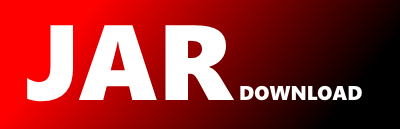
com.stripe.param.CustomerUpdateParams Maven / Gradle / Ivy
// Generated by delombok at Tue Apr 09 17:23:04 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class CustomerUpdateParams extends ApiRequestParams {
/**
* An integer amount in %s that represents the account balance for your customer. Account balances
* only affect invoices. A negative amount represents a credit that decreases the amount due on an
* invoice; a positive amount increases the amount due on an invoice.
*/
@SerializedName("account_balance")
Long accountBalance;
@SerializedName("coupon")
String coupon;
/**
* ID of the default payment source for the customer.
*/
@SerializedName("default_source")
String defaultSource;
/**
* An arbitrary string that you can attach to a customer object. It is displayed alongside the
* customer in the dashboard.
*/
@SerializedName("description")
String description;
/**
* Customer's email address. It's displayed alongside the customer in your dashboard and can be
* useful for searching and tracking. This may be up to *512 characters*.
*/
@SerializedName("email")
String email;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* The prefix for the customer used to generate unique invoice numbers. Must be 3–12 uppercase
* letters or numbers.
*/
@SerializedName("invoice_prefix")
String invoicePrefix;
/**
* Default invoice settings for this customer.
*/
@SerializedName("invoice_settings")
InvoiceSettings invoiceSettings;
/**
* A set of key-value pairs that you can attach to a customer object. It can be useful for storing
* additional information about the customer in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
@SerializedName("shipping")
Object shipping;
@SerializedName("source")
String source;
/**
* The customer's tax information. Appears on invoices emailed to this customer.
*/
@SerializedName("tax_info")
TaxInfo taxInfo;
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the customer's
* trial immediately.
*/
@SerializedName("trial_end")
Object trialEnd;
private CustomerUpdateParams(Long accountBalance, String coupon, String defaultSource, String description, String email, List expand, String invoicePrefix, InvoiceSettings invoiceSettings, Map metadata, Object shipping, String source, TaxInfo taxInfo, Object trialEnd) {
this.accountBalance = accountBalance;
this.coupon = coupon;
this.defaultSource = defaultSource;
this.description = description;
this.email = email;
this.expand = expand;
this.invoicePrefix = invoicePrefix;
this.invoiceSettings = invoiceSettings;
this.metadata = metadata;
this.shipping = shipping;
this.source = source;
this.taxInfo = taxInfo;
this.trialEnd = trialEnd;
}
public static Builder builder() {
return new com.stripe.param.CustomerUpdateParams.Builder();
}
public static class Builder {
private Long accountBalance;
private String coupon;
private String defaultSource;
private String description;
private String email;
private List expand;
private String invoicePrefix;
private InvoiceSettings invoiceSettings;
private Map metadata;
private Object shipping;
private String source;
private TaxInfo taxInfo;
private Object trialEnd;
/**
* Finalize and obtain parameter instance from this builder.
*/
public CustomerUpdateParams build() {
return new CustomerUpdateParams(this.accountBalance, this.coupon, this.defaultSource, this.description, this.email, this.expand, this.invoicePrefix, this.invoiceSettings, this.metadata, this.shipping, this.source, this.taxInfo, this.trialEnd);
}
/**
* An integer amount in %s that represents the account balance for your customer. Account
* balances only affect invoices. A negative amount represents a credit that decreases the
* amount due on an invoice; a positive amount increases the amount due on an invoice.
*/
public Builder setAccountBalance(Long accountBalance) {
this.accountBalance = accountBalance;
return this;
}
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/**
* ID of the default payment source for the customer.
*/
public Builder setDefaultSource(String defaultSource) {
this.defaultSource = defaultSource;
return this;
}
/**
* An arbitrary string that you can attach to a customer object. It is displayed alongside the
* customer in the dashboard.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* Customer's email address. It's displayed alongside the customer in your dashboard and can be
* useful for searching and tracking. This may be up to *512 characters*.
*/
public Builder setEmail(String email) {
this.email = email;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* CustomerUpdateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* CustomerUpdateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* The prefix for the customer used to generate unique invoice numbers. Must be 3–12 uppercase
* letters or numbers.
*/
public Builder setInvoicePrefix(String invoicePrefix) {
this.invoicePrefix = invoicePrefix;
return this;
}
/**
* Default invoice settings for this customer.
*/
public Builder setInvoiceSettings(InvoiceSettings invoiceSettings) {
this.invoiceSettings = invoiceSettings;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* CustomerUpdateParams#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link CustomerUpdateParams#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
public Builder setShipping(Shipping shipping) {
this.shipping = shipping;
return this;
}
/**
* The customer's shipping information. Appears on invoices emailed to this customer.
*/
public Builder setShipping(EmptyParam shipping) {
this.shipping = shipping;
return this;
}
public Builder setSource(String source) {
this.source = source;
return this;
}
/**
* The customer's tax information. Appears on invoices emailed to this customer.
*/
public Builder setTaxInfo(TaxInfo taxInfo) {
this.taxInfo = taxInfo;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the
* customer's trial immediately.
*/
public Builder setTrialEnd(TrialEnd trialEnd) {
this.trialEnd = trialEnd;
return this;
}
/**
* Unix timestamp representing the end of the trial period the customer will get before being
* charged for the first time. This will always overwrite any trials that might apply via a
* subscribed plan. If set, trial_end will override the default trial period of the plan the
* customer is being subscribed to. The special value `now` can be provided to end the
* customer's trial immediately.
*/
public Builder setTrialEnd(Long trialEnd) {
this.trialEnd = trialEnd;
return this;
}
}
public static class InvoiceSettings {
/**
* Default custom fields to be displayed on invoices for this customer.
*/
@SerializedName("custom_fields")
Object customFields;
/**
* ID of the default payment method for the customer.
*/
@SerializedName("default_payment_method")
String defaultPaymentMethod;
/**
* Default footer to be displayed on invoices for this customer.
*/
@SerializedName("footer")
String footer;
private InvoiceSettings(Object customFields, String defaultPaymentMethod, String footer) {
this.customFields = customFields;
this.defaultPaymentMethod = defaultPaymentMethod;
this.footer = footer;
}
public static Builder builder() {
return new com.stripe.param.CustomerUpdateParams.InvoiceSettings.Builder();
}
public static class Builder {
private Object customFields;
private String defaultPaymentMethod;
private String footer;
/**
* Finalize and obtain parameter instance from this builder.
*/
public InvoiceSettings build() {
return new InvoiceSettings(this.customFields, this.defaultPaymentMethod, this.footer);
}
/**
* Default custom fields to be displayed on invoices for this customer.
*/
public Builder setCustomFields(EmptyParam customFields) {
this.customFields = customFields;
return this;
}
/**
* Default custom fields to be displayed on invoices for this customer.
*/
public Builder setCustomFields(List customFields) {
this.customFields = customFields;
return this;
}
/**
* ID of the default payment method for the customer.
*/
public Builder setDefaultPaymentMethod(String defaultPaymentMethod) {
this.defaultPaymentMethod = defaultPaymentMethod;
return this;
}
/**
* Default footer to be displayed on invoices for this customer.
*/
public Builder setFooter(String footer) {
this.footer = footer;
return this;
}
}
public static class CustomField {
/** The name of the custom field. This may be up to 30 characters. */
@SerializedName("name")
String name;
/** The value of the custom field. This may be up to 30 characters. */
@SerializedName("value")
String value;
private CustomField(String name, String value) {
this.name = name;
this.value = value;
}
public static Builder builder() {
return new com.stripe.param.CustomerUpdateParams.InvoiceSettings.CustomField.Builder();
}
public static class Builder {
private String name;
private String value;
/** Finalize and obtain parameter instance from this builder. */
public CustomField build() {
return new CustomField(this.name, this.value);
}
/** The name of the custom field. This may be up to 30 characters. */
public Builder setName(String name) {
this.name = name;
return this;
}
public Builder setValue(String value) {
this.value = value;
return this;
}
}
}
}
/**
* The value of the custom field. This may be up to 30 characters.
*/
public static class Shipping {
/**
* Customer shipping address.
*/
@SerializedName("address")
Address address;
/**
* Customer name.
*/
@SerializedName("name")
String name;
/**
* Customer phone (including extension).
*/
@SerializedName("phone")
String phone;
private Shipping(Address address, String name, String phone) {
this.address = address;
this.name = name;
this.phone = phone;
}
public static Builder builder() {
return new com.stripe.param.CustomerUpdateParams.Shipping.Builder();
}
public static class Builder {
private Address address;
private String name;
private String phone;
/**
* Finalize and obtain parameter instance from this builder.
*/
public Shipping build() {
return new Shipping(this.address, this.name, this.phone);
}
/**
* Customer shipping address.
*/
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/**
* Customer name.
*/
public Builder setName(String name) {
this.name = name;
return this;
}
/**
* Customer phone (including extension).
*/
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
}
public static class Address {
@SerializedName("city")
String city;
@SerializedName("country")
String country;
@SerializedName("line1")
String line1;
@SerializedName("line2")
String line2;
@SerializedName("postal_code")
String postalCode;
@SerializedName("state")
String state;
private Address(String city, String country, String line1, String line2, String postalCode, String state) {
this.city = city;
this.country = country;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new com.stripe.param.CustomerUpdateParams.Shipping.Address.Builder();
}
public static class Builder {
private String city;
private String country;
private String line1;
private String line2;
private String postalCode;
private String state;
public Address build() {
return new Address(this.city, this.country, this.line1, this.line2, this.postalCode, this.state);
}
public Builder setCity(String city) {
this.city = city;
return this;
}
public Builder setCountry(String country) {
this.country = country;
return this;
}
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
public Builder setState(String state) {
this.state = state;
return this;
}
}
}
}
/**
* Finalize and obtain parameter instance from this builder.
*/
public static class TaxInfo {
/**
* The customer's tax ID number.
*/
@SerializedName("tax_id")
String taxId;
/**
* The type of ID number. The only possible value is `vat`
*/
@SerializedName("type")
Type type;
private TaxInfo(String taxId, Type type) {
this.taxId = taxId;
this.type = type;
}
public static Builder builder() {
return new com.stripe.param.CustomerUpdateParams.TaxInfo.Builder();
}
public static class Builder {
private String taxId;
private Type type;
/**
* Finalize and obtain parameter instance from this builder.
*/
public TaxInfo build() {
return new TaxInfo(this.taxId, this.type);
}
/**
* The customer's tax ID number.
*/
public Builder setTaxId(String taxId) {
this.taxId = taxId;
return this;
}
/**
* The type of ID number. The only possible value is `vat`
*/
public Builder setType(Type type) {
this.type = type;
return this;
}
}
public enum Type implements ApiRequestParams.EnumParam {
@SerializedName("vat")
VAT("vat");
private final String value;
Type(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
}
public enum TrialEnd implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now");
private final String value;
TrialEnd(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy