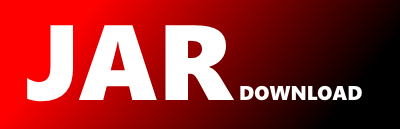
com.studerw.tda.client.TdaClient Maven / Gradle / Ivy
package com.studerw.tda.client;
import com.studerw.tda.model.account.Order;
import com.studerw.tda.model.account.OrderRequest;
import com.studerw.tda.model.account.SecuritiesAccount;
import com.studerw.tda.model.history.PriceHistReq;
import com.studerw.tda.model.history.PriceHistory;
import com.studerw.tda.model.instrument.FullInstrument;
import com.studerw.tda.model.instrument.Instrument;
import com.studerw.tda.model.instrument.Query;
import com.studerw.tda.model.marketdata.Mover;
import com.studerw.tda.model.marketdata.MoversReq;
import com.studerw.tda.model.option.OptionChain;
import com.studerw.tda.model.quote.Quote;
import java.util.List;
/**
* Main interface of the TDA Client. Implementations should be thread safe.
*
* @see HttpTdaClient
*/
public interface TdaClient {
/**
*
* Retrieve historical intraday and end of day quote data for an equity, index, mutual fund,
* forex, option chain, etc. See {@link com.studerw.tda.model.AssetType} for possible types,
* though your account must explicitly have access for some of these. TDA has not implemented all
* the API calls either (Sep 2019).
*
*
*
* - periodType: day
* - period: 10
* - frequencyType: minute
* - frequency: 1
*
*
* @param symbol uppercase symbol
* @return PriceHistory using all other TDA default request parameters. This appears to be a quote
* every minute for 10 days.
**/
PriceHistory priceHistory(String symbol);
/**
*
* Retrieve historical intraday and end of day quote data for an equity, index, mutual fund,
* forex, option chain, etc. See {@link com.studerw.tda.model.AssetType} for possibly types,
* though your account must explicitly have access for some of these. TDA has not implemented all
* the API calls either (Sep 2019). Note that some of the parameters within the {@link
* com.studerw.tda.model.history.PriceHistReq} param be null, and then some of the other arguments
* will be assumed by the non null parameters.
*
*
* @param priceHistReq validated object of request parameters
* @return PriceHistory with a list of {@link com.studerw.tda.model.history.Candle} Candles based
* on the frequency and period / date length.
*/
PriceHistory priceHistory(PriceHistReq priceHistReq);
/**
*
* Fetch detailed quote information for one or more symbols. Currently the API allows symbol types
* of Stocks, Options, Mutual Funds Indexes, and ETFs. Quotes are real-time for accounts
* subscribed to this service; otherwise, quotes are delayed according to exchange and TDA rules.
* The following types of Quote are actually returned and can be casted:
*
*
*
* - {@link com.studerw.tda.model.quote.EquityQuote}
* - {@link com.studerw.tda.model.quote.EtfQuote}
* - {@link com.studerw.tda.model.quote.OptionQuote}
* - {@link com.studerw.tda.model.quote.IndexQuote}
* - {@link com.studerw.tda.model.quote.MutualFundQuote}
* - {@link com.studerw.tda.model.quote.ForexQuote}
*
*
*
* Quote quote = client.fetchQuote("ATD");
* EquityQuote equityQuote = (EquityQuote)quote;
*
*
* @param symbols list of valid symbols. Max of 300 based on TDA docs. Index symbols need to be
* prefixed with a $, e.g. $INX or $SPX.X. Options are in a format like
* the following:
* MSFT_061518P60 for a put, or MSFT_061518C60 for a call. This is the
* Microsoft June 6, 2018 Put/Call at $60.
* @return list of quotes. The {@link Quote} is the base class, but all objects in the list can be
* cast to their actual types by looking at the {@link com.studerw.tda.model.AssetType} attribute.
* field.
*/
List fetchQuotes(List symbols);
/**
*
* Fetch Detailed quote information for one or more symbols. Currently the API allows symbol types
* of Stocks, Options, Mutual Funds and Indexes, and ETFs. Quotes are real-time for accounts
* subscribed to this service; otherwise, quotes are delayed according to exchange and TDA rules.
*
*
*
* Quote quote = client.fetchQuote("ATD");
* EquityQuote equityQuote = (EquityQuote)quote;
*
*
*
* - {@link com.studerw.tda.model.quote.EquityQuote}
* - {@link com.studerw.tda.model.quote.EtfQuote}
* - {@link com.studerw.tda.model.quote.OptionQuote}
* - {@link com.studerw.tda.model.quote.IndexQuote}
* - {@link com.studerw.tda.model.quote.MutualFundQuote}
* - {@link com.studerw.tda.model.quote.ForexQuote}
*
*
* @param symbol list of valid symbols. Max of 300 based on TDA docs. Index symbols need to be
* prefixed with a $, e.g. $INX or $SPX.X. Options are in a format like
* the following:
* MSFT_061518P60 for a put, or MSFT_061518C60 for a call. This is the
* Microsoft June 6, 2018 Put/Call at $60.
* @return a quote. The {@link Quote} is the base class, but all quotes can be cast to their
* actual types by looking at the {@code com.studerw.tda.model.quote.Quote.assetType} field.
*/
Quote fetchQuote(String symbol);
/**
* Fetch an account by the id. By default, balances are included. Positions and Orders can also be
* included based on the parameters.
*
* @param accountId the account. Most users only have a single account
* @param positions whether to include positions
* @param orders whether to include orders
* @return {@link SecuritiesAccount} with the passed id.
*/
SecuritiesAccount getAccount(String accountId, boolean positions, boolean orders);
/**
* Fetch all your accounts. By default, balances are included. Positions and Orders can also be
* included based on the parameters.
*
* @param positions whether to include positions
* @param orders whether to include orders
* @return List of all the user's {@link SecuritiesAccount}.
*/
List getAccounts(boolean positions, boolean orders);
/**
* Partially complete....
* @param symbol in TDA format (e.g. {@code MSFT_061821C120} which denotes MSFT for June 18, 2021 Call at $20.
* @return {@link com.studerw.tda.model.OptionChain}
*/
// OptionChain fetchOptionChain(String symbol);
/**
* A snapshot of the five main indices.
* @return {@link MarketSnapshot} MarketSnapshot
*/
// MarketSnapshot fetchMarketSnapshot();
/**
* This is just an alias method for {@link TdaClient#fetchMarketOverview()} due to the way TDA names their services.
* @return {@link MarketSnapshot} MarketSnapshot
*/
// MarketSnapshot fetchMarketOverview();
/**
* Place an Order.
*
* @param accountId the account under which the order is to be placed
* @param order the order to place
* @see Place Order
* Samples
*/
void placeOrder(String accountId, Order order);
/**
*
* Fetch all orders for a given account using the criteria of the orderRequest. You can just use a
* blank order to use sane defaults.
*
*
*
* Order order = client.fetchOrders("123456789", new OrderRequest());
*
*
* @param accountId the orders from only this account
* @param orderRequest the request.
* @return list of orders specified by the {@link OrderRequest} param.
*/
List fetchOrders(String accountId, OrderRequest orderRequest);
/**
*
* Fetch all orders for all accounts using the criteria of the orderRequest. You can use a blank
* order to use sane defaults.
*
*
*
* Order order = client.fetchOrders(new OrderRequest());
*
*
* @param orderRequest the request.
* @return list of orders specified by the {@link OrderRequest} param.
*/
List fetchOrders(OrderRequest orderRequest);
/**
* Fetch all orders for all accounts using TDA defaults.
*
* @return list of orders for all accounts, using TDA default criteria.
*/
List fetchOrders();
/**
* This call assumes the order under the given parameters definitely exists. If not, a {@link
* RuntimeException} is thrown as, behind the scenes, the TDA API will return a 404 response.
*
* @param accountId account under which the order was originally placed
* @param orderId the id of the order
* @return Order or a RuntimeException if it doesn't exist.
*/
Order fetchOrder(String accountId, Long orderId);
/**
* Cancel an order by account Id and order Id.
*
* @param accountId the account under which this order was placed
* @param orderId the order to cancel
*/
void cancelOrder(String accountId, String orderId);
/**
* Get basic data for an instrument via its CUSIP number. Data includes attributes such as
* description, possible symbol, exchange, etc.
*
*
* Apparently the following instrument types are able to be looked up: {@link
* com.studerw.tda.model.instrument.Instrument.AssetType}.
*
*
* @param cusip (committee on uniform securities identification procedures numbers).
* @return Basic data using a security's CUSIP. The {@link Instrument#getBondPrice()} will return
* null unless the {@code cusip} parameter references a bond.
* @see CUSIP Number
*/
Instrument getInstrumentByCUSIP(String cusip);
/**
* Get basic info for a bond via its CUSIP number.
*
* @param cusip (committee on uniform securities identification procedures numbers).
* @return Basic data of the bond including the price.
* @see CUSIP Number
*/
Instrument getBond(String cusip);
/**
*
* Query TDA for {@link com.studerw.tda.model.instrument.Instrument Instruments} using symbol,
* name, description, cusip, etc. Apparently the following instrument types are queryable: {@link
* com.studerw.tda.model.instrument.Instrument.AssetType}.
*
*
*
* The following {@link com.studerw.tda.model.instrument.Query.QueryType QueryTypes} can be made:
*
*
*
* - SYMBOL_SEARCH: retrieve an instrument using the exact symbol name or CUSIP
* - SYMBOL_REGEX: Retrieve instrument data for all symbols matching regex. For example XYZ.* will return all symbols beginning with XYZ
* - DESCRIPTION_SEARCH: Retrieve instrument data for instruments whose description contains the word supplied. Example: Bank will return all instruments with Bank in the description.
* - DESCRIPTION_REGEX: Search description with full regex support.
* For example XYZ.[A-C] returns all instruments whose descriptions contain a word beginning with XYZ followed by a character A through C.
*
*
* @param query contains the type of query and the search string.
* @return List of 0 or more instruments that matched the query. If you want the full data of an
* instrument, you must use {@link #getFundamentalData} using the exact CUSIP or symbol.
* Note that {@link com.studerw.tda.model.instrument.Instrument#getBondPrice} will return null for
* any instruments not of type BOND.
* @see com.studerw.tda.model.instrument.Query.QueryType
* @see #getFundamentalData
*/
List queryInstruments(Query query);
/**
* Get full fundamental data for a specific security via its CUSIP number or ticker symbol (e.g.
* MSFT). Not all CUSIP numbers work, for example those referencing BONDs, at least as
* of Nov. 2019.
*
* @param id CUSIP number or symbol (e.g. MSFT)
* @return full fundamentals of an instrument using a CUSIP or symbol. The {@link
* Instrument#getBondPrice()} will be empty unless it is a bond referenced by {@code cusip}.
* @see CUSIP Number
*/
FullInstrument getFundamentalData(String id);
/** Note that this call can return an empty list on days the market is closed.
* Top 10 (up or down) movers by value or percent for a particular market
*
* @param moversReq Index must be set, the other fields can be null which will use TDA defaults.
* @return List of top 10 market movers defined by the request.
*/
List fetchMovers(MoversReq moversReq);
/**
*
* @param symbol - should be upper case (e.g. MSFT)
* @return an option chain using all TDA Default parameters
*/
OptionChain getOptionChain(String symbol);
}