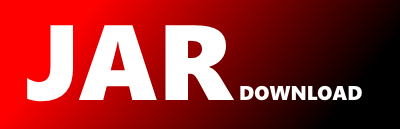
com.stripe.model.PaymentIntent Maven / Gradle / Ivy
// Generated by delombok at Mon Sep 24 15:00:09 CEST 2018
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.List;
import java.util.Map;
public class PaymentIntent extends ApiResource implements MetadataStore, HasId {
String id;
String object;
List allowedSourceTypes;
Long amount;
Long amountCapturable;
Long amountReceived;
ExpandableField application;
Long applicationFeeAmount;
Long canceledAt;
String captureMethod;
ChargeCollection charges;
String clientSecret;
String confirmationMethod;
Long created;
String currency;
ExpandableField customer;
Boolean livemode;
Map metadata;
PaymentIntentSourceAction nextSourceAction;
ExpandableField onBehalfOf;
String receiptEmail;
String returnUrl;
ShippingDetails shipping;
ExpandableField source;
String statementDescriptor;
TransferData transferData;
String status;
//
public String getApplication() {
return (this.application != null) ? this.application.getId() : null;
}
public void setApplication(String applicationId) {
this.application = setExpandableFieldId(applicationId, this.application);
}
public Application getApplicationObject() {
return (this.application != null) ? this.application.getExpanded() : null;
}
public void setApplicationObject(Application c) {
this.application = new ExpandableField(c.getId(), c);
}
//
//
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String customerId) {
this.customer = setExpandableFieldId(customerId, this.customer);
}
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer c) {
this.customer = new ExpandableField(c.getId(), c);
}
//
//
public String getOnBehalfOf() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getId() : null;
}
public void setOnBehalfOf(String onBehalfOfId) {
this.onBehalfOf = setExpandableFieldId(onBehalfOfId, this.onBehalfOf);
}
public Account getOnBehalfOfObject() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getExpanded() : null;
}
public void setOnBehalfOfObject(Account c) {
this.onBehalfOf = new ExpandableField(c.getId(), c);
}
//
//
public String getSource() {
return (this.source != null) ? this.source.getId() : null;
}
public void setSource(String sourceId) {
this.source = setExpandableFieldId(sourceId, this.source);
}
public ExternalAccount getSourceObject() {
return (this.source != null) ? this.source.getExpanded() : null;
}
public void setSourceObject(ExternalAccount c) {
this.source = new ExpandableField(c.getId(), c);
}
//
//
/**
* Cancel a payment intent.
*/
public PaymentIntent cancel() throws StripeException {
return cancel(null, null);
}
/**
* Cancel a payment intent.
*/
public PaymentIntent cancel(Map params) throws StripeException {
return cancel(params, null);
}
/**
* Cancel a payment intent.
*/
public PaymentIntent cancel(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(PaymentIntent.class, this.id) + "/cancel", params, PaymentIntent.class, options);
}
//
//
/**
* Capture a payment intent.
*/
public PaymentIntent capture() throws StripeException {
return capture(null, null);
}
/**
* Capture a payment intent.
*/
public PaymentIntent capture(Map params) throws StripeException {
return capture(params, null);
}
/**
* Capture a payment intent.
*/
public PaymentIntent capture(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(PaymentIntent.class, this.id) + "/capture", params, PaymentIntent.class, options);
}
//
//
/**
* Confirm a payment intent.
*/
public PaymentIntent confirm() throws StripeException {
return confirm(null, null);
}
/**
* Confirm a payment intent.
*/
public PaymentIntent confirm(Map params) throws StripeException {
return confirm(params, null);
}
/**
* Confirm a payment intent.
*/
public PaymentIntent confirm(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(PaymentIntent.class, this.id) + "/confirm", params, PaymentIntent.class, options);
}
//
//
/**
* Create a payment intent.
*/
public static PaymentIntent create(Map params) throws StripeException {
return create(params, null);
}
/**
* Create a payment intent.
*/
public static PaymentIntent create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(PaymentIntent.class), params, PaymentIntent.class, options);
}
//
//
/**
* List all payment intents.
*/
public static PaymentIntentCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all payment intents.
*/
public static PaymentIntentCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(PaymentIntent.class), params, PaymentIntentCollection.class, options);
}
//
//
/**
* Retrieve a payment intent.
*/
public static PaymentIntent retrieve(String id) throws StripeException {
return retrieve(id, null);
}
/**
* Retrieve a payment intent.
*/
public static PaymentIntent retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a payment intent.
