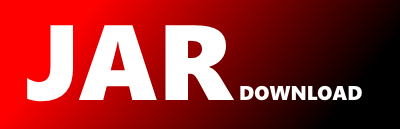
com.stripe.param.InvoiceUpcomingParams Maven / Gradle / Ivy
// Generated by delombok at Tue Apr 16 18:06:13 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class InvoiceUpcomingParams extends ApiRequestParams {
/**
* The code of the coupon to apply. If `subscription` or `subscription_items` is provided, the
* invoice returned will preview updating or creating a subscription with that coupon. Otherwise,
* it will preview applying that coupon to the customer for the next upcoming invoice from among
* the customer's subscriptions. The invoice can be previewed without a coupon by passing this
* value as an empty string.
*/
@SerializedName("coupon")
String coupon;
/**
* The identifier of the customer whose upcoming invoice you'd like to retrieve.
*/
@SerializedName("customer")
String customer;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* List of invoice items to add or update in the upcoming invoice preview.
*/
@SerializedName("invoice_items")
List invoiceItems;
/**
* The identifier of the subscription for which you'd like to retrieve the upcoming invoice. If
* not provided, but a `subscription_items` is provided, you will preview creating a subscription
* with those items. If neither `subscription` nor `subscription_items` is provided, you will
* retrieve the next upcoming invoice from among the customer's subscriptions.
*/
@SerializedName("subscription")
String subscription;
/**
* For new subscriptions, a future timestamp to anchor the subscription's [billing
* cycle](https://stripe.com/docs/subscriptions/billing-cycle). This is used to determine the date
* of the first full invoice, and, for plans with `month` or `year` intervals, the day of the
* month for subsequent invoices. For existing subscriptions, the value can only be set to `now`
* or `unchanged`.
*/
@SerializedName("subscription_billing_cycle_anchor")
Object subscriptionBillingCycleAnchor;
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
@SerializedName("subscription_cancel_at_period_end")
Boolean subscriptionCancelAtPeriodEnd;
@SerializedName("subscription_cancel_now")
Boolean subscriptionCancelNow;
/**
* List of subscription items, each with an attached plan.
*/
@SerializedName("subscription_items")
List subscriptionItems;
/**
* If previewing an update to a subscription, this decides whether the preview will show the
* result of applying prorations or not. If set, one of `subscription_items` or `subscription`,
* and one of `subscription_items` or `subscription_trial_end` are required.
*/
@SerializedName("subscription_prorate")
Boolean subscriptionProrate;
/**
* If previewing an update to a subscription, and doing proration, `subscription_proration_date`
* forces the proration to be calculated as though the update was done at the specified time. The
* time given must be within the current subscription period, and cannot be before the
* subscription was on its current plan. If set, `subscription`, and one of `subscription_items`,
* or `subscription_trial_end` are required. Also, `subscription_proration` cannot be set to
* false.
*/
@SerializedName("subscription_proration_date")
Long subscriptionProrationDate;
/**
* If provided, the invoice returned will preview updating or creating a subscription with that
* tax percent. If set, one of `subscription_items` or `subscription` is required.
*/
@SerializedName("subscription_tax_percent")
BigDecimal subscriptionTaxPercent;
/**
* If provided, the invoice returned will preview updating or creating a subscription with that
* trial end. If set, one of `subscription_items` or `subscription` is required.
*/
@SerializedName("subscription_trial_end")
Object subscriptionTrialEnd;
/**
* Indicates if a plan's `trial_period_days` should be applied to the subscription. Setting
* `subscription_trial_end` per subscription is preferred, and this defaults to `false`. Setting
* this flag to `true` together with `subscription_trial_end` is not allowed.
*/
@SerializedName("subscription_trial_from_plan")
Boolean subscriptionTrialFromPlan;
private InvoiceUpcomingParams(String coupon, String customer, List expand, List invoiceItems, String subscription, Object subscriptionBillingCycleAnchor, Boolean subscriptionCancelAtPeriodEnd, Boolean subscriptionCancelNow, List subscriptionItems, Boolean subscriptionProrate, Long subscriptionProrationDate, BigDecimal subscriptionTaxPercent, Object subscriptionTrialEnd, Boolean subscriptionTrialFromPlan) {
this.coupon = coupon;
this.customer = customer;
this.expand = expand;
this.invoiceItems = invoiceItems;
this.subscription = subscription;
this.subscriptionBillingCycleAnchor = subscriptionBillingCycleAnchor;
this.subscriptionCancelAtPeriodEnd = subscriptionCancelAtPeriodEnd;
this.subscriptionCancelNow = subscriptionCancelNow;
this.subscriptionItems = subscriptionItems;
this.subscriptionProrate = subscriptionProrate;
this.subscriptionProrationDate = subscriptionProrationDate;
this.subscriptionTaxPercent = subscriptionTaxPercent;
this.subscriptionTrialEnd = subscriptionTrialEnd;
this.subscriptionTrialFromPlan = subscriptionTrialFromPlan;
}
public static Builder builder() {
return new com.stripe.param.InvoiceUpcomingParams.Builder();
}
public static class Builder {
private String coupon;
private String customer;
private List expand;
private List invoiceItems;
private String subscription;
private Object subscriptionBillingCycleAnchor;
private Boolean subscriptionCancelAtPeriodEnd;
private Boolean subscriptionCancelNow;
private List subscriptionItems;
private Boolean subscriptionProrate;
private Long subscriptionProrationDate;
private BigDecimal subscriptionTaxPercent;
private Object subscriptionTrialEnd;
private Boolean subscriptionTrialFromPlan;
/**
* Finalize and obtain parameter instance from this builder.
*/
public InvoiceUpcomingParams build() {
return new InvoiceUpcomingParams(this.coupon, this.customer, this.expand, this.invoiceItems, this.subscription, this.subscriptionBillingCycleAnchor, this.subscriptionCancelAtPeriodEnd, this.subscriptionCancelNow, this.subscriptionItems, this.subscriptionProrate, this.subscriptionProrationDate, this.subscriptionTaxPercent, this.subscriptionTrialEnd, this.subscriptionTrialFromPlan);
}
/**
* The code of the coupon to apply. If `subscription` or `subscription_items` is provided, the
* invoice returned will preview updating or creating a subscription with that coupon.
* Otherwise, it will preview applying that coupon to the customer for the next upcoming invoice
* from among the customer's subscriptions. The invoice can be previewed without a coupon by
* passing this value as an empty string.
*/
public Builder setCoupon(String coupon) {
this.coupon = coupon;
return this;
}
/**
* The identifier of the customer whose upcoming invoice you'd like to retrieve.
*/
public Builder setCustomer(String customer) {
this.customer = customer;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* InvoiceUpcomingParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* InvoiceUpcomingParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add an element to `invoiceItems` list. A list is initialized for the first `add/addAll` call,
* and subsequent calls adds additional elements to the original list. See {@link
* InvoiceUpcomingParams#invoiceItems} for the field documentation.
*/
public Builder addInvoiceItem(InvoiceItem element) {
if (this.invoiceItems == null) {
this.invoiceItems = new ArrayList<>();
}
this.invoiceItems.add(element);
return this;
}
/**
* Add all elements to `invoiceItems` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* InvoiceUpcomingParams#invoiceItems} for the field documentation.
*/
public Builder addAllInvoiceItem(List elements) {
if (this.invoiceItems == null) {
this.invoiceItems = new ArrayList<>();
}
this.invoiceItems.addAll(elements);
return this;
}
/**
* The identifier of the subscription for which you'd like to retrieve the upcoming invoice. If
* not provided, but a `subscription_items` is provided, you will preview creating a
* subscription with those items. If neither `subscription` nor `subscription_items` is
* provided, you will retrieve the next upcoming invoice from among the customer's
* subscriptions.
*/
public Builder setSubscription(String subscription) {
this.subscription = subscription;
return this;
}
/**
* For new subscriptions, a future timestamp to anchor the subscription's [billing
* cycle](https://stripe.com/docs/subscriptions/billing-cycle). This is used to determine the
* date of the first full invoice, and, for plans with `month` or `year` intervals, the day of
* the month for subsequent invoices. For existing subscriptions, the value can only be set to
* `now` or `unchanged`.
*/
public Builder setSubscriptionBillingCycleAnchor(SubscriptionBillingCycleAnchor subscriptionBillingCycleAnchor) {
this.subscriptionBillingCycleAnchor = subscriptionBillingCycleAnchor;
return this;
}
/**
* For new subscriptions, a future timestamp to anchor the subscription's [billing
* cycle](https://stripe.com/docs/subscriptions/billing-cycle). This is used to determine the
* date of the first full invoice, and, for plans with `month` or `year` intervals, the day of
* the month for subsequent invoices. For existing subscriptions, the value can only be set to
* `now` or `unchanged`.
*/
public Builder setSubscriptionBillingCycleAnchor(Long subscriptionBillingCycleAnchor) {
this.subscriptionBillingCycleAnchor = subscriptionBillingCycleAnchor;
return this;
}
/**
* Boolean indicating whether this subscription should cancel at the end of the current period.
*/
public Builder setSubscriptionCancelAtPeriodEnd(Boolean subscriptionCancelAtPeriodEnd) {
this.subscriptionCancelAtPeriodEnd = subscriptionCancelAtPeriodEnd;
return this;
}
public Builder setSubscriptionCancelNow(Boolean subscriptionCancelNow) {
this.subscriptionCancelNow = subscriptionCancelNow;
return this;
}
/**
* Add an element to `subscriptionItems` list. A list is initialized for the first `add/addAll`
* call, and subsequent calls adds additional elements to the original list. See {@link
* InvoiceUpcomingParams#subscriptionItems} for the field documentation.
*/
public Builder addSubscriptionItem(SubscriptionItem element) {
if (this.subscriptionItems == null) {
this.subscriptionItems = new ArrayList<>();
}
this.subscriptionItems.add(element);
return this;
}
/**
* Add all elements to `subscriptionItems` list. A list is initialized for the first
* `add/addAll` call, and subsequent calls adds additional elements to the original list. See
* {@link InvoiceUpcomingParams#subscriptionItems} for the field documentation.
*/
public Builder addAllSubscriptionItem(List elements) {
if (this.subscriptionItems == null) {
this.subscriptionItems = new ArrayList<>();
}
this.subscriptionItems.addAll(elements);
return this;
}
/**
* If previewing an update to a subscription, this decides whether the preview will show the
* result of applying prorations or not. If set, one of `subscription_items` or `subscription`,
* and one of `subscription_items` or `subscription_trial_end` are required.
*/
public Builder setSubscriptionProrate(Boolean subscriptionProrate) {
this.subscriptionProrate = subscriptionProrate;
return this;
}
/**
* If previewing an update to a subscription, and doing proration, `subscription_proration_date`
* forces the proration to be calculated as though the update was done at the specified time.
* The time given must be within the current subscription period, and cannot be before the
* subscription was on its current plan. If set, `subscription`, and one of
* `subscription_items`, or `subscription_trial_end` are required. Also,
* `subscription_proration` cannot be set to false.
*/
public Builder setSubscriptionProrationDate(Long subscriptionProrationDate) {
this.subscriptionProrationDate = subscriptionProrationDate;
return this;
}
/**
* If provided, the invoice returned will preview updating or creating a subscription with that
* tax percent. If set, one of `subscription_items` or `subscription` is required.
*/
public Builder setSubscriptionTaxPercent(BigDecimal subscriptionTaxPercent) {
this.subscriptionTaxPercent = subscriptionTaxPercent;
return this;
}
/**
* If provided, the invoice returned will preview updating or creating a subscription with that
* trial end. If set, one of `subscription_items` or `subscription` is required.
*/
public Builder setSubscriptionTrialEnd(SubscriptionTrialEnd subscriptionTrialEnd) {
this.subscriptionTrialEnd = subscriptionTrialEnd;
return this;
}
/**
* If provided, the invoice returned will preview updating or creating a subscription with that
* trial end. If set, one of `subscription_items` or `subscription` is required.
*/
public Builder setSubscriptionTrialEnd(Long subscriptionTrialEnd) {
this.subscriptionTrialEnd = subscriptionTrialEnd;
return this;
}
/**
* Indicates if a plan's `trial_period_days` should be applied to the subscription. Setting
* `subscription_trial_end` per subscription is preferred, and this defaults to `false`. Setting
* this flag to `true` together with `subscription_trial_end` is not allowed.
*/
public Builder setSubscriptionTrialFromPlan(Boolean subscriptionTrialFromPlan) {
this.subscriptionTrialFromPlan = subscriptionTrialFromPlan;
return this;
}
}
public static class InvoiceItem {
/**
* The integer amount in **%s** of previewed invoice item.
*/
@SerializedName("amount")
Long amount;
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies). Only
* applicable to new invoice items.
*/
@SerializedName("currency")
String currency;
/**
* An arbitrary string which you can attach to the invoice item. The description is displayed in
* the invoice for easy tracking.
*/
@SerializedName("description")
String description;
/**
* Explicitly controls whether discounts apply to this invoice item. Defaults to true, except
* for negative invoice items.
*/
@SerializedName("discountable")
Boolean discountable;
/**
* The ID of the invoice item to update in preview. If not specified, a new invoice item will be
* added to the preview of the upcoming invoice.
*/
@SerializedName("invoiceitem")
String invoiceitem;
/**
* A set of key-value pairs that you can attach to an invoice item object. It can be useful for
* storing additional information about the invoice item in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The period associated with this invoice item.
*/
@SerializedName("period")
Period period;
/**
* Non-negative integer. The quantity of units for the invoice item.
*/
@SerializedName("quantity")
Long quantity;
/**
* The integer unit amount in **%s** of the charge to be applied to the upcoming invoice. This
* unit_amount will be multiplied by the quantity to get the full amount. If you want to apply a
* credit to the customer's account, pass a negative unit_amount.
*/
@SerializedName("unit_amount")
Long unitAmount;
private InvoiceItem(Long amount, String currency, String description, Boolean discountable, String invoiceitem, Map metadata, Period period, Long quantity, Long unitAmount) {
this.amount = amount;
this.currency = currency;
this.description = description;
this.discountable = discountable;
this.invoiceitem = invoiceitem;
this.metadata = metadata;
this.period = period;
this.quantity = quantity;
this.unitAmount = unitAmount;
}
public static Builder builder() {
return new com.stripe.param.InvoiceUpcomingParams.InvoiceItem.Builder();
}
public static class Builder {
private Long amount;
private String currency;
private String description;
private Boolean discountable;
private String invoiceitem;
private Map metadata;
private Period period;
private Long quantity;
private Long unitAmount;
/**
* Finalize and obtain parameter instance from this builder.
*/
public InvoiceItem build() {
return new InvoiceItem(this.amount, this.currency, this.description, this.discountable, this.invoiceitem, this.metadata, this.period, this.quantity, this.unitAmount);
}
/**
* The integer amount in **%s** of previewed invoice item.
*/
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies). Only
* applicable to new invoice items.
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* An arbitrary string which you can attach to the invoice item. The description is displayed
* in the invoice for easy tracking.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* Explicitly controls whether discounts apply to this invoice item. Defaults to true, except
* for negative invoice items.
*/
public Builder setDiscountable(Boolean discountable) {
this.discountable = discountable;
return this;
}
/**
* The ID of the invoice item to update in preview. If not specified, a new invoice item will
* be added to the preview of the upcoming invoice.
*/
public Builder setInvoiceitem(String invoiceitem) {
this.invoiceitem = invoiceitem;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* InvoiceItem#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link InvoiceItem#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* The period associated with this invoice item.
*/
public Builder setPeriod(Period period) {
this.period = period;
return this;
}
/**
* Non-negative integer. The quantity of units for the invoice item.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
/**
* The integer unit amount in **%s** of the charge to be applied to the upcoming invoice. This
* unit_amount will be multiplied by the quantity to get the full amount. If you want to apply
* a credit to the customer's account, pass a negative unit_amount.
*/
public Builder setUnitAmount(Long unitAmount) {
this.unitAmount = unitAmount;
return this;
}
}
public static class Period {
/** The end of the period, which must be greater than or equal to the start. */
@SerializedName("end")
Long end;
/** The start of the period. */
@SerializedName("start")
Long start;
private Period(Long end, Long start) {
this.end = end;
this.start = start;
}
public static Builder builder() {
return new com.stripe.param.InvoiceUpcomingParams.InvoiceItem.Period.Builder();
}
public static class Builder {
private Long end;
private Long start;
/** Finalize and obtain parameter instance from this builder. */
public Period build() {
return new Period(this.end, this.start);
}
/** The end of the period, which must be greater than or equal to the start. */
public Builder setEnd(Long end) {
this.end = end;
return this;
}
public Builder setStart(Long start) {
this.start = start;
return this;
}
}
}
}
/**
* The start of the period.
*/
public static class SubscriptionItem {
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
@SerializedName("billing_thresholds")
Object billingThresholds;
/**
* Delete all usage for a given subscription item. Allowed only when `deleted` is set to `true`
* and the current plan's `usage_type` is `metered`.
*/
@SerializedName("clear_usage")
Boolean clearUsage;
/**
* A flag that, if set to `true`, will delete the specified item.
*/
@SerializedName("deleted")
Boolean deleted;
/**
* Subscription item to update.
*/
@SerializedName("id")
String id;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* Plan ID for this item, as a string.
*/
@SerializedName("plan")
String plan;
/**
* Quantity for this item.
*/
@SerializedName("quantity")
Long quantity;
private SubscriptionItem(Object billingThresholds, Boolean clearUsage, Boolean deleted, String id, Map metadata, String plan, Long quantity) {
this.billingThresholds = billingThresholds;
this.clearUsage = clearUsage;
this.deleted = deleted;
this.id = id;
this.metadata = metadata;
this.plan = plan;
this.quantity = quantity;
}
public static Builder builder() {
return new com.stripe.param.InvoiceUpcomingParams.SubscriptionItem.Builder();
}
public static class Builder {
private Object billingThresholds;
private Boolean clearUsage;
private Boolean deleted;
private String id;
private Map metadata;
private String plan;
private Long quantity;
/**
* Finalize and obtain parameter instance from this builder.
*/
public SubscriptionItem build() {
return new SubscriptionItem(this.billingThresholds, this.clearUsage, this.deleted, this.id, this.metadata, this.plan, this.quantity);
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
public Builder setBillingThresholds(BillingThresholds billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Define thresholds at which an invoice will be sent, and the subscription advanced to a new
* billing period.
*/
public Builder setBillingThresholds(EmptyParam billingThresholds) {
this.billingThresholds = billingThresholds;
return this;
}
/**
* Delete all usage for a given subscription item. Allowed only when `deleted` is set to
* `true` and the current plan's `usage_type` is `metered`.
*/
public Builder setClearUsage(Boolean clearUsage) {
this.clearUsage = clearUsage;
return this;
}
/**
* A flag that, if set to `true`, will delete the specified item.
*/
public Builder setDeleted(Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Subscription item to update.
*/
public Builder setId(String id) {
this.id = id;
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll`
* call, and subsequent calls add additional key/value pairs to the original map. See {@link
* SubscriptionItem#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link SubscriptionItem#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* Plan ID for this item, as a string.
*/
public Builder setPlan(String plan) {
this.plan = plan;
return this;
}
/**
* Quantity for this item.
*/
public Builder setQuantity(Long quantity) {
this.quantity = quantity;
return this;
}
}
public static class BillingThresholds {
/** Usage threshold that triggers the subscription to advance to a new billing period. */
@SerializedName("usage_gte")
Long usageGte;
private BillingThresholds(Long usageGte) {
this.usageGte = usageGte;
}
public static Builder builder() {
return new com.stripe.param.InvoiceUpcomingParams.SubscriptionItem.BillingThresholds.Builder();
}
public static class Builder {
private Long usageGte;
/** Finalize and obtain parameter instance from this builder. */
public BillingThresholds build() {
return new BillingThresholds(this.usageGte);
}
public Builder setUsageGte(Long usageGte) {
this.usageGte = usageGte;
return this;
}
}
}
}
/**
* Usage threshold that triggers the subscription to advance to a new billing period.
*/
public enum SubscriptionBillingCycleAnchor implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now"), @SerializedName("unchanged")
UNCHANGED("unchanged");
private final String value;
SubscriptionBillingCycleAnchor(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum SubscriptionTrialEnd implements ApiRequestParams.EnumParam {
@SerializedName("now")
NOW("now");
private final String value;
SubscriptionTrialEnd(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy