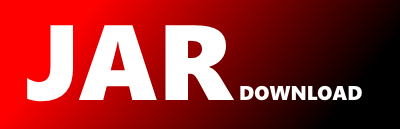
com.stripe.param.ChargeCaptureParams Maven / Gradle / Ivy
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import java.util.ArrayList;
import java.util.List;
public class ChargeCaptureParams extends ApiRequestParams {
/**
* The amount to capture, which must be less than or equal to the original amount. Any additional
* amount will be automatically refunded.
*/
@SerializedName("amount")
Long amount;
/** An application fee to add on to this charge. Can only be used with Stripe Connect. */
@SerializedName("application_fee")
Long applicationFee;
/**
* An application fee amount to add on to this charge, which must be less than or equal to the
* original amount. Can only be used with Stripe Connect.
*/
@SerializedName("application_fee_amount")
Long applicationFeeAmount;
/** Specifies which fields in the response should be expanded. */
@SerializedName("expand")
List expand;
/**
* The email address to send this charge's receipt to. This will override the previously-specified
* email address for this charge, if one was set. Receipts will not be sent in test mode.
*/
@SerializedName("receipt_email")
String receiptEmail;
/**
* An arbitrary string to be used as the dynamic portion of the full descriptor displayed on your
* customer's credit card statement. This value will be prefixed by your [account's statement
* descriptor](https://stripe.com/docs/charges#dynamic-statement-descriptor). As an example, if
* your account's statement descriptor is `RUNCLUB` and the item you're charging for is a race
* ticket, you may want to specify a `statement_descriptor` of `5K RACE`, so that the resulting
* full descriptor would be `RUNCLUB* 5K RACE`. The full descriptor may be up to *22 characters*.
* This value must contain at least one letter, may not include `"'` characters, and will appear
* on your customer's statement in capital letters. Non-ASCII characters are automatically
* stripped. Updating this value will overwrite the previous `statement_descriptor` of this
* charge. While most banks display this information consistently, some may display it incorrectly
* or not at all.
*/
@SerializedName("statement_descriptor")
String statementDescriptor;
/**
* An optional dictionary including the account to automatically transfer to as part of a
* destination charge. [See the Connect
* documentation](https://stripe.com/docs/connect/destination-charges) for details.
*/
@SerializedName("transfer_data")
TransferData transferData;
/**
* A string that identifies this transaction as part of a group. `transfer_group` may only be
* provided if it has not been set. See the [Connect
* documentation](https://stripe.com/docs/connect/charges-transfers#grouping-transactions) for
* details.
*/
@SerializedName("transfer_group")
String transferGroup;
private ChargeCaptureParams(
Long amount,
Long applicationFee,
Long applicationFeeAmount,
List expand,
String receiptEmail,
String statementDescriptor,
TransferData transferData,
String transferGroup) {
this.amount = amount;
this.applicationFee = applicationFee;
this.applicationFeeAmount = applicationFeeAmount;
this.expand = expand;
this.receiptEmail = receiptEmail;
this.statementDescriptor = statementDescriptor;
this.transferData = transferData;
this.transferGroup = transferGroup;
}
public static Builder builder() {
return new com.stripe.param.ChargeCaptureParams.Builder();
}
public static class Builder {
private Long amount;
private Long applicationFee;
private Long applicationFeeAmount;
private List expand;
private String receiptEmail;
private String statementDescriptor;
private TransferData transferData;
private String transferGroup;
/** Finalize and obtain parameter instance from this builder. */
public ChargeCaptureParams build() {
return new ChargeCaptureParams(
this.amount,
this.applicationFee,
this.applicationFeeAmount,
this.expand,
this.receiptEmail,
this.statementDescriptor,
this.transferData,
this.transferGroup);
}
/**
* The amount to capture, which must be less than or equal to the original amount. Any
* additional amount will be automatically refunded.
*/
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/** An application fee to add on to this charge. Can only be used with Stripe Connect. */
public Builder setApplicationFee(Long applicationFee) {
this.applicationFee = applicationFee;
return this;
}
/**
* An application fee amount to add on to this charge, which must be less than or equal to the
* original amount. Can only be used with Stripe Connect.
*/
public Builder setApplicationFeeAmount(Long applicationFeeAmount) {
this.applicationFeeAmount = applicationFeeAmount;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* ChargeCaptureParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* ChargeCaptureParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* The email address to send this charge's receipt to. This will override the
* previously-specified email address for this charge, if one was set. Receipts will not be sent
* in test mode.
*/
public Builder setReceiptEmail(String receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* An arbitrary string to be used as the dynamic portion of the full descriptor displayed on
* your customer's credit card statement. This value will be prefixed by your [account's
* statement descriptor](https://stripe.com/docs/charges#dynamic-statement-descriptor). As an
* example, if your account's statement descriptor is `RUNCLUB` and the item you're charging for
* is a race ticket, you may want to specify a `statement_descriptor` of `5K RACE`, so that the
* resulting full descriptor would be `RUNCLUB* 5K RACE`. The full descriptor may be up to *22
* characters*. This value must contain at least one letter, may not include `"'` characters,
* and will appear on your customer's statement in capital letters. Non-ASCII characters are
* automatically stripped. Updating this value will overwrite the previous
* `statement_descriptor` of this charge. While most banks display this information
* consistently, some may display it incorrectly or not at all.
*/
public Builder setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
/**
* An optional dictionary including the account to automatically transfer to as part of a
* destination charge. [See the Connect
* documentation](https://stripe.com/docs/connect/destination-charges) for details.
*/
public Builder setTransferData(TransferData transferData) {
this.transferData = transferData;
return this;
}
/**
* A string that identifies this transaction as part of a group. `transfer_group` may only be
* provided if it has not been set. See the [Connect
* documentation](https://stripe.com/docs/connect/charges-transfers#grouping-transactions) for
* details.
*/
public Builder setTransferGroup(String transferGroup) {
this.transferGroup = transferGroup;
return this;
}
}
public static class TransferData {
/**
* The amount transferred to the destination account, if specified. By default, the entire
* charge amount is transferred to the destination account.
*/
@SerializedName("amount")
Long amount;
private TransferData(Long amount) {
this.amount = amount;
}
public static Builder builder() {
return new com.stripe.param.ChargeCaptureParams.TransferData.Builder();
}
public static class Builder {
private Long amount;
/** Finalize and obtain parameter instance from this builder. */
public TransferData build() {
return new TransferData(this.amount);
}
/**
* The amount transferred to the destination account, if specified. By default, the entire
* charge amount is transferred to the destination account.
*/
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy