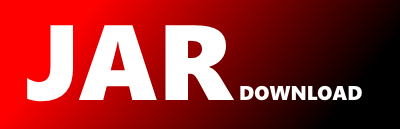
com.styra.opa.openapi.models.operations.ExecutePolicyWithInputResponse Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of opa Show documentation
Show all versions of opa Show documentation
SDK enabling Java developers to easily integrate with the Styra API.
The newest version!
/*
* Code generated by Speakeasy (https://speakeasy.com). DO NOT EDIT.
*/
package com.styra.opa.openapi.models.operations;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.styra.opa.openapi.models.shared.SuccessfulPolicyResponse;
import com.styra.opa.openapi.utils.Response;
import com.styra.opa.openapi.utils.Utils;
import java.io.InputStream;
import java.lang.Integer;
import java.lang.Override;
import java.lang.String;
import java.lang.SuppressWarnings;
import java.net.http.HttpResponse;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import java.util.Optional;
public class ExecutePolicyWithInputResponse implements Response {
/**
* HTTP response content type for this operation
*/
private String contentType;
/**
* HTTP response status code for this operation
*/
private int statusCode;
/**
* Raw HTTP response; suitable for custom response parsing
*/
private HttpResponse rawResponse;
/**
* Success.
* The server also returns 200 if the path refers to an undefined document. In this case, the response will not contain a result property.
*
*/
private Optional extends SuccessfulPolicyResponse> successfulPolicyResponse;
private Map> headers;
@JsonCreator
public ExecutePolicyWithInputResponse(
String contentType,
int statusCode,
HttpResponse rawResponse,
Optional extends SuccessfulPolicyResponse> successfulPolicyResponse,
Map> headers) {
Utils.checkNotNull(contentType, "contentType");
Utils.checkNotNull(statusCode, "statusCode");
Utils.checkNotNull(rawResponse, "rawResponse");
Utils.checkNotNull(successfulPolicyResponse, "successfulPolicyResponse");
headers = Utils.emptyMapIfNull(headers);
this.contentType = contentType;
this.statusCode = statusCode;
this.rawResponse = rawResponse;
this.successfulPolicyResponse = successfulPolicyResponse;
this.headers = headers;
}
public ExecutePolicyWithInputResponse(
String contentType,
int statusCode,
HttpResponse rawResponse,
Map> headers) {
this(contentType, statusCode, rawResponse, Optional.empty(), headers);
}
/**
* HTTP response content type for this operation
*/
@JsonIgnore
public String contentType() {
return contentType;
}
/**
* HTTP response status code for this operation
*/
@JsonIgnore
public int statusCode() {
return statusCode;
}
/**
* Raw HTTP response; suitable for custom response parsing
*/
@JsonIgnore
public HttpResponse rawResponse() {
return rawResponse;
}
/**
* Success.
* The server also returns 200 if the path refers to an undefined document. In this case, the response will not contain a result property.
*
*/
@SuppressWarnings("unchecked")
@JsonIgnore
public Optional successfulPolicyResponse() {
return (Optional) successfulPolicyResponse;
}
@JsonIgnore
public Map> headers() {
return headers;
}
public final static Builder builder() {
return new Builder();
}
/**
* HTTP response content type for this operation
*/
public ExecutePolicyWithInputResponse withContentType(String contentType) {
Utils.checkNotNull(contentType, "contentType");
this.contentType = contentType;
return this;
}
/**
* HTTP response status code for this operation
*/
public ExecutePolicyWithInputResponse withStatusCode(int statusCode) {
Utils.checkNotNull(statusCode, "statusCode");
this.statusCode = statusCode;
return this;
}
/**
* Raw HTTP response; suitable for custom response parsing
*/
public ExecutePolicyWithInputResponse withRawResponse(HttpResponse rawResponse) {
Utils.checkNotNull(rawResponse, "rawResponse");
this.rawResponse = rawResponse;
return this;
}
/**
* Success.
* The server also returns 200 if the path refers to an undefined document. In this case, the response will not contain a result property.
*
*/
public ExecutePolicyWithInputResponse withSuccessfulPolicyResponse(SuccessfulPolicyResponse successfulPolicyResponse) {
Utils.checkNotNull(successfulPolicyResponse, "successfulPolicyResponse");
this.successfulPolicyResponse = Optional.ofNullable(successfulPolicyResponse);
return this;
}
/**
* Success.
* The server also returns 200 if the path refers to an undefined document. In this case, the response will not contain a result property.
*
*/
public ExecutePolicyWithInputResponse withSuccessfulPolicyResponse(Optional extends SuccessfulPolicyResponse> successfulPolicyResponse) {
Utils.checkNotNull(successfulPolicyResponse, "successfulPolicyResponse");
this.successfulPolicyResponse = successfulPolicyResponse;
return this;
}
public ExecutePolicyWithInputResponse withHeaders(Map> headers) {
Utils.checkNotNull(headers, "headers");
this.headers = headers;
return this;
}
@Override
public boolean equals(java.lang.Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
ExecutePolicyWithInputResponse other = (ExecutePolicyWithInputResponse) o;
return
Objects.deepEquals(this.contentType, other.contentType) &&
Objects.deepEquals(this.statusCode, other.statusCode) &&
Objects.deepEquals(this.rawResponse, other.rawResponse) &&
Objects.deepEquals(this.successfulPolicyResponse, other.successfulPolicyResponse) &&
Objects.deepEquals(this.headers, other.headers);
}
@Override
public int hashCode() {
return Objects.hash(
contentType,
statusCode,
rawResponse,
successfulPolicyResponse,
headers);
}
@Override
public String toString() {
return Utils.toString(ExecutePolicyWithInputResponse.class,
"contentType", contentType,
"statusCode", statusCode,
"rawResponse", rawResponse,
"successfulPolicyResponse", successfulPolicyResponse,
"headers", headers);
}
public final static class Builder {
private String contentType;
private Integer statusCode;
private HttpResponse rawResponse;
private Optional extends SuccessfulPolicyResponse> successfulPolicyResponse = Optional.empty();
private Map> headers;
private Builder() {
// force use of static builder() method
}
/**
* HTTP response content type for this operation
*/
public Builder contentType(String contentType) {
Utils.checkNotNull(contentType, "contentType");
this.contentType = contentType;
return this;
}
/**
* HTTP response status code for this operation
*/
public Builder statusCode(int statusCode) {
Utils.checkNotNull(statusCode, "statusCode");
this.statusCode = statusCode;
return this;
}
/**
* Raw HTTP response; suitable for custom response parsing
*/
public Builder rawResponse(HttpResponse rawResponse) {
Utils.checkNotNull(rawResponse, "rawResponse");
this.rawResponse = rawResponse;
return this;
}
/**
* Success.
* The server also returns 200 if the path refers to an undefined document. In this case, the response will not contain a result property.
*
*/
public Builder successfulPolicyResponse(SuccessfulPolicyResponse successfulPolicyResponse) {
Utils.checkNotNull(successfulPolicyResponse, "successfulPolicyResponse");
this.successfulPolicyResponse = Optional.ofNullable(successfulPolicyResponse);
return this;
}
/**
* Success.
* The server also returns 200 if the path refers to an undefined document. In this case, the response will not contain a result property.
*
*/
public Builder successfulPolicyResponse(Optional extends SuccessfulPolicyResponse> successfulPolicyResponse) {
Utils.checkNotNull(successfulPolicyResponse, "successfulPolicyResponse");
this.successfulPolicyResponse = successfulPolicyResponse;
return this;
}
public Builder headers(Map> headers) {
Utils.checkNotNull(headers, "headers");
this.headers = headers;
return this;
}
public ExecutePolicyWithInputResponse build() {
return new ExecutePolicyWithInputResponse(
contentType,
statusCode,
rawResponse,
successfulPolicyResponse,
headers);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy