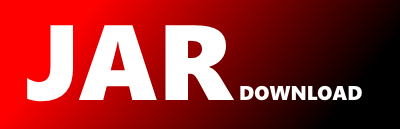
com.stripe.model.issuing.Cardholder Maven / Gradle / Ivy
// Generated by delombok at Fri Nov 19 19:06:04 EST 2021
// File generated from our OpenAPI spec
package com.stripe.model.issuing;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.model.Address;
import com.stripe.model.ExpandableField;
import com.stripe.model.File;
import com.stripe.model.HasId;
import com.stripe.model.MetadataStore;
import com.stripe.model.StripeObject;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.issuing.CardholderCreateParams;
import com.stripe.param.issuing.CardholderListParams;
import com.stripe.param.issuing.CardholderRetrieveParams;
import com.stripe.param.issuing.CardholderUpdateParams;
import java.util.List;
import java.util.Map;
public class Cardholder extends ApiResource implements HasId, MetadataStore {
@SerializedName("billing")
Billing billing;
/**
* Additional information about a {@code company} cardholder.
*/
@SerializedName("company")
Company company;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* The cardholder's email address.
*/
@SerializedName("email")
String email;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
/**
* Additional information about an {@code individual} cardholder.
*/
@SerializedName("individual")
Individual individual;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The cardholder's name. This will be printed on cards issued to them.
*/
@SerializedName("name")
String name;
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code issuing.cardholder}.
*/
@SerializedName("object")
String object;
/**
* The cardholder's phone number. This is required for all cardholders who will be creating EU
* cards. See the 3D
* Secure documentation for more details.
*/
@SerializedName("phone_number")
String phoneNumber;
@SerializedName("requirements")
Requirements requirements;
/**
* Rules that control spending across this cardholder's cards. Refer to our documentation for more
* details.
*/
@SerializedName("spending_controls")
SpendingControls spendingControls;
/**
* Specifies whether to permit authorizations on this cardholder's cards.
*
*
One of {@code active}, {@code blocked}, or {@code inactive}.
*/
@SerializedName("status")
String status;
/**
* One of {@code individual} or {@code company}.
*/
@SerializedName("type")
String type;
/**
* Returns a list of Issuing Cardholder
objects. The objects are sorted in descending
* order by creation date, with the most recently created object appearing first.
*/
public static CardholderCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Issuing Cardholder
objects. The objects are sorted in descending
* order by creation date, with the most recently created object appearing first.
*/
public static CardholderCollection list(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/cardholders");
return ApiResource.requestCollection(url, params, CardholderCollection.class, options);
}
/**
* Returns a list of Issuing Cardholder
objects. The objects are sorted in descending
* order by creation date, with the most recently created object appearing first.
*/
public static CardholderCollection list(CardholderListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* Returns a list of Issuing Cardholder
objects. The objects are sorted in descending
* order by creation date, with the most recently created object appearing first.
*/
public static CardholderCollection list(CardholderListParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/cardholders");
return ApiResource.requestCollection(url, params, CardholderCollection.class, options);
}
/**
* Creates a new Issuing Cardholder
object that can be issued cards.
*/
public static Cardholder create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a new Issuing Cardholder
object that can be issued cards.
*/
public static Cardholder create(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/cardholders");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Cardholder.class, options);
}
/**
* Creates a new Issuing Cardholder
object that can be issued cards.
*/
public static Cardholder create(CardholderCreateParams params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Creates a new Issuing Cardholder
object that can be issued cards.
*/
public static Cardholder create(CardholderCreateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), "/v1/issuing/cardholders");
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Cardholder.class, options);
}
/**
* Retrieves an Issuing Cardholder
object.
*/
public static Cardholder retrieve(String cardholder) throws StripeException {
return retrieve(cardholder, (Map) null, (RequestOptions) null);
}
/**
* Retrieves an Issuing Cardholder
object.
*/
public static Cardholder retrieve(String cardholder, RequestOptions options) throws StripeException {
return retrieve(cardholder, (Map) null, options);
}
/**
* Retrieves an Issuing Cardholder
object.
*/
public static Cardholder retrieve(String cardholder, Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/cardholders/%s", ApiResource.urlEncodeId(cardholder)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Cardholder.class, options);
}
/**
* Retrieves an Issuing Cardholder
object.
*/
public static Cardholder retrieve(String cardholder, CardholderRetrieveParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/cardholders/%s", ApiResource.urlEncodeId(cardholder)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Cardholder.class, options);
}
/**
* Updates the specified Issuing Cardholder
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
@Override
public Cardholder update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified Issuing Cardholder
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
@Override
public Cardholder update(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/cardholders/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Cardholder.class, options);
}
/**
* Updates the specified Issuing Cardholder
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
public Cardholder update(CardholderUpdateParams params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Updates the specified Issuing Cardholder
object by setting the values of the
* parameters passed. Any parameters not provided will be left unchanged.
*/
public Cardholder update(CardholderUpdateParams params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", Stripe.getApiBase(), String.format("/v1/issuing/cardholders/%s", ApiResource.urlEncodeId(this.getId())));
return ApiResource.request(ApiResource.RequestMethod.POST, url, params, Cardholder.class, options);
}
public static class Billing extends StripeObject {
@SerializedName("address")
Address address;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddress(final Address address) {
this.address = address;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Billing)) return false;
final Cardholder.Billing other = (Cardholder.Billing) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Billing;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
return result;
}
}
public static class Company extends StripeObject {
/**
* Whether the company's business ID number was provided.
*/
@SerializedName("tax_id_provided")
Boolean taxIdProvided;
/**
* Whether the company's business ID number was provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getTaxIdProvided() {
return this.taxIdProvided;
}
/**
* Whether the company's business ID number was provided.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxIdProvided(final Boolean taxIdProvided) {
this.taxIdProvided = taxIdProvided;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Company)) return false;
final Cardholder.Company other = (Cardholder.Company) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$taxIdProvided = this.getTaxIdProvided();
final java.lang.Object other$taxIdProvided = other.getTaxIdProvided();
if (this$taxIdProvided == null ? other$taxIdProvided != null : !this$taxIdProvided.equals(other$taxIdProvided)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Company;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $taxIdProvided = this.getTaxIdProvided();
result = result * PRIME + ($taxIdProvided == null ? 43 : $taxIdProvided.hashCode());
return result;
}
}
public static class Individual extends StripeObject {
/**
* The date of birth of this cardholder.
*/
@SerializedName("dob")
DateOfBirth dob;
/**
* The first name of this cardholder.
*/
@SerializedName("first_name")
String firstName;
/**
* The last name of this cardholder.
*/
@SerializedName("last_name")
String lastName;
/**
* Government-issued ID document for this cardholder.
*/
@SerializedName("verification")
Verification verification;
public static class DateOfBirth extends StripeObject {
/**
* The day of birth, between 1 and 31.
*/
@SerializedName("day")
Long day;
/**
* The month of birth, between 1 and 12.
*/
@SerializedName("month")
Long month;
/**
* The four-digit year of birth.
*/
@SerializedName("year")
Long year;
/**
* The day of birth, between 1 and 31.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDay() {
return this.day;
}
/**
* The month of birth, between 1 and 12.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getMonth() {
return this.month;
}
/**
* The four-digit year of birth.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getYear() {
return this.year;
}
/**
* The day of birth, between 1 and 31.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDay(final Long day) {
this.day = day;
}
/**
* The month of birth, between 1 and 12.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMonth(final Long month) {
this.month = month;
}
/**
* The four-digit year of birth.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setYear(final Long year) {
this.year = year;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Individual.DateOfBirth)) return false;
final Cardholder.Individual.DateOfBirth other = (Cardholder.Individual.DateOfBirth) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$day = this.getDay();
final java.lang.Object other$day = other.getDay();
if (this$day == null ? other$day != null : !this$day.equals(other$day)) return false;
final java.lang.Object this$month = this.getMonth();
final java.lang.Object other$month = other.getMonth();
if (this$month == null ? other$month != null : !this$month.equals(other$month)) return false;
final java.lang.Object this$year = this.getYear();
final java.lang.Object other$year = other.getYear();
if (this$year == null ? other$year != null : !this$year.equals(other$year)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Individual.DateOfBirth;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $day = this.getDay();
result = result * PRIME + ($day == null ? 43 : $day.hashCode());
final java.lang.Object $month = this.getMonth();
result = result * PRIME + ($month == null ? 43 : $month.hashCode());
final java.lang.Object $year = this.getYear();
result = result * PRIME + ($year == null ? 43 : $year.hashCode());
return result;
}
}
public static class Verification extends StripeObject {
/** An identifying document, either a passport or local ID card. */
@SerializedName("document")
Document document;
public static class Document extends StripeObject {
/**
* The back of a document returned by a file upload with a {@code purpose}
* value of {@code identity_document}.
*/
@SerializedName("back")
ExpandableField back;
/**
* The front of a document returned by a file upload with a {@code purpose}
* value of {@code identity_document}.
*/
@SerializedName("front")
ExpandableField front;
/** Get ID of expandable {@code back} object. */
public String getBack() {
return (this.back != null) ? this.back.getId() : null;
}
public void setBack(String id) {
this.back = ApiResource.setExpandableFieldId(id, this.back);
}
/** Get expanded {@code back}. */
public File getBackObject() {
return (this.back != null) ? this.back.getExpanded() : null;
}
public void setBackObject(File expandableObject) {
this.back = new ExpandableField(expandableObject.getId(), expandableObject);
}
/** Get ID of expandable {@code front} object. */
public String getFront() {
return (this.front != null) ? this.front.getId() : null;
}
public void setFront(String id) {
this.front = ApiResource.setExpandableFieldId(id, this.front);
}
public File getFrontObject() {
return (this.front != null) ? this.front.getExpanded() : null;
}
public void setFrontObject(File expandableObject) {
this.front = new ExpandableField(expandableObject.getId(), expandableObject);
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Individual.Verification.Document)) return false;
final Cardholder.Individual.Verification.Document other = (Cardholder.Individual.Verification.Document) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$back = this.getBack();
final java.lang.Object other$back = other.getBack();
if (this$back == null ? other$back != null : !this$back.equals(other$back)) return false;
final java.lang.Object this$front = this.getFront();
final java.lang.Object other$front = other.getFront();
if (this$front == null ? other$front != null : !this$front.equals(other$front)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Individual.Verification.Document;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $back = this.getBack();
result = result * PRIME + ($back == null ? 43 : $back.hashCode());
final java.lang.Object $front = this.getFront();
result = result * PRIME + ($front == null ? 43 : $front.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Document getDocument() {
return this.document;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDocument(final Document document) {
this.document = document;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Individual.Verification)) return false;
final Cardholder.Individual.Verification other = (Cardholder.Individual.Verification) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$document = this.getDocument();
final java.lang.Object other$document = other.getDocument();
if (this$document == null ? other$document != null : !this$document.equals(other$document)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Individual.Verification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $document = this.getDocument();
result = result * PRIME + ($document == null ? 43 : $document.hashCode());
return result;
}
}
/**
* The date of birth of this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public DateOfBirth getDob() {
return this.dob;
}
/**
* The first name of this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFirstName() {
return this.firstName;
}
/**
* The last name of this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLastName() {
return this.lastName;
}
/**
* Government-issued ID document for this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Verification getVerification() {
return this.verification;
}
/**
* The date of birth of this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDob(final DateOfBirth dob) {
this.dob = dob;
}
/**
* The first name of this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFirstName(final String firstName) {
this.firstName = firstName;
}
/**
* The last name of this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLastName(final String lastName) {
this.lastName = lastName;
}
/**
* Government-issued ID document for this cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerification(final Verification verification) {
this.verification = verification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Individual)) return false;
final Cardholder.Individual other = (Cardholder.Individual) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$dob = this.getDob();
final java.lang.Object other$dob = other.getDob();
if (this$dob == null ? other$dob != null : !this$dob.equals(other$dob)) return false;
final java.lang.Object this$firstName = this.getFirstName();
final java.lang.Object other$firstName = other.getFirstName();
if (this$firstName == null ? other$firstName != null : !this$firstName.equals(other$firstName)) return false;
final java.lang.Object this$lastName = this.getLastName();
final java.lang.Object other$lastName = other.getLastName();
if (this$lastName == null ? other$lastName != null : !this$lastName.equals(other$lastName)) return false;
final java.lang.Object this$verification = this.getVerification();
final java.lang.Object other$verification = other.getVerification();
if (this$verification == null ? other$verification != null : !this$verification.equals(other$verification)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Individual;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $dob = this.getDob();
result = result * PRIME + ($dob == null ? 43 : $dob.hashCode());
final java.lang.Object $firstName = this.getFirstName();
result = result * PRIME + ($firstName == null ? 43 : $firstName.hashCode());
final java.lang.Object $lastName = this.getLastName();
result = result * PRIME + ($lastName == null ? 43 : $lastName.hashCode());
final java.lang.Object $verification = this.getVerification();
result = result * PRIME + ($verification == null ? 43 : $verification.hashCode());
return result;
}
}
/**
* Get expanded {@code front}.
*/
public static class Requirements extends StripeObject {
/**
* If {@code disabled_reason} is present, all cards will decline authorizations with {@code
* cardholder_verification_required} reason.
*
* One of {@code listed}, {@code rejected.listed}, or {@code under_review}.
*/
@SerializedName("disabled_reason")
String disabledReason;
/**
* Array of fields that need to be collected in order to verify and re-enable the cardholder.
*/
@SerializedName("past_due")
List pastDue;
/**
* If {@code disabled_reason} is present, all cards will decline authorizations with {@code
* cardholder_verification_required} reason.
*
* One of {@code listed}, {@code rejected.listed}, or {@code under_review}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDisabledReason() {
return this.disabledReason;
}
/**
* Array of fields that need to be collected in order to verify and re-enable the cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPastDue() {
return this.pastDue;
}
/**
* If {@code disabled_reason} is present, all cards will decline authorizations with {@code
* cardholder_verification_required} reason.
*
* One of {@code listed}, {@code rejected.listed}, or {@code under_review}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDisabledReason(final String disabledReason) {
this.disabledReason = disabledReason;
}
/**
* Array of fields that need to be collected in order to verify and re-enable the cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPastDue(final List pastDue) {
this.pastDue = pastDue;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.Requirements)) return false;
final Cardholder.Requirements other = (Cardholder.Requirements) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$disabledReason = this.getDisabledReason();
final java.lang.Object other$disabledReason = other.getDisabledReason();
if (this$disabledReason == null ? other$disabledReason != null : !this$disabledReason.equals(other$disabledReason)) return false;
final java.lang.Object this$pastDue = this.getPastDue();
final java.lang.Object other$pastDue = other.getPastDue();
if (this$pastDue == null ? other$pastDue != null : !this$pastDue.equals(other$pastDue)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.Requirements;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $disabledReason = this.getDisabledReason();
result = result * PRIME + ($disabledReason == null ? 43 : $disabledReason.hashCode());
final java.lang.Object $pastDue = this.getPastDue();
result = result * PRIME + ($pastDue == null ? 43 : $pastDue.hashCode());
return result;
}
}
public static class SpendingControls extends StripeObject {
/**
* Array of strings containing categories
* of authorizations to allow. All other categories will be blocked. Cannot be set with {@code
* blocked_categories}.
*/
@SerializedName("allowed_categories")
List allowedCategories;
/**
* Array of strings containing categories
* of authorizations to decline. All other categories will be allowed. Cannot be set with {@code
* allowed_categories}.
*/
@SerializedName("blocked_categories")
List blockedCategories;
/** Limit spending with amount-based rules that apply across this cardholder's cards. */
@SerializedName("spending_limits")
List spendingLimits;
/** Currency of the amounts within {@code spending_limits}. */
@SerializedName("spending_limits_currency")
String spendingLimitsCurrency;
public static class SpendingLimit extends StripeObject {
/** Maximum amount allowed to spend per interval. */
@SerializedName("amount")
Long amount;
/**
* Array of strings containing categories
* this limit applies to. Omitting this field will apply the limit to all categories.
*/
@SerializedName("categories")
List categories;
/**
* Interval (or event) to which the amount applies.
*
* One of {@code all_time}, {@code daily}, {@code monthly}, {@code per_authorization},
* {@code weekly}, or {@code yearly}.
*/
@SerializedName("interval")
String interval;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getCategories() {
return this.categories;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInterval() {
return this.interval;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCategories(final List categories) {
this.categories = categories;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInterval(final String interval) {
this.interval = interval;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.SpendingControls.SpendingLimit)) return false;
final Cardholder.SpendingControls.SpendingLimit other = (Cardholder.SpendingControls.SpendingLimit) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$categories = this.getCategories();
final java.lang.Object other$categories = other.getCategories();
if (this$categories == null ? other$categories != null : !this$categories.equals(other$categories)) return false;
final java.lang.Object this$interval = this.getInterval();
final java.lang.Object other$interval = other.getInterval();
if (this$interval == null ? other$interval != null : !this$interval.equals(other$interval)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.SpendingControls.SpendingLimit;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $categories = this.getCategories();
result = result * PRIME + ($categories == null ? 43 : $categories.hashCode());
final java.lang.Object $interval = this.getInterval();
result = result * PRIME + ($interval == null ? 43 : $interval.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAllowedCategories() {
return this.allowedCategories;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getBlockedCategories() {
return this.blockedCategories;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getSpendingLimits() {
return this.spendingLimits;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSpendingLimitsCurrency() {
return this.spendingLimitsCurrency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAllowedCategories(final List allowedCategories) {
this.allowedCategories = allowedCategories;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBlockedCategories(final List blockedCategories) {
this.blockedCategories = blockedCategories;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSpendingLimits(final List spendingLimits) {
this.spendingLimits = spendingLimits;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSpendingLimitsCurrency(final String spendingLimitsCurrency) {
this.spendingLimitsCurrency = spendingLimitsCurrency;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder.SpendingControls)) return false;
final Cardholder.SpendingControls other = (Cardholder.SpendingControls) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$allowedCategories = this.getAllowedCategories();
final java.lang.Object other$allowedCategories = other.getAllowedCategories();
if (this$allowedCategories == null ? other$allowedCategories != null : !this$allowedCategories.equals(other$allowedCategories)) return false;
final java.lang.Object this$blockedCategories = this.getBlockedCategories();
final java.lang.Object other$blockedCategories = other.getBlockedCategories();
if (this$blockedCategories == null ? other$blockedCategories != null : !this$blockedCategories.equals(other$blockedCategories)) return false;
final java.lang.Object this$spendingLimits = this.getSpendingLimits();
final java.lang.Object other$spendingLimits = other.getSpendingLimits();
if (this$spendingLimits == null ? other$spendingLimits != null : !this$spendingLimits.equals(other$spendingLimits)) return false;
final java.lang.Object this$spendingLimitsCurrency = this.getSpendingLimitsCurrency();
final java.lang.Object other$spendingLimitsCurrency = other.getSpendingLimitsCurrency();
if (this$spendingLimitsCurrency == null ? other$spendingLimitsCurrency != null : !this$spendingLimitsCurrency.equals(other$spendingLimitsCurrency)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder.SpendingControls;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $allowedCategories = this.getAllowedCategories();
result = result * PRIME + ($allowedCategories == null ? 43 : $allowedCategories.hashCode());
final java.lang.Object $blockedCategories = this.getBlockedCategories();
result = result * PRIME + ($blockedCategories == null ? 43 : $blockedCategories.hashCode());
final java.lang.Object $spendingLimits = this.getSpendingLimits();
result = result * PRIME + ($spendingLimits == null ? 43 : $spendingLimits.hashCode());
final java.lang.Object $spendingLimitsCurrency = this.getSpendingLimitsCurrency();
result = result * PRIME + ($spendingLimitsCurrency == null ? 43 : $spendingLimitsCurrency.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Billing getBilling() {
return this.billing;
}
/**
* Additional information about a {@code company} cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Company getCompany() {
return this.company;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
/**
* The cardholder's email address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
/**
* Additional information about an {@code individual} cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Individual getIndividual() {
return this.individual;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* The cardholder's name. This will be printed on cards issued to them.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code issuing.cardholder}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* The cardholder's phone number. This is required for all cardholders who will be creating EU
* cards. See the 3D
* Secure documentation for more details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhoneNumber() {
return this.phoneNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Requirements getRequirements() {
return this.requirements;
}
/**
* Rules that control spending across this cardholder's cards. Refer to our documentation for more
* details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SpendingControls getSpendingControls() {
return this.spendingControls;
}
/**
* Specifies whether to permit authorizations on this cardholder's cards.
*
*
One of {@code active}, {@code blocked}, or {@code inactive}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
/**
* One of {@code individual} or {@code company}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBilling(final Billing billing) {
this.billing = billing;
}
/**
* Additional information about a {@code company} cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCompany(final Company company) {
this.company = company;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* The cardholder's email address.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
/**
* Additional information about an {@code individual} cardholder.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setIndividual(final Individual individual) {
this.individual = individual;
}
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* The cardholder's name. This will be printed on cards issued to them.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*
* Equal to {@code issuing.cardholder}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* The cardholder's phone number. This is required for all cardholders who will be creating EU
* cards. See the 3D
* Secure documentation for more details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPhoneNumber(final String phoneNumber) {
this.phoneNumber = phoneNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRequirements(final Requirements requirements) {
this.requirements = requirements;
}
/**
* Rules that control spending across this cardholder's cards. Refer to our documentation for more
* details.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSpendingControls(final SpendingControls spendingControls) {
this.spendingControls = spendingControls;
}
/**
* Specifies whether to permit authorizations on this cardholder's cards.
*
*
One of {@code active}, {@code blocked}, or {@code inactive}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
/**
* One of {@code individual} or {@code company}.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Cardholder)) return false;
final Cardholder other = (Cardholder) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$billing = this.getBilling();
final java.lang.Object other$billing = other.getBilling();
if (this$billing == null ? other$billing != null : !this$billing.equals(other$billing)) return false;
final java.lang.Object this$company = this.getCompany();
final java.lang.Object other$company = other.getCompany();
if (this$company == null ? other$company != null : !this$company.equals(other$company)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$individual = this.getIndividual();
final java.lang.Object other$individual = other.getIndividual();
if (this$individual == null ? other$individual != null : !this$individual.equals(other$individual)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$phoneNumber = this.getPhoneNumber();
final java.lang.Object other$phoneNumber = other.getPhoneNumber();
if (this$phoneNumber == null ? other$phoneNumber != null : !this$phoneNumber.equals(other$phoneNumber)) return false;
final java.lang.Object this$requirements = this.getRequirements();
final java.lang.Object other$requirements = other.getRequirements();
if (this$requirements == null ? other$requirements != null : !this$requirements.equals(other$requirements)) return false;
final java.lang.Object this$spendingControls = this.getSpendingControls();
final java.lang.Object other$spendingControls = other.getSpendingControls();
if (this$spendingControls == null ? other$spendingControls != null : !this$spendingControls.equals(other$spendingControls)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Cardholder;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $billing = this.getBilling();
result = result * PRIME + ($billing == null ? 43 : $billing.hashCode());
final java.lang.Object $company = this.getCompany();
result = result * PRIME + ($company == null ? 43 : $company.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $individual = this.getIndividual();
result = result * PRIME + ($individual == null ? 43 : $individual.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $phoneNumber = this.getPhoneNumber();
result = result * PRIME + ($phoneNumber == null ? 43 : $phoneNumber.hashCode());
final java.lang.Object $requirements = this.getRequirements();
result = result * PRIME + ($requirements == null ? 43 : $requirements.hashCode());
final java.lang.Object $spendingControls = this.getSpendingControls();
result = result * PRIME + ($spendingControls == null ? 43 : $spendingControls.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}