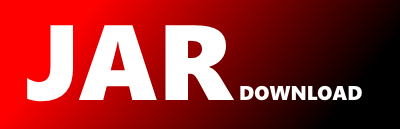
com.styra.opa.openapi.OpaApiClient Maven / Gradle / Ivy
/*
* Code generated by Speakeasy (https://speakeasyapi.dev). DO NOT EDIT.
*/
package com.styra.opa.openapi;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.styra.opa.openapi.models.errors.SDKError;
import com.styra.opa.openapi.models.operations.SDKMethodInterfaces.*;
import com.styra.opa.openapi.utils.HTTPClient;
import com.styra.opa.openapi.utils.HTTPRequest;
import com.styra.opa.openapi.utils.Hook.AfterErrorContextImpl;
import com.styra.opa.openapi.utils.Hook.AfterSuccessContextImpl;
import com.styra.opa.openapi.utils.Hook.BeforeRequestContextImpl;
import com.styra.opa.openapi.utils.JSON;
import com.styra.opa.openapi.utils.Retries.NonRetryableException;
import com.styra.opa.openapi.utils.RetryConfig;
import com.styra.opa.openapi.utils.SerializedBody;
import com.styra.opa.openapi.utils.SpeakeasyHTTPClient;
import com.styra.opa.openapi.utils.Utils;
import java.io.InputStream;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.net.http.HttpRequest;
import java.net.http.HttpResponse;
import java.nio.charset.StandardCharsets;
import java.time.LocalDate;
import java.time.OffsetDateTime;
import java.util.Optional;
import java.util.function.Function;
import java.util.stream.Collectors;
import org.apache.http.NameValuePair;
import org.openapitools.jackson.nullable.JsonNullable;
/**
* https://docs.styra.com/enterprise-opa - Enterprise OPA documentation
*/
public class OpaApiClient implements
MethodCallExecuteDefaultPolicyWithInput,
MethodCallExecutePolicy,
MethodCallExecutePolicyWithInput,
MethodCallExecuteBatchPolicyWithInput,
MethodCallHealth {
/**
* SERVERS contains the list of server urls available to the SDK.
*/
public static final String[] SERVERS = {
/**
* Local development server
*/
"http://localhost:8181",
};
private final SDKConfiguration sdkConfiguration;
/**
* The Builder class allows the configuration of a new instance of the SDK.
*/
public static class Builder {
private final SDKConfiguration sdkConfiguration = new SDKConfiguration();
private Builder() {
}
/**
* Allows the default HTTP client to be overridden with a custom implementation.
*
* @param client The HTTP client to use for all requests.
* @return The builder instance.
*/
public Builder client(HTTPClient client) {
this.sdkConfiguration.defaultClient = client;
return this;
}
/**
* Overrides the default server URL.
*
* @param serverUrl The server URL to use for all requests.
* @return The builder instance.
*/
public Builder serverURL(String serverUrl) {
this.sdkConfiguration.serverUrl = serverUrl;
return this;
}
/**
* Overrides the default server URL with a templated URL populated with the provided parameters.
*
* @param serverUrl The server URL to use for all requests.
* @param params The parameters to use when templating the URL.
* @return The builder instance.
*/
public Builder serverURL(String serverUrl, java.util.Map params) {
this.sdkConfiguration.serverUrl = com.styra.opa.openapi.utils.Utils.templateUrl(serverUrl, params);
return this;
}
/**
* Overrides the default server by index.
*
* @param serverIdx The server to use for all requests.
* @return The builder instance.
*/
public Builder serverIndex(int serverIdx) {
this.sdkConfiguration.serverIdx = serverIdx;
this.sdkConfiguration.serverUrl = SERVERS[serverIdx];
return this;
}
/**
* Overrides the default configuration for retries
*
* @param retryConfig The retry configuration to use for all requests.
* @return The builder instance.
*/
public Builder retryConfig(RetryConfig retryConfig) {
this.sdkConfiguration.retryConfig = Optional.of(retryConfig);
return this;
}
// Visible for testing, will be accessed via reflection
void _hooks(com.styra.opa.openapi.utils.Hooks hooks) {
sdkConfiguration.setHooks(hooks);
}
/**
* Builds a new instance of the SDK.
* @return The SDK instance.
*/
public OpaApiClient build() {
if (sdkConfiguration.defaultClient == null) {
sdkConfiguration.defaultClient = new SpeakeasyHTTPClient();
}
if (sdkConfiguration.serverUrl == null || sdkConfiguration.serverUrl.isBlank()) {
sdkConfiguration.serverUrl = SERVERS[0];
sdkConfiguration.serverIdx = 0;
}
if (sdkConfiguration.serverUrl.endsWith("/")) {
sdkConfiguration.serverUrl = sdkConfiguration.serverUrl.substring(0, sdkConfiguration.serverUrl.length() - 1);
}
return new OpaApiClient(sdkConfiguration);
}
}
/**
* Get a new instance of the SDK builder to configure a new instance of the SDK.
* @return The SDK builder instance.
*/
public static Builder builder() {
return new Builder();
}
private OpaApiClient(SDKConfiguration sdkConfiguration) {
this.sdkConfiguration = sdkConfiguration;
this.sdkConfiguration.initialize();
}
/**
* Execute the default decision given an input
* @return The call builder
*/
public com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputRequestBuilder executeDefaultPolicyWithInput() {
return new com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputRequestBuilder(this);
}
/**
* Execute the default decision given an input
* @param input Arbitrary JSON used within your policies by accessing `input`
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputResponse executeDefaultPolicyWithInput(
com.styra.opa.openapi.models.shared.Input input) throws Exception {
return executeDefaultPolicyWithInput(Optional.empty(), Optional.empty(), input);
}
/**
* Execute the default decision given an input
* @param pretty If parameter is `true`, response will formatted for humans.
* @param acceptEncoding
* @param input Arbitrary JSON used within your policies by accessing `input`
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputResponse executeDefaultPolicyWithInput(
Optional extends Boolean> pretty,
Optional extends com.styra.opa.openapi.models.shared.GzipAcceptEncoding> acceptEncoding,
com.styra.opa.openapi.models.shared.Input input) throws Exception {
com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputRequest request =
com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputRequest
.builder()
.pretty(pretty)
.acceptEncoding(acceptEncoding)
.input(input)
.build();
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
_baseUrl,
"/");
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(request, Utils.JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest, "input", "json", false);
if (_serializedRequestBody == null) {
throw new Exception("Request body is required");
}
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
_req.addQueryParams(Utils.getQueryParams(
com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputRequest.class,
request,
null));
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("executeDefaultPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "404", "4XX", "500", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("executeDefaultPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("executeDefaultPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("executeDefaultPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputResponse.Builder _resBuilder =
com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.styra.opa.openapi.models.operations.ExecuteDefaultPolicyWithInputResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
_res.withHeaders(_httpRes.headers().map());
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.shared.Result _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withResult(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "404")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ClientError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "500")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ServerError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Execute a policy
* @return The call builder
*/
public com.styra.opa.openapi.models.operations.ExecutePolicyRequestBuilder executePolicy() {
return new com.styra.opa.openapi.models.operations.ExecutePolicyRequestBuilder(this);
}
/**
* Execute a policy
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.ExecutePolicyResponse executePolicy(
com.styra.opa.openapi.models.operations.ExecutePolicyRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
com.styra.opa.openapi.models.operations.ExecutePolicyRequest.class,
_baseUrl,
"/v1/data/{path}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
_req.addQueryParams(Utils.getQueryParams(
com.styra.opa.openapi.models.operations.ExecutePolicyRequest.class,
request,
null));
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("executePolicy", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "4XX", "500", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("executePolicy", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("executePolicy", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("executePolicy", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.styra.opa.openapi.models.operations.ExecutePolicyResponse.Builder _resBuilder =
com.styra.opa.openapi.models.operations.ExecutePolicyResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.styra.opa.openapi.models.operations.ExecutePolicyResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
_res.withHeaders(_httpRes.headers().map());
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.shared.SuccessfulPolicyResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withSuccessfulPolicyResponse(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ClientError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "500")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ServerError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Execute a policy given an input
* @return The call builder
*/
public com.styra.opa.openapi.models.operations.ExecutePolicyWithInputRequestBuilder executePolicyWithInput() {
return new com.styra.opa.openapi.models.operations.ExecutePolicyWithInputRequestBuilder(this);
}
/**
* Execute a policy given an input
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.ExecutePolicyWithInputResponse executePolicyWithInput(
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputRequest.class,
_baseUrl,
"/v1/data/{path}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(request, Utils.JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest, "requestBody", "json", false);
if (_serializedRequestBody == null) {
throw new Exception("Request body is required");
}
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
_req.addQueryParams(Utils.getQueryParams(
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputRequest.class,
request,
null));
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("executePolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "4XX", "500", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("executePolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("executePolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("executePolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputResponse.Builder _resBuilder =
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
_res.withHeaders(_httpRes.headers().map());
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.shared.SuccessfulPolicyResponse _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withSuccessfulPolicyResponse(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ClientError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "500")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ServerError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Execute a policy given a batch of inputs
* @return The call builder
*/
public com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputRequestBuilder executeBatchPolicyWithInput() {
return new com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputRequestBuilder(this);
}
/**
* Execute a policy given a batch of inputs
* @param request The request object containing all of the parameters for the API call.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputResponse executeBatchPolicyWithInput(
com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputRequest request) throws Exception {
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputRequest.class,
_baseUrl,
"/v1/batch/data/{path}",
request, null);
HTTPRequest _req = new HTTPRequest(_url, "POST");
Object _convertedRequest = Utils.convertToShape(request, Utils.JsonShape.DEFAULT,
new TypeReference() {});
SerializedBody _serializedRequestBody = Utils.serializeRequestBody(
_convertedRequest, "requestBody", "json", false);
if (_serializedRequestBody == null) {
throw new Exception("Request body is required");
}
_req.setBody(Optional.ofNullable(_serializedRequestBody));
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
_req.addQueryParams(Utils.getQueryParams(
com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputRequest.class,
request,
null));
_req.addHeaders(Utils.getHeadersFromMetadata(request, null));
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("executeBatchPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400", "4XX", "500", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("executeBatchPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("executeBatchPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("executeBatchPolicyWithInput", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputResponse.Builder _resBuilder =
com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.styra.opa.openapi.models.operations.ExecuteBatchPolicyWithInputResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
_res.withHeaders(_httpRes.headers().map());
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.shared.BatchSuccessfulPolicyEvaluation _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withBatchSuccessfulPolicyEvaluation(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "207")) {
_res.withHeaders(_httpRes.headers().map());
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.shared.BatchMixedResults _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withBatchMixedResults(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "400")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.ClientError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "500")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.BatchServerError _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
/**
* Verify the server is operational
* The health API endpoint executes a simple built-in policy query to verify that the server is operational. Optionally it can account for bundle activation as well (useful for “ready” checks at startup).
* @return The call builder
*/
public com.styra.opa.openapi.models.operations.HealthRequestBuilder health() {
return new com.styra.opa.openapi.models.operations.HealthRequestBuilder(this);
}
/**
* Verify the server is operational
* The health API endpoint executes a simple built-in policy query to verify that the server is operational. Optionally it can account for bundle activation as well (useful for “ready” checks at startup).
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.HealthResponse healthDirect() throws Exception {
return health(Optional.empty(), Optional.empty(), Optional.empty());
}
/**
* Verify the server is operational
* The health API endpoint executes a simple built-in policy query to verify that the server is operational. Optionally it can account for bundle activation as well (useful for “ready” checks at startup).
* @param bundles Boolean parameter to account for bundle activation status in response. This includes any discovery bundles or bundles defined in the loaded discovery configuration.
* @param plugins Boolean parameter to account for plugin status in response.
* @param excludePlugin String parameter to exclude a plugin from status checks. Can be added multiple times. Does nothing if plugins is not true. This parameter is useful for special use cases where a plugin depends on the server being fully initialized before it can fully initialize itself.
* @return The response from the API call
* @throws Exception if the API call fails
*/
public com.styra.opa.openapi.models.operations.HealthResponse health(
Optional extends Boolean> bundles,
Optional extends Boolean> plugins,
Optional extends java.util.List> excludePlugin) throws Exception {
com.styra.opa.openapi.models.operations.HealthRequest request =
com.styra.opa.openapi.models.operations.HealthRequest
.builder()
.bundles(bundles)
.plugins(plugins)
.excludePlugin(excludePlugin)
.build();
String _baseUrl = this.sdkConfiguration.serverUrl;
String _url = Utils.generateURL(
_baseUrl,
"/health");
HTTPRequest _req = new HTTPRequest(_url, "GET");
_req.addHeader("Accept", "application/json")
.addHeader("user-agent",
this.sdkConfiguration.userAgent);
_req.addQueryParams(Utils.getQueryParams(
com.styra.opa.openapi.models.operations.HealthRequest.class,
request,
null));
HTTPClient _client = this.sdkConfiguration.defaultClient;
HttpRequest _r =
sdkConfiguration.hooks()
.beforeRequest(
new BeforeRequestContextImpl("health", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_req.build());
HttpResponse _httpRes;
try {
_httpRes = _client.send(_r);
if (Utils.statusCodeMatches(_httpRes.statusCode(), "4XX", "500", "5XX")) {
_httpRes = sdkConfiguration.hooks()
.afterError(
new AfterErrorContextImpl("health", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.of(_httpRes),
Optional.empty());
} else {
_httpRes = sdkConfiguration.hooks()
.afterSuccess(
new AfterSuccessContextImpl("health", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
_httpRes);
}
} catch (Exception _e) {
_httpRes = sdkConfiguration.hooks()
.afterError(new AfterErrorContextImpl("health", Optional.of(java.util.List.of()), sdkConfiguration.securitySource()),
Optional.empty(),
Optional.of(_e));
}
String _contentType = _httpRes
.headers()
.firstValue("Content-Type")
.orElse("application/octet-stream");
com.styra.opa.openapi.models.operations.HealthResponse.Builder _resBuilder =
com.styra.opa.openapi.models.operations.HealthResponse
.builder()
.contentType(_contentType)
.statusCode(_httpRes.statusCode())
.rawResponse(_httpRes);
com.styra.opa.openapi.models.operations.HealthResponse _res = _resBuilder.build();
if (Utils.statusCodeMatches(_httpRes.statusCode(), "200")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.shared.HealthyServer _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
_res.withHealthyServer(java.util.Optional.ofNullable(_out));
return _res;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "500")) {
if (Utils.contentTypeMatches(_contentType, "application/json")) {
com.styra.opa.openapi.models.errors.UnhealthyServer _out = Utils.mapper().readValue(
Utils.toUtf8AndClose(_httpRes.body()),
new TypeReference() {});
throw _out;
} else {
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected content-type received: " + _contentType,
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
if (Utils.statusCodeMatches(_httpRes.statusCode(), "4XX", "5XX")) {
// no content
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"API error occurred",
Utils.toByteArrayAndClose(_httpRes.body()));
}
throw new SDKError(
_httpRes,
_httpRes.statusCode(),
"Unexpected status code received: " + _httpRes.statusCode(),
Utils.toByteArrayAndClose(_httpRes.body()));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy