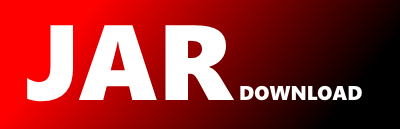
test.xyz.TestPointer Maven / Gradle / Ivy
/*===----------------------------* Java 6 *------------------------------===//
//
// THIS FILE IS GENERATED BY INVAR. DO NOT EDIT !!!
//
//===----------------------------------------------------------------------===*/
package test.xyz;
import invar.lib.CodecError;
import java.io.DataInput;
import java.io.DataInputStream;
import java.io.DataOutput;
import java.io.DataOutputStream;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.LinkedHashMap;
import java.util.LinkedList;
import java.util.Map;
import test.abc.Custom;
import test.abc.Gender;
/** 指针类型测试 */
public final class TestPointer
implements
invar.lib.InvarCodec.BinaryDecode,
invar.lib.InvarCodec.BinaryEncode,
invar.lib.InvarCodec.XMLEncode,
invar.lib.InvarCodec.JSONEncode
{
static public final long CRC32 = 0x6348C9B7L;
static public TestPointer Create()
{
return new TestPointer();
}
private TestPointer self ;
private String stringValue ;
private Custom other ;
private LinkedList listI08 ;
private LinkedHashMap dictI08 ;
private LinkedList>>> listNested ;
private Float numberSingle;
private Gender enumValue ;
private LinkedHashMap hotfix ;/* [AutoAdd] Hotfix */
public TestPointer()
{
self = null;
stringValue = null;
other = null;
listI08 = null;
dictI08 = null;
listNested = null;
numberSingle = 0.0F;
enumValue = Gender.NONE;
hotfix = null;
}
public TestPointer reuse()
{
if (self != null) {
self.reuse();
}
if (stringValue != null) {
stringValue = "";
}
if (other != null) {
other.reuse();
}
if (listI08 != null) {
listI08.clear();
}
if (dictI08 != null) {
dictI08.clear();
}
if (listNested != null) {
listNested.clear();
}
numberSingle = 0.0F;
enumValue = Gender.NONE;
if (hotfix != null) {
hotfix.clear();
}
return this;
}
/** */
@invar.lib.InvarRule(T="test.xyz.TestPointer", S="f0")
public TestPointer getSelf() { return self; }
/** */
@invar.lib.InvarRule(T="string", S="f1")
public String getStringValue() { return stringValue; }
/** */
@invar.lib.InvarRule(T="test.abc.Custom", S="f2")
public Custom getOther() { return other; }
/** */
@invar.lib.InvarRule(T="vec", S="f3")
public LinkedList getListI08() { return listI08; }
/** */
@invar.lib.InvarRule(T="map", S="f4")
public LinkedHashMap getDictI08() { return dictI08; }
/** */
@invar.lib.InvarRule(T="vec>>>", S="f5")
public LinkedList>>> getListNested() { return listNested; }
/** */
@invar.lib.InvarRule(T="float", S="f6")
public Float getNumberSingle() { return numberSingle; }
/** */
@invar.lib.InvarRule(T="test.abc.Gender", S="f7")
public Gender getEnumValue() { return enumValue; }
public Integer getEnumValueV() { return enumValue.value(); }
/** [AutoAdd] Hotfix */
@invar.lib.InvarRule(T="map", S="f8")
public LinkedHashMap getHotfix() { return hotfix; }
/** */
@invar.lib.InvarRule(T="test.xyz.TestPointer", S="f0")
public void setSelf(TestPointer value) { this.self = value; }
/** */
@invar.lib.InvarRule(T="string", S="f1")
public void setStringValue(String value) { this.stringValue = value; }
/** */
@invar.lib.InvarRule(T="test.abc.Custom", S="f2")
public void setOther(Custom value) { this.other = value; }
/** */
@invar.lib.InvarRule(T="vec", S="f3")
public void setListI08(LinkedList value) { this.listI08 = value; }
/** */
@invar.lib.InvarRule(T="map", S="f4")
public void setDictI08(LinkedHashMap value) { this.dictI08 = value; }
/** */
@invar.lib.InvarRule(T="vec>>>", S="f5")
public void setListNested(LinkedList>>> value) { this.listNested = value; }
/** */
@invar.lib.InvarRule(T="float", S="f6")
public void setNumberSingle(Float value) { this.numberSingle = value; }
/** */
@invar.lib.InvarRule(T="test.abc.Gender", S="f7")
public void setEnumValue(Gender value) { this.enumValue = value; }
public void setEnumValueV(Integer value) { this.enumValue = Gender.valueOf(value); }
/** [AutoAdd] Hotfix */
@invar.lib.InvarRule(T="map", S="f8")
public void setHotfix(LinkedHashMap value) { this.hotfix = value; }
/** Shallow copy */
public TestPointer copy(TestPointer from_)
{
if (this == from_ || from_ == null) {
return this;
}
if (from_.self != null) {
self.copy(from_.self);
} else {
self = null;
}
stringValue = from_.stringValue;
if (from_.other != null) {
other.copy(from_.other);
} else {
other = null;
}
if (null == from_.listI08) {
listI08 = null;
} else {
if (null == listI08) { listI08 = new LinkedList(); }
else { listI08.clear(); }
listI08.addAll(from_.listI08);
}
if (null == from_.dictI08) {
dictI08 = null;
} else {
if (null == dictI08) { dictI08 = new LinkedHashMap(); }
else { dictI08.clear(); }
dictI08.putAll(from_.dictI08);
}
if (null == from_.listNested) {
listNested = null;
} else {
if (null == listNested) { listNested = new LinkedList>>>(); }
else { listNested.clear(); }
listNested.addAll(from_.listNested);
}
numberSingle = from_.numberSingle;
enumValue = from_.enumValue;
if (null == from_.hotfix) {
hotfix = null;
} else {
if (null == hotfix) { hotfix = new LinkedHashMap(); }
else { hotfix.clear(); }
hotfix.putAll(from_.hotfix);
}
return this;
} /* copyFrom(...) */
public void read(InputStream from_) throws IOException, CodecError
{
this.read((DataInput)new DataInputStream(from_));
}
public void read(DataInput from_) throws IOException, CodecError
{
byte selfExists = from_.readByte();
if ((byte)0x01 == selfExists) {
if (self == null) { self = TestPointer.Create(); }
self.read(from_);
}
else if ((byte)0x00 == selfExists) { self = null; }
else { throw new CodecError(CodecError.ERR_DECODE_STRUCT_P); }
byte stringValueExists = from_.readByte();
if ((byte)0x01 == stringValueExists) {
stringValue = from_.readUTF();
}
else if ((byte)0x00 == stringValueExists) { stringValue = null; }
else { throw new CodecError(CodecError.ERR_DECODE_STRING_P); }
byte otherExists = from_.readByte();
if ((byte)0x01 == otherExists) {
if (other == null) { other = Custom.Create(); }
other.read(from_);
}
else if ((byte)0x00 == otherExists) { other = null; }
else { throw new CodecError(CodecError.ERR_DECODE_STRUCT_P); }
byte listI08Exists = from_.readByte();
if ((byte)0x01 == listI08Exists) {
if (listI08 == null) { listI08 = new LinkedList(); }
Long lenListI08 = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iListI08 = 0L; iListI08 < lenListI08; ++iListI08) {
java.lang.Byte n1 = from_.readByte();
listI08.add(n1);
}
}
else if ((byte)0x00 == listI08Exists) { listI08 = null; }
else { throw new CodecError(CodecError.ERR_DECODE_VEC_MAP_P); }
byte dictI08Exists = from_.readByte();
if ((byte)0x01 == dictI08Exists) {
if (dictI08 == null) { dictI08 = new LinkedHashMap(); }
Long lenDictI08 = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iDictI08 = 0L; iDictI08 < lenDictI08; ++iDictI08) {
java.lang.Byte k1 = from_.readByte();
java.lang.Byte v1 = from_.readByte();
dictI08.put(k1,v1);
}
}
else if ((byte)0x00 == dictI08Exists) { dictI08 = null; }
else { throw new CodecError(CodecError.ERR_DECODE_VEC_MAP_P); }
byte listNestedExists = from_.readByte();
if ((byte)0x01 == listNestedExists) {
if (listNested == null) { listNested = new LinkedList>>>(); }
Long lenListNested = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iListNested = 0L; iListNested < lenListNested; ++iListNested) {
LinkedList>> n1 = new LinkedList>>(); //read.vec.head
Long lenN1 = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iN1 = 0L; iN1 < lenN1; ++iN1) {
LinkedList> n2 = new LinkedList>(); //read.vec.head
Long lenN2 = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iN2 = 0L; iN2 < lenN2; ++iN2) {
LinkedList n3 = new LinkedList(); //read.vec.head
Long lenN3 = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iN3 = 0L; iN3 < lenN3; ++iN3) {
TestPointer n4 = TestPointer.Create();
n4.read(from_);
n3.add(n4);
}
n2.add(n3);
}
n1.add(n2);
}
listNested.add(n1);
}
}
else if ((byte)0x00 == listNestedExists) { listNested = null; }
else { throw new CodecError(CodecError.ERR_DECODE_VEC_MAP_P); }
numberSingle = from_.readFloat();
enumValue = Gender.valueOf(from_.readInt());
byte hotfixExists = from_.readByte();
if ((byte)0x01 == hotfixExists) {
if (hotfix == null) { hotfix = new LinkedHashMap(); }
Long lenHotfix = from_.readInt() & 0xFFFFFFFFL;
for (Long/*U32*/ iHotfix = 0L; iHotfix < lenHotfix; ++iHotfix) {
java.lang.String k1 = from_.readUTF();
java.lang.String v1 = from_.readUTF();
hotfix.put(k1,v1);
}
}
else if ((byte)0x00 == hotfixExists) { hotfix = null; }
else { throw new CodecError(CodecError.ERR_DECODE_VEC_MAP_P); }
}
public void write(OutputStream dest_) throws IOException
{
this.write((DataOutput)new DataOutputStream(dest_));
}
public void write(DataOutput dest_) throws IOException
{
if (self != null) {
dest_.writeByte((byte)0x01);
self.write(dest_);
} else {
dest_.writeByte((byte)0x00);
}
if (stringValue != null) {
dest_.writeByte((byte)0x01);
dest_.writeUTF(stringValue);
} else {
dest_.writeByte((byte)0x00);
}
if (other != null) {
dest_.writeByte((byte)0x01);
other.write(dest_);
} else {
dest_.writeByte((byte)0x00);
}
if (listI08 != null) {
dest_.writeByte((byte)0x01);
dest_.writeInt(listI08.size());
for (java.lang.Byte n1 : listI08) {
dest_.writeByte(n1);
}
} else {
dest_.writeByte((byte)0x00);
}
if (dictI08 != null) {
dest_.writeByte((byte)0x01);
dest_.writeInt(dictI08.size());
for (Map.Entry dictI08Iter : dictI08.entrySet()) {
java.lang.Byte k1 = dictI08Iter.getKey();
dest_.writeByte(k1);
java.lang.Byte v1 = dictI08Iter.getValue();
dest_.writeByte(v1);
}
} else {
dest_.writeByte((byte)0x00);
}
if (listNested != null) {
dest_.writeByte((byte)0x01);
dest_.writeInt(listNested.size());
for (LinkedList>> n1 : listNested) {
dest_.writeInt(n1.size());
for (LinkedList> n2 : n1) {
dest_.writeInt(n2.size());
for (LinkedList n3 : n2) {
dest_.writeInt(n3.size());
for (TestPointer n4 : n3) {
n4.write(dest_);
}
}
}
}
} else {
dest_.writeByte((byte)0x00);
}
dest_.writeFloat(numberSingle);
dest_.writeInt(enumValue.value());
if (hotfix != null) {
dest_.writeByte((byte)0x01);
dest_.writeInt(hotfix.size());
for (Map.Entry hotfixIter : hotfix.entrySet()) {
java.lang.String k1 = hotfixIter.getKey();
dest_.writeUTF(k1);
java.lang.String v1 = hotfixIter.getValue();
dest_.writeUTF(v1);
}
} else {
dest_.writeByte((byte)0x00);
}
}
public String toString()
{
StringBuilder s = new StringBuilder();
s.append('{');
s.append(getClass().getName());
s.append(", self:");
if (self != null) {
s.append('<').append("TestPointer").append('>');
} else {
s.append("null");
}
s.append(", stringValue:");
if (stringValue != null) {
s.append('"').append(stringValue).append('"');
} else {
s.append("null");
}
s.append(", other:");
if (other != null) {
s.append('<').append("Custom").append('>');
} else {
s.append("null");
}
s.append(", listI08:");
if (listI08 != null) {
s.append('(').append(listI08.size()).append(')');
} else {
s.append("null");
}
s.append(", dictI08:");
if (dictI08 != null) {
s.append('[').append(dictI08.size()).append(']');
} else {
s.append("null");
}
s.append(", listNested:");
if (listNested != null) {
s.append('(').append(listNested.size()).append(')');
} else {
s.append("null");
}
s.append(',').append("numberSingle").append(':');
s.append(numberSingle.toString());
s.append(',').append("enumValue").append(':');
s.append(enumValue.toString());
s.append(", hotfix:");
if (hotfix != null) {
s.append('[').append(hotfix.size()).append(']');
} else {
s.append("null");
}
s.append('}');
return s.toString();
} //TestPointer::toString ()
public String toStringJSON()
{
StringBuilder code = new StringBuilder();
this.writeJSON(code);
return code.toString();
}
public void writeJSON(StringBuilder s_)
{
s_.append('{');
char comma = '\0';
boolean selfExists = (null != self);
if (selfExists) {
s_.append('"').append("self").append('"').append(':'); comma = ','; self.writeJSON(s_);
}
boolean stringValueExists = stringValue != null && stringValue.length() > 0;
if ('\0' != comma && stringValueExists) { s_.append(comma); comma = '\0'; }
if (stringValueExists) {
s_.append('"').append("stringValue").append('"').append(':'); comma = ','; s_.append('"').append(stringValue.toString()).append('"');
}
boolean otherExists = (null != other);
if ('\0' != comma && otherExists) { s_.append(comma); comma = '\0'; }
if (otherExists) {
s_.append('"').append("other").append('"').append(':'); comma = ','; other.writeJSON(s_);
}
boolean listI08Exists = (null != listI08);
if ('\0' != comma && listI08Exists) { s_.append(comma); comma = '\0'; }
if (listI08Exists) {
s_.append('"').append("listI08").append('"').append(':'); comma = ',';
if (null != listI08) {
s_.append('[');
int listI08Size = listI08.size();
int listI08Idx = 0;
for (java.lang.Byte n1 : listI08) { /* vec.for: listI08 */
++listI08Idx;
s_.append(n1.toString());
if (listI08Idx != listI08Size) { s_.append(','); }
}
s_.append(']');
}
}
boolean dictI08Exists = (null != dictI08);
if ('\0' != comma && dictI08Exists) { s_.append(comma); comma = '\0'; }
if (dictI08Exists) {
s_.append('"').append("dictI08").append('"').append(':'); comma = ',';
if (null != dictI08) {
s_.append('{');
int dictI08Size = dictI08.size();
int dictI08Idx = 0;
for (Map.Entry dictI08Iter : dictI08.entrySet()) { /* map.for: dictI08 */
++dictI08Idx;
java.lang.Byte k1 = dictI08Iter.getKey(); /* nest.k */
s_.append('"'); s_.append(k1.toString()); s_.append('"').append(':');
java.lang.Byte v1 = dictI08Iter.getValue(); /* nest.v */
s_.append(v1.toString());
if (dictI08Idx != dictI08Size) { s_.append(','); }
}
s_.append('}');
}
}
boolean listNestedExists = (null != listNested);
if ('\0' != comma && listNestedExists) { s_.append(comma); comma = '\0'; }
if (listNestedExists) {
s_.append('"').append("listNested").append('"').append(':'); comma = ',';
if (null != listNested) {
s_.append('[');
int listNestedSize = listNested.size();
int listNestedIdx = 0;
for (LinkedList>> n1 : listNested) { /* vec.for: listNested */
++listNestedIdx;
if (null != n1) {
s_.append('[');
int n1Size = n1.size();
int n1Idx = 0;
for (LinkedList> n2 : n1) { /* vec.for: n1 */
++n1Idx;
if (null != n2) {
s_.append('[');
int n2Size = n2.size();
int n2Idx = 0;
for (LinkedList n3 : n2) { /* vec.for: n2 */
++n2Idx;
if (null != n3) {
s_.append('[');
int n3Size = n3.size();
int n3Idx = 0;
for (TestPointer n4 : n3) { /* vec.for: n3 */
++n3Idx;
n4.writeJSON(s_);
if (n3Idx != n3Size) { s_.append(','); }
}
s_.append(']');
}
if (n2Idx != n2Size) { s_.append(','); }
}
s_.append(']');
}
if (n1Idx != n1Size) { s_.append(','); }
}
s_.append(']');
}
if (listNestedIdx != listNestedSize) { s_.append(','); }
}
s_.append(']');
}
}
if ('\0' != comma) { s_.append(comma); comma = '\0'; }
s_.append('"').append("numberSingle").append('"').append(':');
s_.append(numberSingle.toString()); comma = ',';
if ('\0' != comma) { s_.append(comma); comma = '\0'; }
s_.append('"').append("enumValue").append('"').append(':');
s_.append(enumValue.value()); comma = ',';
boolean hotfixExists = (null != hotfix);
if ('\0' != comma && hotfixExists) { s_.append(comma); comma = '\0'; }
if (hotfixExists) {
s_.append('"').append("hotfix").append('"').append(':'); comma = ',';
if (null != hotfix) {
s_.append('{');
int hotfixSize = hotfix.size();
int hotfixIdx = 0;
for (Map.Entry hotfixIter : hotfix.entrySet()) { /* map.for: hotfix */
++hotfixIdx;
java.lang.String k1 = hotfixIter.getKey(); /* nest.k */
s_.append('"').append(k1.toString()).append('"'); s_.append(':');
java.lang.String v1 = hotfixIter.getValue(); /* nest.v */
s_.append('"').append(v1.toString()).append('"');
if (hotfixIdx != hotfixSize) { s_.append(','); }
}
s_.append('}');
}
}
s_.append('}');
} /* TestPointer::writeJSON(...) */
public String toStringXML()
{
StringBuilder code = new StringBuilder();
this.writeXML(code, "TestPointer");
return code.toString();
}
public void writeXML(StringBuilder result_, String name_)
{
StringBuilder attrs = new StringBuilder();
StringBuilder nodes = new StringBuilder();
if (self != null) {
self.writeXML(nodes, "self");
}
if (stringValue != null) {
attrs.append(' ').append("stringValue").append('=').append('"');
attrs.append(stringValue).append('"');
}
if (other != null) {
other.writeXML(nodes, "other");
}
if (listI08 != null && listI08.size() > 0) {
nodes.append('<').append("listI08").append('>');
for (java.lang.Byte n1 : listI08) {
nodes.append('<').append("n1").append(' ').append("value").append('=').append('"');
nodes.append(n1.toString()).append('"').append('/').append('>');
}
nodes.append('<').append('/').append("listI08").append('>');
}
if (dictI08 != null && dictI08.size() > 0) {
nodes.append('<').append("dictI08").append('>');
for (Map.Entry dictI08Iter : dictI08.entrySet()) {
java.lang.Byte k1 = dictI08Iter.getKey();
nodes.append('<').append("k1").append(' ').append("value").append('=').append('"');
nodes.append(k1.toString()).append('"').append('/').append('>');
java.lang.Byte v1 = dictI08Iter.getValue();
nodes.append('<').append("v1").append(' ').append("value").append('=').append('"');
nodes.append(v1.toString()).append('"').append('/').append('>');
}
nodes.append('<').append('/').append("dictI08").append('>');
}
if (listNested != null && listNested.size() > 0) {
nodes.append('<').append("listNested").append('>');
for (LinkedList>> n1 : listNested) {
nodes.append('<').append("n1").append('>');
for (LinkedList> n2 : n1) {
nodes.append('<').append("n2").append('>');
for (LinkedList n3 : n2) {
nodes.append('<').append("n3").append('>');
for (TestPointer n4 : n3) {
n4.writeXML(nodes, "n4");
}
nodes.append('<').append('/').append("n3").append('>');
}
nodes.append('<').append('/').append("n2").append('>');
}
nodes.append('<').append('/').append("n1").append('>');
}
nodes.append('<').append('/').append("listNested").append('>');
}
attrs.append(' ').append("numberSingle").append('=').append('"');
attrs.append(numberSingle.toString()).append('"');
attrs.append(' ').append("enumValue").append('=').append('"');
attrs.append(enumValue.value()).append('"');
if (hotfix != null && hotfix.size() > 0) {
nodes.append('<').append("hotfix").append('>');
for (Map.Entry hotfixIter : hotfix.entrySet()) {
java.lang.String k1 = hotfixIter.getKey();
nodes.append('<').append("k1").append(' ').append("value").append('=').append('"');
nodes.append(k1).append('"').append('/').append('>');
java.lang.String v1 = hotfixIter.getValue();
nodes.append('<').append("v1").append(' ').append("value").append('=').append('"');
nodes.append(v1).append('"').append('/').append('>');
}
nodes.append('<').append('/').append("hotfix").append('>');
}
result_.append('<').append(name_).append(attrs);
if (nodes.length() == 0) {
result_.append('/').append('>');
} else {
result_.append('>').append(nodes);
result_.append('<').append('/').append(name_).append('>');
}
} /* TestPointer::writeXML(...) */
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy