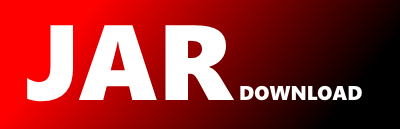
com.stripe.model.Address Maven / Gradle / Ivy
// Generated by delombok at Wed Nov 28 11:15:52 EST 2018
package com.stripe.model;
public final class Address extends StripeObject {
String city;
String country;
String line1;
String line2;
String postalCode;
String state;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCity() {
return this.city;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCountry() {
return this.country;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine1() {
return this.line1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getLine2() {
return this.line2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPostalCode() {
return this.postalCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getState() {
return this.state;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCity(final String city) {
this.city = city;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCountry(final String country) {
this.country = country;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLine1(final String line1) {
this.line1 = line1;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLine2(final String line2) {
this.line2 = line2;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPostalCode(final String postalCode) {
this.postalCode = postalCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setState(final String state) {
this.state = state;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Address)) return false;
final Address other = (Address) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$city = this.getCity();
final java.lang.Object other$city = other.getCity();
if (this$city == null ? other$city != null : !this$city.equals(other$city)) return false;
final java.lang.Object this$country = this.getCountry();
final java.lang.Object other$country = other.getCountry();
if (this$country == null ? other$country != null : !this$country.equals(other$country)) return false;
final java.lang.Object this$line1 = this.getLine1();
final java.lang.Object other$line1 = other.getLine1();
if (this$line1 == null ? other$line1 != null : !this$line1.equals(other$line1)) return false;
final java.lang.Object this$line2 = this.getLine2();
final java.lang.Object other$line2 = other.getLine2();
if (this$line2 == null ? other$line2 != null : !this$line2.equals(other$line2)) return false;
final java.lang.Object this$postalCode = this.getPostalCode();
final java.lang.Object other$postalCode = other.getPostalCode();
if (this$postalCode == null ? other$postalCode != null : !this$postalCode.equals(other$postalCode)) return false;
final java.lang.Object this$state = this.getState();
final java.lang.Object other$state = other.getState();
if (this$state == null ? other$state != null : !this$state.equals(other$state)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Address;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $city = this.getCity();
result = result * PRIME + ($city == null ? 43 : $city.hashCode());
final java.lang.Object $country = this.getCountry();
result = result * PRIME + ($country == null ? 43 : $country.hashCode());
final java.lang.Object $line1 = this.getLine1();
result = result * PRIME + ($line1 == null ? 43 : $line1.hashCode());
final java.lang.Object $line2 = this.getLine2();
result = result * PRIME + ($line2 == null ? 43 : $line2.hashCode());
final java.lang.Object $postalCode = this.getPostalCode();
result = result * PRIME + ($postalCode == null ? 43 : $postalCode.hashCode());
final java.lang.Object $state = this.getState();
result = result * PRIME + ($state == null ? 43 : $state.hashCode());
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy