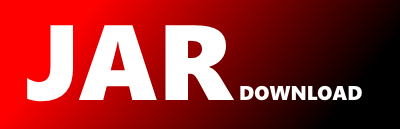
com.stripe.param.SubscriptionItemCreateParams Maven / Gradle / Ivy
// File generated from our OpenAPI spec
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import lombok.Getter;
@Getter
public class SubscriptionItemCreateParams extends ApiRequestParams {
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/** The coupons to redeem into discounts for the subscription item. */
@SerializedName("discounts")
Object discounts;
/** Specifies which fields in the response should be expanded. */
@SerializedName("expand")
List expand;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Set of key-value pairs that you can attach
* to an object. This can be useful for storing additional information about the object in a
* structured format. Individual keys can be unset by posting an empty value to them. All keys can
* be unset by posting an empty value to {@code metadata}.
*/
@SerializedName("metadata")
Map metadata;
/**
* Use {@code allow_incomplete} to transition the subscription to {@code status=past_due} if a
* payment is required but cannot be paid. This allows you to manage scenarios where additional
* user actions are needed to pay a subscription's invoice. For example, SCA regulation may
* require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
* Use {@code default_incomplete} to transition the subscription to {@code status=past_due}
* when payment is required and await explicit confirmation of the invoice's payment intent. This
* allows simpler management of scenarios where additional user actions are needed to pay a
* subscription’s invoice. Such as failed payments, SCA
* regulation, or collecting a mandate for a bank debit payment method.
*
*
Use {@code pending_if_incomplete} to update the subscription using pending updates. When
* you use {@code pending_if_incomplete} you can only pass the parameters supported
* by pending updates.
*
*
Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does not
* update the subscription and returns an error instead. This was the default behavior for API
* versions prior to 2019-03-14. See the changelog to learn more.
*/
@SerializedName("payment_behavior")
PaymentBehavior paymentBehavior;
/** The identifier of the plan to add to the subscription. */
@SerializedName("plan")
String plan;
/** The ID of the price object. */
@SerializedName("price")
String price;
/**
* Data used to generate a new Price object
* inline.
*/
@SerializedName("price_data")
PriceData priceData;
/**
* Determines how to handle prorations when the billing
* cycle changes (e.g., when switching plans, resetting {@code billing_cycle_anchor=now}, or
* starting a trial), or if an item's {@code quantity} changes. The default value is {@code
* create_prorations}.
*/
@SerializedName("proration_behavior")
ProrationBehavior prorationBehavior;
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply the same proration that was previewed with the upcoming invoice endpoint.
*/
@SerializedName("proration_date")
Long prorationDate;
/** The quantity you'd like to apply to the subscription item you're creating. */
@SerializedName("quantity")
Long quantity;
/** Required. The identifier of the subscription to modify. */
@SerializedName("subscription")
String subscription;
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
@SerializedName("tax_rates")
Object taxRates;
/** Options that configure the trial on the subscription item. */
@SerializedName("trial")
Trial trial;
private SubscriptionItemCreateParams(
Object billingThresholds,
Object discounts,
List expand,
Map extraParams,
Map metadata,
PaymentBehavior paymentBehavior,
String plan,
String price,
PriceData priceData,
ProrationBehavior prorationBehavior,
Long prorationDate,
Long quantity,
String subscription,
Object taxRates,
Trial trial) {
this.billingThresholds = billingThresholds;
this.discounts = discounts;
this.expand = expand;
this.extraParams = extraParams;
this.metadata = metadata;
this.paymentBehavior = paymentBehavior;
this.plan = plan;
this.price = price;
this.priceData = priceData;
this.prorationBehavior = prorationBehavior;
this.prorationDate = prorationDate;
this.quantity = quantity;
this.subscription = subscription;
this.taxRates = taxRates;
this.trial = trial;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Object billingThresholds;
private Object discounts;
private List expand;
private Map extraParams;
private Map metadata;
private PaymentBehavior paymentBehavior;
private String plan;
private String price;
private PriceData priceData;
private ProrationBehavior prorationBehavior;
private Long prorationDate;
private Long quantity;
private String subscription;
private Object taxRates;
private Trial trial;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams build() {
return new SubscriptionItemCreateParams(
this.billingThresholds,
this.discounts,
this.expand,
this.extraParams,
this.metadata,
this.paymentBehavior,
this.plan,
this.price,
this.priceData,
this.prorationBehavior,
this.prorationDate,
this.quantity,
this.subscription,
this.taxRates,
this.trial);
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(
SubscriptionItemCreateParams.BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period. When updating, pass an empty string to remove previously-defined thresholds.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Add an element to `discounts` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams#discounts} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addDiscount(SubscriptionItemCreateParams.Discount element) {
if (this.discounts == null || this.discounts instanceof EmptyParam) {
this.discounts = new ArrayList();
}
((List) this.discounts).add(element);
return this;
}
/**
* Add all elements to `discounts` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams#discounts} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllDiscount(List elements) {
if (this.discounts == null || this.discounts instanceof EmptyParam) {
this.discounts = new ArrayList();
}
((List) this.discounts).addAll(elements);
return this;
}
/** The coupons to redeem into discounts for the subscription item. */
public Builder setDiscounts(EmptyParam discounts) {
this.discounts = discounts;
return this;
}
/** The coupons to redeem into discounts for the subscription item. */
public Builder setDiscounts(List discounts) {
this.discounts = discounts;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItemCreateParams#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItemCreateParams#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItemCreateParams#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItemCreateParams#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Use {@code allow_incomplete} to transition the subscription to {@code status=past_due} if a
* payment is required but cannot be paid. This allows you to manage scenarios where additional
* user actions are needed to pay a subscription's invoice. For example, SCA regulation may
* require 3DS authentication to complete payment. See the SCA Migration
* Guide for Billing to learn more. This is the default behavior.
*
* Use {@code default_incomplete} to transition the subscription to {@code status=past_due}
* when payment is required and await explicit confirmation of the invoice's payment intent.
* This allows simpler management of scenarios where additional user actions are needed to pay a
* subscription’s invoice. Such as failed payments, SCA
* regulation, or collecting a mandate for a bank debit payment method.
*
*
Use {@code pending_if_incomplete} to update the subscription using pending updates.
* When you use {@code pending_if_incomplete} you can only pass the parameters supported
* by pending updates.
*
*
Use {@code error_if_incomplete} if you want Stripe to return an HTTP 402 status code if a
* subscription's invoice cannot be paid. For example, if a payment method requires 3DS
* authentication due to SCA regulation and further user action is needed, this parameter does
* not update the subscription and returns an error instead. This was the default behavior for
* API versions prior to 2019-03-14. See the changelog to learn more.
*/
public Builder setPaymentBehavior(
SubscriptionItemCreateParams.PaymentBehavior paymentBehavior) {
this.paymentBehavior = paymentBehavior;
return this;
}
/** The identifier of the plan to add to the subscription. */
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
/** The ID of the price object. */
public Builder setPrice(String price) {
this.price = price;
return this;
}
/**
* Data used to generate a new Price object
* inline.
*/
public Builder setPriceData(SubscriptionItemCreateParams.PriceData priceData) {
this.priceData = priceData;
return this;
}
/**
* Determines how to handle prorations when the
* billing cycle changes (e.g., when switching plans, resetting {@code
* billing_cycle_anchor=now}, or starting a trial), or if an item's {@code quantity} changes.
* The default value is {@code create_prorations}.
*/
public Builder setProrationBehavior(
SubscriptionItemCreateParams.ProrationBehavior prorationBehavior) {
this.prorationBehavior = prorationBehavior;
return this;
}
/**
* If set, the proration will be calculated as though the subscription was updated at the given
* time. This can be used to apply the same proration that was previewed with the upcoming invoice endpoint.
*/
public Builder setProrationDate(Long prorationDate) {
this.prorationDate = prorationDate;
return this;
}
/** The quantity you'd like to apply to the subscription item you're creating. */
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/** Required. The identifier of the subscription to modify. */
public Builder setSubscription(String subscription) {
this.subscription = subscription;
return this;
}
/**
* Add an element to `taxRates` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addTaxRate(String element) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).add(element);
return this;
}
/**
* Add all elements to `taxRates` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams#taxRates} for the field documentation.
*/
@SuppressWarnings("unchecked")
public Builder addAllTaxRate(List elements) {
if (this.taxRates == null || this.taxRates instanceof EmptyParam) {
this.taxRates = new ArrayList();
}
((List) this.taxRates).addAll(elements);
return this;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
public Builder setTaxRates(EmptyParam taxRates) {
this.taxRates = taxRates;
return this;
}
/**
* A list of Tax Rate ids. These Tax Rates
* will override the {@code
* default_tax_rates} on the Subscription. When updating, pass an empty string to remove
* previously-defined tax rates.
*/
public Builder setTaxRates(List taxRates) {
this.taxRates = taxRates;
return this;
}
/** Options that configure the trial on the subscription item. */
public Builder setTrial(SubscriptionItemCreateParams.Trial trial) {
this.trial = trial;
return this;
}
}
@Getter
public static class BillingThresholds {
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Required. Number of units that meets the billing threshold to advance the
* subscription to a new billing period (e.g., it takes 10 $5 units to meet a $50 monetary
* threshold)
*/
@SerializedName("usage_gte")
Long usageGte;
private BillingThresholds(Map extraParams, Long usageGte) {
this.extraParams = extraParams;
this.usageGte = usageGte;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Long usageGte;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.BillingThresholds build() {
return new SubscriptionItemCreateParams.BillingThresholds(this.extraParams, this.usageGte);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItemCreateParams.BillingThresholds#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItemCreateParams.BillingThresholds#extraParams} for the field
* documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Required. Number of units that meets the billing threshold to advance the
* subscription to a new billing period (e.g., it takes 10 $5 units to meet a $50 monetary
* threshold)
*/
public Builder setUsageGte(Long usageGte) {
this.usageGte = usageGte;
return this;
}
}
}
@Getter
public static class Discount {
/** ID of the coupon to create a new discount for. */
@SerializedName("coupon")
String coupon;
/** ID of an existing discount on the object (or one of its ancestors) to reuse. */
@SerializedName("discount")
String discount;
/** Details to determine how long the discount should be applied for. */
@SerializedName("discount_end")
DiscountEnd discountEnd;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** ID of the promotion code to create a new discount for. */
@SerializedName("promotion_code")
String promotionCode;
private Discount(
String coupon,
String discount,
DiscountEnd discountEnd,
Map extraParams,
String promotionCode) {
this.coupon = coupon;
this.discount = discount;
this.discountEnd = discountEnd;
this.extraParams = extraParams;
this.promotionCode = promotionCode;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String coupon;
private String discount;
private DiscountEnd discountEnd;
private Map extraParams;
private String promotionCode;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.Discount build() {
return new SubscriptionItemCreateParams.Discount(
this.coupon, this.discount, this.discountEnd, this.extraParams, this.promotionCode);
}
/** ID of the coupon to create a new discount for. */
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/** ID of an existing discount on the object (or one of its ancestors) to reuse. */
public Builder setDiscount(String discount) {
this.discount = discount;
return this;
}
/** Details to determine how long the discount should be applied for. */
public Builder setDiscountEnd(SubscriptionItemCreateParams.Discount.DiscountEnd discountEnd) {
this.discountEnd = discountEnd;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItemCreateParams.Discount#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItemCreateParams.Discount#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** ID of the promotion code to create a new discount for. */
public Builder setPromotionCode(String promotionCode) {
this.promotionCode = promotionCode;
return this;
}
}
@Getter
public static class DiscountEnd {
/** Time span for the redeemed discount. */
@SerializedName("duration")
Duration duration;
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** A precise Unix timestamp for the discount to end. Must be in the future. */
@SerializedName("timestamp")
Long timestamp;
/**
* Required. The type of calculation made to determine when the discount
* ends.
*/
@SerializedName("type")
Type type;
private DiscountEnd(
Duration duration, Map extraParams, Long timestamp, Type type) {
this.duration = duration;
this.extraParams = extraParams;
this.timestamp = timestamp;
this.type = type;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Duration duration;
private Map extraParams;
private Long timestamp;
private Type type;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.Discount.DiscountEnd build() {
return new SubscriptionItemCreateParams.Discount.DiscountEnd(
this.duration, this.extraParams, this.timestamp, this.type);
}
/** Time span for the redeemed discount. */
public Builder setDuration(
SubscriptionItemCreateParams.Discount.DiscountEnd.Duration duration) {
this.duration = duration;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionItemCreateParams.Discount.DiscountEnd#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionItemCreateParams.Discount.DiscountEnd#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** A precise Unix timestamp for the discount to end. Must be in the future. */
public Builder setTimestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Required. The type of calculation made to determine when the discount
* ends.
*/
public Builder setType(SubscriptionItemCreateParams.Discount.DiscountEnd.Type type) {
this.type = type;
return this;
}
}
@Getter
public static class Duration {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field
* (serialized) name in this param object. Effectively, this map is flattened to its parent
* instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Required. Specifies a type of interval unit. Either {@code day}, {@code
* week}, {@code month} or {@code year}.
*/
@SerializedName("interval")
Interval interval;
/**
* Required. The number of intervals, as an whole number greater than 0.
* Stripe multiplies this by the interval type to get the overall duration.
*/
@SerializedName("interval_count")
Long intervalCount;
private Duration(Map extraParams, Interval interval, Long intervalCount) {
this.extraParams = extraParams;
this.interval = interval;
this.intervalCount = intervalCount;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Interval interval;
private Long intervalCount;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.Discount.DiscountEnd.Duration build() {
return new SubscriptionItemCreateParams.Discount.DiscountEnd.Duration(
this.extraParams, this.interval, this.intervalCount);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionItemCreateParams.Discount.DiscountEnd.Duration#extraParams}
* for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionItemCreateParams.Discount.DiscountEnd.Duration#extraParams}
* for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Required. Specifies a type of interval unit. Either {@code day},
* {@code week}, {@code month} or {@code year}.
*/
public Builder setInterval(
SubscriptionItemCreateParams.Discount.DiscountEnd.Duration.Interval interval) {
this.interval = interval;
return this;
}
/**
* Required. The number of intervals, as an whole number greater than 0.
* Stripe multiplies this by the interval type to get the overall duration.
*/
public Builder setIntervalCount(Long intervalCount) {
this.intervalCount = intervalCount;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("day")
DAY("day"),
@SerializedName("month")
MONTH("month"),
@SerializedName("week")
WEEK("week"),
@SerializedName("year")
YEAR("year");
@Getter(onMethod_ = {@Override})
private final String value;
Interval(String value) {
this.value = value;
}
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("duration")
DURATION("duration"),
@SerializedName("timestamp")
TIMESTAMP("timestamp");
@Getter(onMethod_ = {@Override})
private final String value;
Type(String value) {
this.value = value;
}
}
}
}
@Getter
public static class PriceData {
/**
* Required. Three-letter ISO currency code, in lowercase.
* Must be a supported currency.
*/
@SerializedName("currency")
String currency;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Required. The ID of the product that this price will belong to. */
@SerializedName("product")
String product;
/**
* Required. The recurring components of a price such as {@code interval} and
* {@code interval_count}.
*/
@SerializedName("recurring")
Recurring recurring;
/**
* Only required if a default
* tax behavior was not provided in the Stripe Tax settings. Specifies whether the price is
* considered inclusive of taxes or exclusive of taxes. One of {@code inclusive}, {@code
* exclusive}, or {@code unspecified}. Once specified as either {@code inclusive} or {@code
* exclusive}, it cannot be changed.
*/
@SerializedName("tax_behavior")
TaxBehavior taxBehavior;
/**
* A positive integer in cents (or local equivalent) (or 0 for a free price) representing how
* much to charge.
*/
@SerializedName("unit_amount")
Long unitAmount;
/**
* Same as {@code unit_amount}, but accepts a decimal value in cents (or local equivalent) with
* at most 12 decimal places. Only one of {@code unit_amount} and {@code unit_amount_decimal}
* can be set.
*/
@SerializedName("unit_amount_decimal")
BigDecimal unitAmountDecimal;
private PriceData(
String currency,
Map extraParams,
String product,
Recurring recurring,
TaxBehavior taxBehavior,
Long unitAmount,
BigDecimal unitAmountDecimal) {
this.currency = currency;
this.extraParams = extraParams;
this.product = product;
this.recurring = recurring;
this.taxBehavior = taxBehavior;
this.unitAmount = unitAmount;
this.unitAmountDecimal = unitAmountDecimal;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private String currency;
private Map extraParams;
private String product;
private Recurring recurring;
private TaxBehavior taxBehavior;
private Long unitAmount;
private BigDecimal unitAmountDecimal;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.PriceData build() {
return new SubscriptionItemCreateParams.PriceData(
this.currency,
this.extraParams,
this.product,
this.recurring,
this.taxBehavior,
this.unitAmount,
this.unitAmountDecimal);
}
/**
* Required. Three-letter ISO currency code, in
* lowercase. Must be a supported currency.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItemCreateParams.PriceData#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItemCreateParams.PriceData#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Required. The ID of the product that this price will belong to. */
public Builder setProduct(String product) {
this.product = product;
return this;
}
/**
* Required. The recurring components of a price such as {@code interval} and
* {@code interval_count}.
*/
public Builder setRecurring(SubscriptionItemCreateParams.PriceData.Recurring recurring) {
this.recurring = recurring;
return this;
}
/**
* Only required if a default
* tax behavior was not provided in the Stripe Tax settings. Specifies whether the price
* is considered inclusive of taxes or exclusive of taxes. One of {@code inclusive}, {@code
* exclusive}, or {@code unspecified}. Once specified as either {@code inclusive} or {@code
* exclusive}, it cannot be changed.
*/
public Builder setTaxBehavior(
SubscriptionItemCreateParams.PriceData.TaxBehavior taxBehavior) {
this.taxBehavior = taxBehavior;
return this;
}
/**
* A positive integer in cents (or local equivalent) (or 0 for a free price) representing how
* much to charge.
*/
public Builder setUnitAmount(Long unitAmount) {
this.unitAmount = unitAmount;
return this;
}
/**
* Same as {@code unit_amount}, but accepts a decimal value in cents (or local equivalent)
* with at most 12 decimal places. Only one of {@code unit_amount} and {@code
* unit_amount_decimal} can be set.
*/
public Builder setUnitAmountDecimal(BigDecimal unitAmountDecimal) {
this.unitAmountDecimal = unitAmountDecimal;
return this;
}
}
@Getter
public static class Recurring {
/**
* Map of extra parameters for custom features not available in this client library. The
* content in this map is not serialized under this field's {@code @SerializedName} value.
* Instead, each key/value pair is serialized as if the key is a root-level field (serialized)
* name in this param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/**
* Required. Specifies billing frequency. Either {@code day}, {@code week},
* {@code month} or {@code year}.
*/
@SerializedName("interval")
Interval interval;
/**
* The number of intervals between subscription billings. For example, {@code interval=month}
* and {@code interval_count=3} bills every 3 months. Maximum of three years interval allowed
* (3 years, 36 months, or 156 weeks).
*/
@SerializedName("interval_count")
Long intervalCount;
private Recurring(Map extraParams, Interval interval, Long intervalCount) {
this.extraParams = extraParams;
this.interval = interval;
this.intervalCount = intervalCount;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private Map extraParams;
private Interval interval;
private Long intervalCount;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.PriceData.Recurring build() {
return new SubscriptionItemCreateParams.PriceData.Recurring(
this.extraParams, this.interval, this.intervalCount);
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionItemCreateParams.PriceData.Recurring#extraParams} for the
* field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original
* map. See {@link SubscriptionItemCreateParams.PriceData.Recurring#extraParams} for the
* field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/**
* Required. Specifies billing frequency. Either {@code day}, {@code week},
* {@code month} or {@code year}.
*/
public Builder setInterval(
SubscriptionItemCreateParams.PriceData.Recurring.Interval interval) {
this.interval = interval;
return this;
}
/**
* The number of intervals between subscription billings. For example, {@code
* interval=month} and {@code interval_count=3} bills every 3 months. Maximum of three years
* interval allowed (3 years, 36 months, or 156 weeks).
*/
public Builder setIntervalCount(Long intervalCount) {
this.intervalCount = intervalCount;
return this;
}
}
public enum Interval implements ApiRequestParams.EnumParam {
@SerializedName("day")
DAY("day"),
@SerializedName("month")
MONTH("month"),
@SerializedName("week")
WEEK("week"),
@SerializedName("year")
YEAR("year");
@Getter(onMethod_ = {@Override})
private final String value;
Interval(String value) {
this.value = value;
}
}
}
public enum TaxBehavior implements ApiRequestParams.EnumParam {
@SerializedName("exclusive")
EXCLUSIVE("exclusive"),
@SerializedName("inclusive")
INCLUSIVE("inclusive"),
@SerializedName("unspecified")
UNSPECIFIED("unspecified");
@Getter(onMethod_ = {@Override})
private final String value;
TaxBehavior(String value) {
this.value = value;
}
}
}
@Getter
public static class Trial {
/**
* List of price IDs which, if present on the subscription following a paid trial, constitute
* opting-in to the paid trial. Currently only supports at most 1 price ID.
*/
@SerializedName("converts_to")
List convertsTo;
/**
* Map of extra parameters for custom features not available in this client library. The content
* in this map is not serialized under this field's {@code @SerializedName} value. Instead, each
* key/value pair is serialized as if the key is a root-level field (serialized) name in this
* param object. Effectively, this map is flattened to its parent instance.
*/
@SerializedName(ApiRequestParams.EXTRA_PARAMS_KEY)
Map extraParams;
/** Required. Determines the type of trial for this item. */
@SerializedName("type")
Type type;
private Trial(List convertsTo, Map extraParams, Type type) {
this.convertsTo = convertsTo;
this.extraParams = extraParams;
this.type = type;
}
public static Builder builder() {
return new Builder();
}
public static class Builder {
private List convertsTo;
private Map extraParams;
private Type type;
/** Finalize and obtain parameter instance from this builder. */
public SubscriptionItemCreateParams.Trial build() {
return new SubscriptionItemCreateParams.Trial(this.convertsTo, this.extraParams, this.type);
}
/**
* Add an element to `convertsTo` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams.Trial#convertsTo} for the field documentation.
*/
public Builder addConvertsTo(String element) {
if (this.convertsTo == null) {
this.convertsTo = new ArrayList<>();
}
this.convertsTo.add(element);
return this;
}
/**
* Add all elements to `convertsTo` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* SubscriptionItemCreateParams.Trial#convertsTo} for the field documentation.
*/
public Builder addAllConvertsTo(List elements) {
if (this.convertsTo == null) {
this.convertsTo = new ArrayList<>();
}
this.convertsTo.addAll(elements);
return this;
}
/**
* Add a key/value pair to `extraParams` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItemCreateParams.Trial#extraParams} for the field documentation.
*/
public Builder putExtraParam(String key, Object value) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `extraParams` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItemCreateParams.Trial#extraParams} for the field documentation.
*/
public Builder putAllExtraParam(Map map) {
if (this.extraParams == null) {
this.extraParams = new HashMap<>();
}
this.extraParams.putAll(map);
return this;
}
/** Required. Determines the type of trial for this item. */
public Builder setType(SubscriptionItemCreateParams.Trial.Type type) {
this.type = type;
return this;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("free")
FREE("free"),
@SerializedName("paid")
PAID("paid");
@Getter(onMethod_ = {@Override})
private final String value;
Type(String value) {
this.value = value;
}
}
}
public enum PaymentBehavior implements ApiRequestParams.EnumParam {
@SerializedName("allow_incomplete")
ALLOW_INCOMPLETE("allow_incomplete"),
@SerializedName("default_incomplete")
DEFAULT_INCOMPLETE("default_incomplete"),
@SerializedName("error_if_incomplete")
ERROR_IF_INCOMPLETE("error_if_incomplete"),
@SerializedName("pending_if_incomplete")
PENDING_IF_INCOMPLETE("pending_if_incomplete");
@Getter(onMethod_ = {@Override})
private final String value;
PaymentBehavior(String value) {
this.value = value;
}
}
public enum ProrationBehavior implements ApiRequestParams.EnumParam {
@SerializedName("always_invoice")
ALWAYS_INVOICE("always_invoice"),
@SerializedName("create_prorations")
CREATE_PRORATIONS("create_prorations"),
@SerializedName("none")
NONE("none");
@Getter(onMethod_ = {@Override})
private final String value;
ProrationBehavior(String value) {
this.value = value;
}
}
}