result * PRIME + ($fpx == null ? 43 : $fpx.hashCode());
final java.lang.Object $giropay = this.getGiropay();
result = result * PRIME + ($giropay == null ? 43 : $giropay.hashCode());
final java.lang.Object $grabpay = this.getGrabpay();
result = result * PRIME + ($grabpay == null ? 43 : $grabpay.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $ideal = this.getIdeal();
result = result * PRIME + ($ideal == null ? 43 : $ideal.hashCode());
final java.lang.Object $interacPresent = this.getInteracPresent();
result = result * PRIME + ($interacPresent == null ? 43 : $interacPresent.hashCode());
final java.lang.Object $klarna = this.getKlarna();
result = result * PRIME + ($klarna == null ? 43 : $klarna.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $oxxo = this.getOxxo();
result = result * PRIME + ($oxxo == null ? 43 : $oxxo.hashCode());
final java.lang.Object $p24 = this.getP24();
result = result * PRIME + ($p24 == null ? 43 : $p24.hashCode());
final java.lang.Object $sepaDebit = this.getSepaDebit();
result = result * PRIME + ($sepaDebit == null ? 43 : $sepaDebit.hashCode());
final java.lang.Object $sofort = this.getSofort();
result = result * PRIME + ($sofort == null ? 43 : $sofort.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $wechatPay = this.getWechatPay();
result = result * PRIME + ($wechatPay == null ? 43 : $wechatPay.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}