*/
public static PaymentIntent retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(PaymentIntent.class, id), params, PaymentIntent.class, options);
}
//
//
/**
* Update a payment intent.
*/
@Override
public PaymentIntent update(Map params) throws StripeException {
return update(params, null);
}
/**
* Update a payment intent.
*/
@Override
public PaymentIntent update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(PaymentIntent.class, this.id), params, PaymentIntent.class, options);
}
//
public static class TransferData extends StripeObject {
Long amount;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentIntent.TransferData)) return false;
final PaymentIntent.TransferData other = (PaymentIntent.TransferData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentIntent.TransferData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAllowedSourceTypes() {
return this.allowedSourceTypes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountCapturable() {
return this.amountCapturable;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountReceived() {
return this.amountReceived;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getApplicationFeeAmount() {
return this.applicationFeeAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCanceledAt() {
return this.canceledAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCaptureMethod() {
return this.captureMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ChargeCollection getCharges() {
return this.charges;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getClientSecret() {
return this.clientSecret;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getConfirmationMethod() {
return this.confirmationMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PaymentIntentSourceAction getNextSourceAction() {
return this.nextSourceAction;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReceiptEmail() {
return this.receiptEmail;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReturnUrl() {
return this.returnUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ShippingDetails getShipping() {
return this.shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAllowedSourceTypes(final List allowedSourceTypes) {
this.allowedSourceTypes = allowedSourceTypes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountCapturable(final Long amountCapturable) {
this.amountCapturable = amountCapturable;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountReceived(final Long amountReceived) {
this.amountReceived = amountReceived;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeeAmount(final Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCanceledAt(final Long canceledAt) {
this.canceledAt = canceledAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCaptureMethod(final String captureMethod) {
this.captureMethod = captureMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCharges(final ChargeCollection charges) {
this.charges = charges;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClientSecret(final String clientSecret) {
this.clientSecret = clientSecret;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setConfirmationMethod(final String confirmationMethod) {
this.confirmationMethod = confirmationMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNextSourceAction(final PaymentIntentSourceAction nextSourceAction) {
this.nextSourceAction = nextSourceAction;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiptEmail(final String receiptEmail) {
this.receiptEmail = receiptEmail;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReturnUrl(final String returnUrl) {
this.returnUrl = returnUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShipping(final ShippingDetails shipping) {
this.shipping = shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final TransferData transferData) {
this.transferData = transferData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof PaymentIntent)) return false;
final PaymentIntent other = (PaymentIntent) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$allowedSourceTypes = this.getAllowedSourceTypes();
final java.lang.Object other$allowedSourceTypes = other.getAllowedSourceTypes();
if (this$allowedSourceTypes == null ? other$allowedSourceTypes != null : !this$allowedSourceTypes.equals(other$allowedSourceTypes)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$amountCapturable = this.getAmountCapturable();
final java.lang.Object other$amountCapturable = other.getAmountCapturable();
if (this$amountCapturable == null ? other$amountCapturable != null : !this$amountCapturable.equals(other$amountCapturable)) return false;
final java.lang.Object this$amountReceived = this.getAmountReceived();
final java.lang.Object other$amountReceived = other.getAmountReceived();
if (this$amountReceived == null ? other$amountReceived != null : !this$amountReceived.equals(other$amountReceived)) return false;
final java.lang.Object this$application = this.getApplication();
final java.lang.Object other$application = other.getApplication();
if (this$application == null ? other$application != null : !this$application.equals(other$application)) return false;
final java.lang.Object this$applicationFeeAmount = this.getApplicationFeeAmount();
final java.lang.Object other$applicationFeeAmount = other.getApplicationFeeAmount();
if (this$applicationFeeAmount == null ? other$applicationFeeAmount != null : !this$applicationFeeAmount.equals(other$applicationFeeAmount)) return false;
final java.lang.Object this$canceledAt = this.getCanceledAt();
final java.lang.Object other$canceledAt = other.getCanceledAt();
if (this$canceledAt == null ? other$canceledAt != null : !this$canceledAt.equals(other$canceledAt)) return false;
final java.lang.Object this$captureMethod = this.getCaptureMethod();
final java.lang.Object other$captureMethod = other.getCaptureMethod();
if (this$captureMethod == null ? other$captureMethod != null : !this$captureMethod.equals(other$captureMethod)) return false;
final java.lang.Object this$charges = this.getCharges();
final java.lang.Object other$charges = other.getCharges();
if (this$charges == null ? other$charges != null : !this$charges.equals(other$charges)) return false;
final java.lang.Object this$clientSecret = this.getClientSecret();
final java.lang.Object other$clientSecret = other.getClientSecret();
if (this$clientSecret == null ? other$clientSecret != null : !this$clientSecret.equals(other$clientSecret)) return false;
final java.lang.Object this$confirmationMethod = this.getConfirmationMethod();
final java.lang.Object other$confirmationMethod = other.getConfirmationMethod();
if (this$confirmationMethod == null ? other$confirmationMethod != null : !this$confirmationMethod.equals(other$confirmationMethod)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$nextSourceAction = this.getNextSourceAction();
final java.lang.Object other$nextSourceAction = other.getNextSourceAction();
if (this$nextSourceAction == null ? other$nextSourceAction != null : !this$nextSourceAction.equals(other$nextSourceAction)) return false;
final java.lang.Object this$onBehalfOf = this.getOnBehalfOf();
final java.lang.Object other$onBehalfOf = other.getOnBehalfOf();
if (this$onBehalfOf == null ? other$onBehalfOf != null : !this$onBehalfOf.equals(other$onBehalfOf)) return false;
final java.lang.Object this$receiptEmail = this.getReceiptEmail();
final java.lang.Object other$receiptEmail = other.getReceiptEmail();
if (this$receiptEmail == null ? other$receiptEmail != null : !this$receiptEmail.equals(other$receiptEmail)) return false;
final java.lang.Object this$returnUrl = this.getReturnUrl();
final java.lang.Object other$returnUrl = other.getReturnUrl();
if (this$returnUrl == null ? other$returnUrl != null : !this$returnUrl.equals(other$returnUrl)) return false;
final java.lang.Object this$shipping = this.getShipping();
final java.lang.Object other$shipping = other.getShipping();
if (this$shipping == null ? other$shipping != null : !this$shipping.equals(other$shipping)) return false;
final java.lang.Object this$source = this.getSource();
final java.lang.Object other$source = other.getSource();
if (this$source == null ? other$source != null : !this$source.equals(other$source)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof PaymentIntent;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $allowedSourceTypes = this.getAllowedSourceTypes();
result = result * PRIME + ($allowedSourceTypes == null ? 43 : $allowedSourceTypes.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $amountCapturable = this.getAmountCapturable();
result = result * PRIME + ($amountCapturable == null ? 43 : $amountCapturable.hashCode());
final java.lang.Object $amountReceived = this.getAmountReceived();
result = result * PRIME + ($amountReceived == null ? 43 : $amountReceived.hashCode());
final java.lang.Object $application = this.getApplication();
result = result * PRIME + ($application == null ? 43 : $application.hashCode());
final java.lang.Object $applicationFeeAmount = this.getApplicationFeeAmount();
result = result * PRIME + ($applicationFeeAmount == null ? 43 : $applicationFeeAmount.hashCode());
final java.lang.Object $canceledAt = this.getCanceledAt();
result = result * PRIME + ($canceledAt == null ? 43 : $canceledAt.hashCode());
final java.lang.Object $captureMethod = this.getCaptureMethod();
result = result * PRIME + ($captureMethod == null ? 43 : $captureMethod.hashCode());
final java.lang.Object $charges = this.getCharges();
result = result * PRIME + ($charges == null ? 43 : $charges.hashCode());
final java.lang.Object $clientSecret = this.getClientSecret();
result = result * PRIME + ($clientSecret == null ? 43 : $clientSecret.hashCode());
final java.lang.Object $confirmationMethod = this.getConfirmationMethod();
result = result * PRIME + ($confirmationMethod == null ? 43 : $confirmationMethod.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $nextSourceAction = this.getNextSourceAction();
result = result * PRIME + ($nextSourceAction == null ? 43 : $nextSourceAction.hashCode());
final java.lang.Object $onBehalfOf = this.getOnBehalfOf();
result = result * PRIME + ($onBehalfOf == null ? 43 : $onBehalfOf.hashCode());
final java.lang.Object $receiptEmail = this.getReceiptEmail();
result = result * PRIME + ($receiptEmail == null ? 43 : $receiptEmail.hashCode());
final java.lang.Object $returnUrl = this.getReturnUrl();
result = result * PRIME + ($returnUrl == null ? 43 : $returnUrl.hashCode());
final java.lang.Object $shipping = this.getShipping();
result = result * PRIME + ($shipping == null ? 43 : $shipping.hashCode());
final java.lang.Object $source = this.getSource();
result = result * PRIME + ($source == null ? 43 : $source.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy