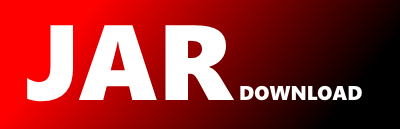
com.stytch.java.consumer.api.m2mclientssecrets.M2MClientsSecrets.kt Maven / Gradle / Ivy
package com.stytch.java.consumer.api.m2mclientssecrets
// !!!
// WARNING: This file is autogenerated
// Only modify code within MANUAL() sections
// or your changes may be overwritten later!
// !!!
import com.squareup.moshi.Moshi
import com.stytch.java.common.InstantAdapter
import com.stytch.java.common.StytchResult
import com.stytch.java.consumer.models.m2mclientssecrets.RotateCancelRequest
import com.stytch.java.consumer.models.m2mclientssecrets.RotateCancelResponse
import com.stytch.java.consumer.models.m2mclientssecrets.RotateRequest
import com.stytch.java.consumer.models.m2mclientssecrets.RotateResponse
import com.stytch.java.consumer.models.m2mclientssecrets.RotateStartRequest
import com.stytch.java.consumer.models.m2mclientssecrets.RotateStartResponse
import com.stytch.java.http.HttpClient
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.async
import kotlinx.coroutines.future.asCompletableFuture
import kotlinx.coroutines.launch
import kotlinx.coroutines.withContext
import java.util.concurrent.CompletableFuture
public interface Secrets {
/**
* Initiate the rotation of an M2M client secret. After this endpoint is called, both the client's `client_secret` and
* `next_client_secret` will be valid. To complete the secret rotation flow, update all usages of `client_secret` to
* `next_client_secret` and call the
* [Rotate Secret Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret)[Rotate Secret Endpoint](https://stytch.com/docs/api/m2m-rotate-secret) to complete the flow.
* Secret rotation can be cancelled using the
* [Rotate Cancel Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-cancel)[Rotate Cancel Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-cancel).
*
* **Important:** This is the only time you will be able to view the generated `next_client_secret` in the API response.
* Stytch stores a hash of the `next_client_secret` and cannot recover the value if lost. Be sure to persist the
* `next_client_secret` in a secure location. If the `next_client_secret` is lost, you will need to trigger a secret
* rotation flow to receive another one.
*/
public suspend fun rotateStart(data: RotateStartRequest): StytchResult
/**
* Initiate the rotation of an M2M client secret. After this endpoint is called, both the client's `client_secret` and
* `next_client_secret` will be valid. To complete the secret rotation flow, update all usages of `client_secret` to
* `next_client_secret` and call the
* [Rotate Secret Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret)[Rotate Secret Endpoint](https://stytch.com/docs/api/m2m-rotate-secret) to complete the flow.
* Secret rotation can be cancelled using the
* [Rotate Cancel Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-cancel)[Rotate Cancel Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-cancel).
*
* **Important:** This is the only time you will be able to view the generated `next_client_secret` in the API response.
* Stytch stores a hash of the `next_client_secret` and cannot recover the value if lost. Be sure to persist the
* `next_client_secret` in a secure location. If the `next_client_secret` is lost, you will need to trigger a secret
* rotation flow to receive another one.
*/
public fun rotateStart(
data: RotateStartRequest,
callback: (StytchResult) -> Unit,
)
/**
* Initiate the rotation of an M2M client secret. After this endpoint is called, both the client's `client_secret` and
* `next_client_secret` will be valid. To complete the secret rotation flow, update all usages of `client_secret` to
* `next_client_secret` and call the
* [Rotate Secret Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret)[Rotate Secret Endpoint](https://stytch.com/docs/api/m2m-rotate-secret) to complete the flow.
* Secret rotation can be cancelled using the
* [Rotate Cancel Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-cancel)[Rotate Cancel Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-cancel).
*
* **Important:** This is the only time you will be able to view the generated `next_client_secret` in the API response.
* Stytch stores a hash of the `next_client_secret` and cannot recover the value if lost. Be sure to persist the
* `next_client_secret` in a secure location. If the `next_client_secret` is lost, you will need to trigger a secret
* rotation flow to receive another one.
*/
public fun rotateStartCompletable(data: RotateStartRequest): CompletableFuture>
/**
* Cancel the rotation of an M2M client secret started with the
* [Start Secret Rotation Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-start)
* [Start Secret Rotation Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-start).
* After this endpoint is called, the client's `next_client_secret` is discarded and only the original `client_secret`
* will be valid.
*/
public suspend fun rotateCancel(data: RotateCancelRequest): StytchResult
/**
* Cancel the rotation of an M2M client secret started with the
* [Start Secret Rotation Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-start)
* [Start Secret Rotation Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-start).
* After this endpoint is called, the client's `next_client_secret` is discarded and only the original `client_secret`
* will be valid.
*/
public fun rotateCancel(
data: RotateCancelRequest,
callback: (StytchResult) -> Unit,
)
/**
* Cancel the rotation of an M2M client secret started with the
* [Start Secret Rotation Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-start)
* [Start Secret Rotation Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-start).
* After this endpoint is called, the client's `next_client_secret` is discarded and only the original `client_secret`
* will be valid.
*/
public fun rotateCancelCompletable(data: RotateCancelRequest): CompletableFuture>
/**
* Complete the rotation of an M2M client secret started with the
* [Start Secret Rotation Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-start)
* [Start Secret Rotation Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-start).
* After this endpoint is called, the client's `next_client_secret` becomes its `client_secret` and the previous
* `client_secret` will no longer be valid.
*/
public suspend fun rotate(data: RotateRequest): StytchResult
/**
* Complete the rotation of an M2M client secret started with the
* [Start Secret Rotation Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-start)
* [Start Secret Rotation Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-start).
* After this endpoint is called, the client's `next_client_secret` becomes its `client_secret` and the previous
* `client_secret` will no longer be valid.
*/
public fun rotate(
data: RotateRequest,
callback: (StytchResult) -> Unit,
)
/**
* Complete the rotation of an M2M client secret started with the
* [Start Secret Rotation Endpoint](https://stytch.com/docs/b2b/api/m2m-rotate-secret-start)
* [Start Secret Rotation Endpoint](https://stytch.com/docs/api/m2m-rotate-secret-start).
* After this endpoint is called, the client's `next_client_secret` becomes its `client_secret` and the previous
* `client_secret` will no longer be valid.
*/
public fun rotateCompletable(data: RotateRequest): CompletableFuture>
}
internal class SecretsImpl(
private val httpClient: HttpClient,
private val coroutineScope: CoroutineScope,
) : Secrets {
private val moshi = Moshi.Builder().add(InstantAdapter()).build()
override suspend fun rotateStart(data: RotateStartRequest): StytchResult =
withContext(Dispatchers.IO) {
var headers = emptyMap()
val asJson = moshi.adapter(RotateStartRequest::class.java).toJson(data)
httpClient.post("/v1/m2m/clients/${data.clientId}/secrets/rotate/start", asJson, headers)
}
override fun rotateStart(
data: RotateStartRequest,
callback: (StytchResult) -> Unit,
) {
coroutineScope.launch {
callback(rotateStart(data))
}
}
override fun rotateStartCompletable(data: RotateStartRequest): CompletableFuture> =
coroutineScope.async {
rotateStart(data)
}.asCompletableFuture()
override suspend fun rotateCancel(data: RotateCancelRequest): StytchResult =
withContext(Dispatchers.IO) {
var headers = emptyMap()
val asJson = moshi.adapter(RotateCancelRequest::class.java).toJson(data)
httpClient.post("/v1/m2m/clients/${data.clientId}/secrets/rotate/cancel", asJson, headers)
}
override fun rotateCancel(
data: RotateCancelRequest,
callback: (StytchResult) -> Unit,
) {
coroutineScope.launch {
callback(rotateCancel(data))
}
}
override fun rotateCancelCompletable(data: RotateCancelRequest): CompletableFuture> =
coroutineScope.async {
rotateCancel(data)
}.asCompletableFuture()
override suspend fun rotate(data: RotateRequest): StytchResult =
withContext(Dispatchers.IO) {
var headers = emptyMap()
val asJson = moshi.adapter(RotateRequest::class.java).toJson(data)
httpClient.post("/v1/m2m/clients/${data.clientId}/secrets/rotate", asJson, headers)
}
override fun rotate(
data: RotateRequest,
callback: (StytchResult) -> Unit,
) {
coroutineScope.launch {
callback(rotate(data))
}
}
override fun rotateCompletable(data: RotateRequest): CompletableFuture> =
coroutineScope.async {
rotate(data)
}.asCompletableFuture()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy