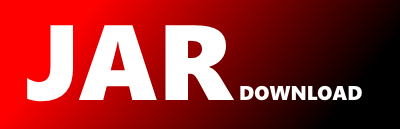
com.stripe.model.Quote Maven / Gradle / Ivy
// Generated by delombok at Fri Nov 19 19:06:04 EST 2021
// File generated from our OpenAPI spec
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.QuoteAcceptParams;
import com.stripe.param.QuoteCancelParams;
import com.stripe.param.QuoteCreateParams;
import com.stripe.param.QuoteFinalizeQuoteParams;
import com.stripe.param.QuoteListComputedUpfrontLineItemsParams;
import com.stripe.param.QuoteListLineItemsParams;
import com.stripe.param.QuoteListParams;
import com.stripe.param.QuotePdfParams;
import com.stripe.param.QuoteRetrieveParams;
import com.stripe.param.QuoteUpdateParams;
import java.io.InputStream;
import java.math.BigDecimal;
import java.util.List;
import java.util.Map;
import java.util.stream.Collectors;
public class Quote extends ApiResource implements HasId, MetadataStore {
/**
* Total before any discounts or taxes are applied.
*/
@SerializedName("amount_subtotal")
Long amountSubtotal;
/**
* Total after discounts and taxes are applied.
*/
@SerializedName("amount_total")
Long amountTotal;
/**
* The amount of the application fee (if any) that will be requested to be applied to the payment
* and transferred to the application owner's Stripe account. Only applicable if there are no line
* items with recurring prices on the quote.
*/
@SerializedName("application_fee_amount")
Long applicationFeeAmount;
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. Only applicable if there are line items with recurring prices on the
* quote.
*/
@SerializedName("application_fee_percent")
BigDecimal applicationFeePercent;
@SerializedName("automatic_tax")
AutomaticTax automaticTax;
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay invoices at the end of the subscription cycle or on finalization
* using the default payment method attached to the subscription or customer. When sending an
* invoice, Stripe will email your customer an invoice with payment instructions. Defaults to
* {@code charge_automatically}.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@SerializedName("collection_method")
String collectionMethod;
@SerializedName("computed")
Computed computed;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* The customer which this quote belongs to. A customer is required before finalizing the quote.
* Once specified, it cannot be changed.
*/
@SerializedName("customer")
ExpandableField customer;
/**
* The tax rates applied to this quote.
*/
@SerializedName("default_tax_rates")
List> defaultTaxRates;
/**
* A description that will be displayed on the quote PDF.
*/
@SerializedName("description")
String description;
/**
* The discounts applied to this quote.
*/
@SerializedName("discounts")
List> discounts;
/**
* The date on which the quote will be canceled if in {@code open} or {@code draft} status.
* Measured in seconds since the Unix epoch.
*/
@SerializedName("expires_at")
Long expiresAt;
/**
* A footer that will be displayed on the quote PDF.
*/
@SerializedName("footer")
String footer;
/**
* Details of the quote that was cloned. See the cloning documentation for more details.
*/
@SerializedName("from_quote")
FromQuote fromQuote;
/**
* A header that will be displayed on the quote PDF.
*/
@SerializedName("header")
String header;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* The invoice that was created from this quote.
*/
@SerializedName("invoice")
ExpandableField invoice;
/**
* All invoices will be billed using the specified settings.
*/
@SerializedName("invoice_settings")
InvoiceSettings invoiceSettings;
/**
* A list of items the customer is being quoted for.
*/
@SerializedName("line_items")
LineItemCollection lineItems;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* A unique number that identifies this particular quote. This number is assigned once the quote
* is finalized.
*/
@SerializedName("number")
String number;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code quote}.
*/
@SerializedName("object")
String object;
/**
* The account on behalf of which to charge. See the Connect
* documentation for details.
*/
@SerializedName("on_behalf_of")
ExpandableField onBehalfOf;
/**
* The status of the quote.
*
* One of {@code accepted}, {@code canceled}, {@code draft}, or {@code open}.
*/
@SerializedName("status")
String status;
@SerializedName("status_transitions")
StatusTransitions statusTransitions;
/**
* The subscription that was created or updated from this quote.
*/
@SerializedName("subscription")
ExpandableField subscription;
@SerializedName("subscription_data")
SubscriptionData subscriptionData;
/**
* The subscription schedule that was created or updated from this quote.
*/
@SerializedName("subscription_schedule")
ExpandableField subscriptionSchedule;
@SerializedName("total_details")
TotalDetails totalDetails;
/**
* The account (if any) the payments will be attributed to for tax reporting, and where funds from
* each payment will be transferred to for each of the invoices.
*/
@SerializedName("transfer_data")
TransferData transferData;
/**
* Get ID of expandable {@code customer} object.
*/
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String id) {
this.customer = ApiResource.setExpandableFieldId(id, this.customer);
}
/**
* Get expanded {@code customer}.
*/
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer expandableObject) {
this.customer = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code invoice} object.
*/
public String getInvoice() {
return (this.invoice != null) ? this.invoice.getId() : null;
}
public void setInvoice(String id) {
this.invoice = ApiResource.setExpandableFieldId(id, this.invoice);
}
/**
* Get expanded {@code invoice}.
*/
public Invoice getInvoiceObject() {
return (this.invoice != null) ? this.invoice.getExpanded() : null;
}
public void setInvoiceObject(Invoice expandableObject) {
this.invoice = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code onBehalfOf} object.
*/
public String getOnBehalfOf() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getId() : null;
}
public void setOnBehalfOf(String id) {
this.onBehalfOf = ApiResource.setExpandableFieldId(id, this.onBehalfOf);
}
/**
* Get expanded {@code onBehalfOf}.
*/
public Account getOnBehalfOfObject() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getExpanded() : null;
}
public void setOnBehalfOfObject(Account expandableObject) {
this.onBehalfOf = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code subscription} object.
*/
public String getSubscription() {
return (this.subscription != null) ? this.subscription.getId() : null;
}
public void setSubscription(String id) {
this.subscription = ApiResource.setExpandableFieldId(id, this.subscription);
}
/**
* Get expanded {@code subscription}.
*/
public Subscription getSubscriptionObject() {
return (this.subscription != null) ? this.subscription.getExpanded() : null;
}
public void setSubscriptionObject(Subscription expandableObject) {
this.subscription = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get ID of expandable {@code subscriptionSchedule} object.
*/
public String getSubscriptionSchedule() {
return (this.subscriptionSchedule != null) ? this.subscriptionSchedule.getId() : null;
}
public void setSubscriptionSchedule(String id) {
this.subscriptionSchedule = ApiResource.setExpandableFieldId(id, this.subscriptionSchedule);
}
/**
* Get expanded {@code subscriptionSchedule}.
*/
public SubscriptionSchedule getSubscriptionScheduleObject() {
return (this.subscriptionSchedule != null) ? this.subscriptionSchedule.getExpanded() : null;
}
public void setSubscriptionScheduleObject(SubscriptionSchedule expandableObject) {
this.subscriptionSchedule = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Get IDs of expandable {@code defaultTaxRates} object list.
*/
public List getDefaultTaxRates() {
return (this.defaultTaxRates != null) ? this.defaultTaxRates.stream().map(x -> x.getId()).collect(Collectors.toList()) : null;
}
public void setDefaultTaxRates(List ids) {
if (ids == null) {
this.defaultTaxRates = null;
return;
}
if (this.defaultTaxRates.stream().map(x -> x.getId()).collect(Collectors.toList()).equals(ids)) {
// noop if the ids are equal to what are already present
return;
}
this.defaultTaxRates = (ids != null) ? ids.stream().map(id -> new ExpandableField(id, null)).collect(Collectors.toList()) : null;
}
/**
* Get expanded {@code defaultTaxRates}.
*/
public List getDefaultTaxRateObjects() {
return (this.defaultTaxRates != null) ? this.defaultTaxRates.stream().map(x -> x.getExpanded()).collect(Collectors.toList()) : null;
}
public void setDefaultTaxRateObjects(List objs) {
this.defaultTaxRates = objs != null ? objs.stream().map(x -> new ExpandableField(x.getId(), x)).collect(Collectors.toList()) : null;
}
/**
* Get IDs of expandable {@code discounts} object list.
*/
public List getDiscounts() {
return (this.discounts != null) ? this.discounts.stream().map(x -> x.getId()).collect(Collectors.toList()) : null;
}
public void setDiscounts(List ids) {
if (ids == null) {
this.discounts = null;
return;
}
if (this.discounts.stream().map(x -> x.getId()).collect(Collectors.toList()).equals(ids)) {
// noop if the ids are equal to what are already present
return;
}
this.discounts = (ids != null) ? ids.stream().map(id -> new ExpandableField(id, null)).collect(Collectors.toList()) : null;
}
/**
* Get expanded {@code discounts}.
*/
public List getDiscountObjects() {
return (this.discounts != null) ? this.discounts.stream().map(x -> x.getExpanded()).collect(Collectors.toList()) : null;
}
public void setDiscountObjects(List objs) {
this.discounts = objs != null ? objs.stream().map(x -> new ExpandableField(x.getId(), x)).collect(Collectors.toList()) : null;
}
/**
* Retrieves the quote with the given ID.
*/
public static Quote retrieve(String quote) throws StripeException {
return retrieve(quote, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the quote with the given ID.
*/
public static Quote retrieve(String quote, RequestOptions options) throws StripeException {
return retrieve(quote, (Map) null, options);
}
/**
* Retrieves the quote with the given ID.
*/
public static Quote retrieve(String quote, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s", ApiResource.urlEncodeId(quote)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Quote.class, options);
}
/**
* Retrieves the quote with the given ID.
*/
public static Quote retrieve(String quote, QuoteRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s", ApiResource.urlEncodeId(quote)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Quote.class, options);
}
/**
* A quote models prices and services for a customer. Default options for header
,
* description
, footer
, and expires_at
can be set in the
* dashboard via the quote
* template.
*/
public static Quote create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* A quote models prices and services for a customer. Default options for header
,
* description
, footer
, and expires_at
can be set in the
* dashboard via the quote
* template.
*/
public static Quote create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/quotes");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* A quote models prices and services for a customer. Default options for header
,
* description
, footer
, and expires_at
can be set in the
* dashboard via the quote
* template.
*/
public static Quote create(QuoteCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* A quote models prices and services for a customer. Default options for header
,
* description
, footer
, and expires_at
can be set in the
* dashboard via the quote
* template.
*/
public static Quote create(QuoteCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/quotes");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* A quote models prices and services for a customer.
*/
@Override
public Quote update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* A quote models prices and services for a customer.
*/
@Override
public Quote update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* A quote models prices and services for a customer.
*/
public Quote update(QuoteUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* A quote models prices and services for a customer.
*/
public Quote update(QuoteUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Cancels the quote.
*/
public Quote cancel() throws StripeException {
return cancel((Map) null, (RequestOptions) null);
}
/**
* Cancels the quote.
*/
public Quote cancel(RequestOptions options) throws StripeException {
return cancel((Map) null, options);
}
/**
* Cancels the quote.
*/
public Quote cancel(Map params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* Cancels the quote.
*/
public Quote cancel(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/cancel", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Cancels the quote.
*/
public Quote cancel(QuoteCancelParams params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* Cancels the quote.
*/
public Quote cancel(QuoteCancelParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/cancel", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Finalizes the quote.
*/
public Quote finalizeQuote() throws StripeException {
return finalizeQuote((Map) null, (RequestOptions) null);
}
/**
* Finalizes the quote.
*/
public Quote finalizeQuote(RequestOptions options) throws StripeException {
return finalizeQuote((Map) null, options);
}
/**
* Finalizes the quote.
*/
public Quote finalizeQuote(Map params) throws StripeException {
return finalizeQuote(params, (RequestOptions) null);
}
/**
* Finalizes the quote.
*/
public Quote finalizeQuote(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/finalize", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Finalizes the quote.
*/
public Quote finalizeQuote(QuoteFinalizeQuoteParams params) throws StripeException {
return finalizeQuote(params, (RequestOptions) null);
}
/**
* Finalizes the quote.
*/
public Quote finalizeQuote(QuoteFinalizeQuoteParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/finalize", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Accepts the specified quote.
*/
public Quote accept() throws StripeException {
return accept((Map) null, (RequestOptions) null);
}
/**
* Accepts the specified quote.
*/
public Quote accept(RequestOptions options) throws StripeException {
return accept((Map) null, options);
}
/**
* Accepts the specified quote.
*/
public Quote accept(Map params) throws StripeException {
return accept(params, (RequestOptions) null);
}
/**
* Accepts the specified quote.
*/
public Quote accept(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/accept", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Accepts the specified quote.
*/
public Quote accept(QuoteAcceptParams params) throws StripeException {
return accept(params, (RequestOptions) null);
}
/**
* Accepts the specified quote.
*/
public Quote accept(QuoteAcceptParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/accept", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Quote.class, options);
}
/**
* Returns a list of your quotes.
*/
public static QuoteCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of your quotes.
*/
public static QuoteCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/quotes");
return ApiResource.requestCollection(url, params, QuoteCollection.class, options);
}
/**
* Returns a list of your quotes.
*/
public static QuoteCollection list(QuoteListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of your quotes.
*/
public static QuoteCollection list(QuoteListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/quotes");
return ApiResource.requestCollection(url, params, QuoteCollection.class, options);
}
/**
* When retrieving a quote, there is an includable line_items property containing
* the first handful of those items. There is also a URL where you can retrieve the full
* (paginated) list of line items.
*/
public LineItemCollection listLineItems() throws StripeException {
return listLineItems((Map) null, (RequestOptions) null);
}
/**
* When retrieving a quote, there is an includable line_items property containing
* the first handful of those items. There is also a URL where you can retrieve the full
* (paginated) list of line items.
*/
public LineItemCollection listLineItems(Map params) throws StripeException {
return listLineItems(params, (RequestOptions) null);
}
/**
* When retrieving a quote, there is an includable line_items property containing
* the first handful of those items. There is also a URL where you can retrieve the full
* (paginated) list of line items.
*/
public LineItemCollection listLineItems(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/line_items", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestCollection(url, params, LineItemCollection.class, options);
}
/**
* When retrieving a quote, there is an includable line_items property containing
* the first handful of those items. There is also a URL where you can retrieve the full
* (paginated) list of line items.
*/
public LineItemCollection listLineItems(QuoteListLineItemsParams params) throws StripeException {
return listLineItems(params, (RequestOptions) null);
}
/**
* When retrieving a quote, there is an includable line_items property containing
* the first handful of those items. There is also a URL where you can retrieve the full
* (paginated) list of line items.
*/
public LineItemCollection listLineItems(QuoteListLineItemsParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/line_items", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestCollection(url, params, LineItemCollection.class, options);
}
/**
* When retrieving a quote, there is an includable computed.upfront.line_items
* property containing the first handful of those items. There is also a URL where you can
* retrieve the full (paginated) list of upfront line items.
*/
public LineItemCollection listComputedUpfrontLineItems() throws StripeException {
return listComputedUpfrontLineItems((Map) null, (RequestOptions) null);
}
/**
* When retrieving a quote, there is an includable computed.upfront.line_items
* property containing the first handful of those items. There is also a URL where you can
* retrieve the full (paginated) list of upfront line items.
*/
public LineItemCollection listComputedUpfrontLineItems(Map params) throws StripeException {
return listComputedUpfrontLineItems(params, (RequestOptions) null);
}
/**
* When retrieving a quote, there is an includable computed.upfront.line_items
* property containing the first handful of those items. There is also a URL where you can
* retrieve the full (paginated) list of upfront line items.
*/
public LineItemCollection listComputedUpfrontLineItems(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/computed_upfront_line_items", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestCollection(url, params, LineItemCollection.class, options);
}
/**
* When retrieving a quote, there is an includable computed.upfront.line_items
* property containing the first handful of those items. There is also a URL where you can
* retrieve the full (paginated) list of upfront line items.
*/
public LineItemCollection listComputedUpfrontLineItems(QuoteListComputedUpfrontLineItemsParams params) throws StripeException {
return listComputedUpfrontLineItems(params, (RequestOptions) null);
}
/**
* When retrieving a quote, there is an includable computed.upfront.line_items
* property containing the first handful of those items. There is also a URL where you can
* retrieve the full (paginated) list of upfront line items.
*/
public LineItemCollection listComputedUpfrontLineItems(QuoteListComputedUpfrontLineItemsParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/quotes/%s/computed_upfront_line_items", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestCollection(url, params, LineItemCollection.class, options);
}
/**
* Download the PDF for a finalized quote.
*/
public InputStream pdf() throws StripeException {
return pdf((Map) null, (RequestOptions) null);
}
/**
* Download the PDF for a finalized quote.
*/
public InputStream pdf(Map params) throws StripeException {
return pdf(params, (RequestOptions) null);
}
/**
* Download the PDF for a finalized quote.
*/
public InputStream pdf(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getUploadBase(), String.format("/v1/quotes/%s/pdf", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestStream(ApiResource.RequestMethod.GET, url, params, options);
}
/**
* Download the PDF for a finalized quote.
*/
public InputStream pdf(QuotePdfParams params) throws StripeException {
return pdf(params, (RequestOptions) null);
}
/**
* Download the PDF for a finalized quote.
*/
public InputStream pdf(QuotePdfParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getUploadBase(), String.format("/v1/quotes/%s/pdf", ApiResource.urlEncodeId(this.getId())));
return ApiResource.requestStream(ApiResource.RequestMethod.GET, url, params, options);
}
public static class AutomaticTax extends StripeObject {
/**
* Automatically calculate taxes.
*/
@SerializedName("enabled")
Boolean enabled;
/**
* The status of the most recent automated tax calculation for this quote.
*
* One of {@code complete}, {@code failed}, or {@code requires_location_inputs}.
*/
@SerializedName("status")
String status;
/**
* Automatically calculate taxes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getEnabled() {
return this.enabled;
}
/**
* The status of the most recent automated tax calculation for this quote.
*
*
One of {@code complete}, {@code failed}, or {@code requires_location_inputs}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* Automatically calculate taxes.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEnabled(final Boolean enabled) {
this.enabled = enabled;
}
/**
* The status of the most recent automated tax calculation for this quote.
*
*
One of {@code complete}, {@code failed}, or {@code requires_location_inputs}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.AutomaticTax)) return false;
final Quote.AutomaticTax other = (Quote.AutomaticTax) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$enabled = this.getEnabled();
final java.lang.Object other$enabled = other.getEnabled();
if (this$enabled == null ? other$enabled != null : !this$enabled.equals(other$enabled)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.AutomaticTax;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $enabled = this.getEnabled();
result = result * PRIME + ($enabled == null ? 43 : $enabled.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
}
public static class Computed extends StripeObject {
/**
* The definitive totals and line items the customer will be charged on a recurring basis. Takes
* into account the line items with recurring prices and discounts with {@code duration=forever}
* coupons only. Defaults to {@code null} if no inputted line items with recurring prices.
*/
@SerializedName("recurring")
Recurring recurring;
@SerializedName("upfront")
Upfront upfront;
/**
* The definitive totals and line items the customer will be charged on a recurring basis. Takes
* into account the line items with recurring prices and discounts with {@code duration=forever}
* coupons only. Defaults to {@code null} if no inputted line items with recurring prices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Recurring getRecurring() {
return this.recurring;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Upfront getUpfront() {
return this.upfront;
}
/**
* The definitive totals and line items the customer will be charged on a recurring basis. Takes
* into account the line items with recurring prices and discounts with {@code duration=forever}
* coupons only. Defaults to {@code null} if no inputted line items with recurring prices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRecurring(final Recurring recurring) {
this.recurring = recurring;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUpfront(final Upfront upfront) {
this.upfront = upfront;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.Computed)) return false;
final Quote.Computed other = (Quote.Computed) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$recurring = this.getRecurring();
final java.lang.Object other$recurring = other.getRecurring();
if (this$recurring == null ? other$recurring != null : !this$recurring.equals(other$recurring)) return false;
final java.lang.Object this$upfront = this.getUpfront();
final java.lang.Object other$upfront = other.getUpfront();
if (this$upfront == null ? other$upfront != null : !this$upfront.equals(other$upfront)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.Computed;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $recurring = this.getRecurring();
result = result * PRIME + ($recurring == null ? 43 : $recurring.hashCode());
final java.lang.Object $upfront = this.getUpfront();
result = result * PRIME + ($upfront == null ? 43 : $upfront.hashCode());
return result;
}
}
public static class FromQuote extends StripeObject {
/**
* Whether this quote is a revision of a different quote.
*/
@SerializedName("is_revision")
Boolean isRevision;
/**
* The quote that was cloned.
*/
@SerializedName("quote")
ExpandableField quote;
/**
* Get ID of expandable {@code quote} object.
*/
public String getQuote() {
return (this.quote != null) ? this.quote.getId() : null;
}
public void setQuote(String id) {
this.quote = ApiResource.setExpandableFieldId(id, this.quote);
}
/**
* Get expanded {@code quote}.
*/
public Quote getQuoteObject() {
return (this.quote != null) ? this.quote.getExpanded() : null;
}
public void setQuoteObject(Quote expandableObject) {
this.quote = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* Whether this quote is a revision of a different quote.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getIsRevision() {
return this.isRevision;
}
/**
* Whether this quote is a revision of a different quote.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIsRevision(final Boolean isRevision) {
this.isRevision = isRevision;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.FromQuote)) return false;
final Quote.FromQuote other = (Quote.FromQuote) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$isRevision = this.getIsRevision();
final java.lang.Object other$isRevision = other.getIsRevision();
if (this$isRevision == null ? other$isRevision != null : !this$isRevision.equals(other$isRevision)) return false;
final java.lang.Object this$quote = this.getQuote();
final java.lang.Object other$quote = other.getQuote();
if (this$quote == null ? other$quote != null : !this$quote.equals(other$quote)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.FromQuote;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $isRevision = this.getIsRevision();
result = result * PRIME + ($isRevision == null ? 43 : $isRevision.hashCode());
final java.lang.Object $quote = this.getQuote();
result = result * PRIME + ($quote == null ? 43 : $quote.hashCode());
return result;
}
}
public static class InvoiceSettings extends StripeObject {
/**
* Number of days within which a customer must pay invoices generated by this quote. This value
* will be {@code null} for quotes where {@code collection_method=charge_automatically}.
*/
@SerializedName("days_until_due")
Long daysUntilDue;
/**
* Number of days within which a customer must pay invoices generated by this quote. This value
* will be {@code null} for quotes where {@code collection_method=charge_automatically}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDaysUntilDue() {
return this.daysUntilDue;
}
/**
* Number of days within which a customer must pay invoices generated by this quote. This value
* will be {@code null} for quotes where {@code collection_method=charge_automatically}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDaysUntilDue(final Long daysUntilDue) {
this.daysUntilDue = daysUntilDue;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.InvoiceSettings)) return false;
final Quote.InvoiceSettings other = (Quote.InvoiceSettings) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$daysUntilDue = this.getDaysUntilDue();
final java.lang.Object other$daysUntilDue = other.getDaysUntilDue();
if (this$daysUntilDue == null ? other$daysUntilDue != null : !this$daysUntilDue.equals(other$daysUntilDue)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.InvoiceSettings;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $daysUntilDue = this.getDaysUntilDue();
result = result * PRIME + ($daysUntilDue == null ? 43 : $daysUntilDue.hashCode());
return result;
}
}
public static class Recurring extends StripeObject {
/**
* Total before any discounts or taxes are applied.
*/
@SerializedName("amount_subtotal")
Long amountSubtotal;
/**
* Total after discounts and taxes are applied.
*/
@SerializedName("amount_total")
Long amountTotal;
/**
* The frequency at which a subscription is billed. One of {@code day}, {@code week}, {@code
* month} or {@code year}.
*/
@SerializedName("interval")
String interval;
/**
* The number of intervals (specified in the {@code interval} attribute) between subscription
* billings. For example, {@code interval=month} and {@code interval_count=3} bills every 3
* months.
*/
@SerializedName("interval_count")
Long intervalCount;
@SerializedName("total_details")
TotalDetails totalDetails;
/**
* Total before any discounts or taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountSubtotal() {
return this.amountSubtotal;
}
/**
* Total after discounts and taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTotal() {
return this.amountTotal;
}
/**
* The frequency at which a subscription is billed. One of {@code day}, {@code week}, {@code
* month} or {@code year}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInterval() {
return this.interval;
}
/**
* The number of intervals (specified in the {@code interval} attribute) between subscription
* billings. For example, {@code interval=month} and {@code interval_count=3} bills every 3
* months.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getIntervalCount() {
return this.intervalCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TotalDetails getTotalDetails() {
return this.totalDetails;
}
/**
* Total before any discounts or taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountSubtotal(final Long amountSubtotal) {
this.amountSubtotal = amountSubtotal;
}
/**
* Total after discounts and taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTotal(final Long amountTotal) {
this.amountTotal = amountTotal;
}
/**
* The frequency at which a subscription is billed. One of {@code day}, {@code week}, {@code
* month} or {@code year}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInterval(final String interval) {
this.interval = interval;
}
/**
* The number of intervals (specified in the {@code interval} attribute) between subscription
* billings. For example, {@code interval=month} and {@code interval_count=3} bills every 3
* months.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIntervalCount(final Long intervalCount) {
this.intervalCount = intervalCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotalDetails(final TotalDetails totalDetails) {
this.totalDetails = totalDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.Recurring)) return false;
final Quote.Recurring other = (Quote.Recurring) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountSubtotal = this.getAmountSubtotal();
final java.lang.Object other$amountSubtotal = other.getAmountSubtotal();
if (this$amountSubtotal == null ? other$amountSubtotal != null : !this$amountSubtotal.equals(other$amountSubtotal)) return false;
final java.lang.Object this$amountTotal = this.getAmountTotal();
final java.lang.Object other$amountTotal = other.getAmountTotal();
if (this$amountTotal == null ? other$amountTotal != null : !this$amountTotal.equals(other$amountTotal)) return false;
final java.lang.Object this$intervalCount = this.getIntervalCount();
final java.lang.Object other$intervalCount = other.getIntervalCount();
if (this$intervalCount == null ? other$intervalCount != null : !this$intervalCount.equals(other$intervalCount)) return false;
final java.lang.Object this$interval = this.getInterval();
final java.lang.Object other$interval = other.getInterval();
if (this$interval == null ? other$interval != null : !this$interval.equals(other$interval)) return false;
final java.lang.Object this$totalDetails = this.getTotalDetails();
final java.lang.Object other$totalDetails = other.getTotalDetails();
if (this$totalDetails == null ? other$totalDetails != null : !this$totalDetails.equals(other$totalDetails)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.Recurring;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountSubtotal = this.getAmountSubtotal();
result = result * PRIME + ($amountSubtotal == null ? 43 : $amountSubtotal.hashCode());
final java.lang.Object $amountTotal = this.getAmountTotal();
result = result * PRIME + ($amountTotal == null ? 43 : $amountTotal.hashCode());
final java.lang.Object $intervalCount = this.getIntervalCount();
result = result * PRIME + ($intervalCount == null ? 43 : $intervalCount.hashCode());
final java.lang.Object $interval = this.getInterval();
result = result * PRIME + ($interval == null ? 43 : $interval.hashCode());
final java.lang.Object $totalDetails = this.getTotalDetails();
result = result * PRIME + ($totalDetails == null ? 43 : $totalDetails.hashCode());
return result;
}
}
public static class StatusTransitions extends StripeObject {
/**
* The time that the quote was accepted. Measured in seconds since Unix epoch.
*/
@SerializedName("accepted_at")
Long acceptedAt;
/**
* The time that the quote was canceled. Measured in seconds since Unix epoch.
*/
@SerializedName("canceled_at")
Long canceledAt;
/**
* The time that the quote was finalized. Measured in seconds since Unix epoch.
*/
@SerializedName("finalized_at")
Long finalizedAt;
/**
* The time that the quote was accepted. Measured in seconds since Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAcceptedAt() {
return this.acceptedAt;
}
/**
* The time that the quote was canceled. Measured in seconds since Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCanceledAt() {
return this.canceledAt;
}
/**
* The time that the quote was finalized. Measured in seconds since Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getFinalizedAt() {
return this.finalizedAt;
}
/**
* The time that the quote was accepted. Measured in seconds since Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAcceptedAt(final Long acceptedAt) {
this.acceptedAt = acceptedAt;
}
/**
* The time that the quote was canceled. Measured in seconds since Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCanceledAt(final Long canceledAt) {
this.canceledAt = canceledAt;
}
/**
* The time that the quote was finalized. Measured in seconds since Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFinalizedAt(final Long finalizedAt) {
this.finalizedAt = finalizedAt;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.StatusTransitions)) return false;
final Quote.StatusTransitions other = (Quote.StatusTransitions) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$acceptedAt = this.getAcceptedAt();
final java.lang.Object other$acceptedAt = other.getAcceptedAt();
if (this$acceptedAt == null ? other$acceptedAt != null : !this$acceptedAt.equals(other$acceptedAt)) return false;
final java.lang.Object this$canceledAt = this.getCanceledAt();
final java.lang.Object other$canceledAt = other.getCanceledAt();
if (this$canceledAt == null ? other$canceledAt != null : !this$canceledAt.equals(other$canceledAt)) return false;
final java.lang.Object this$finalizedAt = this.getFinalizedAt();
final java.lang.Object other$finalizedAt = other.getFinalizedAt();
if (this$finalizedAt == null ? other$finalizedAt != null : !this$finalizedAt.equals(other$finalizedAt)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.StatusTransitions;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $acceptedAt = this.getAcceptedAt();
result = result * PRIME + ($acceptedAt == null ? 43 : $acceptedAt.hashCode());
final java.lang.Object $canceledAt = this.getCanceledAt();
result = result * PRIME + ($canceledAt == null ? 43 : $canceledAt.hashCode());
final java.lang.Object $finalizedAt = this.getFinalizedAt();
result = result * PRIME + ($finalizedAt == null ? 43 : $finalizedAt.hashCode());
return result;
}
}
public static class SubscriptionData extends StripeObject {
/**
* When creating a new subscription, the date of which the subscription schedule will start
* after the quote is accepted. This date is ignored if it is in the past when the quote is
* accepted. Measured in seconds since the Unix epoch.
*/
@SerializedName("effective_date")
Long effectiveDate;
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time.
*/
@SerializedName("trial_period_days")
Long trialPeriodDays;
/**
* When creating a new subscription, the date of which the subscription schedule will start
* after the quote is accepted. This date is ignored if it is in the past when the quote is
* accepted. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEffectiveDate() {
return this.effectiveDate;
}
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTrialPeriodDays() {
return this.trialPeriodDays;
}
/**
* When creating a new subscription, the date of which the subscription schedule will start
* after the quote is accepted. This date is ignored if it is in the past when the quote is
* accepted. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEffectiveDate(final Long effectiveDate) {
this.effectiveDate = effectiveDate;
}
/**
* Integer representing the number of trial period days before the customer is charged for the
* first time.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTrialPeriodDays(final Long trialPeriodDays) {
this.trialPeriodDays = trialPeriodDays;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.SubscriptionData)) return false;
final Quote.SubscriptionData other = (Quote.SubscriptionData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$effectiveDate = this.getEffectiveDate();
final java.lang.Object other$effectiveDate = other.getEffectiveDate();
if (this$effectiveDate == null ? other$effectiveDate != null : !this$effectiveDate.equals(other$effectiveDate)) return false;
final java.lang.Object this$trialPeriodDays = this.getTrialPeriodDays();
final java.lang.Object other$trialPeriodDays = other.getTrialPeriodDays();
if (this$trialPeriodDays == null ? other$trialPeriodDays != null : !this$trialPeriodDays.equals(other$trialPeriodDays)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.SubscriptionData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $effectiveDate = this.getEffectiveDate();
result = result * PRIME + ($effectiveDate == null ? 43 : $effectiveDate.hashCode());
final java.lang.Object $trialPeriodDays = this.getTrialPeriodDays();
result = result * PRIME + ($trialPeriodDays == null ? 43 : $trialPeriodDays.hashCode());
return result;
}
}
public static class TotalDetails extends StripeObject {
/**
* This is the sum of all the line item discounts.
*/
@SerializedName("amount_discount")
Long amountDiscount;
/**
* This is the sum of all the line item shipping amounts.
*/
@SerializedName("amount_shipping")
Long amountShipping;
/**
* This is the sum of all the line item tax amounts.
*/
@SerializedName("amount_tax")
Long amountTax;
@SerializedName("breakdown")
Breakdown breakdown;
public static class Breakdown extends StripeObject {
/** The aggregated line item discounts. */
@SerializedName("discounts")
List discounts;
@SerializedName("taxes")
List taxes;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDiscounts() {
return this.discounts;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getTaxes() {
return this.taxes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscounts(final List discounts) {
this.discounts = discounts;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxes(final List taxes) {
this.taxes = taxes;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.TotalDetails.Breakdown)) return false;
final Quote.TotalDetails.Breakdown other = (Quote.TotalDetails.Breakdown) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$discounts = this.getDiscounts();
final java.lang.Object other$discounts = other.getDiscounts();
if (this$discounts == null ? other$discounts != null : !this$discounts.equals(other$discounts)) return false;
final java.lang.Object this$taxes = this.getTaxes();
final java.lang.Object other$taxes = other.getTaxes();
if (this$taxes == null ? other$taxes != null : !this$taxes.equals(other$taxes)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.TotalDetails.Breakdown;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $discounts = this.getDiscounts();
result = result * PRIME + ($discounts == null ? 43 : $discounts.hashCode());
final java.lang.Object $taxes = this.getTaxes();
result = result * PRIME + ($taxes == null ? 43 : $taxes.hashCode());
return result;
}
}
/**
* This is the sum of all the line item discounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountDiscount() {
return this.amountDiscount;
}
/**
* This is the sum of all the line item shipping amounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountShipping() {
return this.amountShipping;
}
/**
* This is the sum of all the line item tax amounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTax() {
return this.amountTax;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Breakdown getBreakdown() {
return this.breakdown;
}
/**
* This is the sum of all the line item discounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountDiscount(final Long amountDiscount) {
this.amountDiscount = amountDiscount;
}
/**
* This is the sum of all the line item shipping amounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountShipping(final Long amountShipping) {
this.amountShipping = amountShipping;
}
/**
* This is the sum of all the line item tax amounts.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTax(final Long amountTax) {
this.amountTax = amountTax;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBreakdown(final Breakdown breakdown) {
this.breakdown = breakdown;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.TotalDetails)) return false;
final Quote.TotalDetails other = (Quote.TotalDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountDiscount = this.getAmountDiscount();
final java.lang.Object other$amountDiscount = other.getAmountDiscount();
if (this$amountDiscount == null ? other$amountDiscount != null : !this$amountDiscount.equals(other$amountDiscount)) return false;
final java.lang.Object this$amountShipping = this.getAmountShipping();
final java.lang.Object other$amountShipping = other.getAmountShipping();
if (this$amountShipping == null ? other$amountShipping != null : !this$amountShipping.equals(other$amountShipping)) return false;
final java.lang.Object this$amountTax = this.getAmountTax();
final java.lang.Object other$amountTax = other.getAmountTax();
if (this$amountTax == null ? other$amountTax != null : !this$amountTax.equals(other$amountTax)) return false;
final java.lang.Object this$breakdown = this.getBreakdown();
final java.lang.Object other$breakdown = other.getBreakdown();
if (this$breakdown == null ? other$breakdown != null : !this$breakdown.equals(other$breakdown)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.TotalDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountDiscount = this.getAmountDiscount();
result = result * PRIME + ($amountDiscount == null ? 43 : $amountDiscount.hashCode());
final java.lang.Object $amountShipping = this.getAmountShipping();
result = result * PRIME + ($amountShipping == null ? 43 : $amountShipping.hashCode());
final java.lang.Object $amountTax = this.getAmountTax();
result = result * PRIME + ($amountTax == null ? 43 : $amountTax.hashCode());
final java.lang.Object $breakdown = this.getBreakdown();
result = result * PRIME + ($breakdown == null ? 43 : $breakdown.hashCode());
return result;
}
}
/**
* The aggregated line item tax amounts by rate.
*/
public static class TransferData extends StripeObject {
/**
* The amount in %s that will be transferred to the destination account when the invoice is
* paid. By default, the entire amount is transferred to the destination.
*/
@SerializedName("amount")
Long amount;
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* destination account. By default, the entire amount will be transferred to the destination.
*/
@SerializedName("amount_percent")
BigDecimal amountPercent;
/**
* The account where funds from the payment will be transferred to upon payment success.
*/
@SerializedName("destination")
ExpandableField destination;
/**
* Get ID of expandable {@code destination} object.
*/
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String id) {
this.destination = ApiResource.setExpandableFieldId(id, this.destination);
}
/**
* Get expanded {@code destination}.
*/
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account expandableObject) {
this.destination = new ExpandableField(expandableObject.getId(), expandableObject);
}
/**
* The amount in %s that will be transferred to the destination account when the invoice is
* paid. By default, the entire amount is transferred to the destination.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* destination account. By default, the entire amount will be transferred to the destination.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getAmountPercent() {
return this.amountPercent;
}
/**
* The amount in %s that will be transferred to the destination account when the invoice is
* paid. By default, the entire amount is transferred to the destination.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents
* the percentage of the subscription invoice subtotal that will be transferred to the
* destination account. By default, the entire amount will be transferred to the destination.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountPercent(final BigDecimal amountPercent) {
this.amountPercent = amountPercent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.TransferData)) return false;
final Quote.TransferData other = (Quote.TransferData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$amountPercent = this.getAmountPercent();
final java.lang.Object other$amountPercent = other.getAmountPercent();
if (this$amountPercent == null ? other$amountPercent != null : !this$amountPercent.equals(other$amountPercent)) return false;
final java.lang.Object this$destination = this.getDestination();
final java.lang.Object other$destination = other.getDestination();
if (this$destination == null ? other$destination != null : !this$destination.equals(other$destination)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.TransferData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $amountPercent = this.getAmountPercent();
result = result * PRIME + ($amountPercent == null ? 43 : $amountPercent.hashCode());
final java.lang.Object $destination = this.getDestination();
result = result * PRIME + ($destination == null ? 43 : $destination.hashCode());
return result;
}
}
public static class Upfront extends StripeObject {
/** Total before any discounts or taxes are applied. */
@SerializedName("amount_subtotal")
Long amountSubtotal;
/** Total after discounts and taxes are applied. */
@SerializedName("amount_total")
Long amountTotal;
/**
* The line items that will appear on the next invoice after this quote is accepted. This does
* not include pending invoice items that exist on the customer but may still be included in the
* next invoice.
*/
@SerializedName("line_items")
LineItemCollection lineItems;
@SerializedName("total_details")
TotalDetails totalDetails;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountSubtotal() {
return this.amountSubtotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTotal() {
return this.amountTotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public LineItemCollection getLineItems() {
return this.lineItems;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TotalDetails getTotalDetails() {
return this.totalDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountSubtotal(final Long amountSubtotal) {
this.amountSubtotal = amountSubtotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTotal(final Long amountTotal) {
this.amountTotal = amountTotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLineItems(final LineItemCollection lineItems) {
this.lineItems = lineItems;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotalDetails(final TotalDetails totalDetails) {
this.totalDetails = totalDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote.Upfront)) return false;
final Quote.Upfront other = (Quote.Upfront) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountSubtotal = this.getAmountSubtotal();
final java.lang.Object other$amountSubtotal = other.getAmountSubtotal();
if (this$amountSubtotal == null ? other$amountSubtotal != null : !this$amountSubtotal.equals(other$amountSubtotal)) return false;
final java.lang.Object this$amountTotal = this.getAmountTotal();
final java.lang.Object other$amountTotal = other.getAmountTotal();
if (this$amountTotal == null ? other$amountTotal != null : !this$amountTotal.equals(other$amountTotal)) return false;
final java.lang.Object this$lineItems = this.getLineItems();
final java.lang.Object other$lineItems = other.getLineItems();
if (this$lineItems == null ? other$lineItems != null : !this$lineItems.equals(other$lineItems)) return false;
final java.lang.Object this$totalDetails = this.getTotalDetails();
final java.lang.Object other$totalDetails = other.getTotalDetails();
if (this$totalDetails == null ? other$totalDetails != null : !this$totalDetails.equals(other$totalDetails)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote.Upfront;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountSubtotal = this.getAmountSubtotal();
result = result * PRIME + ($amountSubtotal == null ? 43 : $amountSubtotal.hashCode());
final java.lang.Object $amountTotal = this.getAmountTotal();
result = result * PRIME + ($amountTotal == null ? 43 : $amountTotal.hashCode());
final java.lang.Object $lineItems = this.getLineItems();
result = result * PRIME + ($lineItems == null ? 43 : $lineItems.hashCode());
final java.lang.Object $totalDetails = this.getTotalDetails();
result = result * PRIME + ($totalDetails == null ? 43 : $totalDetails.hashCode());
return result;
}
}
/**
* Total before any discounts or taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountSubtotal() {
return this.amountSubtotal;
}
/**
* Total after discounts and taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountTotal() {
return this.amountTotal;
}
/**
* The amount of the application fee (if any) that will be requested to be applied to the payment
* and transferred to the application owner's Stripe account. Only applicable if there are no line
* items with recurring prices on the quote.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getApplicationFeeAmount() {
return this.applicationFeeAmount;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. Only applicable if there are line items with recurring prices on the
* quote.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getApplicationFeePercent() {
return this.applicationFeePercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AutomaticTax getAutomaticTax() {
return this.automaticTax;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay invoices at the end of the subscription cycle or on finalization
* using the default payment method attached to the subscription or customer. When sending an
* invoice, Stripe will email your customer an invoice with payment instructions. Defaults to
* {@code charge_automatically}.
*
* One of {@code charge_automatically}, or {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCollectionMethod() {
return this.collectionMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Computed getComputed() {
return this.computed;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
/**
* A description that will be displayed on the quote PDF.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
/**
* The date on which the quote will be canceled if in {@code open} or {@code draft} status.
* Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getExpiresAt() {
return this.expiresAt;
}
/**
* A footer that will be displayed on the quote PDF.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFooter() {
return this.footer;
}
/**
* Details of the quote that was cloned. See the cloning documentation for more details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public FromQuote getFromQuote() {
return this.fromQuote;
}
/**
* A header that will be displayed on the quote PDF.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHeader() {
return this.header;
}
/**
* All invoices will be billed using the specified settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceSettings getInvoiceSettings() {
return this.invoiceSettings;
}
/**
* A list of items the customer is being quoted for.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public LineItemCollection getLineItems() {
return this.lineItems;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* A unique number that identifies this particular quote. This number is assigned once the quote
* is finalized.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNumber() {
return this.number;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code quote}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* The status of the quote.
*
*
One of {@code accepted}, {@code canceled}, {@code draft}, or {@code open}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public StatusTransitions getStatusTransitions() {
return this.statusTransitions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionData getSubscriptionData() {
return this.subscriptionData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TotalDetails getTotalDetails() {
return this.totalDetails;
}
/**
* The account (if any) the payments will be attributed to for tax reporting, and where funds from
* each payment will be transferred to for each of the invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
/**
* Total before any discounts or taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountSubtotal(final Long amountSubtotal) {
this.amountSubtotal = amountSubtotal;
}
/**
* Total after discounts and taxes are applied.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountTotal(final Long amountTotal) {
this.amountTotal = amountTotal;
}
/**
* The amount of the application fee (if any) that will be requested to be applied to the payment
* and transferred to the application owner's Stripe account. Only applicable if there are no line
* items with recurring prices on the quote.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeeAmount(final Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
}
/**
* A non-negative decimal between 0 and 100, with at most two decimal places. This represents the
* percentage of the subscription invoice subtotal that will be transferred to the application
* owner's Stripe account. Only applicable if there are line items with recurring prices on the
* quote.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeePercent(final BigDecimal applicationFeePercent) {
this.applicationFeePercent = applicationFeePercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAutomaticTax(final AutomaticTax automaticTax) {
this.automaticTax = automaticTax;
}
/**
* Either {@code charge_automatically}, or {@code send_invoice}. When charging automatically,
* Stripe will attempt to pay invoices at the end of the subscription cycle or on finalization
* using the default payment method attached to the subscription or customer. When sending an
* invoice, Stripe will email your customer an invoice with payment instructions. Defaults to
* {@code charge_automatically}.
*
*
One of {@code charge_automatically}, or {@code send_invoice}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCollectionMethod(final String collectionMethod) {
this.collectionMethod = collectionMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setComputed(final Computed computed) {
this.computed = computed;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Three-letter ISO currency code,
* in lowercase. Must be a supported currency.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
/**
* A description that will be displayed on the quote PDF.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
/**
* The date on which the quote will be canceled if in {@code open} or {@code draft} status.
* Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setExpiresAt(final Long expiresAt) {
this.expiresAt = expiresAt;
}
/**
* A footer that will be displayed on the quote PDF.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFooter(final String footer) {
this.footer = footer;
}
/**
* Details of the quote that was cloned. See the cloning documentation for more details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFromQuote(final FromQuote fromQuote) {
this.fromQuote = fromQuote;
}
/**
* A header that will be displayed on the quote PDF.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHeader(final String header) {
this.header = header;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* All invoices will be billed using the specified settings.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceSettings(final InvoiceSettings invoiceSettings) {
this.invoiceSettings = invoiceSettings;
}
/**
* A list of items the customer is being quoted for.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLineItems(final LineItemCollection lineItems) {
this.lineItems = lineItems;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* A unique number that identifies this particular quote. This number is assigned once the quote
* is finalized.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNumber(final String number) {
this.number = number;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code quote}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* The status of the quote.
*
*
One of {@code accepted}, {@code canceled}, {@code draft}, or {@code open}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatusTransitions(final StatusTransitions statusTransitions) {
this.statusTransitions = statusTransitions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptionData(final SubscriptionData subscriptionData) {
this.subscriptionData = subscriptionData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotalDetails(final TotalDetails totalDetails) {
this.totalDetails = totalDetails;
}
/**
* The account (if any) the payments will be attributed to for tax reporting, and where funds from
* each payment will be transferred to for each of the invoices.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final TransferData transferData) {
this.transferData = transferData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Quote)) return false;
final Quote other = (Quote) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountSubtotal = this.getAmountSubtotal();
final java.lang.Object other$amountSubtotal = other.getAmountSubtotal();
if (this$amountSubtotal == null ? other$amountSubtotal != null : !this$amountSubtotal.equals(other$amountSubtotal)) return false;
final java.lang.Object this$amountTotal = this.getAmountTotal();
final java.lang.Object other$amountTotal = other.getAmountTotal();
if (this$amountTotal == null ? other$amountTotal != null : !this$amountTotal.equals(other$amountTotal)) return false;
final java.lang.Object this$applicationFeeAmount = this.getApplicationFeeAmount();
final java.lang.Object other$applicationFeeAmount = other.getApplicationFeeAmount();
if (this$applicationFeeAmount == null ? other$applicationFeeAmount != null : !this$applicationFeeAmount.equals(other$applicationFeeAmount)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$expiresAt = this.getExpiresAt();
final java.lang.Object other$expiresAt = other.getExpiresAt();
if (this$expiresAt == null ? other$expiresAt != null : !this$expiresAt.equals(other$expiresAt)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$applicationFeePercent = this.getApplicationFeePercent();
final java.lang.Object other$applicationFeePercent = other.getApplicationFeePercent();
if (this$applicationFeePercent == null ? other$applicationFeePercent != null : !this$applicationFeePercent.equals(other$applicationFeePercent)) return false;
final java.lang.Object this$automaticTax = this.getAutomaticTax();
final java.lang.Object other$automaticTax = other.getAutomaticTax();
if (this$automaticTax == null ? other$automaticTax != null : !this$automaticTax.equals(other$automaticTax)) return false;
final java.lang.Object this$collectionMethod = this.getCollectionMethod();
final java.lang.Object other$collectionMethod = other.getCollectionMethod();
if (this$collectionMethod == null ? other$collectionMethod != null : !this$collectionMethod.equals(other$collectionMethod)) return false;
final java.lang.Object this$computed = this.getComputed();
final java.lang.Object other$computed = other.getComputed();
if (this$computed == null ? other$computed != null : !this$computed.equals(other$computed)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$defaultTaxRates = this.getDefaultTaxRates();
final java.lang.Object other$defaultTaxRates = other.getDefaultTaxRates();
if (this$defaultTaxRates == null ? other$defaultTaxRates != null : !this$defaultTaxRates.equals(other$defaultTaxRates)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$discounts = this.getDiscounts();
final java.lang.Object other$discounts = other.getDiscounts();
if (this$discounts == null ? other$discounts != null : !this$discounts.equals(other$discounts)) return false;
final java.lang.Object this$footer = this.getFooter();
final java.lang.Object other$footer = other.getFooter();
if (this$footer == null ? other$footer != null : !this$footer.equals(other$footer)) return false;
final java.lang.Object this$fromQuote = this.getFromQuote();
final java.lang.Object other$fromQuote = other.getFromQuote();
if (this$fromQuote == null ? other$fromQuote != null : !this$fromQuote.equals(other$fromQuote)) return false;
final java.lang.Object this$header = this.getHeader();
final java.lang.Object other$header = other.getHeader();
if (this$header == null ? other$header != null : !this$header.equals(other$header)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$invoice = this.getInvoice();
final java.lang.Object other$invoice = other.getInvoice();
if (this$invoice == null ? other$invoice != null : !this$invoice.equals(other$invoice)) return false;
final java.lang.Object this$invoiceSettings = this.getInvoiceSettings();
final java.lang.Object other$invoiceSettings = other.getInvoiceSettings();
if (this$invoiceSettings == null ? other$invoiceSettings != null : !this$invoiceSettings.equals(other$invoiceSettings)) return false;
final java.lang.Object this$lineItems = this.getLineItems();
final java.lang.Object other$lineItems = other.getLineItems();
if (this$lineItems == null ? other$lineItems != null : !this$lineItems.equals(other$lineItems)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$number = this.getNumber();
final java.lang.Object other$number = other.getNumber();
if (this$number == null ? other$number != null : !this$number.equals(other$number)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$onBehalfOf = this.getOnBehalfOf();
final java.lang.Object other$onBehalfOf = other.getOnBehalfOf();
if (this$onBehalfOf == null ? other$onBehalfOf != null : !this$onBehalfOf.equals(other$onBehalfOf)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$statusTransitions = this.getStatusTransitions();
final java.lang.Object other$statusTransitions = other.getStatusTransitions();
if (this$statusTransitions == null ? other$statusTransitions != null : !this$statusTransitions.equals(other$statusTransitions)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
final java.lang.Object this$subscriptionData = this.getSubscriptionData();
final java.lang.Object other$subscriptionData = other.getSubscriptionData();
if (this$subscriptionData == null ? other$subscriptionData != null : !this$subscriptionData.equals(other$subscriptionData)) return false;
final java.lang.Object this$subscriptionSchedule = this.getSubscriptionSchedule();
final java.lang.Object other$subscriptionSchedule = other.getSubscriptionSchedule();
if (this$subscriptionSchedule == null ? other$subscriptionSchedule != null : !this$subscriptionSchedule.equals(other$subscriptionSchedule)) return false;
final java.lang.Object this$totalDetails = this.getTotalDetails();
final java.lang.Object other$totalDetails = other.getTotalDetails();
if (this$totalDetails == null ? other$totalDetails != null : !this$totalDetails.equals(other$totalDetails)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Quote;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountSubtotal = this.getAmountSubtotal();
result = result * PRIME + ($amountSubtotal == null ? 43 : $amountSubtotal.hashCode());
final java.lang.Object $amountTotal = this.getAmountTotal();
result = result * PRIME + ($amountTotal == null ? 43 : $amountTotal.hashCode());
final java.lang.Object $applicationFeeAmount = this.getApplicationFeeAmount();
result = result * PRIME + ($applicationFeeAmount == null ? 43 : $applicationFeeAmount.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $expiresAt = this.getExpiresAt();
result = result * PRIME + ($expiresAt == null ? 43 : $expiresAt.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $applicationFeePercent = this.getApplicationFeePercent();
result = result * PRIME + ($applicationFeePercent == null ? 43 : $applicationFeePercent.hashCode());
final java.lang.Object $automaticTax = this.getAutomaticTax();
result = result * PRIME + ($automaticTax == null ? 43 : $automaticTax.hashCode());
final java.lang.Object $collectionMethod = this.getCollectionMethod();
result = result * PRIME + ($collectionMethod == null ? 43 : $collectionMethod.hashCode());
final java.lang.Object $computed = this.getComputed();
result = result * PRIME + ($computed == null ? 43 : $computed.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $defaultTaxRates = this.getDefaultTaxRates();
result = result * PRIME + ($defaultTaxRates == null ? 43 : $defaultTaxRates.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $discounts = this.getDiscounts();
result = result * PRIME + ($discounts == null ? 43 : $discounts.hashCode());
final java.lang.Object $footer = this.getFooter();
result = result * PRIME + ($footer == null ? 43 : $footer.hashCode());
final java.lang.Object $fromQuote = this.getFromQuote();
result = result * PRIME + ($fromQuote == null ? 43 : $fromQuote.hashCode());
final java.lang.Object $header = this.getHeader();
result = result * PRIME + ($header == null ? 43 : $header.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $invoice = this.getInvoice();
result = result * PRIME + ($invoice == null ? 43 : $invoice.hashCode());
final java.lang.Object $invoiceSettings = this.getInvoiceSettings();
result = result * PRIME + ($invoiceSettings == null ? 43 : $invoiceSettings.hashCode());
final java.lang.Object $lineItems = this.getLineItems();
result = result * PRIME + ($lineItems == null ? 43 : $lineItems.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $number = this.getNumber();
result = result * PRIME + ($number == null ? 43 : $number.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $onBehalfOf = this.getOnBehalfOf();
result = result * PRIME + ($onBehalfOf == null ? 43 : $onBehalfOf.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $statusTransitions = this.getStatusTransitions();
result = result * PRIME + ($statusTransitions == null ? 43 : $statusTransitions.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
final java.lang.Object $subscriptionData = this.getSubscriptionData();
result = result * PRIME + ($subscriptionData == null ? 43 : $subscriptionData.hashCode());
final java.lang.Object $subscriptionSchedule = this.getSubscriptionSchedule();
result = result * PRIME + ($subscriptionSchedule == null ? 43 : $subscriptionSchedule.hashCode());
final java.lang.Object $totalDetails = this.getTotalDetails();
result = result * PRIME + ($totalDetails == null ? 43 : $totalDetails.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}