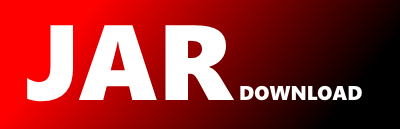
com.stripe.model.Product Maven / Gradle / Ivy
package com.stripe.model;
import java.util.List;
import java.util.Map;
import com.stripe.exception.APIConnectionException;
import com.stripe.exception.APIException;
import com.stripe.exception.AuthenticationException;
import com.stripe.exception.CardException;
import com.stripe.exception.InvalidRequestException;
import com.stripe.net.APIResource;
import com.stripe.net.RequestOptions;
public class Product extends APIResource implements HasId, MetadataStore {
String id;
String object;
Boolean active;
List attributes;
String caption;
Long created;
List deactivateOn;
String description;
List images;
Boolean livemode;
Map metadata;
String name;
PackageDimensions packageDimensions;
Boolean shippable;
SKUCollection skus;
Long updated;
String url;
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public String getObject() {
return object;
}
public void setObject(String object) {
this.object = object;
}
public Boolean getActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public List getAttributes() {
return attributes;
}
public void setAttributes(List attributes) {
this.attributes = attributes;
}
public String getCaption() {
return caption;
}
public void setCaption(String caption) {
this.caption = caption;
}
public Long getCreated() {
return created;
}
public void setCreated(Long created) {
this.created = created;
}
public List getDeactivateOn() {
return deactivateOn;
}
public void setDeactivateOn(List deactivateOn) {
this.deactivateOn = deactivateOn;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public List getImages() {
return images;
}
public void setImages(List images) {
this.images = images;
}
public Boolean getLivemode() {
return livemode;
}
public void setLivemode(Boolean livemode) {
this.livemode = livemode;
}
public Map getMetadata() {
return metadata;
}
public void setMetadata(Map metadata) {
this.metadata = metadata;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public PackageDimensions getPackageDimensions() {
return packageDimensions;
}
public void setPackageDimensions(PackageDimensions packageDimensions) {
this.packageDimensions = packageDimensions;
}
public Boolean getShippable() {
return shippable;
}
public void setShippable(Boolean shippable) {
this.shippable = shippable;
}
public SKUCollection getSkus() {
return skus;
}
public void setSkus(SKUCollection skus) {
this.skus = skus;
}
public Long getUpdated() {
return updated;
}
public void setUpdated(Long updated) {
this.updated = updated;
}
public String getURL() {
return url;
}
public void setURL(String url) {
this.url = url;
}
public static Product create(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return create(params, null);
}
public static Product retrieve(String id)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return retrieve(id, null);
}
public Product update(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return update(params, null);
}
public static Product create(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return request(RequestMethod.POST, classURL(Product.class), params, Product.class, options);
}
public static Product retrieve(String id, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return request(RequestMethod.GET, instanceURL(Product.class, id), null, Product.class, options);
}
public DeletedProduct delete()
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return delete(null);
}
public DeletedProduct delete(RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return request(RequestMethod.DELETE, instanceURL(Product.class, this.id), null, DeletedProduct.class, options);
}
public static ProductCollection list(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return list(params, null);
}
public static ProductCollection list(Map params,
RequestOptions options) throws AuthenticationException,
InvalidRequestException, APIConnectionException, CardException,
APIException {
return requestCollection(classURL(Product.class), params, ProductCollection.class, options);
}
@Deprecated
public static ProductCollection all(Map params)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return list(params, null);
}
@Deprecated
public static ProductCollection all(Map params,
RequestOptions options) throws AuthenticationException,
InvalidRequestException, APIConnectionException, CardException,
APIException {
return list(params, options);
}
public Product update(Map params, RequestOptions options)
throws AuthenticationException, InvalidRequestException,
APIConnectionException, CardException, APIException {
return request(RequestMethod.POST, instanceURL(Product.class, this.id), params, Product.class, options);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy