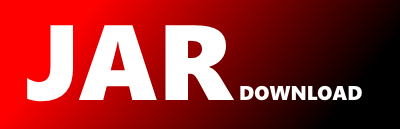
com.styra.opa.openapi.models.errors.ServerErrorLocation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opa Show documentation
Show all versions of opa Show documentation
SDK enabling Java developers to easily integrate with the Styra API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.styra.opa.openapi.models.errors;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.styra.opa.openapi.utils.Utils;
import java.lang.Long;
import java.lang.Override;
import java.lang.String;
import java.util.Objects;
public class ServerErrorLocation {
@JsonProperty("file")
private String file;
@JsonProperty("row")
private long row;
@JsonProperty("col")
private long col;
@JsonCreator
public ServerErrorLocation(
@JsonProperty("file") String file,
@JsonProperty("row") long row,
@JsonProperty("col") long col) {
Utils.checkNotNull(file, "file");
Utils.checkNotNull(row, "row");
Utils.checkNotNull(col, "col");
this.file = file;
this.row = row;
this.col = col;
}
@JsonIgnore
public String file() {
return file;
}
@JsonIgnore
public long row() {
return row;
}
@JsonIgnore
public long col() {
return col;
}
public final static Builder builder() {
return new Builder();
}
public ServerErrorLocation withFile(String file) {
Utils.checkNotNull(file, "file");
this.file = file;
return this;
}
public ServerErrorLocation withRow(long row) {
Utils.checkNotNull(row, "row");
this.row = row;
return this;
}
public ServerErrorLocation withCol(long col) {
Utils.checkNotNull(col, "col");
this.col = col;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ServerErrorLocation other = (ServerErrorLocation) o;
return
Objects.deepEquals(this.file, other.file) &&
Objects.deepEquals(this.row, other.row) &&
Objects.deepEquals(this.col, other.col);
}
@Override
public int hashCode() {
return Objects.hash(
file,
row,
col);
}
@Override
public String toString() {
return Utils.toString(ServerErrorLocation.class,
"file", file,
"row", row,
"col", col);
}
public final static class Builder {
private String file;
private Long row;
private Long col;
private Builder() {
// force use of static builder() method
}
public Builder file(String file) {
Utils.checkNotNull(file, "file");
this.file = file;
return this;
}
public Builder row(long row) {
Utils.checkNotNull(row, "row");
this.row = row;
return this;
}
public Builder col(long col) {
Utils.checkNotNull(col, "col");
this.col = col;
return this;
}
public ServerErrorLocation build() {
return new ServerErrorLocation(
file,
row,
col);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy