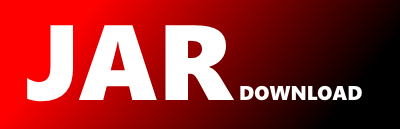
com.stripe.model.Transfer Maven / Gradle / Ivy
// Generated by delombok at Thu Nov 08 14:01:03 PST 2018
package com.stripe.model;
import com.stripe.exception.StripeException;
import com.stripe.net.ApiResource;
import com.stripe.net.RequestOptions;
import java.util.List;
import java.util.Map;
public class Transfer extends ApiResource implements MetadataStore, HasId {
String id;
String object;
Long amount;
Long amountReversed;
String applicationFee;
ExpandableField balanceTransaction;
BankAccount bankAccount;
Long created;
String currency;
Long date;
String description;
ExpandableField destination;
ExpandableField destinationPayment;
String failureCode;
String failureMessage;
Boolean livemode;
Map metadata;
TransferReversalCollection reversals;
Boolean reversed;
ExpandableField sourceTransaction;
String sourceType;
String statementDescriptor;
String status;
String transferGroup;
String type;
/**
* The {@code account} attribute.
*
* @deprecated Prefer using the {@link #bankAccount} attribute instead.
* @see API version 2014-05-19
*/
@Deprecated
BankAccount account;
/**
* The {@code other_transfers} attribute.
*
* @deprecated Prefer using the {@link BalanceTransaction#list} method instead.
* @see API version 2014-08-04
*/
@Deprecated
List otherTransfers;
@Deprecated
String recipient;
/**
* The {@code statement_description} attribute.
*
* @deprecated Prefer using the {@link #statementDescriptor} attribute instead.
* @see API version 2014-12-17
*/
@Deprecated
String statementDescription;
/**
* The {@code summary} attribute.
*
* @deprecated Prefer using the {@link BalanceTransaction#list} method instead.
* @see API version 2014-08-04
*/
@Deprecated
Summary summary;
//
public String getBalanceTransaction() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getId() : null;
}
public void setBalanceTransaction(String balanceTransactionId) {
this.balanceTransaction = setExpandableFieldId(balanceTransactionId, this.balanceTransaction);
}
public BalanceTransaction getBalanceTransactionObject() {
return (this.balanceTransaction != null) ? this.balanceTransaction.getExpanded() : null;
}
public void setBalanceTransactionObject(BalanceTransaction c) {
this.balanceTransaction = new ExpandableField(c.getId(), c);
}
//
//
public String getDestination() {
return (this.destination != null) ? this.destination.getId() : null;
}
public void setDestination(String destinationId) {
this.destination = ApiResource.setExpandableFieldId(destinationId, this.destination);
}
public Account getDestinationObject() {
return (this.destination != null) ? this.destination.getExpanded() : null;
}
public void setDestinationObject(Account c) {
this.destination = new ExpandableField(c.getId(), c);
}
//
//
public String getDestinationPayment() {
return (this.destinationPayment != null) ? this.destinationPayment.getId() : null;
}
public void setDestinationPayment(String destinationPaymentId) {
this.destinationPayment = setExpandableFieldId(destinationPaymentId, this.destinationPayment);
}
public Charge getDestinationPaymentObject() {
return (this.destinationPayment != null) ? this.destinationPayment.getExpanded() : null;
}
public void setDestinationPaymentObject(Charge destinationPayment) {
this.destinationPayment = new ExpandableField(destinationPayment.getId(), destinationPayment);
}
//
/**
* Returns the {@code reversals} list.
*
* @return the {@code reversals} list
*/
public TransferReversalCollection getReversals() {
if (reversals.getUrl() == null) {
reversals.setUrl(String.format("/v1/transfers/%s/reversals", getId()));
}
return reversals;
}
//
public String getSourceTransaction() {
return (this.sourceTransaction != null) ? this.sourceTransaction.getId() : null;
}
public void setSourceTransaction(String sourceTransactionId) {
this.sourceTransaction = setExpandableFieldId(sourceTransactionId, this.sourceTransaction);
}
public Charge getSourceTransactionObject() {
return (this.sourceTransaction != null) ? this.sourceTransaction.getExpanded() : null;
}
public void setSourceTransactionObject(Charge sourceTransaction) {
this.sourceTransaction = new ExpandableField(sourceTransaction.getId(), sourceTransaction);
}
//
//
/**
* Cancel a transfer.
*
* @deprecated Use the {#link Payout#cancel()} method instead.
*/
@Deprecated
public Transfer cancel() throws StripeException {
return cancel((RequestOptions) null);
}
/**
* Cancel a transfer.
*
* @deprecated Use the {#link Payout#cancel(RequestOptions)} method instead.
*/
@Deprecated
public Transfer cancel(RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Transfer.class, this.id) + "/cancel", null, Transfer.class, options);
}
//
//
/**
* Create a transfer.
*/
public static Transfer create(Map params) throws StripeException {
return create(params, (RequestOptions) null);
}
/**
* Create a transfer.
*/
public static Transfer create(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, classUrl(Transfer.class), params, Transfer.class, options);
}
//
//
/**
* List all transfers.
*/
public static TransferCollection list(Map params) throws StripeException {
return list(params, null);
}
/**
* List all transfers.
*/
public static TransferCollection list(Map params, RequestOptions options) throws StripeException {
return requestCollection(classUrl(Transfer.class), params, TransferCollection.class, options);
}
//
//
/**
* Retrive a transfer.
*/
public static Transfer retrieve(String id) throws StripeException {
return retrieve(id, (RequestOptions) null);
}
/**
* Retrive a transfer.
*/
public static Transfer retrieve(String id, RequestOptions options) throws StripeException {
return retrieve(id, null, options);
}
/**
* Retrieve a transfer.
*/
public static Transfer retrieve(String id, Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.GET, instanceUrl(Transfer.class, id), params, Transfer.class, options);
}
//
//
/**
* Retrieve a transfer's transactions.
*
* @deprecated Prefer using the {@link BalanceTransaction#list(Map)} method with
* the {@code payout} parameter.
*/
@Deprecated
public TransferTransactionCollection transactions(Map params) throws StripeException {
return transactions(params, (RequestOptions) null);
}
/**
* Retrieve a transfer's transactions.
*
* @deprecated Prefer using the {@link BalanceTransaction#list(Map, RequestOptions)} method with
* the {@code payout} parameter.
*/
@Deprecated
public TransferTransactionCollection transactions(Map params, RequestOptions options) throws StripeException {
String url = String.format("%s%s", instanceUrl(Transfer.class, this.getId()), "/transactions");
return requestCollection(url, params, TransferTransactionCollection.class, options);
}
//
//
/**
* Update a transfer.
*/
@Override
public Transfer update(Map params) throws StripeException {
return update(params, (RequestOptions) null);
}
/**
* Update a transfer.
*/
@Override
public Transfer update(Map params, RequestOptions options) throws StripeException {
return request(RequestMethod.POST, instanceUrl(Transfer.class, this.id), params, Transfer.class, options);
}
//
public static class Summary extends StripeObject {
Long adjustmentCount;
Long adjustmentGross;
Long chargeCount;
Long chargeFees;
Long chargeGross;
Long net;
Long refundCount;
Long refundFees;
Long refundGross;
Long validationCount;
Long validationFees;
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAdjustmentCount() {
return this.adjustmentCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAdjustmentGross() {
return this.adjustmentGross;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getChargeCount() {
return this.chargeCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getChargeFees() {
return this.chargeFees;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getChargeGross() {
return this.chargeGross;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getNet() {
return this.net;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getRefundCount() {
return this.refundCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getRefundFees() {
return this.refundFees;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getRefundGross() {
return this.refundGross;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getValidationCount() {
return this.validationCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getValidationFees() {
return this.validationFees;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAdjustmentCount(final Long adjustmentCount) {
this.adjustmentCount = adjustmentCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAdjustmentGross(final Long adjustmentGross) {
this.adjustmentGross = adjustmentGross;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setChargeCount(final Long chargeCount) {
this.chargeCount = chargeCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setChargeFees(final Long chargeFees) {
this.chargeFees = chargeFees;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setChargeGross(final Long chargeGross) {
this.chargeGross = chargeGross;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setNet(final Long net) {
this.net = net;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefundCount(final Long refundCount) {
this.refundCount = refundCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefundFees(final Long refundFees) {
this.refundFees = refundFees;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRefundGross(final Long refundGross) {
this.refundGross = refundGross;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValidationCount(final Long validationCount) {
this.validationCount = validationCount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setValidationFees(final Long validationFees) {
this.validationFees = validationFees;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transfer.Summary)) return false;
final Transfer.Summary other = (Transfer.Summary) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$adjustmentCount = this.getAdjustmentCount();
final java.lang.Object other$adjustmentCount = other.getAdjustmentCount();
if (this$adjustmentCount == null ? other$adjustmentCount != null : !this$adjustmentCount.equals(other$adjustmentCount)) return false;
final java.lang.Object this$adjustmentGross = this.getAdjustmentGross();
final java.lang.Object other$adjustmentGross = other.getAdjustmentGross();
if (this$adjustmentGross == null ? other$adjustmentGross != null : !this$adjustmentGross.equals(other$adjustmentGross)) return false;
final java.lang.Object this$chargeCount = this.getChargeCount();
final java.lang.Object other$chargeCount = other.getChargeCount();
if (this$chargeCount == null ? other$chargeCount != null : !this$chargeCount.equals(other$chargeCount)) return false;
final java.lang.Object this$chargeFees = this.getChargeFees();
final java.lang.Object other$chargeFees = other.getChargeFees();
if (this$chargeFees == null ? other$chargeFees != null : !this$chargeFees.equals(other$chargeFees)) return false;
final java.lang.Object this$chargeGross = this.getChargeGross();
final java.lang.Object other$chargeGross = other.getChargeGross();
if (this$chargeGross == null ? other$chargeGross != null : !this$chargeGross.equals(other$chargeGross)) return false;
final java.lang.Object this$net = this.getNet();
final java.lang.Object other$net = other.getNet();
if (this$net == null ? other$net != null : !this$net.equals(other$net)) return false;
final java.lang.Object this$refundCount = this.getRefundCount();
final java.lang.Object other$refundCount = other.getRefundCount();
if (this$refundCount == null ? other$refundCount != null : !this$refundCount.equals(other$refundCount)) return false;
final java.lang.Object this$refundFees = this.getRefundFees();
final java.lang.Object other$refundFees = other.getRefundFees();
if (this$refundFees == null ? other$refundFees != null : !this$refundFees.equals(other$refundFees)) return false;
final java.lang.Object this$refundGross = this.getRefundGross();
final java.lang.Object other$refundGross = other.getRefundGross();
if (this$refundGross == null ? other$refundGross != null : !this$refundGross.equals(other$refundGross)) return false;
final java.lang.Object this$validationCount = this.getValidationCount();
final java.lang.Object other$validationCount = other.getValidationCount();
if (this$validationCount == null ? other$validationCount != null : !this$validationCount.equals(other$validationCount)) return false;
final java.lang.Object this$validationFees = this.getValidationFees();
final java.lang.Object other$validationFees = other.getValidationFees();
if (this$validationFees == null ? other$validationFees != null : !this$validationFees.equals(other$validationFees)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transfer.Summary;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $adjustmentCount = this.getAdjustmentCount();
result = result * PRIME + ($adjustmentCount == null ? 43 : $adjustmentCount.hashCode());
final java.lang.Object $adjustmentGross = this.getAdjustmentGross();
result = result * PRIME + ($adjustmentGross == null ? 43 : $adjustmentGross.hashCode());
final java.lang.Object $chargeCount = this.getChargeCount();
result = result * PRIME + ($chargeCount == null ? 43 : $chargeCount.hashCode());
final java.lang.Object $chargeFees = this.getChargeFees();
result = result * PRIME + ($chargeFees == null ? 43 : $chargeFees.hashCode());
final java.lang.Object $chargeGross = this.getChargeGross();
result = result * PRIME + ($chargeGross == null ? 43 : $chargeGross.hashCode());
final java.lang.Object $net = this.getNet();
result = result * PRIME + ($net == null ? 43 : $net.hashCode());
final java.lang.Object $refundCount = this.getRefundCount();
result = result * PRIME + ($refundCount == null ? 43 : $refundCount.hashCode());
final java.lang.Object $refundFees = this.getRefundFees();
result = result * PRIME + ($refundFees == null ? 43 : $refundFees.hashCode());
final java.lang.Object $refundGross = this.getRefundGross();
result = result * PRIME + ($refundGross == null ? 43 : $refundGross.hashCode());
final java.lang.Object $validationCount = this.getValidationCount();
result = result * PRIME + ($validationCount == null ? 43 : $validationCount.hashCode());
final java.lang.Object $validationFees = this.getValidationFees();
result = result * PRIME + ($validationFees == null ? 43 : $validationFees.hashCode());
return result;
}
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmount() {
return this.amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getAmountReversed() {
return this.amountReversed;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getApplicationFee() {
return this.applicationFee;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BankAccount getBankAccount() {
return this.bankAccount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getCurrency() {
return this.currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getDate() {
return this.date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getDescription() {
return this.description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFailureCode() {
return this.failureCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getFailureMessage() {
return this.failureMessage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getReversed() {
return this.reversed;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSourceType() {
return this.sourceType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescriptor() {
return this.statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatus() {
return this.status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getTransferGroup() {
return this.transferGroup;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getType() {
return this.type;
}
/**
* The {@code account} attribute.
*
* @return the {@code account} attribute
* @deprecated Prefer using the {@link #bankAccount} attribute instead.
* @see API version 2014-05-19
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public BankAccount getAccount() {
return this.account;
}
/**
* The {@code other_transfers} attribute.
*
* @return the {@code other_transfers} attribute
* @deprecated Prefer using the {@link BalanceTransaction#list} method instead.
* @see API version 2014-08-04
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getOtherTransfers() {
return this.otherTransfers;
}
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRecipient() {
return this.recipient;
}
/**
* The {@code statement_description} attribute.
*
* @return the {@code statement_description} attribute
* @deprecated Prefer using the {@link #statementDescriptor} attribute instead.
* @see API version 2014-12-17
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getStatementDescription() {
return this.statementDescription;
}
/**
* The {@code summary} attribute.
*
* @return the {@code summary} attribute
* @deprecated Prefer using the {@link BalanceTransaction#list} method instead.
* @see API version 2014-08-04
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Summary getSummary() {
return this.summary;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmount(final Long amount) {
this.amount = amount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAmountReversed(final Long amountReversed) {
this.amountReversed = amountReversed;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setApplicationFee(final String applicationFee) {
this.applicationFee = applicationFee;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setBankAccount(final BankAccount bankAccount) {
this.bankAccount = bankAccount;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCurrency(final String currency) {
this.currency = currency;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDate(final Long date) {
this.date = date;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setDescription(final String description) {
this.description = description;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFailureCode(final String failureCode) {
this.failureCode = failureCode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setFailureMessage(final String failureMessage) {
this.failureMessage = failureMessage;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReversals(final TransferReversalCollection reversals) {
this.reversals = reversals;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setReversed(final Boolean reversed) {
this.reversed = reversed;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSourceType(final String sourceType) {
this.sourceType = sourceType;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescriptor(final String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatus(final String status) {
this.status = status;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setTransferGroup(final String transferGroup) {
this.transferGroup = transferGroup;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setType(final String type) {
this.type = type;
}
/**
* The {@code account} attribute.
*
* @deprecated Prefer using the {@link #bankAccount} attribute instead.
* @see API version 2014-05-19
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setAccount(final BankAccount account) {
this.account = account;
}
/**
* The {@code other_transfers} attribute.
*
* @deprecated Prefer using the {@link BalanceTransaction#list} method instead.
* @see API version 2014-08-04
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setOtherTransfers(final List otherTransfers) {
this.otherTransfers = otherTransfers;
}
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRecipient(final String recipient) {
this.recipient = recipient;
}
/**
* The {@code statement_description} attribute.
*
* @deprecated Prefer using the {@link #statementDescriptor} attribute instead.
* @see API version 2014-12-17
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setStatementDescription(final String statementDescription) {
this.statementDescription = statementDescription;
}
/**
* The {@code summary} attribute.
*
* @deprecated Prefer using the {@link BalanceTransaction#list} method instead.
* @see API version 2014-08-04
*/
@java.lang.Deprecated
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSummary(final Summary summary) {
this.summary = summary;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof Transfer)) return false;
final Transfer other = (Transfer) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$amount = this.getAmount();
final java.lang.Object other$amount = other.getAmount();
if (this$amount == null ? other$amount != null : !this$amount.equals(other$amount)) return false;
final java.lang.Object this$amountReversed = this.getAmountReversed();
final java.lang.Object other$amountReversed = other.getAmountReversed();
if (this$amountReversed == null ? other$amountReversed != null : !this$amountReversed.equals(other$amountReversed)) return false;
final java.lang.Object this$applicationFee = this.getApplicationFee();
final java.lang.Object other$applicationFee = other.getApplicationFee();
if (this$applicationFee == null ? other$applicationFee != null : !this$applicationFee.equals(other$applicationFee)) return false;
final java.lang.Object this$balanceTransaction = this.getBalanceTransaction();
final java.lang.Object other$balanceTransaction = other.getBalanceTransaction();
if (this$balanceTransaction == null ? other$balanceTransaction != null : !this$balanceTransaction.equals(other$balanceTransaction)) return false;
final java.lang.Object this$bankAccount = this.getBankAccount();
final java.lang.Object other$bankAccount = other.getBankAccount();
if (this$bankAccount == null ? other$bankAccount != null : !this$bankAccount.equals(other$bankAccount)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$currency = this.getCurrency();
final java.lang.Object other$currency = other.getCurrency();
if (this$currency == null ? other$currency != null : !this$currency.equals(other$currency)) return false;
final java.lang.Object this$date = this.getDate();
final java.lang.Object other$date = other.getDate();
if (this$date == null ? other$date != null : !this$date.equals(other$date)) return false;
final java.lang.Object this$description = this.getDescription();
final java.lang.Object other$description = other.getDescription();
if (this$description == null ? other$description != null : !this$description.equals(other$description)) return false;
final java.lang.Object this$destination = this.getDestination();
final java.lang.Object other$destination = other.getDestination();
if (this$destination == null ? other$destination != null : !this$destination.equals(other$destination)) return false;
final java.lang.Object this$destinationPayment = this.getDestinationPayment();
final java.lang.Object other$destinationPayment = other.getDestinationPayment();
if (this$destinationPayment == null ? other$destinationPayment != null : !this$destinationPayment.equals(other$destinationPayment)) return false;
final java.lang.Object this$failureCode = this.getFailureCode();
final java.lang.Object other$failureCode = other.getFailureCode();
if (this$failureCode == null ? other$failureCode != null : !this$failureCode.equals(other$failureCode)) return false;
final java.lang.Object this$failureMessage = this.getFailureMessage();
final java.lang.Object other$failureMessage = other.getFailureMessage();
if (this$failureMessage == null ? other$failureMessage != null : !this$failureMessage.equals(other$failureMessage)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$reversals = this.getReversals();
final java.lang.Object other$reversals = other.getReversals();
if (this$reversals == null ? other$reversals != null : !this$reversals.equals(other$reversals)) return false;
final java.lang.Object this$reversed = this.getReversed();
final java.lang.Object other$reversed = other.getReversed();
if (this$reversed == null ? other$reversed != null : !this$reversed.equals(other$reversed)) return false;
final java.lang.Object this$sourceTransaction = this.getSourceTransaction();
final java.lang.Object other$sourceTransaction = other.getSourceTransaction();
if (this$sourceTransaction == null ? other$sourceTransaction != null : !this$sourceTransaction.equals(other$sourceTransaction)) return false;
final java.lang.Object this$sourceType = this.getSourceType();
final java.lang.Object other$sourceType = other.getSourceType();
if (this$sourceType == null ? other$sourceType != null : !this$sourceType.equals(other$sourceType)) return false;
final java.lang.Object this$statementDescriptor = this.getStatementDescriptor();
final java.lang.Object other$statementDescriptor = other.getStatementDescriptor();
if (this$statementDescriptor == null ? other$statementDescriptor != null : !this$statementDescriptor.equals(other$statementDescriptor)) return false;
final java.lang.Object this$status = this.getStatus();
final java.lang.Object other$status = other.getStatus();
if (this$status == null ? other$status != null : !this$status.equals(other$status)) return false;
final java.lang.Object this$transferGroup = this.getTransferGroup();
final java.lang.Object other$transferGroup = other.getTransferGroup();
if (this$transferGroup == null ? other$transferGroup != null : !this$transferGroup.equals(other$transferGroup)) return false;
final java.lang.Object this$type = this.getType();
final java.lang.Object other$type = other.getType();
if (this$type == null ? other$type != null : !this$type.equals(other$type)) return false;
final java.lang.Object this$account = this.getAccount();
final java.lang.Object other$account = other.getAccount();
if (this$account == null ? other$account != null : !this$account.equals(other$account)) return false;
final java.lang.Object this$otherTransfers = this.getOtherTransfers();
final java.lang.Object other$otherTransfers = other.getOtherTransfers();
if (this$otherTransfers == null ? other$otherTransfers != null : !this$otherTransfers.equals(other$otherTransfers)) return false;
final java.lang.Object this$recipient = this.getRecipient();
final java.lang.Object other$recipient = other.getRecipient();
if (this$recipient == null ? other$recipient != null : !this$recipient.equals(other$recipient)) return false;
final java.lang.Object this$statementDescription = this.getStatementDescription();
final java.lang.Object other$statementDescription = other.getStatementDescription();
if (this$statementDescription == null ? other$statementDescription != null : !this$statementDescription.equals(other$statementDescription)) return false;
final java.lang.Object this$summary = this.getSummary();
final java.lang.Object other$summary = other.getSummary();
if (this$summary == null ? other$summary != null : !this$summary.equals(other$summary)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof Transfer;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $amount = this.getAmount();
result = result * PRIME + ($amount == null ? 43 : $amount.hashCode());
final java.lang.Object $amountReversed = this.getAmountReversed();
result = result * PRIME + ($amountReversed == null ? 43 : $amountReversed.hashCode());
final java.lang.Object $applicationFee = this.getApplicationFee();
result = result * PRIME + ($applicationFee == null ? 43 : $applicationFee.hashCode());
final java.lang.Object $balanceTransaction = this.getBalanceTransaction();
result = result * PRIME + ($balanceTransaction == null ? 43 : $balanceTransaction.hashCode());
final java.lang.Object $bankAccount = this.getBankAccount();
result = result * PRIME + ($bankAccount == null ? 43 : $bankAccount.hashCode());
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $currency = this.getCurrency();
result = result * PRIME + ($currency == null ? 43 : $currency.hashCode());
final java.lang.Object $date = this.getDate();
result = result * PRIME + ($date == null ? 43 : $date.hashCode());
final java.lang.Object $description = this.getDescription();
result = result * PRIME + ($description == null ? 43 : $description.hashCode());
final java.lang.Object $destination = this.getDestination();
result = result * PRIME + ($destination == null ? 43 : $destination.hashCode());
final java.lang.Object $destinationPayment = this.getDestinationPayment();
result = result * PRIME + ($destinationPayment == null ? 43 : $destinationPayment.hashCode());
final java.lang.Object $failureCode = this.getFailureCode();
result = result * PRIME + ($failureCode == null ? 43 : $failureCode.hashCode());
final java.lang.Object $failureMessage = this.getFailureMessage();
result = result * PRIME + ($failureMessage == null ? 43 : $failureMessage.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $reversals = this.getReversals();
result = result * PRIME + ($reversals == null ? 43 : $reversals.hashCode());
final java.lang.Object $reversed = this.getReversed();
result = result * PRIME + ($reversed == null ? 43 : $reversed.hashCode());
final java.lang.Object $sourceTransaction = this.getSourceTransaction();
result = result * PRIME + ($sourceTransaction == null ? 43 : $sourceTransaction.hashCode());
final java.lang.Object $sourceType = this.getSourceType();
result = result * PRIME + ($sourceType == null ? 43 : $sourceType.hashCode());
final java.lang.Object $statementDescriptor = this.getStatementDescriptor();
result = result * PRIME + ($statementDescriptor == null ? 43 : $statementDescriptor.hashCode());
final java.lang.Object $status = this.getStatus();
result = result * PRIME + ($status == null ? 43 : $status.hashCode());
final java.lang.Object $transferGroup = this.getTransferGroup();
result = result * PRIME + ($transferGroup == null ? 43 : $transferGroup.hashCode());
final java.lang.Object $type = this.getType();
result = result * PRIME + ($type == null ? 43 : $type.hashCode());
final java.lang.Object $account = this.getAccount();
result = result * PRIME + ($account == null ? 43 : $account.hashCode());
final java.lang.Object $otherTransfers = this.getOtherTransfers();
result = result * PRIME + ($otherTransfers == null ? 43 : $otherTransfers.hashCode());
final java.lang.Object $recipient = this.getRecipient();
result = result * PRIME + ($recipient == null ? 43 : $recipient.hashCode());
final java.lang.Object $statementDescription = this.getStatementDescription();
result = result * PRIME + ($statementDescription == null ? 43 : $statementDescription.hashCode());
final java.lang.Object $summary = this.getSummary();
result = result * PRIME + ($summary == null ? 43 : $summary.hashCode());
return result;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy