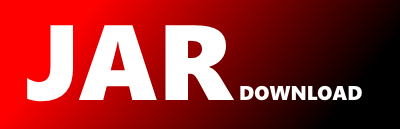
com.stripe.model.sigma.ScheduledQueryRun Maven / Gradle / Ivy
// Generated by delombok at Sat Feb 16 18:44:45 CET 2019
package com.stripe.model.sigma;
import com.stripe.exception.StripeException;
import com.stripe.model.File;
import com.stripe.model.HasId;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.Map;
public class ScheduledQueryRun extends ApiResource implements HasId {
String id;
String object;
Long created;
Long dataLoadTime;
String error;
File file;
Boolean livemode;
Long resultAvailableUntil;
String sql;
String status;
String title;
//
/**
* List all scheduled query runs.
*/
public static ScheduledQueryRunCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all scheduled query runs.
*/
public static ScheduledQueryRunCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(ScheduledQueryRun.class), params, ScheduledQueryRunCollection.class, options);
}
//
//
/**
* Retrieve a scheduled query run.
*/
public static ScheduledQueryRun retrieve(String id) throws StripeException {
return retrieve(id, null);
}
/**
* Retrieve a scheduled query run.
*/
public static ScheduledQueryRun retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a scheduled query run.
*/
public static ScheduledQueryRun retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(ScheduledQueryRun.class, id), params, ScheduledQueryRun.class, options);
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDataLoadTime() {
return this.dataLoadTime;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getError() {
return this.error;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public File getFile() {
return this.file;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getResultAvailableUntil() {
return this.resultAvailableUntil;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSql() {
return this.sql;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTitle() {
return this.title;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDataLoadTime(final Long dataLoadTime) {
this.dataLoadTime = dataLoadTime;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setError(final String error) {
this.error = error;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFile(final File file) {
this.file = file;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setResultAvailableUntil(final Long resultAvailableUntil) {
this.resultAvailableUntil = resultAvailableUntil;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSql(final String sql) {
this.sql = sql;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTitle(final String title) {
this.title = title;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof ScheduledQueryRun)) return false;
final ScheduledQueryRun other = (ScheduledQueryRun) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$dataLoadTime = this.getDataLoadTime();
final java.lang.Object other$dataLoadTime = other.getDataLoadTime();
if (this$dataLoadTime == null ? other$dataLoadTime != null : !this$dataLoadTime.equals(other$dataLoadTime)) return false;
final java.lang.Object this$error = this.getError();
final java.lang.Object other$error = other.getError();
if (this$error == null ? other$error != null : !this$error.equals(other$error)) return false;
final java.lang.Object this$file = this.getFile();
final java.lang.Object other$file = other.getFile();
if (this$file == null ? other$file != null : !this$file.equals(other$file)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$resultAvailableUntil = this.getResultAvailableUntil();
final java.lang.Object other$resultAvailableUntil = other.getResultAvailableUntil();
if (this$resultAvailableUntil == null ? other$resultAvailableUntil != null : !this$resultAvailableUntil.equals(other$resultAvailableUntil)) return false;
final java.lang.Object this$sql = this.getSql();
final java.lang.Object other$sql = other.getSql();
if (this$sql == null ? other$sql != null : !this$sql.equals(other$sql)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$title = this.getTitle();
final java.lang.Object other$title = other.getTitle();
if (this$title == null ? other$title != null : !this$title.equals(other$title)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof ScheduledQueryRun;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $dataLoadTime = this.getDataLoadTime();
result = result * PRIME + ($dataLoadTime == null ? 43 : $dataLoadTime.hashCode());
final java.lang.Object $error = this.getError();
result = result * PRIME + ($error == null ? 43 : $error.hashCode());
final java.lang.Object $file = this.getFile();
result = result * PRIME + ($file == null ? 43 : $file.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $resultAvailableUntil = this.getResultAvailableUntil();
result = result * PRIME + ($resultAvailableUntil == null ? 43 : $resultAvailableUntil.hashCode());
final java.lang.Object $sql = this.getSql();
result = result * PRIME + ($sql == null ? 43 : $sql.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $title = this.getTitle();
result = result * PRIME + ($title == null ? 43 : $title.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
//
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy