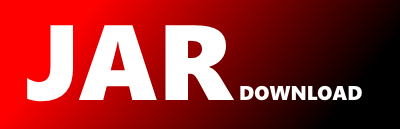
com.studerw.tda.parse.TdaJsonParser Maven / Gradle / Ivy
package com.studerw.tda.parse;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.DeserializationFeature;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.studerw.tda.model.account.Order;
import com.studerw.tda.model.account.SecuritiesAccount;
import com.studerw.tda.model.history.PriceHistory;
import com.studerw.tda.model.instrument.FullInstrument;
import com.studerw.tda.model.instrument.Instrument;
import com.studerw.tda.model.marketdata.Mover;
import com.studerw.tda.model.option.OptionChain;
import com.studerw.tda.model.quote.Quote;
import java.io.BufferedInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class TdaJsonParser {
private static final Logger LOGGER = LoggerFactory.getLogger(TdaJsonParser.class);
/**
* @param in inputstream of JSON from rest call to TDA. The stream will be closed upon return.
* @return list of objects that extend Quote.
*/
public List parseQuotes(InputStream in) {
LOGGER.trace("parsing quotes...");
try (BufferedInputStream bIn = new BufferedInputStream(in)) {
LinkedHashMap quotesMap = DefaultMapper.fromJson(bIn, new TypeReference>() {});
LOGGER.debug("returned a map of size: {}", quotesMap.size());
List quotes = new ArrayList<>();
quotesMap.forEach((k, v) -> quotes.add(v));
return quotes;
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
/**
* @param in {@link InputStream} of JSON from TDA; the stream will be closed upon return.
* @return PriceHistory
*/
public PriceHistory parsePriceHistory(InputStream in) {
LOGGER.trace("parsing quotes...");
try (BufferedInputStream bIn = new BufferedInputStream(in)) {
final PriceHistory priceHistory = DefaultMapper.fromJson(bIn, PriceHistory.class);
LOGGER.debug("returned a price history for {} of size: {}", priceHistory.getSymbol(),
priceHistory.getCandles().size());
return priceHistory;
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
/**
* @param in {@link InputStream} of JSON from TDA; the stream will be closed upon return.
* @return SecuritiesAccount
*/
public SecuritiesAccount parseAccount(InputStream in) {
LOGGER.trace("parsing securitiesAccount...");
try (BufferedInputStream bIn = new BufferedInputStream(in)) {
//Cannot use defaultMapper because account is wrapped in '{ securitiesAccount: {...} }'
final ObjectMapper objMapper = new ObjectMapper();
objMapper.enable(DeserializationFeature.UNWRAP_ROOT_VALUE);
final SecuritiesAccount securitiesAccount = objMapper.readValue(bIn, SecuritiesAccount.class);
LOGGER.debug("returned a securitiesAccount of type: {}",
securitiesAccount.getClass().getName());
return securitiesAccount;
} catch (IOException e) {
e.printStackTrace();
throw new RuntimeException(e);
}
}
/**
* @param in {@link InputStream} of JSON from TDA; the stream will be closed upon return.
* @return List of SecuritiesAccounts
*/
public List parseAccounts(InputStream in) {
LOGGER.trace("parsing securitiesAccounts...");
try (BufferedInputStream bIn = new BufferedInputStream(in)) {
TypeReference>> typeReference = new TypeReference>>() {
};
ObjectMapper mapper = new ObjectMapper();
List accounts = new ArrayList<>();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy