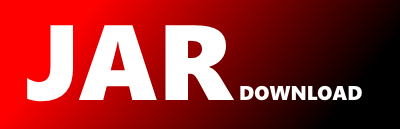
com.strongloop.android.remoting.BeanUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of strong-remoting-android Show documentation
Show all versions of strong-remoting-android Show documentation
Android client for strong-remoting
The newest version!
package com.strongloop.android.remoting;
import android.util.Log;
import java.lang.reflect.Array;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class BeanUtil {
public static void setProperties(Object object, Map properties, boolean includeSuperClasses) {
if (object == null || properties == null) {
return;
}
Class> objectClass = object.getClass();
for (Map.Entry entry : properties.entrySet()) {
String key = entry.getKey();
Object value = entry.getValue();
if (key == null) continue;
if (key.length() == 0) continue;
String setterName = "set" + Character.toUpperCase(key.charAt(0)) + key.substring(1);
Method setter = null;
// Try to use the exact setter
if (value != null) {
try {
if (includeSuperClasses) {
setter = objectClass.getMethod(setterName, value.getClass());
} else {
setter = objectClass.getDeclaredMethod(setterName, value.getClass());
}
} catch (Exception ex) {
}
}
// Find a more generic setter
if (setter == null) {
Method[] methods = includeSuperClasses ? objectClass.getMethods() : objectClass.getDeclaredMethods();
for (Method method : methods) {
if (method.getName().equals(setterName)) {
Class>[] parameterTypes = method.getParameterTypes();
if (parameterTypes.length == 1 && isAssignableFrom(parameterTypes[0], value)) {
setter = method;
break;
}
}
}
}
// Invoke
if (setter != null) {
if (setter.getAnnotation(Transient.class) != null) continue;
try {
setter.invoke(object, value);
} catch (Exception e) {
Log.e("BeanUtil", setterName + "() failed", e);
}
}
}
}
public static Map getProperties(Object object, boolean includeSuperClasses, boolean deepCopy) {
HashMap map = new HashMap();
if (object == null) {
return map;
}
Class> objectClass = object.getClass();
Method[] methods = includeSuperClasses ? objectClass.getMethods() : objectClass.getDeclaredMethods();
for (Method method : methods) {
if (method.getDeclaringClass() == java.lang.Object.class) continue;
if (method.getParameterTypes().length > 0) continue;
if (method.getReturnType().equals(Void.TYPE)) continue;
if (method.getAnnotation(Transient.class) != null) continue;
String methodName = method.getName();
String propertyName = "";
if (methodName.startsWith("get")) {
propertyName = methodName.substring(3);
} else if (methodName.startsWith("is")) {
propertyName = methodName.substring(2);
}
if (propertyName.length() > 0 && Character.isUpperCase(propertyName.charAt(0))) {
propertyName = Character.toLowerCase(propertyName.charAt(0)) + propertyName.substring(1);
Object value = null;
try {
value = method.invoke(object);
} catch (Exception e) {
Log.e("BeanUtil", method.getName() + "() failed", e);
}
if (!deepCopy) {
map.put(propertyName, value);
} else {
if (isSimpleObject(value)) {
map.put(propertyName, value);
} else if (value instanceof Map) {
Map submap = new HashMap();
for (Map.Entry, ?> entry : ((Map, ?>) value).entrySet()) {
submap.put(String.valueOf(entry.getKey()), convertObject(entry.getValue(), includeSuperClasses));
}
map.put(propertyName, submap);
} else if (value instanceof Iterable) {
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy