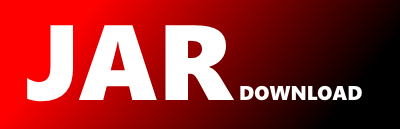
com.stripe.param.PaymentIntentConfirmParams Maven / Gradle / Ivy
// Generated by com.stripe.generator.entity.SdkBuilder
package com.stripe.param;
import com.google.gson.annotations.SerializedName;
import com.stripe.net.ApiRequestParams;
import com.stripe.param.common.EmptyParam;
import java.util.ArrayList;
import java.util.List;
public class PaymentIntentConfirmParams extends ApiRequestParams {
/** Specifies which fields in the response should be expanded. */
@SerializedName("expand")
List expand;
/** ID of the payment method to attach to this PaymentIntent. */
@SerializedName("payment_method")
String paymentMethod;
/** Email address that the receipt for the resulting payment will be sent to. */
@SerializedName("receipt_email")
String receiptEmail;
/**
* The URL to redirect your customer back to after they authenticate or cancel their payment on
* the payment method's app or site. If you'd prefer to redirect to a mobile application, you can
* alternatively supply an application URI scheme. This parameter is only used for cards and other
* redirect-based payment methods.
*/
@SerializedName("return_url")
String returnUrl;
/**
* Set to `true` to save the PaymentIntent's payment method (either `source` or `payment_method`)
* to the associated customer. If the payment method is already attached, this parameter does
* nothing. This parameter defaults to `false` and applies to the payment method passed in the
* same request or the current payment method attached to the PaymentIntent and must be specified
* again if a new payment method is added.
*/
@SerializedName("save_payment_method")
Boolean savePaymentMethod;
/** Shipping information for this PaymentIntent. */
@SerializedName("shipping")
Object shipping;
/** ID of the Source object to attach to this PaymentIntent. */
@SerializedName("source")
String source;
private PaymentIntentConfirmParams(
List expand,
String paymentMethod,
String receiptEmail,
String returnUrl,
Boolean savePaymentMethod,
Object shipping,
String source) {
this.expand = expand;
this.paymentMethod = paymentMethod;
this.receiptEmail = receiptEmail;
this.returnUrl = returnUrl;
this.savePaymentMethod = savePaymentMethod;
this.shipping = shipping;
this.source = source;
}
public static Builder builder() {
return new com.stripe.param.PaymentIntentConfirmParams.Builder();
}
public static class Builder {
private List expand;
private String paymentMethod;
private String receiptEmail;
private String returnUrl;
private Boolean savePaymentMethod;
private Object shipping;
private String source;
/** Finalize and obtain parameter instance from this builder. */
public PaymentIntentConfirmParams build() {
return new PaymentIntentConfirmParams(
this.expand,
this.paymentMethod,
this.receiptEmail,
this.returnUrl,
this.savePaymentMethod,
this.shipping,
this.source);
}
/**
* Add an element to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentConfirmParams#expand} for the field documentation.
*/
public Builder addExpand(String element) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.add(element);
return this;
}
/**
* Add all elements to `expand` list. A list is initialized for the first `add/addAll` call, and
* subsequent calls adds additional elements to the original list. See {@link
* PaymentIntentConfirmParams#expand} for the field documentation.
*/
public Builder addAllExpand(List elements) {
if (this.expand == null) {
this.expand = new ArrayList<>();
}
this.expand.addAll(elements);
return this;
}
/** ID of the payment method to attach to this PaymentIntent. */
public Builder setPaymentMethod(String paymentMethod) {
this.paymentMethod = paymentMethod;
return this;
}
/** Email address that the receipt for the resulting payment will be sent to. */
public Builder setReceiptEmail(String receiptEmail) {
this.receiptEmail = receiptEmail;
return this;
}
/**
* The URL to redirect your customer back to after they authenticate or cancel their payment on
* the payment method's app or site. If you'd prefer to redirect to a mobile application, you
* can alternatively supply an application URI scheme. This parameter is only used for cards and
* other redirect-based payment methods.
*/
public Builder setReturnUrl(String returnUrl) {
this.returnUrl = returnUrl;
return this;
}
/**
* Set to `true` to save the PaymentIntent's payment method (either `source` or
* `payment_method`) to the associated customer. If the payment method is already attached, this
* parameter does nothing. This parameter defaults to `false` and applies to the payment method
* passed in the same request or the current payment method attached to the PaymentIntent and
* must be specified again if a new payment method is added.
*/
public Builder setSavePaymentMethod(Boolean savePaymentMethod) {
this.savePaymentMethod = savePaymentMethod;
return this;
}
/** Shipping information for this PaymentIntent. */
public Builder setShipping(Shipping shipping) {
this.shipping = shipping;
return this;
}
/** Shipping information for this PaymentIntent. */
public Builder setShipping(EmptyParam shipping) {
this.shipping = shipping;
return this;
}
/** ID of the Source object to attach to this PaymentIntent. */
public Builder setSource(String source) {
this.source = source;
return this;
}
}
public static class Shipping {
/** Shipping address. */
@SerializedName("address")
Address address;
/** The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc. */
@SerializedName("carrier")
String carrier;
/** Recipient name. */
@SerializedName("name")
String name;
/** Recipient phone (including extension). */
@SerializedName("phone")
String phone;
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
@SerializedName("tracking_number")
String trackingNumber;
private Shipping(
Address address, String carrier, String name, String phone, String trackingNumber) {
this.address = address;
this.carrier = carrier;
this.name = name;
this.phone = phone;
this.trackingNumber = trackingNumber;
}
public static Builder builder() {
return new com.stripe.param.PaymentIntentConfirmParams.Shipping.Builder();
}
public static class Builder {
private Address address;
private String carrier;
private String name;
private String phone;
private String trackingNumber;
/** Finalize and obtain parameter instance from this builder. */
public Shipping build() {
return new Shipping(this.address, this.carrier, this.name, this.phone, this.trackingNumber);
}
/** Shipping address. */
public Builder setAddress(Address address) {
this.address = address;
return this;
}
/** The delivery service that shipped a physical product, such as Fedex, UPS, USPS, etc. */
public Builder setCarrier(String carrier) {
this.carrier = carrier;
return this;
}
/** Recipient name. */
public Builder setName(String name) {
this.name = name;
return this;
}
/** Recipient phone (including extension). */
public Builder setPhone(String phone) {
this.phone = phone;
return this;
}
/**
* The tracking number for a physical product, obtained from the delivery service. If multiple
* tracking numbers were generated for this purchase, please separate them with commas.
*/
public Builder setTrackingNumber(String trackingNumber) {
this.trackingNumber = trackingNumber;
return this;
}
}
public static class Address {
@SerializedName("city")
String city;
@SerializedName("country")
String country;
@SerializedName("line1")
String line1;
@SerializedName("line2")
String line2;
@SerializedName("postal_code")
String postalCode;
@SerializedName("state")
String state;
private Address(
String city,
String country,
String line1,
String line2,
String postalCode,
String state) {
this.city = city;
this.country = country;
this.line1 = line1;
this.line2 = line2;
this.postalCode = postalCode;
this.state = state;
}
public static Builder builder() {
return new com.stripe.param.PaymentIntentConfirmParams.Shipping.Address.Builder();
}
public static class Builder {
private String city;
private String country;
private String line1;
private String line2;
private String postalCode;
private String state;
/** Finalize and obtain parameter instance from this builder. */
public Address build() {
return new Address(
this.city, this.country, this.line1, this.line2, this.postalCode, this.state);
}
public Builder setCity(String city) {
this.city = city;
return this;
}
public Builder setCountry(String country) {
this.country = country;
return this;
}
public Builder setLine1(String line1) {
this.line1 = line1;
return this;
}
public Builder setLine2(String line2) {
this.line2 = line2;
return this;
}
public Builder setPostalCode(String postalCode) {
this.postalCode = postalCode;
return this;
}
public Builder setState(String state) {
this.state = state;
return this;
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy