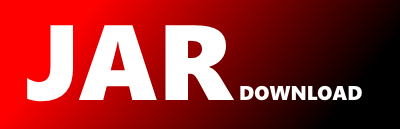
com.styra.opa.openapi.models.errors.ClientError Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opa Show documentation
Show all versions of opa Show documentation
SDK enabling Java developers to easily integrate with the Styra API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.styra.opa.openapi.models.errors;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonInclude.Include;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.styra.opa.openapi.utils.Utils;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.util.List;
import java.util.Objects;
import java.util.Optional;
/**
* ClientError - Bad Request
*/
public class ClientError extends RuntimeException {
@JsonProperty("code")
private String code;
@JsonProperty("message")
private String message;
@JsonInclude(Include.NON_ABSENT)
@JsonProperty("errors")
private Optional extends List> errors;
@JsonCreator
public ClientError(
@JsonProperty("code") String code,
@JsonProperty("message") String message,
@JsonProperty("errors") Optional extends List> errors) {
Utils.checkNotNull(code, "code");
Utils.checkNotNull(message, "message");
Utils.checkNotNull(errors, "errors");
this.code = code;
this.message = message;
this.errors = errors;
}
public ClientError(
String code,
String message) {
this(code, message, Optional.empty());
}
public String code(){
return code;
}
public String message(){
return message;
}
@SuppressWarnings("unchecked")
public Optional> errors(){
return (Optional>) errors;
}
public final static Builder builder() {
return new Builder();
}
public ClientError withCode(String code) {
Utils.checkNotNull(code, "code");
this.code = code;
return this;
}
public ClientError withMessage(String message) {
Utils.checkNotNull(message, "message");
this.message = message;
return this;
}
public ClientError withErrors(List errors) {
Utils.checkNotNull(errors, "errors");
this.errors = Optional.ofNullable(errors);
return this;
}
public ClientError withErrors(Optional extends List> errors) {
Utils.checkNotNull(errors, "errors");
this.errors = errors;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ClientError other = (ClientError) o;
return
Objects.deepEquals(this.code, other.code) &&
Objects.deepEquals(this.message, other.message) &&
Objects.deepEquals(this.errors, other.errors);
}
@Override
public int hashCode() {
return java.util.Objects.hash(
code,
message,
errors);
}
@Override
public String toString() {
return Utils.toString(ClientError.class,
"code", code,
"message", message,
"errors", errors);
}
public final static class Builder {
private String code;
private String message;
private Optional extends List> errors = Optional.empty();
private Builder() {
// force use of static builder() method
}
public Builder code(String code) {
Utils.checkNotNull(code, "code");
this.code = code;
return this;
}
public Builder message(String message) {
Utils.checkNotNull(message, "message");
this.message = message;
return this;
}
public Builder errors(List errors) {
Utils.checkNotNull(errors, "errors");
this.errors = Optional.ofNullable(errors);
return this;
}
public Builder errors(Optional extends List> errors) {
Utils.checkNotNull(errors, "errors");
this.errors = errors;
return this;
}
public ClientError build() {
return new ClientError(
code,
message,
errors);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy