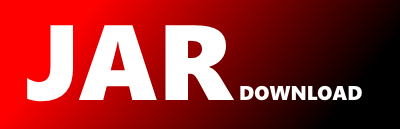
com.stripe.model.Event Maven / Gradle / Ivy
// File generated from our OpenAPI spec
package com.stripe.model;
import com.google.gson.JsonObject;
import com.google.gson.JsonParseException;
import com.google.gson.annotations.SerializedName;
import com.stripe.Stripe;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import com.stripe.param.EventListParams;
import com.stripe.param.EventRetrieveParams;
import java.util.Map;
import lombok.EqualsAndHashCode;
import lombok.Getter;
import lombok.Setter;
/**
* Events are our way of letting you know when something interesting happens in your account. When
* an interesting event occurs, we create a new {@code Event} object. For example, when a charge
* succeeds, we create a {@code charge.succeeded} event; and when an invoice payment attempt fails,
* we create an {@code invoice.payment_failed} event. Note that many API requests may cause multiple
* events to be created. For example, if you create a new subscription for a customer, you will
* receive both a {@code customer.subscription.created} event and a {@code charge.succeeded} event.
*
* Events occur when the state of another API resource changes. The state of that resource at the
* time of the change is embedded in the event's data field. For example, a {@code charge.succeeded}
* event will contain a charge, and an {@code invoice.payment_failed} event will contain an invoice.
*
*
As with other API resources, you can use endpoints to retrieve an individual event or a list of events from the API. We also have a
* separate webhooks system for sending the
* {@code Event} objects directly to an endpoint on your server. Webhooks are managed in your account settings, and our Using Webhooks guide will help you get set up.
*
*
When using Connect, you can also receive
* notifications of events that occur in connected accounts. For these events, there will be an
* additional {@code account} attribute in the received {@code Event} object.
*
*
NOTE: Right now, access to events through the Retrieve Event API is guaranteed only for
* 30 days.
*/
@Getter
@Setter
@EqualsAndHashCode(callSuper = false)
public class Event extends ApiResource implements HasId {
/** The connected account that originated the event. */
@SerializedName("account")
String account;
/**
* The Stripe API version used to render {@code data}. Note: This property is populated only
* for events on or after October 31, 2014.
*/
@SerializedName("api_version")
String apiVersion;
/** Time at which the object was created. Measured in seconds since the Unix epoch. */
@SerializedName("created")
Long created;
@SerializedName("data")
Data data;
/** Unique identifier for the object. */
@Getter(onMethod_ = {@Override})
@SerializedName("id")
String id;
/**
* Has the value {@code true} if the object exists in live mode or the value {@code false} if the
* object exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* String representing the object's type. Objects of the same type share the same value.
*
*
Equal to {@code event}.
*/
@SerializedName("object")
String object;
/**
* Number of webhooks that have yet to be successfully delivered (i.e., to return a 20x response)
* to the URLs you've specified.
*/
@SerializedName("pending_webhooks")
Long pendingWebhooks;
/** Information on the API request that instigated the event. */
@SerializedName("request")
Request request;
/** Description of the event (e.g., {@code invoice.created} or {@code charge.refunded}). */
@SerializedName("type")
String type;
/**
* List events, going back up to 30 days. Each event data is rendered according to Stripe API
* version at its creation time, specified in event object {@code api_version} attribute
* (not according to your current Stripe API version or {@code Stripe-Version} header).
*/
public static EventCollection list(Map params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* List events, going back up to 30 days. Each event data is rendered according to Stripe API
* version at its creation time, specified in event object {@code api_version} attribute
* (not according to your current Stripe API version or {@code Stripe-Version} header).
*/
public static EventCollection list(Map params, RequestOptions options)
throws StripeException {
String url = ApiResource.fullUrl(Stripe.getApiBase(), options, "/v1/events");
return ApiResource.requestCollection(url, params, EventCollection.class, options);
}
/**
* List events, going back up to 30 days. Each event data is rendered according to Stripe API
* version at its creation time, specified in event object {@code api_version} attribute
* (not according to your current Stripe API version or {@code Stripe-Version} header).
*/
public static EventCollection list(EventListParams params) throws StripeException {
return list(params, (RequestOptions) null);
}
/**
* List events, going back up to 30 days. Each event data is rendered according to Stripe API
* version at its creation time, specified in event object {@code api_version} attribute
* (not according to your current Stripe API version or {@code Stripe-Version} header).
*/
public static EventCollection list(EventListParams params, RequestOptions options)
throws StripeException {
String url = ApiResource.fullUrl(Stripe.getApiBase(), options, "/v1/events");
return ApiResource.requestCollection(url, params, EventCollection.class, options);
}
/**
* Retrieves the details of an event. Supply the unique identifier of the event, which you might
* have received in a webhook.
*/
public static Event retrieve(String id) throws StripeException {
return retrieve(id, (Map) null, (RequestOptions) null);
}
/**
* Retrieves the details of an event. Supply the unique identifier of the event, which you might
* have received in a webhook.
*/
public static Event retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, (Map) null, options);
}
/**
* Retrieves the details of an event. Supply the unique identifier of the event, which you might
* have received in a webhook.
*/
public static Event retrieve(String id, Map params, RequestOptions options)
throws StripeException {
String url =
ApiResource.fullUrl(
Stripe.getApiBase(),
options,
String.format("/v1/events/%s", ApiResource.urlEncodeId(id)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Event.class, options);
}
/**
* Retrieves the details of an event. Supply the unique identifier of the event, which you might
* have received in a webhook.
*/
public static Event retrieve(String id, EventRetrieveParams params, RequestOptions options)
throws StripeException {
String url =
ApiResource.fullUrl(
Stripe.getApiBase(),
options,
String.format("/v1/events/%s", ApiResource.urlEncodeId(id)));
return ApiResource.request(ApiResource.RequestMethod.GET, url, params, Event.class, options);
}
/**
* Get deserialization helper to handle failure due to schema incompatibility. When event API
* version matches that of the library's pinned version, the following integration pattern is
* safe.
*
*
* Event event = getEvent(); // either from webhook or event endpoint
* EventDataObjectDeserializer deserializer = event.getDataObjectDeserializer();
* Optional<StripeObject> stripeObject = deserializer.getObject();
*
*
* You can ensure that webhook events has the same API version by creating webhook endpoint
* specifying api version](https://stripe.com/docs/api/webhook_endpoints/create) as {@link
* com.stripe.Stripe#API_VERSION}. For reading from old webhook endpoints or old events with
* potential schema incompatibility, see {@link EventDataObjectDeserializer#deserialize()} and
* {@link EventDataObjectDeserializer#deserializeUnsafe()}.
*/
public EventDataObjectDeserializer getDataObjectDeserializer() {
return new EventDataObjectDeserializer(apiVersion, type, data.object);
}
@Getter
@Setter
@EqualsAndHashCode(callSuper = false)
public static class Data extends StripeObject {
/**
* Object containing the names of the updated attributes and their values prior to the event
* (only included in events of type {@code *.updated}). If an array attribute has any updated
* elements, this object contains the entire array. In Stripe API versions 2017-04-06 or
* earlier, an updated array attribute in this object includes only the updated array elements.
*/
@SerializedName("previous_attributes")
Map previousAttributes;
/**
* Raw JSON object intended to be deserialized as {@code StripeObject}. The deserialization
* should be deferred to the user. See the now deprecated method {@link #getObject()}.
*/
@SerializedName("object")
JsonObject object;
/**
* @deprecated Deprecated in favor of getting {@code StripeObject} from {@link
* Event#getDataObjectDeserializer()} and {@link EventDataObjectDeserializer#getObject()}.
* Throws {@link JsonParseException} deserialization failure due to general invalid JSON, or
* more specifically when JSON data and model class have incompatible schemas.
* @return Deserialized StripeObject for event data.
*/
@Deprecated
public StripeObject getObject() {
return StripeObject.deserializeStripeObject(object);
}
}
@Getter
@Setter
@EqualsAndHashCode(callSuper = false)
public static class Request extends StripeObject implements HasId {
/**
* ID of the API request that caused the event. If null, the event was automatic (e.g., Stripe's
* automatic subscription handling). Request logs are available in the dashboard, but currently not in the API.
*/
@Getter(onMethod_ = {@Override})
@SerializedName("id")
String id;
/**
* The idempotency key transmitted during the request, if any. Note: This property is
* populated only for events on or after May 23, 2017.
*/
@SerializedName("idempotency_key")
String idempotencyKey;
}
}