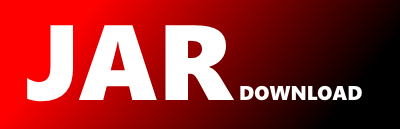
com.stripe.model.Invoice Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 16 18:44:44 CET 2019
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.math.BigDecimal;
import java.util.List;
import java.util.Map;
public class Invoice extends ApiResource implements MetadataStore, HasId {
String id;
String object;
Long amountDue;
Long amountPaid;
Long amountRemaining;
Long applicationFeeAmount;
Long attemptCount;
Boolean attempted;
Boolean autoAdvance;
String billing;
String billingReason;
ExpandableField charge;
Long created;
String currency;
List customFields;
ExpandableField customer;
Long date;
ExpandableField defaultSource;
Boolean deleted;
String description;
Discount discount;
Long dueDate;
Long endingBalance;
Long finalizedAt;
String footer;
String hostedInvoiceUrl;
String invoicePdf;
InvoiceLineItemCollection lines;
Boolean livemode;
Map metadata;
Long nextPaymentAttempt;
String number;
Boolean paid;
Long periodEnd;
Long periodStart;
String receiptNumber;
Long startingBalance;
String statementDescriptor;
String status;
ExpandableField subscription;
Long subscriptionProrationDate;
Long subtotal;
Long tax;
BigDecimal taxPercent;
ThresholdReason thresholdReason;
Long total;
TransferData transferData;
Long webhooksDeliveredAt;
/**
* The {@code applicationFee} attribute.
*
* @deprecated Prefer using the {@code applicationFeeAmount} attribute instead.
*/
@Deprecated
Long applicationFee;
/**
* The {@code closed} attribute.
*
* @deprecated Prefer using the {@code status} attribute instead.
*/
@Deprecated
Boolean closed;
/**
* The {@code forgiven} attribute.
*
* @deprecated Prefer using the {@code status} attribute instead.
*/
@Deprecated
Boolean forgiven;
//
public String getCharge() {
return (this.charge != null) ? this.charge.getId() : null;
}
public void setCharge(String chargeId) {
this.charge = setExpandableFieldId(chargeId, this.charge);
}
public Charge getChargeObject() {
return (this.charge != null) ? this.charge.getExpanded() : null;
}
public void setChargeObject(Charge charge) {
this.charge = new ExpandableField<>(charge.getId(), charge);
}
//
//
public String getCustomer() {
return (this.customer != null) ? this.customer.getId() : null;
}
public void setCustomer(String customerId) {
this.customer = setExpandableFieldId(customerId, this.customer);
}
public Customer getCustomerObject() {
return (this.customer != null) ? this.customer.getExpanded() : null;
}
public void setCustomerObject(Customer customer) {
this.customer = new ExpandableField<>(customer.getId(), customer);
}
//
//
public String getDefaultSource() {
return (this.defaultSource != null) ? this.defaultSource.getId() : null;
}
public void setDefaultSource(String defaultSourceId) {
this.defaultSource = setExpandableFieldId(defaultSourceId, this.defaultSource);
}
public ExternalAccount getDefaultSourceObject() {
return (this.defaultSource != null) ? this.defaultSource.getExpanded() : null;
}
public void setDefaultSourceObject(ExternalAccount c) {
this.defaultSource = new ExpandableField<>(c.getId(), c);
}
//
//
public String getSubscription() {
return (this.subscription != null) ? this.subscription.getId() : null;
}
public void setSubscription(String subscriptionId) {
this.subscription = setExpandableFieldId(subscriptionId, this.subscription);
}
public Subscription getSubscriptionObject() {
return (this.subscription != null) ? this.subscription.getExpanded() : null;
}
public void setSubscriptionObject(Subscription subscription) {
this.subscription = new ExpandableField<>(subscription.getId(), subscription);
}
//
//
/**
* Create an invoice.
*/
public static Invoice create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Create an invoice.
*/
public static Invoice create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Invoice.class), params, Invoice.class, options);
}
//
//
/**
* Delete an invoice.
*/
public Invoice delete() throws StripeException {
return delete((RequestOptions) null);
}
/**
* Delete an invoice.
*/
public Invoice delete(RequestOptions options) throws StripeException {
return request(RequestMethod.DELETE, instanceUrl(Invoice.class, this.id), null, Invoice.class, options);
}
//
//
/**
* List all invoices.
*/
public static InvoiceCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all invoices.
*/
public static InvoiceCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(Invoice.class), params, InvoiceCollection.class, options);
}
//
//
/**
* Finalize an invoice.
*/
public Invoice finalizeInvoice() throws StripeException {
return this.finalizeInvoice((RequestOptions) null);
}
/**
* Finalize an invoice.
*/
public Invoice finalizeInvoice(RequestOptions options) throws StripeException {
return finalizeInvoice(null, options);
}
/**
* Finalize an invoice.
*/
public Invoice finalizeInvoice(Map params) throws StripeException {
return this.finalizeInvoice(params, null);
}
/**
* Finalize an invoice.
*/
public Invoice finalizeInvoice(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/finalize", instanceUrl(Invoice.class, this.getId())), params, Invoice.class, options);
}
//
//
/**
* Mark an invoice as uncollectible.
*/
public Invoice markUncollectible() throws StripeException {
return this.markUncollectible((RequestOptions) null);
}
/**
* Mark an invoice as uncollectible.
*/
public Invoice markUncollectible(RequestOptions options) throws StripeException {
return markUncollectible(null, options);
}
/**
* Mark an invoice as uncollectible.
*/
public Invoice markUncollectible(Map params) throws StripeException {
return this.markUncollectible(params, null);
}
/**
* Mark an invoice as uncollectible.
*/
public Invoice markUncollectible(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/mark_uncollectible", instanceUrl(Invoice.class, this.getId())), params, Invoice.class, options);
}
//
//
/**
* Pay an invoice.
*/
public Invoice pay() throws StripeException {
return this.pay((RequestOptions) null);
}
/**
* Pay an invoice.
*/
public Invoice pay(RequestOptions options) throws StripeException {
return pay(null, options);
}
/**
* Pay an invoice.
*/
public Invoice pay(Map params) throws StripeException {
return this.pay(params, null);
}
/**
* Pay an invoice.
*/
public Invoice pay(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/pay", instanceUrl(Invoice.class, this.getId())), params, Invoice.class, options);
}
//
//
/**
* Retrieve an invoice.
*/
public static Invoice retrieve(String id) throws StripeException {
return retrieve(id, (RequestOptions) null);
}
/**
* Retrieve an invoice.
*/
public static Invoice retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve an invoice.
*/
public static Invoice retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Invoice.class, id), params, Invoice.class, options);
}
//
//
/**
* send an invoice.
*/
public Invoice sendInvoice() throws StripeException {
return this.sendInvoice((RequestOptions) null);
}
/**
* send an invoice.
*/
public Invoice sendInvoice(RequestOptions options) throws StripeException {
return sendInvoice(null, options);
}
/**
* send an invoice.
*/
public Invoice sendInvoice(Map params) throws StripeException {
return this.sendInvoice(params, null);
}
/**
* send an invoice.
*/
public Invoice sendInvoice(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/send", instanceUrl(Invoice.class, this.getId())), params, Invoice.class, options);
}
//
//
/**
* Retrieve an upcoming invoice.
*/
public static Invoice upcoming(Map params) throws StripeException {
return upcoming(params, (RequestOptions) null);
}
/**
* Retrieve an upcoming invoice.
*/
public static Invoice upcoming(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, String.format("%s/upcoming", classUrl(Invoice.class)), params, Invoice.class, options);
}
//
//
/**
* Update an invoice.
*/
@Override
public Invoice update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Update an invoice.
*/
@Override
public Invoice update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Invoice.class, this.id), params, Invoice.class, options);
}
//
//
/**
* void an invoice.
*/
public Invoice voidInvoice() throws StripeException {
return this.voidInvoice((RequestOptions) null);
}
/**
* void an invoice.
*/
public Invoice voidInvoice(RequestOptions options) throws StripeException {
return voidInvoice(null, options);
}
/**
* void an invoice.
*/
public Invoice voidInvoice(Map params) throws StripeException {
return this.voidInvoice(params, null);
}
/**
* void an invoice.
*/
public Invoice voidInvoice(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/void", instanceUrl(Invoice.class, this.getId())), params, Invoice.class, options);
}
//
public static class CustomField extends StripeObject {
String name;
String value;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValue(final String value) {
this.value = value;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.CustomField)) return false;
final Invoice.CustomField other = (Invoice.CustomField) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$value = this.getValue();
final java.lang.Object other$value = other.getValue();
if (this$value == null ? other$value != null : !this$value.equals(other$value)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.CustomField;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $value = this.getValue();
result = result * PRIME + ($value == null ? 43 : $value.hashCode());
return result;
}
}
public static class ThresholdReason extends StripeObject {
Long amountGte;
List itemReasons;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountGte() {
return this.amountGte;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getItemReasons() {
return this.itemReasons;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountGte(final Long amountGte) {
this.amountGte = amountGte;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setItemReasons(final List itemReasons) {
this.itemReasons = itemReasons;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.ThresholdReason)) return false;
final Invoice.ThresholdReason other = (Invoice.ThresholdReason) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$amountGte = this.getAmountGte();
final java.lang.Object other$amountGte = other.getAmountGte();
if (this$amountGte == null ? other$amountGte != null : !this$amountGte.equals(other$amountGte)) return false;
final java.lang.Object this$itemReasons = this.getItemReasons();
final java.lang.Object other$itemReasons = other.getItemReasons();
if (this$itemReasons == null ? other$itemReasons != null : !this$itemReasons.equals(other$itemReasons)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.ThresholdReason;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $amountGte = this.getAmountGte();
result = result * PRIME + ($amountGte == null ? 43 : $amountGte.hashCode());
final java.lang.Object $itemReasons = this.getItemReasons();
result = result * PRIME + ($itemReasons == null ? 43 : $itemReasons.hashCode());
return result;
}
}
public static class ThresholdItemReason extends StripeObject {
List lineItemIds;
Long usageGte;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getLineItemIds() {
return this.lineItemIds;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getUsageGte() {
return this.usageGte;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLineItemIds(final List lineItemIds) {
this.lineItemIds = lineItemIds;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsageGte(final Long usageGte) {
this.usageGte = usageGte;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice.ThresholdItemReason)) return false;
final Invoice.ThresholdItemReason other = (Invoice.ThresholdItemReason) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$lineItemIds = this.getLineItemIds();
final java.lang.Object other$lineItemIds = other.getLineItemIds();
if (this$lineItemIds == null ? other$lineItemIds != null : !this$lineItemIds.equals(other$lineItemIds)) return false;
final java.lang.Object this$usageGte = this.getUsageGte();
final java.lang.Object other$usageGte = other.getUsageGte();
if (this$usageGte == null ? other$usageGte != null : !this$usageGte.equals(other$usageGte)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice.ThresholdItemReason;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $lineItemIds = this.getLineItemIds();
result = result * PRIME + ($lineItemIds == null ? 43 : $lineItemIds.hashCode());
final java.lang.Object $usageGte = this.getUsageGte();
result = result * PRIME + ($usageGte == null ? 43 : $usageGte.hashCode());
return result;
}
}
public static class TransferData extends StripeObject {
ExpandableField destination;
//
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String destinationId) {
this.destination = setExpandableFieldId(destinationId, this.destination);
}
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account c) {
this.destination = new ExpandableField<>(c.getId(), c);
}
//
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountDue() {
return this.amountDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountPaid() {
return this.amountPaid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountRemaining() {
return this.amountRemaining;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getApplicationFeeAmount() {
return this.applicationFeeAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAttemptCount() {
return this.attemptCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAttempted() {
return this.attempted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getAutoAdvance() {
return this.autoAdvance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBilling() {
return this.billing;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getBillingReason() {
return this.billingReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getCustomFields() {
return this.customFields;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDate() {
return this.date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getDeleted() {
return this.deleted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Discount getDiscount() {
return this.discount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDueDate() {
return this.dueDate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getEndingBalance() {
return this.endingBalance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getFinalizedAt() {
return this.finalizedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFooter() {
return this.footer;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getHostedInvoiceUrl() {
return this.hostedInvoiceUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getInvoicePdf() {
return this.invoicePdf;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public InvoiceLineItemCollection getLines() {
return this.lines;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getNextPaymentAttempt() {
return this.nextPaymentAttempt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getNumber() {
return this.number;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getPaid() {
return this.paid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPeriodEnd() {
return this.periodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getPeriodStart() {
return this.periodStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReceiptNumber() {
return this.receiptNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getStartingBalance() {
return this.startingBalance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getSubscriptionProrationDate() {
return this.subscriptionProrationDate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getSubtotal() {
return this.subtotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTax() {
return this.tax;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BigDecimal getTaxPercent() {
return this.taxPercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ThresholdReason getThresholdReason() {
return this.thresholdReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getTotal() {
return this.total;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public TransferData getTransferData() {
return this.transferData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getWebhooksDeliveredAt() {
return this.webhooksDeliveredAt;
}
/**
* The {@code applicationFee} attribute.
*
* @deprecated Prefer using the {@code applicationFeeAmount} attribute instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getApplicationFee() {
return this.applicationFee;
}
/**
* The {@code closed} attribute.
*
* @deprecated Prefer using the {@code status} attribute instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getClosed() {
return this.closed;
}
/**
* The {@code forgiven} attribute.
*
* @deprecated Prefer using the {@code status} attribute instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getForgiven() {
return this.forgiven;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountDue(final Long amountDue) {
this.amountDue = amountDue;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountPaid(final Long amountPaid) {
this.amountPaid = amountPaid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountRemaining(final Long amountRemaining) {
this.amountRemaining = amountRemaining;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFeeAmount(final Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttemptCount(final Long attemptCount) {
this.attemptCount = attemptCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttempted(final Boolean attempted) {
this.attempted = attempted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAutoAdvance(final Boolean autoAdvance) {
this.autoAdvance = autoAdvance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBilling(final String billing) {
this.billing = billing;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBillingReason(final String billingReason) {
this.billingReason = billingReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCustomFields(final List customFields) {
this.customFields = customFields;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDate(final Long date) {
this.date = date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDeleted(final Boolean deleted) {
this.deleted = deleted;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDiscount(final Discount discount) {
this.discount = discount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDueDate(final Long dueDate) {
this.dueDate = dueDate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEndingBalance(final Long endingBalance) {
this.endingBalance = endingBalance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFinalizedAt(final Long finalizedAt) {
this.finalizedAt = finalizedAt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFooter(final String footer) {
this.footer = footer;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setHostedInvoiceUrl(final String hostedInvoiceUrl) {
this.hostedInvoiceUrl = hostedInvoiceUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoicePdf(final String invoicePdf) {
this.invoicePdf = invoicePdf;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLines(final InvoiceLineItemCollection lines) {
this.lines = lines;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNextPaymentAttempt(final Long nextPaymentAttempt) {
this.nextPaymentAttempt = nextPaymentAttempt;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNumber(final String number) {
this.number = number;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPaid(final Boolean paid) {
this.paid = paid;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPeriodEnd(final Long periodEnd) {
this.periodEnd = periodEnd;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPeriodStart(final Long periodStart) {
this.periodStart = periodStart;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiptNumber(final String receiptNumber) {
this.receiptNumber = receiptNumber;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStartingBalance(final Long startingBalance) {
this.startingBalance = startingBalance;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubscriptionProrationDate(final Long subscriptionProrationDate) {
this.subscriptionProrationDate = subscriptionProrationDate;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSubtotal(final Long subtotal) {
this.subtotal = subtotal;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTax(final Long tax) {
this.tax = tax;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTaxPercent(final BigDecimal taxPercent) {
this.taxPercent = taxPercent;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setThresholdReason(final ThresholdReason thresholdReason) {
this.thresholdReason = thresholdReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTotal(final Long total) {
this.total = total;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferData(final TransferData transferData) {
this.transferData = transferData;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setWebhooksDeliveredAt(final Long webhooksDeliveredAt) {
this.webhooksDeliveredAt = webhooksDeliveredAt;
}
/**
* The {@code applicationFee} attribute.
*
* @deprecated Prefer using the {@code applicationFeeAmount} attribute instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFee(final Long applicationFee) {
this.applicationFee = applicationFee;
}
/**
* The {@code closed} attribute.
*
* @deprecated Prefer using the {@code status} attribute instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClosed(final Boolean closed) {
this.closed = closed;
}
/**
* The {@code forgiven} attribute.
*
* @deprecated Prefer using the {@code status} attribute instead.
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setForgiven(final Boolean forgiven) {
this.forgiven = forgiven;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Invoice)) return false;
final Invoice other = (Invoice) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$amountDue = this.getAmountDue();
final java.lang.Object other$amountDue = other.getAmountDue();
if (this$amountDue == null ? other$amountDue != null : !this$amountDue.equals(other$amountDue)) return false;
final java.lang.Object this$amountPaid = this.getAmountPaid();
final java.lang.Object other$amountPaid = other.getAmountPaid();
if (this$amountPaid == null ? other$amountPaid != null : !this$amountPaid.equals(other$amountPaid)) return false;
final java.lang.Object this$amountRemaining = this.getAmountRemaining();
final java.lang.Object other$amountRemaining = other.getAmountRemaining();
if (this$amountRemaining == null ? other$amountRemaining != null : !this$amountRemaining.equals(other$amountRemaining)) return false;
final java.lang.Object this$applicationFeeAmount = this.getApplicationFeeAmount();
final java.lang.Object other$applicationFeeAmount = other.getApplicationFeeAmount();
if (this$applicationFeeAmount == null ? other$applicationFeeAmount != null : !this$applicationFeeAmount.equals(other$applicationFeeAmount)) return false;
final java.lang.Object this$attemptCount = this.getAttemptCount();
final java.lang.Object other$attemptCount = other.getAttemptCount();
if (this$attemptCount == null ? other$attemptCount != null : !this$attemptCount.equals(other$attemptCount)) return false;
final java.lang.Object this$attempted = this.getAttempted();
final java.lang.Object other$attempted = other.getAttempted();
if (this$attempted == null ? other$attempted != null : !this$attempted.equals(other$attempted)) return false;
final java.lang.Object this$autoAdvance = this.getAutoAdvance();
final java.lang.Object other$autoAdvance = other.getAutoAdvance();
if (this$autoAdvance == null ? other$autoAdvance != null : !this$autoAdvance.equals(other$autoAdvance)) return false;
final java.lang.Object this$billing = this.getBilling();
final java.lang.Object other$billing = other.getBilling();
if (this$billing == null ? other$billing != null : !this$billing.equals(other$billing)) return false;
final java.lang.Object this$billingReason = this.getBillingReason();
final java.lang.Object other$billingReason = other.getBillingReason();
if (this$billingReason == null ? other$billingReason != null : !this$billingReason.equals(other$billingReason)) return false;
final java.lang.Object this$charge = this.getCharge();
final java.lang.Object other$charge = other.getCharge();
if (this$charge == null ? other$charge != null : !this$charge.equals(other$charge)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$customFields = this.getCustomFields();
final java.lang.Object other$customFields = other.getCustomFields();
if (this$customFields == null ? other$customFields != null : !this$customFields.equals(other$customFields)) return false;
final java.lang.Object this$customer = this.getCustomer();
final java.lang.Object other$customer = other.getCustomer();
if (this$customer == null ? other$customer != null : !this$customer.equals(other$customer)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
final java.lang.Object this$defaultSource = this.getDefaultSource();
final java.lang.Object other$defaultSource = other.getDefaultSource();
if (this$defaultSource == null ? other$defaultSource != null : !this$defaultSource.equals(other$defaultSource)) return false;
final java.lang.Object this$deleted = this.getDeleted();
final java.lang.Object other$deleted = other.getDeleted();
if (this$deleted == null ? other$deleted != null : !this$deleted.equals(other$deleted)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$discount = this.getDiscount();
final java.lang.Object other$discount = other.getDiscount();
if (this$discount == null ? other$discount != null : !this$discount.equals(other$discount)) return false;
final java.lang.Object this$dueDate = this.getDueDate();
final java.lang.Object other$dueDate = other.getDueDate();
if (this$dueDate == null ? other$dueDate != null : !this$dueDate.equals(other$dueDate)) return false;
final java.lang.Object this$endingBalance = this.getEndingBalance();
final java.lang.Object other$endingBalance = other.getEndingBalance();
if (this$endingBalance == null ? other$endingBalance != null : !this$endingBalance.equals(other$endingBalance)) return false;
final java.lang.Object this$finalizedAt = this.getFinalizedAt();
final java.lang.Object other$finalizedAt = other.getFinalizedAt();
if (this$finalizedAt == null ? other$finalizedAt != null : !this$finalizedAt.equals(other$finalizedAt)) return false;
final java.lang.Object this$footer = this.getFooter();
final java.lang.Object other$footer = other.getFooter();
if (this$footer == null ? other$footer != null : !this$footer.equals(other$footer)) return false;
final java.lang.Object this$hostedInvoiceUrl = this.getHostedInvoiceUrl();
final java.lang.Object other$hostedInvoiceUrl = other.getHostedInvoiceUrl();
if (this$hostedInvoiceUrl == null ? other$hostedInvoiceUrl != null : !this$hostedInvoiceUrl.equals(other$hostedInvoiceUrl)) return false;
final java.lang.Object this$invoicePdf = this.getInvoicePdf();
final java.lang.Object other$invoicePdf = other.getInvoicePdf();
if (this$invoicePdf == null ? other$invoicePdf != null : !this$invoicePdf.equals(other$invoicePdf)) return false;
final java.lang.Object this$lines = this.getLines();
final java.lang.Object other$lines = other.getLines();
if (this$lines == null ? other$lines != null : !this$lines.equals(other$lines)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$nextPaymentAttempt = this.getNextPaymentAttempt();
final java.lang.Object other$nextPaymentAttempt = other.getNextPaymentAttempt();
if (this$nextPaymentAttempt == null ? other$nextPaymentAttempt != null : !this$nextPaymentAttempt.equals(other$nextPaymentAttempt)) return false;
final java.lang.Object this$number = this.getNumber();
final java.lang.Object other$number = other.getNumber();
if (this$number == null ? other$number != null : !this$number.equals(other$number)) return false;
final java.lang.Object this$paid = this.getPaid();
final java.lang.Object other$paid = other.getPaid();
if (this$paid == null ? other$paid != null : !this$paid.equals(other$paid)) return false;
final java.lang.Object this$periodEnd = this.getPeriodEnd();
final java.lang.Object other$periodEnd = other.getPeriodEnd();
if (this$periodEnd == null ? other$periodEnd != null : !this$periodEnd.equals(other$periodEnd)) return false;
final java.lang.Object this$periodStart = this.getPeriodStart();
final java.lang.Object other$periodStart = other.getPeriodStart();
if (this$periodStart == null ? other$periodStart != null : !this$periodStart.equals(other$periodStart)) return false;
final java.lang.Object this$receiptNumber = this.getReceiptNumber();
final java.lang.Object other$receiptNumber = other.getReceiptNumber();
if (this$receiptNumber == null ? other$receiptNumber != null : !this$receiptNumber.equals(other$receiptNumber)) return false;
final java.lang.Object this$startingBalance = this.getStartingBalance();
final java.lang.Object other$startingBalance = other.getStartingBalance();
if (this$startingBalance == null ? other$startingBalance != null : !this$startingBalance.equals(other$startingBalance)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$subscription = this.getSubscription();
final java.lang.Object other$subscription = other.getSubscription();
if (this$subscription == null ? other$subscription != null : !this$subscription.equals(other$subscription)) return false;
final java.lang.Object this$subscriptionProrationDate = this.getSubscriptionProrationDate();
final java.lang.Object other$subscriptionProrationDate = other.getSubscriptionProrationDate();
if (this$subscriptionProrationDate == null ? other$subscriptionProrationDate != null : !this$subscriptionProrationDate.equals(other$subscriptionProrationDate)) return false;
final java.lang.Object this$subtotal = this.getSubtotal();
final java.lang.Object other$subtotal = other.getSubtotal();
if (this$subtotal == null ? other$subtotal != null : !this$subtotal.equals(other$subtotal)) return false;
final java.lang.Object this$tax = this.getTax();
final java.lang.Object other$tax = other.getTax();
if (this$tax == null ? other$tax != null : !this$tax.equals(other$tax)) return false;
final java.lang.Object this$taxPercent = this.getTaxPercent();
final java.lang.Object other$taxPercent = other.getTaxPercent();
if (this$taxPercent == null ? other$taxPercent != null : !this$taxPercent.equals(other$taxPercent)) return false;
final java.lang.Object this$thresholdReason = this.getThresholdReason();
final java.lang.Object other$thresholdReason = other.getThresholdReason();
if (this$thresholdReason == null ? other$thresholdReason != null : !this$thresholdReason.equals(other$thresholdReason)) return false;
final java.lang.Object this$total = this.getTotal();
final java.lang.Object other$total = other.getTotal();
if (this$total == null ? other$total != null : !this$total.equals(other$total)) return false;
final java.lang.Object this$transferData = this.getTransferData();
final java.lang.Object other$transferData = other.getTransferData();
if (this$transferData == null ? other$transferData != null : !this$transferData.equals(other$transferData)) return false;
final java.lang.Object this$webhooksDeliveredAt = this.getWebhooksDeliveredAt();
final java.lang.Object other$webhooksDeliveredAt = other.getWebhooksDeliveredAt();
if (this$webhooksDeliveredAt == null ? other$webhooksDeliveredAt != null : !this$webhooksDeliveredAt.equals(other$webhooksDeliveredAt)) return false;
final java.lang.Object this$applicationFee = this.getApplicationFee();
final java.lang.Object other$applicationFee = other.getApplicationFee();
if (this$applicationFee == null ? other$applicationFee != null : !this$applicationFee.equals(other$applicationFee)) return false;
final java.lang.Object this$closed = this.getClosed();
final java.lang.Object other$closed = other.getClosed();
if (this$closed == null ? other$closed != null : !this$closed.equals(other$closed)) return false;
final java.lang.Object this$forgiven = this.getForgiven();
final java.lang.Object other$forgiven = other.getForgiven();
if (this$forgiven == null ? other$forgiven != null : !this$forgiven.equals(other$forgiven)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Invoice;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $amountDue = this.getAmountDue();
result = result * PRIME + ($amountDue == null ? 43 : $amountDue.hashCode());
final java.lang.Object $amountPaid = this.getAmountPaid();
result = result * PRIME + ($amountPaid == null ? 43 : $amountPaid.hashCode());
final java.lang.Object $amountRemaining = this.getAmountRemaining();
result = result * PRIME + ($amountRemaining == null ? 43 : $amountRemaining.hashCode());
final java.lang.Object $applicationFeeAmount = this.getApplicationFeeAmount();
result = result * PRIME + ($applicationFeeAmount == null ? 43 : $applicationFeeAmount.hashCode());
final java.lang.Object $attemptCount = this.getAttemptCount();
result = result * PRIME + ($attemptCount == null ? 43 : $attemptCount.hashCode());
final java.lang.Object $attempted = this.getAttempted();
result = result * PRIME + ($attempted == null ? 43 : $attempted.hashCode());
final java.lang.Object $autoAdvance = this.getAutoAdvance();
result = result * PRIME + ($autoAdvance == null ? 43 : $autoAdvance.hashCode());
final java.lang.Object $billing = this.getBilling();
result = result * PRIME + ($billing == null ? 43 : $billing.hashCode());
final java.lang.Object $billingReason = this.getBillingReason();
result = result * PRIME + ($billingReason == null ? 43 : $billingReason.hashCode());
final java.lang.Object $charge = this.getCharge();
result = result * PRIME + ($charge == null ? 43 : $charge.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $customFields = this.getCustomFields();
result = result * PRIME + ($customFields == null ? 43 : $customFields.hashCode());
final java.lang.Object $customer = this.getCustomer();
result = result * PRIME + ($customer == null ? 43 : $customer.hashCode());
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
final java.lang.Object $defaultSource = this.getDefaultSource();
result = result * PRIME + ($defaultSource == null ? 43 : $defaultSource.hashCode());
final java.lang.Object $deleted = this.getDeleted();
result = result * PRIME + ($deleted == null ? 43 : $deleted.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $discount = this.getDiscount();
result = result * PRIME + ($discount == null ? 43 : $discount.hashCode());
final java.lang.Object $dueDate = this.getDueDate();
result = result * PRIME + ($dueDate == null ? 43 : $dueDate.hashCode());
final java.lang.Object $endingBalance = this.getEndingBalance();
result = result * PRIME + ($endingBalance == null ? 43 : $endingBalance.hashCode());
final java.lang.Object $finalizedAt = this.getFinalizedAt();
result = result * PRIME + ($finalizedAt == null ? 43 : $finalizedAt.hashCode());
final java.lang.Object $footer = this.getFooter();
result = result * PRIME + ($footer == null ? 43 : $footer.hashCode());
final java.lang.Object $hostedInvoiceUrl = this.getHostedInvoiceUrl();
result = result * PRIME + ($hostedInvoiceUrl == null ? 43 : $hostedInvoiceUrl.hashCode());
final java.lang.Object $invoicePdf = this.getInvoicePdf();
result = result * PRIME + ($invoicePdf == null ? 43 : $invoicePdf.hashCode());
final java.lang.Object $lines = this.getLines();
result = result * PRIME + ($lines == null ? 43 : $lines.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $nextPaymentAttempt = this.getNextPaymentAttempt();
result = result * PRIME + ($nextPaymentAttempt == null ? 43 : $nextPaymentAttempt.hashCode());
final java.lang.Object $number = this.getNumber();
result = result * PRIME + ($number == null ? 43 : $number.hashCode());
final java.lang.Object $paid = this.getPaid();
result = result * PRIME + ($paid == null ? 43 : $paid.hashCode());
final java.lang.Object $periodEnd = this.getPeriodEnd();
result = result * PRIME + ($periodEnd == null ? 43 : $periodEnd.hashCode());
final java.lang.Object $periodStart = this.getPeriodStart();
result = result * PRIME + ($periodStart == null ? 43 : $periodStart.hashCode());
final java.lang.Object $receiptNumber = this.getReceiptNumber();
result = result * PRIME + ($receiptNumber == null ? 43 : $receiptNumber.hashCode());
final java.lang.Object $startingBalance = this.getStartingBalance();
result = result * PRIME + ($startingBalance == null ? 43 : $startingBalance.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $subscription = this.getSubscription();
result = result * PRIME + ($subscription == null ? 43 : $subscription.hashCode());
final java.lang.Object $subscriptionProrationDate = this.getSubscriptionProrationDate();
result = result * PRIME + ($subscriptionProrationDate == null ? 43 : $subscriptionProrationDate.hashCode());
final java.lang.Object $subtotal = this.getSubtotal();
result = result * PRIME + ($subtotal == null ? 43 : $subtotal.hashCode());
final java.lang.Object $tax = this.getTax();
result = result * PRIME + ($tax == null ? 43 : $tax.hashCode());
final java.lang.Object $taxPercent = this.getTaxPercent();
result = result * PRIME + ($taxPercent == null ? 43 : $taxPercent.hashCode());
final java.lang.Object $thresholdReason = this.getThresholdReason();
result = result * PRIME + ($thresholdReason == null ? 43 : $thresholdReason.hashCode());
final java.lang.Object $total = this.getTotal();
result = result * PRIME + ($total == null ? 43 : $total.hashCode());
final java.lang.Object $transferData = this.getTransferData();
result = result * PRIME + ($transferData == null ? 43 : $transferData.hashCode());
final java.lang.Object $webhooksDeliveredAt = this.getWebhooksDeliveredAt();
result = result * PRIME + ($webhooksDeliveredAt == null ? 43 : $webhooksDeliveredAt.hashCode());
final java.lang.Object $applicationFee = this.getApplicationFee();
result = result * PRIME + ($applicationFee == null ? 43 : $applicationFee.hashCode());
final java.lang.Object $closed = this.getClosed();
result = result * PRIME + ($closed == null ? 43 : $closed.hashCode());
final java.lang.Object $forgiven = this.getForgiven();
result = result * PRIME + ($forgiven == null ? 43 : $forgiven.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy