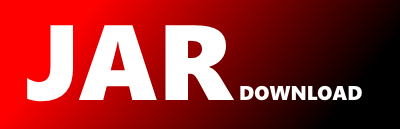
com.stripe.model.Subscription Maven / Gradle / Ivy
// Generated by delombok at Wed Nov 28 11:15:52 EST 2018
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.math.BigDecimal;
import java.util.Map;
public class Subscription extends ApiResource implements MetadataStore, HasId {
String id;
String object;
BigDecimal applicationFeePercent;
String billing;
Long billingCycleAnchor;
Boolean cancelAtPeriodEnd;
Long canceledAt;
Long created;
Long currentPeriodEnd;
Long currentPeriodStart;
ExpandableField customer;
Long daysUntilDue;
ExpandableField defaultSource;
Discount discount;
Long endedAt;
SubscriptionItemCollection items;
Boolean livemode;
Map metadata;
Plan plan;
Long quantity;
Long start;
String status;
BigDecimal taxPercent;
Long trialEnd;
Long trialStart;
//
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String customerId) {
this.customer = setExpandableFieldId(customerId, this.customer);
}
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer c) {
this.customer = new ExpandableField(c.getId(), c);
}
//
//
public String getDefaultSource() {
return (this.defaultSource != null) ? this.defaultSource.getId() : null;
}
public void setDefaultSource(String defaultSourceId) {
this.defaultSource = setExpandableFieldId(defaultSourceId, this.defaultSource);
}
public ExternalAccount getDefaultSourceObject() {
return (this.defaultSource != null) ? this.defaultSource.getExpanded() : null;
}
public void setDefaultSourceObject(ExternalAccount c) {
this.defaultSource = new ExpandableField(c.getId(), c);
}
//
//
public SubscriptionItemCollection getSubscriptionItems() {
return items;
}
public void setSubscriptionItems(SubscriptionItemCollection items) {
this.items = items;
}
//
//
/**
* Cancel a subscription.
*/
public Subscription cancel(Map params) throws StripeException {
return cancel(params, (RequestOptions) null);
}
/**
* Cancel a subscription.
*/
public Subscription cancel(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.DELETE, instanceUrl(Subscription.class, id), params, Subscription.class, options);
}
//
//
/**
* Create a subscription.
*/
public static Subscription create(Map params) throws StripeException {
return create(params, null);
}
/**
* Create a subscription.
*/
public static Subscription create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Subscription.class), params, Subscription.class, options);
}
//
//
/**
* Delete a subscription discount.
*/
public void deleteDiscount() throws StripeException {
deleteDiscount((RequestOptions) null);
}
/**
* Delete a subscription discount.
*/
public void deleteDiscount(RequestOptions options) throws StripeException {
request(RequestMethod.DELETE, String.format("%s/discount", instanceUrl(Subscription.class, id)), null, Discount.class, options);
}
//
//
/**
* List subscriptions.
*/
public static SubscriptionCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List subscriptions.
*/
public static SubscriptionCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(Subscription.class), params, SubscriptionCollection.class, options);
}
//
//
/**
* Retrieve a subscription.
*/
public static Subscription retrieve(String id) throws StripeException {
return retrieve(id, null);
}
/**
* Retrieve a subscription.
*/
public static Subscription retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a subscription.
*/
public static Subscription retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Subscription.class, id), params, Subscription.class, options);
}
//
//
/**
* Update a subscription.
*/
@Override
public Subscription update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Update a subscription.
*/
@Override
public Subscription update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Subscription.class, id), params, Subscription.class, options);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getApplicationFeePercent() {
return this.applicationFeePercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBilling() {
return this.billing;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getBillingCycleAnchor() {
return this.billingCycleAnchor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getCancelAtPeriodEnd() {
return this.cancelAtPeriodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCanceledAt() {
return this.canceledAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCurrentPeriodEnd() {
return this.currentPeriodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCurrentPeriodStart() {
return this.currentPeriodStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDaysUntilDue() {
return this.daysUntilDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Discount getDiscount() {
return this.discount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEndedAt() {
return this.endedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Plan getPlan() {
return this.plan;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStart() {
return this.start;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getTaxPercent() {
return this.taxPercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTrialEnd() {
return this.trialEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTrialStart() {
return this.trialStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeePercent(final BigDecimal applicationFeePercent) {
this.applicationFeePercent = applicationFeePercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBilling(final String billing) {
this.billing = billing;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingCycleAnchor(final Long billingCycleAnchor) {
this.billingCycleAnchor = billingCycleAnchor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCancelAtPeriodEnd(final Boolean cancelAtPeriodEnd) {
this.cancelAtPeriodEnd = cancelAtPeriodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCanceledAt(final Long canceledAt) {
this.canceledAt = canceledAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentPeriodEnd(final Long currentPeriodEnd) {
this.currentPeriodEnd = currentPeriodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrentPeriodStart(final Long currentPeriodStart) {
this.currentPeriodStart = currentPeriodStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDaysUntilDue(final Long daysUntilDue) {
this.daysUntilDue = daysUntilDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscount(final Discount discount) {
this.discount = discount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEndedAt(final Long endedAt) {
this.endedAt = endedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPlan(final Plan plan) {
this.plan = plan;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStart(final Long start) {
this.start = start;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxPercent(final BigDecimal taxPercent) {
this.taxPercent = taxPercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTrialEnd(final Long trialEnd) {
this.trialEnd = trialEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTrialStart(final Long trialStart) {
this.trialStart = trialStart;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Subscription)) return false;
final Subscription other = (Subscription) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$applicationFeePercent = this.getApplicationFeePercent();
final java.lang.Object other$applicationFeePercent = other.getApplicationFeePercent();
if (this$applicationFeePercent == null ? other$applicationFeePercent != null : !this$applicationFeePercent.equals(other$applicationFeePercent)) return false;
final java.lang.Object this$billing = this.getBilling();
final java.lang.Object other$billing = other.getBilling();
if (this$billing == null ? other$billing != null : !this$billing.equals(other$billing)) return false;
final java.lang.Object this$billingCycleAnchor = this.getBillingCycleAnchor();
final java.lang.Object other$billingCycleAnchor = other.getBillingCycleAnchor();
if (this$billingCycleAnchor == null ? other$billingCycleAnchor != null : !this$billingCycleAnchor.equals(other$billingCycleAnchor)) return false;
final java.lang.Object this$cancelAtPeriodEnd = this.getCancelAtPeriodEnd();
final java.lang.Object other$cancelAtPeriodEnd = other.getCancelAtPeriodEnd();
if (this$cancelAtPeriodEnd == null ? other$cancelAtPeriodEnd != null : !this$cancelAtPeriodEnd.equals(other$cancelAtPeriodEnd)) return false;
final java.lang.Object this$canceledAt = this.getCanceledAt();
final java.lang.Object other$canceledAt = other.getCanceledAt();
if (this$canceledAt == null ? other$canceledAt != null : !this$canceledAt.equals(other$canceledAt)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currentPeriodEnd = this.getCurrentPeriodEnd();
final java.lang.Object other$currentPeriodEnd = other.getCurrentPeriodEnd();
if (this$currentPeriodEnd == null ? other$currentPeriodEnd != null : !this$currentPeriodEnd.equals(other$currentPeriodEnd)) return false;
final java.lang.Object this$currentPeriodStart = this.getCurrentPeriodStart();
final java.lang.Object other$currentPeriodStart = other.getCurrentPeriodStart();
if (this$currentPeriodStart == null ? other$currentPeriodStart != null : !this$currentPeriodStart.equals(other$currentPeriodStart)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$daysUntilDue = this.getDaysUntilDue();
final java.lang.Object other$daysUntilDue = other.getDaysUntilDue();
if (this$daysUntilDue == null ? other$daysUntilDue != null : !this$daysUntilDue.equals(other$daysUntilDue)) return false;
final java.lang.Object this$defaultSource = this.getDefaultSource();
final java.lang.Object other$defaultSource = other.getDefaultSource();
if (this$defaultSource == null ? other$defaultSource != null : !this$defaultSource.equals(other$defaultSource)) return false;
final java.lang.Object this$discount = this.getDiscount();
final java.lang.Object other$discount = other.getDiscount();
if (this$discount == null ? other$discount != null : !this$discount.equals(other$discount)) return false;
final java.lang.Object this$endedAt = this.getEndedAt();
final java.lang.Object other$endedAt = other.getEndedAt();
if (this$endedAt == null ? other$endedAt != null : !this$endedAt.equals(other$endedAt)) return false;
final java.lang.Object this$items = this.items;
final java.lang.Object other$items = other.items;
if (this$items == null ? other$items != null : !this$items.equals(other$items)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$plan = this.getPlan();
final java.lang.Object other$plan = other.getPlan();
if (this$plan == null ? other$plan != null : !this$plan.equals(other$plan)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
final java.lang.Object this$start = this.getStart();
final java.lang.Object other$start = other.getStart();
if (this$start == null ? other$start != null : !this$start.equals(other$start)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$taxPercent = this.getTaxPercent();
final java.lang.Object other$taxPercent = other.getTaxPercent();
if (this$taxPercent == null ? other$taxPercent != null : !this$taxPercent.equals(other$taxPercent)) return false;
final java.lang.Object this$trialEnd = this.getTrialEnd();
final java.lang.Object other$trialEnd = other.getTrialEnd();
if (this$trialEnd == null ? other$trialEnd != null : !this$trialEnd.equals(other$trialEnd)) return false;
final java.lang.Object this$trialStart = this.getTrialStart();
final java.lang.Object other$trialStart = other.getTrialStart();
if (this$trialStart == null ? other$trialStart != null : !this$trialStart.equals(other$trialStart)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Subscription;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $applicationFeePercent = this.getApplicationFeePercent();
result = result * PRIME + ($applicationFeePercent == null ? 43 : $applicationFeePercent.hashCode());
final java.lang.Object $billing = this.getBilling();
result = result * PRIME + ($billing == null ? 43 : $billing.hashCode());
final java.lang.Object $billingCycleAnchor = this.getBillingCycleAnchor();
result = result * PRIME + ($billingCycleAnchor == null ? 43 : $billingCycleAnchor.hashCode());
final java.lang.Object $cancelAtPeriodEnd = this.getCancelAtPeriodEnd();
result = result * PRIME + ($cancelAtPeriodEnd == null ? 43 : $cancelAtPeriodEnd.hashCode());
final java.lang.Object $canceledAt = this.getCanceledAt();
result = result * PRIME + ($canceledAt == null ? 43 : $canceledAt.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currentPeriodEnd = this.getCurrentPeriodEnd();
result = result * PRIME + ($currentPeriodEnd == null ? 43 : $currentPeriodEnd.hashCode());
final java.lang.Object $currentPeriodStart = this.getCurrentPeriodStart();
result = result * PRIME + ($currentPeriodStart == null ? 43 : $currentPeriodStart.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $daysUntilDue = this.getDaysUntilDue();
result = result * PRIME + ($daysUntilDue == null ? 43 : $daysUntilDue.hashCode());
final java.lang.Object $defaultSource = this.getDefaultSource();
result = result * PRIME + ($defaultSource == null ? 43 : $defaultSource.hashCode());
final java.lang.Object $discount = this.getDiscount();
result = result * PRIME + ($discount == null ? 43 : $discount.hashCode());
final java.lang.Object $endedAt = this.getEndedAt();
result = result * PRIME + ($endedAt == null ? 43 : $endedAt.hashCode());
final java.lang.Object $items = this.items;
result = result * PRIME + ($items == null ? 43 : $items.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $plan = this.getPlan();
result = result * PRIME + ($plan == null ? 43 : $plan.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
final java.lang.Object $start = this.getStart();
result = result * PRIME + ($start == null ? 43 : $start.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $taxPercent = this.getTaxPercent();
result = result * PRIME + ($taxPercent == null ? 43 : $taxPercent.hashCode());
final java.lang.Object $trialEnd = this.getTrialEnd();
result = result * PRIME + ($trialEnd == null ? 43 : $trialEnd.hashCode());
final java.lang.Object $trialStart = this.getTrialStart();
result = result * PRIME + ($trialStart == null ? 43 : $trialStart.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy