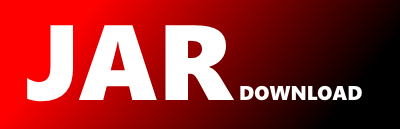
com.stripe.model.SubscriptionScheduleRevision Maven / Gradle / Ivy
// Generated by delombok at Fri May 03 17:08:00 PDT 2019
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.model;
import com.google.gson.annotations.SerializedName;
import java.util.List;
import java.util.Map;
public class SubscriptionScheduleRevision extends StripeObject implements HasId {
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@SerializedName("created")
Long created;
/**
* Unique identifier for the object.
*/
@SerializedName("id")
String id;
@SerializedName("invoice_settings")
SubscriptionSchedule.InvoiceSettings invoiceSettings;
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@SerializedName("livemode")
Boolean livemode;
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@SerializedName("metadata")
Map metadata;
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@SerializedName("object")
String object;
/**
* Configuration for the subscription schedule's phases.
*/
@SerializedName("phases")
List phases;
@SerializedName("previous_revision")
String previousRevision;
/**
* Behavior of the subscription schedule and underlying subscription when it ends.
*/
@SerializedName("renewal_behavior")
String renewalBehavior;
/**
* Interval and duration at which the subscription schedule renews for when it ends if
* `renewal_behavior` is `renew`.
*/
@SerializedName("renewal_interval")
SubscriptionSchedule.RenewalInterval renewalInterval;
/**
* ID of the subscription schedule the revision points to.
*/
@SerializedName("schedule")
String schedule;
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Long getCreated() {
return this.created;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionSchedule.InvoiceSettings getInvoiceSettings() {
return this.invoiceSettings;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Boolean getLivemode() {
return this.livemode;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public Map getMetadata() {
return this.metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getObject() {
return this.object;
}
/**
* Configuration for the subscription schedule's phases.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public List getPhases() {
return this.phases;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getPreviousRevision() {
return this.previousRevision;
}
/**
* Behavior of the subscription schedule and underlying subscription when it ends.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getRenewalBehavior() {
return this.renewalBehavior;
}
/**
* Interval and duration at which the subscription schedule renews for when it ends if
* `renewal_behavior` is `renew`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public SubscriptionSchedule.RenewalInterval getRenewalInterval() {
return this.renewalInterval;
}
/**
* ID of the subscription schedule the revision points to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getSchedule() {
return this.schedule;
}
/**
* Time at which the object was created. Measured in seconds since the Unix epoch.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setCreated(final Long created) {
this.created = created;
}
/**
* Unique identifier for the object.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setId(final String id) {
this.id = id;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setInvoiceSettings(final SubscriptionSchedule.InvoiceSettings invoiceSettings) {
this.invoiceSettings = invoiceSettings;
}
/**
* Has the value `true` if the object exists in live mode or the value `false` if the object
* exists in test mode.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setLivemode(final Boolean livemode) {
this.livemode = livemode;
}
/**
* Set of key-value pairs that you can attach to an object. This can be useful for storing
* additional information about the object in a structured format.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setMetadata(final Map metadata) {
this.metadata = metadata;
}
/**
* String representing the object's type. Objects of the same type share the same value.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setObject(final String object) {
this.object = object;
}
/**
* Configuration for the subscription schedule's phases.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPhases(final List phases) {
this.phases = phases;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setPreviousRevision(final String previousRevision) {
this.previousRevision = previousRevision;
}
/**
* Behavior of the subscription schedule and underlying subscription when it ends.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRenewalBehavior(final String renewalBehavior) {
this.renewalBehavior = renewalBehavior;
}
/**
* Interval and duration at which the subscription schedule renews for when it ends if
* `renewal_behavior` is `renew`.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setRenewalInterval(final SubscriptionSchedule.RenewalInterval renewalInterval) {
this.renewalInterval = renewalInterval;
}
/**
* ID of the subscription schedule the revision points to.
*/
@java.lang.SuppressWarnings("all")
@lombok.Generated
public void setSchedule(final String schedule) {
this.schedule = schedule;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public boolean equals(final java.lang.Object o) {
if (o == this) return true;
if (!(o instanceof SubscriptionScheduleRevision)) return false;
final SubscriptionScheduleRevision other = (SubscriptionScheduleRevision) o;
if (!other.canEqual((java.lang.Object) this)) return false;
final java.lang.Object this$created = this.getCreated();
final java.lang.Object other$created = other.getCreated();
if (this$created == null ? other$created != null : !this$created.equals(other$created)) return false;
final java.lang.Object this$id = this.getId();
final java.lang.Object other$id = other.getId();
if (this$id == null ? other$id != null : !this$id.equals(other$id)) return false;
final java.lang.Object this$invoiceSettings = this.getInvoiceSettings();
final java.lang.Object other$invoiceSettings = other.getInvoiceSettings();
if (this$invoiceSettings == null ? other$invoiceSettings != null : !this$invoiceSettings.equals(other$invoiceSettings)) return false;
final java.lang.Object this$livemode = this.getLivemode();
final java.lang.Object other$livemode = other.getLivemode();
if (this$livemode == null ? other$livemode != null : !this$livemode.equals(other$livemode)) return false;
final java.lang.Object this$metadata = this.getMetadata();
final java.lang.Object other$metadata = other.getMetadata();
if (this$metadata == null ? other$metadata != null : !this$metadata.equals(other$metadata)) return false;
final java.lang.Object this$object = this.getObject();
final java.lang.Object other$object = other.getObject();
if (this$object == null ? other$object != null : !this$object.equals(other$object)) return false;
final java.lang.Object this$phases = this.getPhases();
final java.lang.Object other$phases = other.getPhases();
if (this$phases == null ? other$phases != null : !this$phases.equals(other$phases)) return false;
final java.lang.Object this$previousRevision = this.getPreviousRevision();
final java.lang.Object other$previousRevision = other.getPreviousRevision();
if (this$previousRevision == null ? other$previousRevision != null : !this$previousRevision.equals(other$previousRevision)) return false;
final java.lang.Object this$renewalBehavior = this.getRenewalBehavior();
final java.lang.Object other$renewalBehavior = other.getRenewalBehavior();
if (this$renewalBehavior == null ? other$renewalBehavior != null : !this$renewalBehavior.equals(other$renewalBehavior)) return false;
final java.lang.Object this$renewalInterval = this.getRenewalInterval();
final java.lang.Object other$renewalInterval = other.getRenewalInterval();
if (this$renewalInterval == null ? other$renewalInterval != null : !this$renewalInterval.equals(other$renewalInterval)) return false;
final java.lang.Object this$schedule = this.getSchedule();
final java.lang.Object other$schedule = other.getSchedule();
if (this$schedule == null ? other$schedule != null : !this$schedule.equals(other$schedule)) return false;
return true;
}
@java.lang.SuppressWarnings("all")
@lombok.Generated
protected boolean canEqual(final java.lang.Object other) {
return other instanceof SubscriptionScheduleRevision;
}
@java.lang.Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public int hashCode() {
final int PRIME = 59;
int result = 1;
final java.lang.Object $created = this.getCreated();
result = result * PRIME + ($created == null ? 43 : $created.hashCode());
final java.lang.Object $id = this.getId();
result = result * PRIME + ($id == null ? 43 : $id.hashCode());
final java.lang.Object $invoiceSettings = this.getInvoiceSettings();
result = result * PRIME + ($invoiceSettings == null ? 43 : $invoiceSettings.hashCode());
final java.lang.Object $livemode = this.getLivemode();
result = result * PRIME + ($livemode == null ? 43 : $livemode.hashCode());
final java.lang.Object $metadata = this.getMetadata();
result = result * PRIME + ($metadata == null ? 43 : $metadata.hashCode());
final java.lang.Object $object = this.getObject();
result = result * PRIME + ($object == null ? 43 : $object.hashCode());
final java.lang.Object $phases = this.getPhases();
result = result * PRIME + ($phases == null ? 43 : $phases.hashCode());
final java.lang.Object $previousRevision = this.getPreviousRevision();
result = result * PRIME + ($previousRevision == null ? 43 : $previousRevision.hashCode());
final java.lang.Object $renewalBehavior = this.getRenewalBehavior();
result = result * PRIME + ($renewalBehavior == null ? 43 : $renewalBehavior.hashCode());
final java.lang.Object $renewalInterval = this.getRenewalInterval();
result = result * PRIME + ($renewalInterval == null ? 43 : $renewalInterval.hashCode());
final java.lang.Object $schedule = this.getSchedule();
result = result * PRIME + ($schedule == null ? 43 : $schedule.hashCode());
return result;
}
/**
* Unique identifier for the object.
*/
@Override
@java.lang.SuppressWarnings("all")
@lombok.Generated
public String getId() {
return this.id;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy