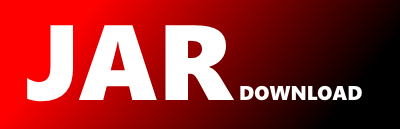
com.stripe.param.PayoutCreateParams Maven / Gradle / Ivy
// Generated by delombok at Wed Apr 24 18:37:47 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class PayoutCreateParams extends ApiRequestParams {
/**
* A positive integer in cents representing how much to payout.
*/
@SerializedName("amount")
Long amount;
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
@SerializedName("currency")
String currency;
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
@SerializedName("description")
String description;
/**
* The ID of a bank account or a card to send the payout to. If no destination is supplied, the
* default external account for the specified currency will be used.
*/
@SerializedName("destination")
String destination;
/**
* Specifies which fields in the response should be expanded.
*/
@SerializedName("expand")
List expand;
/**
* A set of key-value pairs that you can attach to a payout object. It can be useful for storing
* additional information about the payout in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* The method used to send this payout, which can be `standard` or `instant`. `instant` is only
* supported for payouts to debit cards. (See [Instant payouts for marketplaces for more
* information](https://stripe.com/blog/instant-payouts-for-marketplaces).)
*/
@SerializedName("method")
Method method;
/**
* The source balance to draw this payout from. Balances for different payment sources are kept
* separately. You can find the amounts with the balances API. One of `bank_account` or `card`.
*/
@SerializedName("source_type")
SourceType sourceType;
/**
* A string to be displayed on the recipient's bank or card statement. This may be at most 22
* characters. Attempting to use a `statement_descriptor` longer than 22 characters will return an
* error. Note: Most banks will truncate this information and/or display it inconsistently. Some
* may not display it at all.
*/
@SerializedName("statement_descriptor")
String statementDescriptor;
private PayoutCreateParams(Long amount, String currency, String description, String destination, List expand, Map metadata, Method method, SourceType sourceType, String statementDescriptor) {
this.amount = amount;
this.currency = currency;
this.description = description;
this.destination = destination;
this.expand = expand;
this.metadata = metadata;
this.method = method;
this.sourceType = sourceType;
this.statementDescriptor = statementDescriptor;
}
public static Builder builder() {
return new com.stripe.param.PayoutCreateParams.Builder();
}
public static class Builder {
private Long amount;
private String currency;
private String description;
private String destination;
private List expand;
private Map metadata;
private Method method;
private SourceType sourceType;
private String statementDescriptor;
/**
* Finalize and obtain parameter instance from this builder.
*/
public PayoutCreateParams build() {
return new PayoutCreateParams(this.amount, this.currency, this.description, this.destination, this.expand, this.metadata, this.method, this.sourceType, this.statementDescriptor);
}
/**
* A positive integer in cents representing how much to payout.
*/
public Builder setAmount(Long amount) {
this.amount = amount;
return this;
}
/**
* Three-letter [ISO currency code](https://www.iso.org/iso-4217-currency-codes.html), in
* lowercase. Must be a [supported currency](https://stripe.com/docs/currencies).
*/
public Builder setCurrency(String currency) {
this.currency = currency;
return this;
}
/**
* An arbitrary string attached to the object. Often useful for displaying to users.
*/
public Builder setDescription(String description) {
this.description = description;
return this;
}
/**
* The ID of a bank account or a card to send the payout to. If no destination is supplied, the
* default external account for the specified currency will be used.
*/
public Builder setDestination(String destination) {
this.destination = destination;
return this;
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PayoutCreateParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PayoutCreateParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/**
* Add a key/value pair to `metadata` map. A map is initialized for the first `put/putAll` call,
* and subsequent calls add additional key/value pairs to the original map. See {@link
* PayoutCreateParams#metadata} for the field documentation.
*/
public Builder putMetadata(String key, String value) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.put(key, value);
return this;
}
/**
* Add all map key/value pairs to `metadata` map. A map is initialized for the first
* `put/putAll` call, and subsequent calls add additional key/value pairs to the original map.
* See {@link PayoutCreateParams#metadata} for the field documentation.
*/
public Builder putAllMetadata(Map map) {
if (this.metadata == null) {
this.metadata = new HashMap<>();
}
this.metadata.putAll(map);
return this;
}
/**
* The method used to send this payout, which can be `standard` or `instant`. `instant` is only
* supported for payouts to debit cards. (See [Instant payouts for marketplaces for more
* information](https://stripe.com/blog/instant-payouts-for-marketplaces).)
*/
public Builder setMethod(Method method) {
this.method = method;
return this;
}
/**
* The source balance to draw this payout from. Balances for different payment sources are kept
* separately. You can find the amounts with the balances API. One of `bank_account` or `card`.
*/
public Builder setSourceType(SourceType sourceType) {
this.sourceType = sourceType;
return this;
}
/**
* A string to be displayed on the recipient's bank or card statement. This may be at most 22
* characters. Attempting to use a `statement_descriptor` longer than 22 characters will return
* an error. Note: Most banks will truncate this information and/or display it inconsistently.
* Some may not display it at all.
*/
public Builder setStatementDescriptor(String statementDescriptor) {
this.statementDescriptor = statementDescriptor;
return this;
}
}
public enum Method implements ApiRequestParams.EnumParam {
@SerializedName("instant")
INSTANT("instant"), @SerializedName("standard")
STANDARD("standard");
private final String value;
Method(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
public enum SourceType implements ApiRequestParams.EnumParam {
@SerializedName("bank_account")
BANK_ACCOUNT("bank_account"), @SerializedName("card")
CARD("card");
private final String value;
SourceType(String value) {
this.value = value;
}
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getValue() {
return this.value;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy