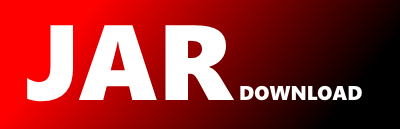
com.stripe.model.Product Maven / Gradle / Ivy
// Generated by delombok at Wed Nov 28 11:15:52 EST 2018
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.List;
import java.util.Map;
public class Product extends ApiResource implements HasId, MetadataStore {
String id;
String object;
Boolean active;
List attributes;
String caption;
Long created;
List deactivateOn;
String description;
List images;
Boolean livemode;
Map metadata;
String name;
PackageDimensions packageDimensions;
Boolean shippable;
SkuCollection skus;
String statementDescriptor;
String type;
Long updated;
String unitLabel;
String url;
Boolean deleted;
//
/**
* Create a product.
*/
public static Product create(Map params) throws StripeException {
return create(params, null);
}
/**
* Create a product.
*/
public static Product create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Product.class), params, Product.class, options);
}
//
//
/**
* Delete a product.
*/
public Product delete() throws StripeException {
return delete(null);
}
/**
* Delete a product.
*/
public Product delete(RequestOptions options) throws StripeException {
return request(RequestMethod.DELETE, instanceUrl(Product.class, this.id), null, Product.class, options);
}
//
//
/**
* List all products.
*/
public static ProductCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all products.
*/
public static ProductCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(Product.class), params, ProductCollection.class, options);
}
//
//
/**
* Retrieve a product.
*/
public static Product retrieve(String id) throws StripeException {
return retrieve(id, null);
}
/**
* Retrieve a product.
*/
public static Product retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a product.
*/
public static Product retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Product.class, id), params, Product.class, options);
}
//
//
/**
* Update a product.
*/
@Override
public Product update(Map params) throws StripeException {
return update(params, null);
}
/**
* Update a product.
*/
@Override
public Product update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Product.class, this.id), params, Product.class, options);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getActive() {
return this.active;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getAttributes() {
return this.attributes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCaption() {
return this.caption;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getDeactivateOn() {
return this.deactivateOn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getImages() {
return this.images;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public PackageDimensions getPackageDimensions() {
return this.packageDimensions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getShippable() {
return this.shippable;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SkuCollection getSkus() {
return this.skus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUpdated() {
return this.updated;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUnitLabel() {
return this.unitLabel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setActive(final Boolean active) {
this.active = active;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttributes(final List attributes) {
this.attributes = attributes;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCaption(final String caption) {
this.caption = caption;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeactivateOn(final List deactivateOn) {
this.deactivateOn = deactivateOn;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setImages(final List images) {
this.images = images;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPackageDimensions(final PackageDimensions packageDimensions) {
this.packageDimensions = packageDimensions;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippable(final Boolean shippable) {
this.shippable = shippable;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSkus(final SkuCollection skus) {
this.skus = skus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUpdated(final Long updated) {
this.updated = updated;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnitLabel(final String unitLabel) {
this.unitLabel = unitLabel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Product)) return false;
final Product other = (Product) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$active = this.getActive();
final java.lang.Object other$active = other.getActive();
if (this$active == null ? other$active != null : !this$active.equals(other$active)) return false;
final java.lang.Object this$attributes = this.getAttributes();
final java.lang.Object other$attributes = other.getAttributes();
if (this$attributes == null ? other$attributes != null : !this$attributes.equals(other$attributes)) return false;
final java.lang.Object this$caption = this.getCaption();
final java.lang.Object other$caption = other.getCaption();
if (this$caption == null ? other$caption != null : !this$caption.equals(other$caption)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$deactivateOn = this.getDeactivateOn();
final java.lang.Object other$deactivateOn = other.getDeactivateOn();
if (this$deactivateOn == null ? other$deactivateOn != null : !this$deactivateOn.equals(other$deactivateOn)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$images = this.getImages();
final java.lang.Object other$images = other.getImages();
if (this$images == null ? other$images != null : !this$images.equals(other$images)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$packageDimensions = this.getPackageDimensions();
final java.lang.Object other$packageDimensions = other.getPackageDimensions();
if (this$packageDimensions == null ? other$packageDimensions != null : !this$packageDimensions.equals(other$packageDimensions)) return false;
final java.lang.Object this$shippable = this.getShippable();
final java.lang.Object other$shippable = other.getShippable();
if (this$shippable == null ? other$shippable != null : !this$shippable.equals(other$shippable)) return false;
final java.lang.Object this$skus = this.getSkus();
final java.lang.Object other$skus = other.getSkus();
if (this$skus == null ? other$skus != null : !this$skus.equals(other$skus)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$updated = this.getUpdated();
final java.lang.Object other$updated = other.getUpdated();
if (this$updated == null ? other$updated != null : !this$updated.equals(other$updated)) return false;
final java.lang.Object this$unitLabel = this.getUnitLabel();
final java.lang.Object other$unitLabel = other.getUnitLabel();
if (this$unitLabel == null ? other$unitLabel != null : !this$unitLabel.equals(other$unitLabel)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Product;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $active = this.getActive();
result = result * PRIME + ($active == null ? 43 : $active.hashCode());
final java.lang.Object $attributes = this.getAttributes();
result = result * PRIME + ($attributes == null ? 43 : $attributes.hashCode());
final java.lang.Object $caption = this.getCaption();
result = result * PRIME + ($caption == null ? 43 : $caption.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $deactivateOn = this.getDeactivateOn();
result = result * PRIME + ($deactivateOn == null ? 43 : $deactivateOn.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $images = this.getImages();
result = result * PRIME + ($images == null ? 43 : $images.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $packageDimensions = this.getPackageDimensions();
result = result * PRIME + ($packageDimensions == null ? 43 : $packageDimensions.hashCode());
final java.lang.Object $shippable = this.getShippable();
result = result * PRIME + ($shippable == null ? 43 : $shippable.hashCode());
final java.lang.Object $skus = this.getSkus();
result = result * PRIME + ($skus == null ? 43 : $skus.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $updated = this.getUpdated();
result = result * PRIME + ($updated == null ? 43 : $updated.hashCode());
final java.lang.Object $unitLabel = this.getUnitLabel();
result = result * PRIME + ($unitLabel == null ? 43 : $unitLabel.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy