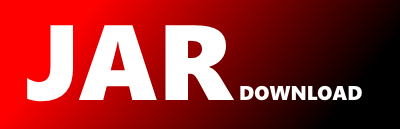
com.stripe.model.Charge Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 16 18:44:45 CET 2019
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.Collections;
import java.util.List;
import java.util.Map;
public class Charge extends ApiResource implements MetadataStore, HasId {
public static final String FRAUD_DETAILS = "fraud_details";
String id;
String object;
Long amount;
Long amountRefunded;
ExpandableField application;
ExpandableField applicationFee;
Long applicationFeeAmount;
AlternateStatementDescriptors alternateStatementDescriptors;
ExpandableField balanceTransaction;
Boolean captured;
Long created;
String currency;
ExpandableField customer;
String description;
ExpandableField destination;
ExpandableField dispute;
String failureCode;
String failureMessage;
FraudDetails fraudDetails;
ExpandableField invoice;
Level3 level3;
Boolean livemode;
Map metadata;
ExpandableField onBehalfOf;
ExpandableField order;
Outcome outcome;
Boolean paid;
String receiptEmail;
String receiptNumber;
String receiptUrl;
Boolean refunded;
ChargeRefundCollection refunds;
ExpandableField review;
ShippingDetails shipping;
ExternalAccount source;
ExpandableField sourceTransfer;
String statementDescriptor;
String status;
ExpandableField transfer;
TransferData transferData;
String transferGroup;
// Please note that these field are for internal use only and are not typically returned
// as part of standard API requests.
String authorizationCode;
/**
* The {@code card} attribute.
*
* @deprecated Prefer using the {@link #source} attribute instead.
* @see API version 2015-02-18
*/
@Deprecated
Card card;
/**
* The {@code disputed} attribute.
*
* @deprecated Prefer using the {@link #dispute} attribute instead.
* @see API version 2012-11-07
*/
@Deprecated
Boolean disputed;
/**
* The {@code statement_description} attribute.
*
* @deprecated Prefer using the {@link #statementDescriptor} attribute instead.
* @see API version 2014-12-17
*/
@Deprecated
String statementDescription;
//
public String getApplication() {
return (this.application != null) ? this.application.getId() : null;
}
public void setApplication(String applicationId) {
this.application = setExpandableFieldId(applicationId, this.application);
}
public Application getApplicationObject() {
return (this.application != null) ? this.application.getExpanded() : null;
}
public void setApplicationObject(Application c) {
this.application = new ExpandableField<>(c.getId(), c);
}
//
//
public String getApplicationFee() {
return (this.applicationFee != null) ? this.applicationFee.getId() : null;
}
public void setApplicationFee(String applicationFeeId) {
this.applicationFee = setExpandableFieldId(applicationFeeId, this.applicationFee);
}
public ApplicationFee getApplicationFeeObject() {
return (this.applicationFee != null) ? this.applicationFee.getExpanded() : null;
}
public void setApplicationFeeObject(ApplicationFee c) {
this.applicationFee = new ExpandableField<>(c.getId(), c);
}
//
//
public String getBalanceTransaction() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getId() : null;
}
public void setBalanceTransaction(String balanceTransactionId) {
this.balanceTransaction = setExpandableFieldId(balanceTransactionId, this.balanceTransaction);
}
public BalanceTransaction getBalanceTransactionObject() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getExpanded() : null;
}
public void setBalanceTransactionObject(BalanceTransaction c) {
this.balanceTransaction = new ExpandableField<>(c.getId(), c);
}
//
//
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String customerId) {
this.customer = setExpandableFieldId(customerId, this.customer);
}
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer c) {
this.customer = new ExpandableField<>(c.getId(), c);
}
//
//
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String destinationId) {
this.destination = ApiResource.setExpandableFieldId(destinationId, this.destination);
}
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account c) {
this.destination = new ExpandableField<>(c.getId(), c);
}
//
//
public String getDispute() {
return (this.dispute != null) ? this.dispute.getId() : null;
}
public void setDispute(String disputeId) {
this.dispute = setExpandableFieldId(disputeId, this.dispute);
}
public Dispute getDisputeObject() {
return (this.dispute != null) ? this.dispute.getExpanded() : null;
}
public void setDisputeObject(Dispute dispute) {
this.dispute = new ExpandableField<>(dispute.getId(), dispute);
}
//
//
public String getInvoice() {
return (this.invoice != null) ? this.invoice.getId() : null;
}
public void setInvoice(String invoiceId) {
this.invoice = setExpandableFieldId(invoiceId, this.invoice);
}
public Invoice getInvoiceObject() {
return (this.invoice != null) ? this.invoice.getExpanded() : null;
}
public void setInvoiceObject(Invoice c) {
this.invoice = new ExpandableField<>(c.getId(), c);
}
//
//
public String getOnBehalfOf() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getId() : null;
}
public void setOnBehalfOf(String onBehalfOfId) {
this.onBehalfOf = ApiResource.setExpandableFieldId(onBehalfOfId, this.onBehalfOf);
}
public Account getOnBehalfOfObject() {
return (this.onBehalfOf != null) ? this.onBehalfOf.getExpanded() : null;
}
public void setOnBehalfOfObject(Account c) {
this.onBehalfOf = new ExpandableField<>(c.getId(), c);
}
//
//
public String getOrder() {
return (this.order != null) ? this.order.getId() : null;
}
public void setOrder(String orderId) {
this.order = setExpandableFieldId(orderId, this.order);
}
public Order getOrderObject() {
return (this.order != null) ? this.order.getExpanded() : null;
}
public void setOrderObject(Order c) {
this.order = new ExpandableField<>(c.getId(), c);
}
//
/**
* Returns the {@code refunds} list.
*
* @return the {@code refunds} list
*/
public ChargeRefundCollection getRefunds() {
// API versions 2014-05-19 and earlier render charge refunds as an array
// instead of an object, meaning there is no sublist URL.
if (refunds != null && refunds.getUrl() == null) {
refunds.setUrl(String.format("/v1/charges/%s/refunds", getId()));
}
return refunds;
}
//
public String getReview() {
return (this.review != null) ? this.review.getId() : null;
}
public void setReview(String reviewId) {
this.review = setExpandableFieldId(reviewId, this.review);
}
public Review getReviewObject() {
return (this.review != null) ? this.review.getExpanded() : null;
}
public void setReviewObject(Review r) {
this.review = new ExpandableField<>(r.getId(), r);
}
//
//
public String getSourceTransfer() {
return (this.sourceTransfer != null) ? this.sourceTransfer.getId() : null;
}
public void setSourceTransfer(String sourceTransferId) {
this.sourceTransfer = setExpandableFieldId(sourceTransferId, this.sourceTransfer);
}
public Transfer getSourceTransferObject() {
return (this.sourceTransfer != null) ? this.sourceTransfer.getExpanded() : null;
}
public void setSourceTransferObject(Transfer c) {
this.sourceTransfer = new ExpandableField<>(c.getId(), c);
}
//
//
public String getTransfer() {
return (this.transfer != null) ? this.transfer.getId() : null;
}
public void setTransfer(String transferId) {
this.transfer = setExpandableFieldId(transferId, this.transfer);
}
public Transfer getTransferObject() {
return (this.transfer != null) ? this.transfer.getExpanded() : null;
}
public void setTransferObject(Transfer c) {
this.transfer = new ExpandableField<>(c.getId(), c);
}
//
//
/**
* Capture a charge.
*/
public Charge capture() throws StripeException {
return this.capture(null, (RequestOptions) null);
}
/**
* Capture a charge.
*/
public Charge capture(RequestOptions options) throws StripeException {
return this.capture(null, options);
}
/**
* Capture a charge.
*/
public Charge capture(Map params) throws StripeException {
return this.capture(params, (RequestOptions) null);
}
/**
* Capture a charge.
*/
public Charge capture(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/capture", instanceUrl(Charge.class, this.getId())), params, Charge.class, options);
}
//
//
/**
* Create a charge.
*/
public static Charge create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Create a charge.
*/
public static Charge create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Charge.class), params, Charge.class, options);
}
//
//
/**
* List all charges.
*/
public static ChargeCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all charges.
*/
public static ChargeCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(Charge.class), params, ChargeCollection.class, options);
}
//
//
/**
* Mark the charge as fraudulent.
*/
public Charge markFraudulent(RequestOptions options) throws StripeException {
Map params = Collections.singletonMap(FRAUD_DETAILS, Collections.singletonMap(FraudDetails.USER_REPORT, "fraudulent"));
return this.update(params, options);
}
//
//
/**
* Mark the charge as safe.
*/
public Charge markSafe(RequestOptions options) throws StripeException {
Map params = Collections.singletonMap(FRAUD_DETAILS, Collections.singletonMap(FraudDetails.USER_REPORT, "safe"));
return this.update(params, options);
}
//
//
/**
* Refund the charge.
*
* @deprecated Prefer using the {@link Refund#create(Map)} method instead.
*/
@Deprecated
public Charge refund() throws StripeException {
return this.refund(null, (RequestOptions) null);
}
/**
* Refund the charge.
*
* @deprecated Prefer using the {@link Refund#create(Map, RequestOptions)} method instead.
*/
@Deprecated
public Charge refund(RequestOptions options) throws StripeException {
return this.refund(null, options);
}
/**
* Refund the charge.
*
* @deprecated Prefer using the {@link Refund#create(Map)} method instead.
*/
@Deprecated
public Charge refund(Map params) throws StripeException {
return this.refund(params, (RequestOptions) null);
}
/**
* Refund the charge.
*
* @deprecated Prefer using the {@link Refund#create(Map, RequestOptions)} method instead.
*/
@Deprecated
public Charge refund(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/refund", instanceUrl(Charge.class, this.getId())), params, Charge.class, options);
}
//
//
/**
* Retrieve a charge.
*/
public static Charge retrieve(String id) throws StripeException {
return retrieve(id, (RequestOptions) null);
}
/**
* Retrieve a charge.
*/
public static Charge retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a charge.
*/
public static Charge retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Charge.class, id), params, Charge.class, options);
}
//
//
/**
* Update a charge.
*/
@Override
public Charge update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Update a charge.
*/
@Override
public Charge update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Charge.class, id), params, Charge.class, options);
}
//
public static class AlternateStatementDescriptors extends StripeObject {
String kana;
String kanji;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getKana() {
return this.kana;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getKanji() {
return this.kanji;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setKana(final String kana) {
this.kana = kana;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setKanji(final String kanji) {
this.kanji = kanji;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.AlternateStatementDescriptors)) return false;
final Charge.AlternateStatementDescriptors other = (Charge.AlternateStatementDescriptors) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$kana = this.getKana();
final java.lang.Object other$kana = other.getKana();
if (this$kana == null ? other$kana != null : !this$kana.equals(other$kana)) return false;
final java.lang.Object this$kanji = this.getKanji();
final java.lang.Object other$kanji = other.getKanji();
if (this$kanji == null ? other$kanji != null : !this$kanji.equals(other$kanji)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.AlternateStatementDescriptors;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $kana = this.getKana();
result = result * PRIME + ($kana == null ? 43 : $kana.hashCode());
final java.lang.Object $kanji = this.getKanji();
result = result * PRIME + ($kanji == null ? 43 : $kanji.hashCode());
return result;
}
}
public static class FraudDetails extends StripeObject {
public static final String USER_REPORT = "user_report";
String userReport;
String stripeReport;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUserReport() {
return this.userReport;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStripeReport() {
return this.stripeReport;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUserReport(final String userReport) {
this.userReport = userReport;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStripeReport(final String stripeReport) {
this.stripeReport = stripeReport;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.FraudDetails)) return false;
final Charge.FraudDetails other = (Charge.FraudDetails) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$userReport = this.getUserReport();
final java.lang.Object other$userReport = other.getUserReport();
if (this$userReport == null ? other$userReport != null : !this$userReport.equals(other$userReport)) return false;
final java.lang.Object this$stripeReport = this.getStripeReport();
final java.lang.Object other$stripeReport = other.getStripeReport();
if (this$stripeReport == null ? other$stripeReport != null : !this$stripeReport.equals(other$stripeReport)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.FraudDetails;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $userReport = this.getUserReport();
result = result * PRIME + ($userReport == null ? 43 : $userReport.hashCode());
final java.lang.Object $stripeReport = this.getStripeReport();
result = result * PRIME + ($stripeReport == null ? 43 : $stripeReport.hashCode());
return result;
}
}
public static class Level3 extends StripeObject {
String customerReference;
List lineItems;
String merchantReference;
String shippingAddressZip;
String shippingFromZip;
Long shippingAmount;
public static class LineItem extends StripeObject {
Long discountAmount;
String productCode;
String productDescription;
Long quantity;
Long taxAmount;
Long unitCost;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDiscountAmount() {
return this.discountAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProductCode() {
return this.productCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getProductDescription() {
return this.productDescription;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getQuantity() {
return this.quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTaxAmount() {
return this.taxAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUnitCost() {
return this.unitCost;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscountAmount(final Long discountAmount) {
this.discountAmount = discountAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProductCode(final String productCode) {
this.productCode = productCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setProductDescription(final String productDescription) {
this.productDescription = productDescription;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setQuantity(final Long quantity) {
this.quantity = quantity;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxAmount(final Long taxAmount) {
this.taxAmount = taxAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUnitCost(final Long unitCost) {
this.unitCost = unitCost;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.Level3.LineItem)) return false;
final Charge.Level3.LineItem other = (Charge.Level3.LineItem) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$discountAmount = this.getDiscountAmount();
final java.lang.Object other$discountAmount = other.getDiscountAmount();
if (this$discountAmount == null ? other$discountAmount != null : !this$discountAmount.equals(other$discountAmount)) return false;
final java.lang.Object this$productCode = this.getProductCode();
final java.lang.Object other$productCode = other.getProductCode();
if (this$productCode == null ? other$productCode != null : !this$productCode.equals(other$productCode)) return false;
final java.lang.Object this$productDescription = this.getProductDescription();
final java.lang.Object other$productDescription = other.getProductDescription();
if (this$productDescription == null ? other$productDescription != null : !this$productDescription.equals(other$productDescription)) return false;
final java.lang.Object this$quantity = this.getQuantity();
final java.lang.Object other$quantity = other.getQuantity();
if (this$quantity == null ? other$quantity != null : !this$quantity.equals(other$quantity)) return false;
final java.lang.Object this$taxAmount = this.getTaxAmount();
final java.lang.Object other$taxAmount = other.getTaxAmount();
if (this$taxAmount == null ? other$taxAmount != null : !this$taxAmount.equals(other$taxAmount)) return false;
final java.lang.Object this$unitCost = this.getUnitCost();
final java.lang.Object other$unitCost = other.getUnitCost();
if (this$unitCost == null ? other$unitCost != null : !this$unitCost.equals(other$unitCost)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.Level3.LineItem;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $discountAmount = this.getDiscountAmount();
result = result * PRIME + ($discountAmount == null ? 43 : $discountAmount.hashCode());
final java.lang.Object $productCode = this.getProductCode();
result = result * PRIME + ($productCode == null ? 43 : $productCode.hashCode());
final java.lang.Object $productDescription = this.getProductDescription();
result = result * PRIME + ($productDescription == null ? 43 : $productDescription.hashCode());
final java.lang.Object $quantity = this.getQuantity();
result = result * PRIME + ($quantity == null ? 43 : $quantity.hashCode());
final java.lang.Object $taxAmount = this.getTaxAmount();
result = result * PRIME + ($taxAmount == null ? 43 : $taxAmount.hashCode());
final java.lang.Object $unitCost = this.getUnitCost();
result = result * PRIME + ($unitCost == null ? 43 : $unitCost.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCustomerReference() {
return this.customerReference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getLineItems() {
return this.lineItems;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getMerchantReference() {
return this.merchantReference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getShippingAddressZip() {
return this.shippingAddressZip;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getShippingFromZip() {
return this.shippingFromZip;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getShippingAmount() {
return this.shippingAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomerReference(final String customerReference) {
this.customerReference = customerReference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLineItems(final List lineItems) {
this.lineItems = lineItems;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMerchantReference(final String merchantReference) {
this.merchantReference = merchantReference;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippingAddressZip(final String shippingAddressZip) {
this.shippingAddressZip = shippingAddressZip;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippingFromZip(final String shippingFromZip) {
this.shippingFromZip = shippingFromZip;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShippingAmount(final Long shippingAmount) {
this.shippingAmount = shippingAmount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.Level3)) return false;
final Charge.Level3 other = (Charge.Level3) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$customerReference = this.getCustomerReference();
final java.lang.Object other$customerReference = other.getCustomerReference();
if (this$customerReference == null ? other$customerReference != null : !this$customerReference.equals(other$customerReference)) return false;
final java.lang.Object this$lineItems = this.getLineItems();
final java.lang.Object other$lineItems = other.getLineItems();
if (this$lineItems == null ? other$lineItems != null : !this$lineItems.equals(other$lineItems)) return false;
final java.lang.Object this$merchantReference = this.getMerchantReference();
final java.lang.Object other$merchantReference = other.getMerchantReference();
if (this$merchantReference == null ? other$merchantReference != null : !this$merchantReference.equals(other$merchantReference)) return false;
final java.lang.Object this$shippingAddressZip = this.getShippingAddressZip();
final java.lang.Object other$shippingAddressZip = other.getShippingAddressZip();
if (this$shippingAddressZip == null ? other$shippingAddressZip != null : !this$shippingAddressZip.equals(other$shippingAddressZip)) return false;
final java.lang.Object this$shippingFromZip = this.getShippingFromZip();
final java.lang.Object other$shippingFromZip = other.getShippingFromZip();
if (this$shippingFromZip == null ? other$shippingFromZip != null : !this$shippingFromZip.equals(other$shippingFromZip)) return false;
final java.lang.Object this$shippingAmount = this.getShippingAmount();
final java.lang.Object other$shippingAmount = other.getShippingAmount();
if (this$shippingAmount == null ? other$shippingAmount != null : !this$shippingAmount.equals(other$shippingAmount)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.Level3;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $customerReference = this.getCustomerReference();
result = result * PRIME + ($customerReference == null ? 43 : $customerReference.hashCode());
final java.lang.Object $lineItems = this.getLineItems();
result = result * PRIME + ($lineItems == null ? 43 : $lineItems.hashCode());
final java.lang.Object $merchantReference = this.getMerchantReference();
result = result * PRIME + ($merchantReference == null ? 43 : $merchantReference.hashCode());
final java.lang.Object $shippingAddressZip = this.getShippingAddressZip();
result = result * PRIME + ($shippingAddressZip == null ? 43 : $shippingAddressZip.hashCode());
final java.lang.Object $shippingFromZip = this.getShippingFromZip();
result = result * PRIME + ($shippingFromZip == null ? 43 : $shippingFromZip.hashCode());
final java.lang.Object $shippingAmount = this.getShippingAmount();
result = result * PRIME + ($shippingAmount == null ? 43 : $shippingAmount.hashCode());
return result;
}
}
public static class Outcome extends ApiResource {
String networkStatus;
String reason;
String riskLevel;
Long riskScore;
ExpandableField rule;
String sellerMessage;
String type;
//
/**
* Returns the {@code rule} object, if expanded. If not expanded, use {@link #getRuleId()} to
* get the ID.
*
* @return the {@code rule} ID
* @deprecated In recent API versions, this attribute is no longer automatically expanded.
* Prefer using the {@link #getRuleId()} and {@link #getRuleObject()} methods instead.
* @see API version 2017-02-14
*/
@Deprecated
public Rule getRule() {
return (this.rule != null) ? this.rule.getExpanded() : null;
}
@Deprecated
public void setRule(Rule rule) {
this.rule = new ExpandableField<>(rule.getId(), rule);
}
public String getRuleId() {
return (this.rule != null) ? this.rule.getId() : null;
}
public void setRuleId(String ruleId) {
this.rule = setExpandableFieldId(ruleId, this.rule);
}
public Rule getRuleObject() {
return (this.rule != null) ? this.rule.getExpanded() : null;
}
public void setRuleObject(Rule rule) {
this.rule = new ExpandableField<>(rule.getId(), rule);
}
//
public static class Rule extends StripeObject implements HasId {
String id;
String action;
String predicate;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAction() {
return this.action;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPredicate() {
return this.predicate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAction(final String action) {
this.action = action;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPredicate(final String predicate) {
this.predicate = predicate;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.Outcome.Rule)) return false;
final Charge.Outcome.Rule other = (Charge.Outcome.Rule) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$action = this.getAction();
final java.lang.Object other$action = other.getAction();
if (this$action == null ? other$action != null : !this$action.equals(other$action)) return false;
final java.lang.Object this$predicate = this.getPredicate();
final java.lang.Object other$predicate = other.getPredicate();
if (this$predicate == null ? other$predicate != null : !this$predicate.equals(other$predicate)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.Outcome.Rule;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $action = this.getAction();
result = result * PRIME + ($action == null ? 43 : $action.hashCode());
final java.lang.Object $predicate = this.getPredicate();
result = result * PRIME + ($predicate == null ? 43 : $predicate.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNetworkStatus() {
return this.networkStatus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReason() {
return this.reason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRiskLevel() {
return this.riskLevel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getRiskScore() {
return this.riskScore;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSellerMessage() {
return this.sellerMessage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNetworkStatus(final String networkStatus) {
this.networkStatus = networkStatus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReason(final String reason) {
this.reason = reason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRiskLevel(final String riskLevel) {
this.riskLevel = riskLevel;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRiskScore(final Long riskScore) {
this.riskScore = riskScore;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSellerMessage(final String sellerMessage) {
this.sellerMessage = sellerMessage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.Outcome)) return false;
final Charge.Outcome other = (Charge.Outcome) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$networkStatus = this.getNetworkStatus();
final java.lang.Object other$networkStatus = other.getNetworkStatus();
if (this$networkStatus == null ? other$networkStatus != null : !this$networkStatus.equals(other$networkStatus)) return false;
final java.lang.Object this$reason = this.getReason();
final java.lang.Object other$reason = other.getReason();
if (this$reason == null ? other$reason != null : !this$reason.equals(other$reason)) return false;
final java.lang.Object this$riskLevel = this.getRiskLevel();
final java.lang.Object other$riskLevel = other.getRiskLevel();
if (this$riskLevel == null ? other$riskLevel != null : !this$riskLevel.equals(other$riskLevel)) return false;
final java.lang.Object this$riskScore = this.getRiskScore();
final java.lang.Object other$riskScore = other.getRiskScore();
if (this$riskScore == null ? other$riskScore != null : !this$riskScore.equals(other$riskScore)) return false;
final java.lang.Object this$rule = this.getRule();
final java.lang.Object other$rule = other.getRule();
if (this$rule == null ? other$rule != null : !this$rule.equals(other$rule)) return false;
final java.lang.Object this$sellerMessage = this.getSellerMessage();
final java.lang.Object other$sellerMessage = other.getSellerMessage();
if (this$sellerMessage == null ? other$sellerMessage != null : !this$sellerMessage.equals(other$sellerMessage)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.Outcome;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $networkStatus = this.getNetworkStatus();
result = result * PRIME + ($networkStatus == null ? 43 : $networkStatus.hashCode());
final java.lang.Object $reason = this.getReason();
result = result * PRIME + ($reason == null ? 43 : $reason.hashCode());
final java.lang.Object $riskLevel = this.getRiskLevel();
result = result * PRIME + ($riskLevel == null ? 43 : $riskLevel.hashCode());
final java.lang.Object $riskScore = this.getRiskScore();
result = result * PRIME + ($riskScore == null ? 43 : $riskScore.hashCode());
final java.lang.Object $rule = this.getRule();
result = result * PRIME + ($rule == null ? 43 : $rule.hashCode());
final java.lang.Object $sellerMessage = this.getSellerMessage();
result = result * PRIME + ($sellerMessage == null ? 43 : $sellerMessage.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
return result;
}
}
public static class TransferData extends StripeObject {
Long amount;
ExpandableField destination;
//
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String destinationId) {
this.destination = setExpandableFieldId(destinationId, this.destination);
}
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account c) {
this.destination = new ExpandableField<>(c.getId(), c);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge.TransferData)) return false;
final Charge.TransferData other = (Charge.TransferData) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$destination = this.getDestination();
final java.lang.Object other$destination = other.getDestination();
if (this$destination == null ? other$destination != null : !this$destination.equals(other$destination)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge.TransferData;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $destination = this.getDestination();
result = result * PRIME + ($destination == null ? 43 : $destination.hashCode());
return result;
}
//
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountRefunded() {
return this.amountRefunded;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getApplicationFeeAmount() {
return this.applicationFeeAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public AlternateStatementDescriptors getAlternateStatementDescriptors() {
return this.alternateStatementDescriptors;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getCaptured() {
return this.captured;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFailureCode() {
return this.failureCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFailureMessage() {
return this.failureMessage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public FraudDetails getFraudDetails() {
return this.fraudDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Level3 getLevel3() {
return this.level3;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Outcome getOutcome() {
return this.outcome;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getPaid() {
return this.paid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReceiptEmail() {
return this.receiptEmail;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReceiptNumber() {
return this.receiptNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReceiptUrl() {
return this.receiptUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getRefunded() {
return this.refunded;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ShippingDetails getShipping() {
return this.shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ExternalAccount getSource() {
return this.source;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTransferGroup() {
return this.transferGroup;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAuthorizationCode() {
return this.authorizationCode;
}
/**
* The {@code card} attribute.
*
* @return the {@code card} attribute
* @deprecated Prefer using the {@link #source} attribute instead.
* @see API version 2015-02-18
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Card getCard() {
return this.card;
}
/**
* The {@code disputed} attribute.
*
* @return the {@code disputed} attribute
* @deprecated Prefer using the {@link #dispute} attribute instead.
* @see API version 2012-11-07
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDisputed() {
return this.disputed;
}
/**
* The {@code statement_description} attribute.
*
* @return the {@code cards} attribute
* @deprecated Prefer using the {@link #statementDescriptor} attribute instead.
* @see API version 2014-12-17
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescription() {
return this.statementDescription;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountRefunded(final Long amountRefunded) {
this.amountRefunded = amountRefunded;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeeAmount(final Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAlternateStatementDescriptors(final AlternateStatementDescriptors alternateStatementDescriptors) {
this.alternateStatementDescriptors = alternateStatementDescriptors;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCaptured(final Boolean captured) {
this.captured = captured;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFailureCode(final String failureCode) {
this.failureCode = failureCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFailureMessage(final String failureMessage) {
this.failureMessage = failureMessage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFraudDetails(final FraudDetails fraudDetails) {
this.fraudDetails = fraudDetails;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLevel3(final Level3 level3) {
this.level3 = level3;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOutcome(final Outcome outcome) {
this.outcome = outcome;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaid(final Boolean paid) {
this.paid = paid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiptEmail(final String receiptEmail) {
this.receiptEmail = receiptEmail;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiptNumber(final String receiptNumber) {
this.receiptNumber = receiptNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiptUrl(final String receiptUrl) {
this.receiptUrl = receiptUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefunded(final Boolean refunded) {
this.refunded = refunded;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefunds(final ChargeRefundCollection refunds) {
this.refunds = refunds;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setShipping(final ShippingDetails shipping) {
this.shipping = shipping;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSource(final ExternalAccount source) {
this.source = source;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final TransferData transferData) {
this.transferData = transferData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferGroup(final String transferGroup) {
this.transferGroup = transferGroup;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAuthorizationCode(final String authorizationCode) {
this.authorizationCode = authorizationCode;
}
/**
* The {@code card} attribute.
*
* @deprecated Prefer using the {@link #source} attribute instead.
* @see API version 2015-02-18
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCard(final Card card) {
this.card = card;
}
/**
* The {@code disputed} attribute.
*
* @deprecated Prefer using the {@link #dispute} attribute instead.
* @see API version 2012-11-07
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDisputed(final Boolean disputed) {
this.disputed = disputed;
}
/**
* The {@code statement_description} attribute.
*
* @deprecated Prefer using the {@link #statementDescriptor} attribute instead.
* @see API version 2014-12-17
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescription(final String statementDescription) {
this.statementDescription = statementDescription;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Charge)) return false;
final Charge other = (Charge) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$amountRefunded = this.getAmountRefunded();
final java.lang.Object other$amountRefunded = other.getAmountRefunded();
if (this$amountRefunded == null ? other$amountRefunded != null : !this$amountRefunded.equals(other$amountRefunded)) return false;
final java.lang.Object this$application = this.getApplication();
final java.lang.Object other$application = other.getApplication();
if (this$application == null ? other$application != null : !this$application.equals(other$application)) return false;
final java.lang.Object this$applicationFee = this.getApplicationFee();
final java.lang.Object other$applicationFee = other.getApplicationFee();
if (this$applicationFee == null ? other$applicationFee != null : !this$applicationFee.equals(other$applicationFee)) return false;
final java.lang.Object this$applicationFeeAmount = this.getApplicationFeeAmount();
final java.lang.Object other$applicationFeeAmount = other.getApplicationFeeAmount();
if (this$applicationFeeAmount == null ? other$applicationFeeAmount != null : !this$applicationFeeAmount.equals(other$applicationFeeAmount)) return false;
final java.lang.Object this$alternateStatementDescriptors = this.getAlternateStatementDescriptors();
final java.lang.Object other$alternateStatementDescriptors = other.getAlternateStatementDescriptors();
if (this$alternateStatementDescriptors == null ? other$alternateStatementDescriptors != null : !this$alternateStatementDescriptors.equals(other$alternateStatementDescriptors)) return false;
final java.lang.Object this$balanceTransaction = this.getBalanceTransaction();
final java.lang.Object other$balanceTransaction = other.getBalanceTransaction();
if (this$balanceTransaction == null ? other$balanceTransaction != null : !this$balanceTransaction.equals(other$balanceTransaction)) return false;
final java.lang.Object this$captured = this.getCaptured();
final java.lang.Object other$captured = other.getCaptured();
if (this$captured == null ? other$captured != null : !this$captured.equals(other$captured)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$destination = this.getDestination();
final java.lang.Object other$destination = other.getDestination();
if (this$destination == null ? other$destination != null : !this$destination.equals(other$destination)) return false;
final java.lang.Object this$dispute = this.getDispute();
final java.lang.Object other$dispute = other.getDispute();
if (this$dispute == null ? other$dispute != null : !this$dispute.equals(other$dispute)) return false;
final java.lang.Object this$failureCode = this.getFailureCode();
final java.lang.Object other$failureCode = other.getFailureCode();
if (this$failureCode == null ? other$failureCode != null : !this$failureCode.equals(other$failureCode)) return false;
final java.lang.Object this$failureMessage = this.getFailureMessage();
final java.lang.Object other$failureMessage = other.getFailureMessage();
if (this$failureMessage == null ? other$failureMessage != null : !this$failureMessage.equals(other$failureMessage)) return false;
final java.lang.Object this$fraudDetails = this.getFraudDetails();
final java.lang.Object other$fraudDetails = other.getFraudDetails();
if (this$fraudDetails == null ? other$fraudDetails != null : !this$fraudDetails.equals(other$fraudDetails)) return false;
final java.lang.Object this$invoice = this.getInvoice();
final java.lang.Object other$invoice = other.getInvoice();
if (this$invoice == null ? other$invoice != null : !this$invoice.equals(other$invoice)) return false;
final java.lang.Object this$level3 = this.getLevel3();
final java.lang.Object other$level3 = other.getLevel3();
if (this$level3 == null ? other$level3 != null : !this$level3.equals(other$level3)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$onBehalfOf = this.getOnBehalfOf();
final java.lang.Object other$onBehalfOf = other.getOnBehalfOf();
if (this$onBehalfOf == null ? other$onBehalfOf != null : !this$onBehalfOf.equals(other$onBehalfOf)) return false;
final java.lang.Object this$order = this.getOrder();
final java.lang.Object other$order = other.getOrder();
if (this$order == null ? other$order != null : !this$order.equals(other$order)) return false;
final java.lang.Object this$outcome = this.getOutcome();
final java.lang.Object other$outcome = other.getOutcome();
if (this$outcome == null ? other$outcome != null : !this$outcome.equals(other$outcome)) return false;
final java.lang.Object this$paid = this.getPaid();
final java.lang.Object other$paid = other.getPaid();
if (this$paid == null ? other$paid != null : !this$paid.equals(other$paid)) return false;
final java.lang.Object this$receiptEmail = this.getReceiptEmail();
final java.lang.Object other$receiptEmail = other.getReceiptEmail();
if (this$receiptEmail == null ? other$receiptEmail != null : !this$receiptEmail.equals(other$receiptEmail)) return false;
final java.lang.Object this$receiptNumber = this.getReceiptNumber();
final java.lang.Object other$receiptNumber = other.getReceiptNumber();
if (this$receiptNumber == null ? other$receiptNumber != null : !this$receiptNumber.equals(other$receiptNumber)) return false;
final java.lang.Object this$receiptUrl = this.getReceiptUrl();
final java.lang.Object other$receiptUrl = other.getReceiptUrl();
if (this$receiptUrl == null ? other$receiptUrl != null : !this$receiptUrl.equals(other$receiptUrl)) return false;
final java.lang.Object this$refunded = this.getRefunded();
final java.lang.Object other$refunded = other.getRefunded();
if (this$refunded == null ? other$refunded != null : !this$refunded.equals(other$refunded)) return false;
final java.lang.Object this$refunds = this.getRefunds();
final java.lang.Object other$refunds = other.getRefunds();
if (this$refunds == null ? other$refunds != null : !this$refunds.equals(other$refunds)) return false;
final java.lang.Object this$review = this.getReview();
final java.lang.Object other$review = other.getReview();
if (this$review == null ? other$review != null : !this$review.equals(other$review)) return false;
final java.lang.Object this$shipping = this.getShipping();
final java.lang.Object other$shipping = other.getShipping();
if (this$shipping == null ? other$shipping != null : !this$shipping.equals(other$shipping)) return false;
final java.lang.Object this$source = this.getSource();
final java.lang.Object other$source = other.getSource();
if (this$source == null ? other$source != null : !this$source.equals(other$source)) return false;
final java.lang.Object this$sourceTransfer = this.getSourceTransfer();
final java.lang.Object other$sourceTransfer = other.getSourceTransfer();
if (this$sourceTransfer == null ? other$sourceTransfer != null : !this$sourceTransfer.equals(other$sourceTransfer)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$transfer = this.getTransfer();
final java.lang.Object other$transfer = other.getTransfer();
if (this$transfer == null ? other$transfer != null : !this$transfer.equals(other$transfer)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
final java.lang.Object this$transferGroup = this.getTransferGroup();
final java.lang.Object other$transferGroup = other.getTransferGroup();
if (this$transferGroup == null ? other$transferGroup != null : !this$transferGroup.equals(other$transferGroup)) return false;
final java.lang.Object this$authorizationCode = this.getAuthorizationCode();
final java.lang.Object other$authorizationCode = other.getAuthorizationCode();
if (this$authorizationCode == null ? other$authorizationCode != null : !this$authorizationCode.equals(other$authorizationCode)) return false;
final java.lang.Object this$card = this.getCard();
final java.lang.Object other$card = other.getCard();
if (this$card == null ? other$card != null : !this$card.equals(other$card)) return false;
final java.lang.Object this$disputed = this.getDisputed();
final java.lang.Object other$disputed = other.getDisputed();
if (this$disputed == null ? other$disputed != null : !this$disputed.equals(other$disputed)) return false;
final java.lang.Object this$statementDescription = this.getStatementDescription();
final java.lang.Object other$statementDescription = other.getStatementDescription();
if (this$statementDescription == null ? other$statementDescription != null : !this$statementDescription.equals(other$statementDescription)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Charge;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $amountRefunded = this.getAmountRefunded();
result = result * PRIME + ($amountRefunded == null ? 43 : $amountRefunded.hashCode());
final java.lang.Object $application = this.getApplication();
result = result * PRIME + ($application == null ? 43 : $application.hashCode());
final java.lang.Object $applicationFee = this.getApplicationFee();
result = result * PRIME + ($applicationFee == null ? 43 : $applicationFee.hashCode());
final java.lang.Object $applicationFeeAmount = this.getApplicationFeeAmount();
result = result * PRIME + ($applicationFeeAmount == null ? 43 : $applicationFeeAmount.hashCode());
final java.lang.Object $alternateStatementDescriptors = this.getAlternateStatementDescriptors();
result = result * PRIME + ($alternateStatementDescriptors == null ? 43 : $alternateStatementDescriptors.hashCode());
final java.lang.Object $balanceTransaction = this.getBalanceTransaction();
result = result * PRIME + ($balanceTransaction == null ? 43 : $balanceTransaction.hashCode());
final java.lang.Object $captured = this.getCaptured();
result = result * PRIME + ($captured == null ? 43 : $captured.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $destination = this.getDestination();
result = result * PRIME + ($destination == null ? 43 : $destination.hashCode());
final java.lang.Object $dispute = this.getDispute();
result = result * PRIME + ($dispute == null ? 43 : $dispute.hashCode());
final java.lang.Object $failureCode = this.getFailureCode();
result = result * PRIME + ($failureCode == null ? 43 : $failureCode.hashCode());
final java.lang.Object $failureMessage = this.getFailureMessage();
result = result * PRIME + ($failureMessage == null ? 43 : $failureMessage.hashCode());
final java.lang.Object $fraudDetails = this.getFraudDetails();
result = result * PRIME + ($fraudDetails == null ? 43 : $fraudDetails.hashCode());
final java.lang.Object $invoice = this.getInvoice();
result = result * PRIME + ($invoice == null ? 43 : $invoice.hashCode());
final java.lang.Object $level3 = this.getLevel3();
result = result * PRIME + ($level3 == null ? 43 : $level3.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $onBehalfOf = this.getOnBehalfOf();
result = result * PRIME + ($onBehalfOf == null ? 43 : $onBehalfOf.hashCode());
final java.lang.Object $order = this.getOrder();
result = result * PRIME + ($order == null ? 43 : $order.hashCode());
final java.lang.Object $outcome = this.getOutcome();
result = result * PRIME + ($outcome == null ? 43 : $outcome.hashCode());
final java.lang.Object $paid = this.getPaid();
result = result * PRIME + ($paid == null ? 43 : $paid.hashCode());
final java.lang.Object $receiptEmail = this.getReceiptEmail();
result = result * PRIME + ($receiptEmail == null ? 43 : $receiptEmail.hashCode());
final java.lang.Object $receiptNumber = this.getReceiptNumber();
result = result * PRIME + ($receiptNumber == null ? 43 : $receiptNumber.hashCode());
final java.lang.Object $receiptUrl = this.getReceiptUrl();
result = result * PRIME + ($receiptUrl == null ? 43 : $receiptUrl.hashCode());
final java.lang.Object $refunded = this.getRefunded();
result = result * PRIME + ($refunded == null ? 43 : $refunded.hashCode());
final java.lang.Object $refunds = this.getRefunds();
result = result * PRIME + ($refunds == null ? 43 : $refunds.hashCode());
final java.lang.Object $review = this.getReview();
result = result * PRIME + ($review == null ? 43 : $review.hashCode());
final java.lang.Object $shipping = this.getShipping();
result = result * PRIME + ($shipping == null ? 43 : $shipping.hashCode());
final java.lang.Object $source = this.getSource();
result = result * PRIME + ($source == null ? 43 : $source.hashCode());
final java.lang.Object $sourceTransfer = this.getSourceTransfer();
result = result * PRIME + ($sourceTransfer == null ? 43 : $sourceTransfer.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $transfer = this.getTransfer();
result = result * PRIME + ($transfer == null ? 43 : $transfer.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
final java.lang.Object $transferGroup = this.getTransferGroup();
result = result * PRIME + ($transferGroup == null ? 43 : $transferGroup.hashCode());
final java.lang.Object $authorizationCode = this.getAuthorizationCode();
result = result * PRIME + ($authorizationCode == null ? 43 : $authorizationCode.hashCode());
final java.lang.Object $card = this.getCard();
result = result * PRIME + ($card == null ? 43 : $card.hashCode());
final java.lang.Object $disputed = this.getDisputed();
result = result * PRIME + ($disputed == null ? 43 : $disputed.hashCode());
final java.lang.Object $statementDescription = this.getStatementDescription();
result = result * PRIME + ($statementDescription == null ? 43 : $statementDescription.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy