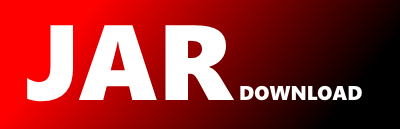
com.stripe.model.Customer Maven / Gradle / Ivy
// Generated by delombok at Wed Nov 28 11:15:52 EST 2018
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.Map;
public class Customer extends ApiResource implements MetadataStore, HasId {
String id;
String object;
Long accountBalance;
Long created;
String currency;
ExpandableField defaultSource;
Boolean deleted;
Boolean delinquent;
String description;
Discount discount;
String email;
String invoicePrefix;
Boolean livemode;
Map metadata;
ShippingDetails shipping;
ExternalAccountCollection sources;
CustomerSubscriptionCollection subscriptions;
TaxInfo taxInfo;
TaxInfoVerification taxInfoVerification;
/**
* The {@code businessVatId} attribute.
*
* @deprecated Prefer using the {@link #taxInfo} attribute instead.
* @see API version 2018-08-23
*/
@Deprecated
String businessVatId;
/**
* The {@code cards} attribute.
*
* @deprecated Prefer using the {@link #sources} attribute instead.
* @see API version 2015-02-18
*/
@Deprecated
CustomerCardCollection cards;
/**
* The {@code default_card} attribute.
*
* @deprecated Prefer using the {@link #defaultSource} attribute instead.
* @see API version 2015-02-18
*/
@Deprecated
String defaultCard;
/**
* The {@code next_recurring_charge} attribute.
*
* @deprecated Prefer using the {@link Invoice#upcoming} method instead.
* @see API version 2012-03-25
*/
@Deprecated
NextRecurringCharge nextRecurringCharge;
/**
* The {@code subscription} attribute.
*
* @deprecated Prefer using the {@link #subscriptions} attribute instead.
* @see API version 2014-01-31
*/
@Deprecated
Subscription subscription;
/**
* The {@code trial_end} attribute.
*
* @deprecated Prefer using the {@link #subscriptions} attribute instead.
* @see API version 2014-01-31
*/
@Deprecated
Long trialEnd;
//
public String getDefaultSource() {
return (this.defaultSource != null) ? this.defaultSource.getId() : null;
}
public void setDefaultSource(String defaultSourceId) {
this.defaultSource = setExpandableFieldId(defaultSourceId, this.defaultSource);
}
public ExternalAccount getDefaultSourceObject() {
return (this.defaultSource != null) ? this.defaultSource.getExpanded() : null;
}
public void setDefaultSourceObject(ExternalAccount c) {
this.defaultSource = new ExpandableField(c.getId(), c);
}
//
//
/**
* Create a customer.
*/
public static Customer create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Create a customer.
*/
public static Customer create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Customer.class), params, Customer.class, options);
}
//
//
/**
* Delete a customer.
*/
public Customer delete() throws StripeException {
return delete((RequestOptions) null);
}
/**
* Delete a customer.
*/
public Customer delete(RequestOptions options) throws StripeException {
return request(RequestMethod.DELETE, instanceUrl(Customer.class, this.id), null, Customer.class, options);
}
//
//
/**
* Delete a customer discount.
*/
public void deleteDiscount() throws StripeException {
deleteDiscount((RequestOptions) null);
}
/**
* Delete a customer discount.
*/
public void deleteDiscount(RequestOptions options) throws StripeException {
request(RequestMethod.DELETE, String.format("%s/discount", instanceUrl(Customer.class, this.id)), null, Discount.class, options);
}
//
//
/**
* List all customers.
*/
public static CustomerCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all customers.
*/
public static CustomerCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(Customer.class), params, CustomerCollection.class, options);
}
//
//
/**
* Retrieve a customer.
*/
public static Customer retrieve(String id) throws StripeException {
return retrieve(id, (RequestOptions) null);
}
/**
* Retrieve a customer.
*/
public static Customer retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a customer.
*/
public static Customer retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Customer.class, id), params, Customer.class, options);
}
//
//
/**
* Update a customer.
*/
@Override
public Customer update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Update a customer.
*/
@Override
public Customer update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Customer.class, this.id), params, Customer.class, options);
}
//
public static class NextRecurringCharge extends StripeObject {
Long amount;
String date;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDate() {
return this.date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDate(final String date) {
this.date = date;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Customer.NextRecurringCharge)) return false;
final Customer.NextRecurringCharge other = (Customer.NextRecurringCharge) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Customer.NextRecurringCharge;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
return result;
}
}
public static class TaxInfo extends StripeObject {
String taxId;
String type;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTaxId() {
return this.taxId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxId(final String taxId) {
this.taxId = taxId;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Customer.TaxInfo)) return false;
final Customer.TaxInfo other = (Customer.TaxInfo) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$taxId = this.getTaxId();
final java.lang.Object other$taxId = other.getTaxId();
if (this$taxId == null ? other$taxId != null : !this$taxId.equals(other$taxId)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Customer.TaxInfo;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $taxId = this.getTaxId();
result = result * PRIME + ($taxId == null ? 43 : $taxId.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}
public static class TaxInfoVerification extends StripeObject {
String status;
String verifiedName;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedName() {
return this.verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedName(final String verifiedName) {
this.verifiedName = verifiedName;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Customer.TaxInfoVerification)) return false;
final Customer.TaxInfoVerification other = (Customer.TaxInfoVerification) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$verifiedName = this.getVerifiedName();
final java.lang.Object other$verifiedName = other.getVerifiedName();
if (this$verifiedName == null ? other$verifiedName != null : !this$verifiedName.equals(other$verifiedName)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Customer.TaxInfoVerification;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $verifiedName = this.getVerifiedName();
result = result * PRIME + ($verifiedName == null ? 43 : $verifiedName.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAccountBalance() {
return this.accountBalance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDelinquent() {
return this.delinquent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Discount getDiscount() {
return this.discount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoicePrefix() {
return this.invoicePrefix;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ShippingDetails getShipping() {
return this.shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ExternalAccountCollection getSources() {
return this.sources;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerSubscriptionCollection getSubscriptions() {
return this.subscriptions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TaxInfo getTaxInfo() {
return this.taxInfo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TaxInfoVerification getTaxInfoVerification() {
return this.taxInfoVerification;
}
/**
* The {@code businessVatId} attribute.
*
* @return the {@code businessVatId} attribute
* @deprecated Prefer using the {@link #taxInfo} attribute instead.
* @see API version 2018-08-23
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBusinessVatId() {
return this.businessVatId;
}
/**
* The {@code cards} attribute.
*
* @return the {@code cards} attribute
* @deprecated Prefer using the {@link #sources} attribute instead.
* @see API version 2015-02-18
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CustomerCardCollection getCards() {
return this.cards;
}
/**
* The {@code default_card} attribute.
*
* @return the {@code default_card} attribute
* @deprecated Prefer using the {@link #defaultSource} attribute instead.
* @see API version 2015-02-18
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDefaultCard() {
return this.defaultCard;
}
/**
* The {@code next_recurring_charge} attribute.
*
* @return the {@code next_recurring_charge} attribute
* @deprecated Prefer using the {@link Invoice#upcoming} method instead.
* @see API version 2012-03-25
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public NextRecurringCharge getNextRecurringCharge() {
return this.nextRecurringCharge;
}
/**
* The {@code subscription} attribute.
*
* @return the {@code subscription} attribute
* @deprecated Prefer using the {@link #subscriptions} attribute instead.
* @see API version 2014-01-31
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Subscription getSubscription() {
return this.subscription;
}
/**
* The {@code trial_end} attribute.
*
* @return the {@code trial_end} attribute
* @deprecated Prefer using the {@link #subscriptions} attribute instead.
* @see API version 2014-01-31
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTrialEnd() {
return this.trialEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccountBalance(final Long accountBalance) {
this.accountBalance = accountBalance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDelinquent(final Boolean delinquent) {
this.delinquent = delinquent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscount(final Discount discount) {
this.discount = discount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoicePrefix(final String invoicePrefix) {
this.invoicePrefix = invoicePrefix;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShipping(final ShippingDetails shipping) {
this.shipping = shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSources(final ExternalAccountCollection sources) {
this.sources = sources;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptions(final CustomerSubscriptionCollection subscriptions) {
this.subscriptions = subscriptions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxInfo(final TaxInfo taxInfo) {
this.taxInfo = taxInfo;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxInfoVerification(final TaxInfoVerification taxInfoVerification) {
this.taxInfoVerification = taxInfoVerification;
}
/**
* The {@code businessVatId} attribute.
*
* @deprecated Prefer using the {@link #taxInfo} attribute instead.
* @see API version 2018-08-23
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBusinessVatId(final String businessVatId) {
this.businessVatId = businessVatId;
}
/**
* The {@code cards} attribute.
*
* @deprecated Prefer using the {@link #sources} attribute instead.
* @see API version 2015-02-18
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCards(final CustomerCardCollection cards) {
this.cards = cards;
}
/**
* The {@code default_card} attribute.
*
* @deprecated Prefer using the {@link #defaultSource} attribute instead.
* @see API version 2015-02-18
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDefaultCard(final String defaultCard) {
this.defaultCard = defaultCard;
}
/**
* The {@code next_recurring_charge} attribute.
*
* @deprecated Prefer using the {@link Invoice#upcoming} method instead.
* @see API version 2012-03-25
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNextRecurringCharge(final NextRecurringCharge nextRecurringCharge) {
this.nextRecurringCharge = nextRecurringCharge;
}
/**
* The {@code subscription} attribute.
*
* @deprecated Prefer using the {@link #subscriptions} attribute instead.
* @see API version 2014-01-31
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscription(final Subscription subscription) {
this.subscription = subscription;
}
/**
* The {@code trial_end} attribute.
*
* @deprecated Prefer using the {@link #subscriptions} attribute instead.
* @see API version 2014-01-31
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTrialEnd(final Long trialEnd) {
this.trialEnd = trialEnd;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Customer)) return false;
final Customer other = (Customer) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$accountBalance = this.getAccountBalance();
final java.lang.Object other$accountBalance = other.getAccountBalance();
if (this$accountBalance == null ? other$accountBalance != null : !this$accountBalance.equals(other$accountBalance)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$defaultSource = this.getDefaultSource();
final java.lang.Object other$defaultSource = other.getDefaultSource();
if (this$defaultSource == null ? other$defaultSource != null : !this$defaultSource.equals(other$defaultSource)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$delinquent = this.getDelinquent();
final java.lang.Object other$delinquent = other.getDelinquent();
if (this$delinquent == null ? other$delinquent != null : !this$delinquent.equals(other$delinquent)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$discount = this.getDiscount();
final java.lang.Object other$discount = other.getDiscount();
if (this$discount == null ? other$discount != null : !this$discount.equals(other$discount)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$invoicePrefix = this.getInvoicePrefix();
final java.lang.Object other$invoicePrefix = other.getInvoicePrefix();
if (this$invoicePrefix == null ? other$invoicePrefix != null : !this$invoicePrefix.equals(other$invoicePrefix)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$shipping = this.getShipping();
final java.lang.Object other$shipping = other.getShipping();
if (this$shipping == null ? other$shipping != null : !this$shipping.equals(other$shipping)) return false;
final java.lang.Object this$sources = this.getSources();
final java.lang.Object other$sources = other.getSources();
if (this$sources == null ? other$sources != null : !this$sources.equals(other$sources)) return false;
final java.lang.Object this$subscriptions = this.getSubscriptions();
final java.lang.Object other$subscriptions = other.getSubscriptions();
if (this$subscriptions == null ? other$subscriptions != null : !this$subscriptions.equals(other$subscriptions)) return false;
final java.lang.Object this$taxInfo = this.getTaxInfo();
final java.lang.Object other$taxInfo = other.getTaxInfo();
if (this$taxInfo == null ? other$taxInfo != null : !this$taxInfo.equals(other$taxInfo)) return false;
final java.lang.Object this$taxInfoVerification = this.getTaxInfoVerification();
final java.lang.Object other$taxInfoVerification = other.getTaxInfoVerification();
if (this$taxInfoVerification == null ? other$taxInfoVerification != null : !this$taxInfoVerification.equals(other$taxInfoVerification)) return false;
final java.lang.Object this$businessVatId = this.getBusinessVatId();
final java.lang.Object other$businessVatId = other.getBusinessVatId();
if (this$businessVatId == null ? other$businessVatId != null : !this$businessVatId.equals(other$businessVatId)) return false;
final java.lang.Object this$cards = this.getCards();
final java.lang.Object other$cards = other.getCards();
if (this$cards == null ? other$cards != null : !this$cards.equals(other$cards)) return false;
final java.lang.Object this$defaultCard = this.getDefaultCard();
final java.lang.Object other$defaultCard = other.getDefaultCard();
if (this$defaultCard == null ? other$defaultCard != null : !this$defaultCard.equals(other$defaultCard)) return false;
final java.lang.Object this$nextRecurringCharge = this.getNextRecurringCharge();
final java.lang.Object other$nextRecurringCharge = other.getNextRecurringCharge();
if (this$nextRecurringCharge == null ? other$nextRecurringCharge != null : !this$nextRecurringCharge.equals(other$nextRecurringCharge)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
final java.lang.Object this$trialEnd = this.getTrialEnd();
final java.lang.Object other$trialEnd = other.getTrialEnd();
if (this$trialEnd == null ? other$trialEnd != null : !this$trialEnd.equals(other$trialEnd)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Customer;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $accountBalance = this.getAccountBalance();
result = result * PRIME + ($accountBalance == null ? 43 : $accountBalance.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $defaultSource = this.getDefaultSource();
result = result * PRIME + ($defaultSource == null ? 43 : $defaultSource.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $delinquent = this.getDelinquent();
result = result * PRIME + ($delinquent == null ? 43 : $delinquent.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $discount = this.getDiscount();
result = result * PRIME + ($discount == null ? 43 : $discount.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $invoicePrefix = this.getInvoicePrefix();
result = result * PRIME + ($invoicePrefix == null ? 43 : $invoicePrefix.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $shipping = this.getShipping();
result = result * PRIME + ($shipping == null ? 43 : $shipping.hashCode());
final java.lang.Object $sources = this.getSources();
result = result * PRIME + ($sources == null ? 43 : $sources.hashCode());
final java.lang.Object $subscriptions = this.getSubscriptions();
result = result * PRIME + ($subscriptions == null ? 43 : $subscriptions.hashCode());
final java.lang.Object $taxInfo = this.getTaxInfo();
result = result * PRIME + ($taxInfo == null ? 43 : $taxInfo.hashCode());
final java.lang.Object $taxInfoVerification = this.getTaxInfoVerification();
result = result * PRIME + ($taxInfoVerification == null ? 43 : $taxInfoVerification.hashCode());
final java.lang.Object $businessVatId = this.getBusinessVatId();
result = result * PRIME + ($businessVatId == null ? 43 : $businessVatId.hashCode());
final java.lang.Object $cards = this.getCards();
result = result * PRIME + ($cards == null ? 43 : $cards.hashCode());
final java.lang.Object $defaultCard = this.getDefaultCard();
result = result * PRIME + ($defaultCard == null ? 43 : $defaultCard.hashCode());
final java.lang.Object $nextRecurringCharge = this.getNextRecurringCharge();
result = result * PRIME + ($nextRecurringCharge == null ? 43 : $nextRecurringCharge.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
final java.lang.Object $trialEnd = this.getTrialEnd();
result = result * PRIME + ($trialEnd == null ? 43 : $trialEnd.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy