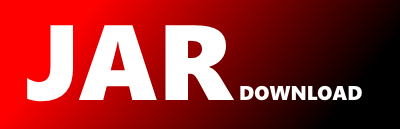
com.stripe.model.Source Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 16 18:44:45 CET 2019
package com.stripe.model;
import com.stripe.exception.InvalidRequestException;
import com.stripe.exception.StripeException;
import com.stripe.net.RequestOptions;
import java.util.Map;
public class Source extends ExternalAccount implements HasSourceTypeData {
Long amount;
String clientSecret;
CodeVerificationFlow codeVerification;
Long created;
String currency;
String flow;
Boolean livemode;
Owner owner;
ReceiverFlow receiver;
RedirectFlow redirect;
String statementDescriptor;
String status;
String type;
String usage;
// Type-specific properties
Map typeData;
// APIResource methods
public String getSourceInstanceUrl() throws InvalidRequestException {
return instanceUrl(Source.class, this.getId());
}
//
/**
* Create a source.
*/
public static Source create(Map params) throws StripeException {
return create(params, null);
}
/**
* Create a source.
*/
public static Source create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Source.class), params, Source.class, options);
}
//
//
/**
* Source objects cannot be deleted. Calling this method will raise an
* {@link InvalidRequestException}. Call {@link #detach} to detach the source from a
* customer object.
*/
@Override
public Source delete(RequestOptions options) throws StripeException {
throw new InvalidRequestException("Source objects cannot be deleted. If you want to detach the source from a customer object, use detach().", null, null, null, 0, null);
}
//
//
/**
* Detach a source.
*/
public Source detach() throws StripeException {
return detach(null, null);
}
/**
* Detach a source.
*/
public Source detach(Map params) throws StripeException {
return detach(params, null);
}
/**
* Detach a source.
*/
public Source detach(Map params, RequestOptions options) throws StripeException {
if (this.getCustomer() != null) {
String url = String.format("%s/%s/sources/%s", classUrl(Customer.class), this.getCustomer(), this.getId());
return request(RequestMethod.DELETE, url, params, Source.class, options);
} else {
throw new InvalidRequestException("This source object does not appear to be currently attached to a customer object.", null, null, null, 0, null);
}
}
//
//
/**
* Retrieve a source.
*/
public static Source retrieve(String id) throws StripeException {
return retrieve(id, null);
}
/**
* Retrieve a source.
*/
public static Source retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a source.
*/
public static Source retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Source.class, id), params, Source.class, options);
}
//
//
/**
* Retrieve a source's transactions.
*/
public SourceTransactionCollection sourceTransactions(Map params) throws StripeException {
return sourceTransactions(params, null);
}
/**
* Retrieve a source's transactions.
*/
public SourceTransactionCollection sourceTransactions(Map params, RequestOptions options) throws StripeException {
String url = instanceUrl(Source.class, this.getId()) + "/source_transactions";
return requestCollection(url, params, SourceTransactionCollection.class, options);
}
//
//
/**
* Update a source.
*/
@Override
public Source update(Map params) throws StripeException {
return update(params, null);
}
/**
* Update a source.
*/
@Override
public Source update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, this.getSourceInstanceUrl(), params, Source.class, options);
}
//
//
/**
* Verify a source.
*/
@Override
public Source verify(Map params) throws StripeException {
return verify(params, null);
}
/**
* Verify a source.
*/
@Override
public Source verify(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, String.format("%s/verify", this.getSourceInstanceUrl()), params, Source.class, options);
}
//
public static class CodeVerificationFlow extends StripeObject {
Long attemptsRemaining;
String status;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAttemptsRemaining() {
return this.attemptsRemaining;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAttemptsRemaining(final Long attemptsRemaining) {
this.attemptsRemaining = attemptsRemaining;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Source.CodeVerificationFlow)) return false;
final Source.CodeVerificationFlow other = (Source.CodeVerificationFlow) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$attemptsRemaining = this.getAttemptsRemaining();
final java.lang.Object other$attemptsRemaining = other.getAttemptsRemaining();
if (this$attemptsRemaining == null ? other$attemptsRemaining != null : !this$attemptsRemaining.equals(other$attemptsRemaining)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Source.CodeVerificationFlow;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $attemptsRemaining = this.getAttemptsRemaining();
result = result * PRIME + ($attemptsRemaining == null ? 43 : $attemptsRemaining.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
return result;
}
}
public static class Owner extends StripeObject {
Address address;
Address verifiedAddress;
String name;
String verifiedName;
String phone;
String verifiedPhone;
String email;
String verifiedEmail;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getAddress() {
return this.address;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Address getVerifiedAddress() {
return this.verifiedAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getName() {
return this.name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedName() {
return this.verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPhone() {
return this.phone;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedPhone() {
return this.verifiedPhone;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getEmail() {
return this.email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getVerifiedEmail() {
return this.verifiedEmail;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddress(final Address address) {
this.address = address;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedAddress(final Address verifiedAddress) {
this.verifiedAddress = verifiedAddress;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setName(final String name) {
this.name = name;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedName(final String verifiedName) {
this.verifiedName = verifiedName;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPhone(final String phone) {
this.phone = phone;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedPhone(final String verifiedPhone) {
this.verifiedPhone = verifiedPhone;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setEmail(final String email) {
this.email = email;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setVerifiedEmail(final String verifiedEmail) {
this.verifiedEmail = verifiedEmail;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Source.Owner)) return false;
final Source.Owner other = (Source.Owner) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
final java.lang.Object this$verifiedAddress = this.getVerifiedAddress();
final java.lang.Object other$verifiedAddress = other.getVerifiedAddress();
if (this$verifiedAddress == null ? other$verifiedAddress != null : !this$verifiedAddress.equals(other$verifiedAddress)) return false;
final java.lang.Object this$name = this.getName();
final java.lang.Object other$name = other.getName();
if (this$name == null ? other$name != null : !this$name.equals(other$name)) return false;
final java.lang.Object this$verifiedName = this.getVerifiedName();
final java.lang.Object other$verifiedName = other.getVerifiedName();
if (this$verifiedName == null ? other$verifiedName != null : !this$verifiedName.equals(other$verifiedName)) return false;
final java.lang.Object this$phone = this.getPhone();
final java.lang.Object other$phone = other.getPhone();
if (this$phone == null ? other$phone != null : !this$phone.equals(other$phone)) return false;
final java.lang.Object this$verifiedPhone = this.getVerifiedPhone();
final java.lang.Object other$verifiedPhone = other.getVerifiedPhone();
if (this$verifiedPhone == null ? other$verifiedPhone != null : !this$verifiedPhone.equals(other$verifiedPhone)) return false;
final java.lang.Object this$email = this.getEmail();
final java.lang.Object other$email = other.getEmail();
if (this$email == null ? other$email != null : !this$email.equals(other$email)) return false;
final java.lang.Object this$verifiedEmail = this.getVerifiedEmail();
final java.lang.Object other$verifiedEmail = other.getVerifiedEmail();
if (this$verifiedEmail == null ? other$verifiedEmail != null : !this$verifiedEmail.equals(other$verifiedEmail)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Source.Owner;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
final java.lang.Object $verifiedAddress = this.getVerifiedAddress();
result = result * PRIME + ($verifiedAddress == null ? 43 : $verifiedAddress.hashCode());
final java.lang.Object $name = this.getName();
result = result * PRIME + ($name == null ? 43 : $name.hashCode());
final java.lang.Object $verifiedName = this.getVerifiedName();
result = result * PRIME + ($verifiedName == null ? 43 : $verifiedName.hashCode());
final java.lang.Object $phone = this.getPhone();
result = result * PRIME + ($phone == null ? 43 : $phone.hashCode());
final java.lang.Object $verifiedPhone = this.getVerifiedPhone();
result = result * PRIME + ($verifiedPhone == null ? 43 : $verifiedPhone.hashCode());
final java.lang.Object $email = this.getEmail();
result = result * PRIME + ($email == null ? 43 : $email.hashCode());
final java.lang.Object $verifiedEmail = this.getVerifiedEmail();
result = result * PRIME + ($verifiedEmail == null ? 43 : $verifiedEmail.hashCode());
return result;
}
}
public static class ReceiverFlow extends StripeObject {
String refundAttributesStatus;
String refundAttributesMethod;
Long amountReceived;
Long amountReturned;
Long amountCharged;
String address;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRefundAttributesStatus() {
return this.refundAttributesStatus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRefundAttributesMethod() {
return this.refundAttributesMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountReceived() {
return this.amountReceived;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountReturned() {
return this.amountReturned;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountCharged() {
return this.amountCharged;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getAddress() {
return this.address;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefundAttributesStatus(final String refundAttributesStatus) {
this.refundAttributesStatus = refundAttributesStatus;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefundAttributesMethod(final String refundAttributesMethod) {
this.refundAttributesMethod = refundAttributesMethod;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountReceived(final Long amountReceived) {
this.amountReceived = amountReceived;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountReturned(final Long amountReturned) {
this.amountReturned = amountReturned;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountCharged(final Long amountCharged) {
this.amountCharged = amountCharged;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAddress(final String address) {
this.address = address;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Source.ReceiverFlow)) return false;
final Source.ReceiverFlow other = (Source.ReceiverFlow) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$refundAttributesStatus = this.getRefundAttributesStatus();
final java.lang.Object other$refundAttributesStatus = other.getRefundAttributesStatus();
if (this$refundAttributesStatus == null ? other$refundAttributesStatus != null : !this$refundAttributesStatus.equals(other$refundAttributesStatus)) return false;
final java.lang.Object this$refundAttributesMethod = this.getRefundAttributesMethod();
final java.lang.Object other$refundAttributesMethod = other.getRefundAttributesMethod();
if (this$refundAttributesMethod == null ? other$refundAttributesMethod != null : !this$refundAttributesMethod.equals(other$refundAttributesMethod)) return false;
final java.lang.Object this$amountReceived = this.getAmountReceived();
final java.lang.Object other$amountReceived = other.getAmountReceived();
if (this$amountReceived == null ? other$amountReceived != null : !this$amountReceived.equals(other$amountReceived)) return false;
final java.lang.Object this$amountReturned = this.getAmountReturned();
final java.lang.Object other$amountReturned = other.getAmountReturned();
if (this$amountReturned == null ? other$amountReturned != null : !this$amountReturned.equals(other$amountReturned)) return false;
final java.lang.Object this$amountCharged = this.getAmountCharged();
final java.lang.Object other$amountCharged = other.getAmountCharged();
if (this$amountCharged == null ? other$amountCharged != null : !this$amountCharged.equals(other$amountCharged)) return false;
final java.lang.Object this$address = this.getAddress();
final java.lang.Object other$address = other.getAddress();
if (this$address == null ? other$address != null : !this$address.equals(other$address)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Source.ReceiverFlow;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $refundAttributesStatus = this.getRefundAttributesStatus();
result = result * PRIME + ($refundAttributesStatus == null ? 43 : $refundAttributesStatus.hashCode());
final java.lang.Object $refundAttributesMethod = this.getRefundAttributesMethod();
result = result * PRIME + ($refundAttributesMethod == null ? 43 : $refundAttributesMethod.hashCode());
final java.lang.Object $amountReceived = this.getAmountReceived();
result = result * PRIME + ($amountReceived == null ? 43 : $amountReceived.hashCode());
final java.lang.Object $amountReturned = this.getAmountReturned();
result = result * PRIME + ($amountReturned == null ? 43 : $amountReturned.hashCode());
final java.lang.Object $amountCharged = this.getAmountCharged();
result = result * PRIME + ($amountCharged == null ? 43 : $amountCharged.hashCode());
final java.lang.Object $address = this.getAddress();
result = result * PRIME + ($address == null ? 43 : $address.hashCode());
return result;
}
}
public static class RedirectFlow extends StripeObject {
String failureReason;
String returnUrl;
String status;
String url;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFailureReason() {
return this.failureReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getReturnUrl() {
return this.returnUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUrl() {
return this.url;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFailureReason(final String failureReason) {
this.failureReason = failureReason;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReturnUrl(final String returnUrl) {
this.returnUrl = returnUrl;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUrl(final String url) {
this.url = url;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Source.RedirectFlow)) return false;
final Source.RedirectFlow other = (Source.RedirectFlow) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$failureReason = this.getFailureReason();
final java.lang.Object other$failureReason = other.getFailureReason();
if (this$failureReason == null ? other$failureReason != null : !this$failureReason.equals(other$failureReason)) return false;
final java.lang.Object this$returnUrl = this.getReturnUrl();
final java.lang.Object other$returnUrl = other.getReturnUrl();
if (this$returnUrl == null ? other$returnUrl != null : !this$returnUrl.equals(other$returnUrl)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$url = this.getUrl();
final java.lang.Object other$url = other.getUrl();
if (this$url == null ? other$url != null : !this$url.equals(other$url)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Source.RedirectFlow;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $failureReason = this.getFailureReason();
result = result * PRIME + ($failureReason == null ? 43 : $failureReason.hashCode());
final java.lang.Object $returnUrl = this.getReturnUrl();
result = result * PRIME + ($returnUrl == null ? 43 : $returnUrl.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $url = this.getUrl();
result = result * PRIME + ($url == null ? 43 : $url.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getClientSecret() {
return this.clientSecret;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public CodeVerificationFlow getCodeVerification() {
return this.codeVerification;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFlow() {
return this.flow;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Owner getOwner() {
return this.owner;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public ReceiverFlow getReceiver() {
return this.receiver;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public RedirectFlow getRedirect() {
return this.redirect;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getUsage() {
return this.usage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setClientSecret(final String clientSecret) {
this.clientSecret = clientSecret;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCodeVerification(final CodeVerificationFlow codeVerification) {
this.codeVerification = codeVerification;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFlow(final String flow) {
this.flow = flow;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOwner(final Owner owner) {
this.owner = owner;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReceiver(final ReceiverFlow receiver) {
this.receiver = receiver;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRedirect(final RedirectFlow redirect) {
this.redirect = redirect;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setUsage(final String usage) {
this.usage = usage;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Source)) return false;
final Source other = (Source) o;
if (!other.canEqual((java.lang.Object) this)) return false;
if (!super.equals(o)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$clientSecret = this.getClientSecret();
final java.lang.Object other$clientSecret = other.getClientSecret();
if (this$clientSecret == null ? other$clientSecret != null : !this$clientSecret.equals(other$clientSecret)) return false;
final java.lang.Object this$codeVerification = this.getCodeVerification();
final java.lang.Object other$codeVerification = other.getCodeVerification();
if (this$codeVerification == null ? other$codeVerification != null : !this$codeVerification.equals(other$codeVerification)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$flow = this.getFlow();
final java.lang.Object other$flow = other.getFlow();
if (this$flow == null ? other$flow != null : !this$flow.equals(other$flow)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$owner = this.getOwner();
final java.lang.Object other$owner = other.getOwner();
if (this$owner == null ? other$owner != null : !this$owner.equals(other$owner)) return false;
final java.lang.Object this$receiver = this.getReceiver();
final java.lang.Object other$receiver = other.getReceiver();
if (this$receiver == null ? other$receiver != null : !this$receiver.equals(other$receiver)) return false;
final java.lang.Object this$redirect = this.getRedirect();
final java.lang.Object other$redirect = other.getRedirect();
if (this$redirect == null ? other$redirect != null : !this$redirect.equals(other$redirect)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$usage = this.getUsage();
final java.lang.Object other$usage = other.getUsage();
if (this$usage == null ? other$usage != null : !this$usage.equals(other$usage)) return false;
final java.lang.Object this$typeData = this.getTypeData();
final java.lang.Object other$typeData = other.getTypeData();
if (this$typeData == null ? other$typeData != null : !this$typeData.equals(other$typeData)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Source;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = super.hashCode();
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $clientSecret = this.getClientSecret();
result = result * PRIME + ($clientSecret == null ? 43 : $clientSecret.hashCode());
final java.lang.Object $codeVerification = this.getCodeVerification();
result = result * PRIME + ($codeVerification == null ? 43 : $codeVerification.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $flow = this.getFlow();
result = result * PRIME + ($flow == null ? 43 : $flow.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $owner = this.getOwner();
result = result * PRIME + ($owner == null ? 43 : $owner.hashCode());
final java.lang.Object $receiver = this.getReceiver();
result = result * PRIME + ($receiver == null ? 43 : $receiver.hashCode());
final java.lang.Object $redirect = this.getRedirect();
result = result * PRIME + ($redirect == null ? 43 : $redirect.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $usage = this.getUsage();
result = result * PRIME + ($usage == null ? 43 : $usage.hashCode());
final java.lang.Object $typeData = this.getTypeData();
result = result * PRIME + ($typeData == null ? 43 : $typeData.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getTypeData() {
return this.typeData;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTypeData(final Map typeData) {
this.typeData = typeData;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy