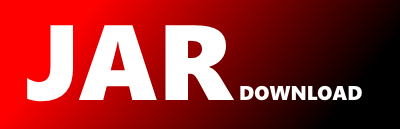
com.stytch.java.consumer.api.otpwhatsapp.OTPWhatsapp.kt Maven / Gradle / Ivy
package com.stytch.java.consumer.api.otpwhatsapp
// !!!
// WARNING: This file is autogenerated
// Only modify code within MANUAL() sections
// or your changes may be overwritten later!
// !!!
import com.squareup.moshi.Moshi
import com.stytch.java.common.InstantAdapter
import com.stytch.java.common.StytchResult
import com.stytch.java.consumer.models.otpwhatsapp.LoginOrCreateRequest
import com.stytch.java.consumer.models.otpwhatsapp.LoginOrCreateResponse
import com.stytch.java.consumer.models.otpwhatsapp.SendRequest
import com.stytch.java.consumer.models.otpwhatsapp.SendResponse
import com.stytch.java.http.HttpClient
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.async
import kotlinx.coroutines.future.asCompletableFuture
import kotlinx.coroutines.launch
import kotlinx.coroutines.withContext
import java.util.concurrent.CompletableFuture
public interface Whatsapp {
/**
* Send a One-Time Passcode (OTP) to a User's WhatsApp. If you'd like to create a user and send them a passcode with one
* request, use our [log in or create](https://stytch.com/docs/api/whatsapp-login-or-create) endpoint.
*
* Note that sending another OTP code before the first has expired will invalidate the first code.
*
* ### Cost to send SMS OTP
* Before configuring SMS or WhatsApp OTPs, please review how Stytch
* [bills the costs of international OTPs](https://stytch.com/pricing) and understand how to protect your app against
* [toll fraud](https://stytch.com/docs/guides/passcodes/toll-fraud/overview).
*
* ### Add a phone number to an existing user
*
* This endpoint also allows you to add a new phone number to an existing Stytch User. Including a `user_id`,
* `session_token`, or `session_jwt` in your Send one-time passcode by WhatsApp request will add the new, unverified phone
* number to the existing Stytch User. If the user successfully authenticates within 5 minutes, the new phone number will
* be marked as verified and remain permanently on the existing Stytch User. Otherwise, it will be removed from the User
* object, and any subsequent login requests using that phone number will create a new User.
*
* ### Next steps
*
* Collect the OTP which was delivered to the user. Call [Authenticate OTP](https://stytch.com/docs/api/authenticate-otp)
* using the OTP `code` along with the `phone_id` found in the response as the `method_id`.
*/
public suspend fun send(data: SendRequest): StytchResult
/**
* Send a One-Time Passcode (OTP) to a User's WhatsApp. If you'd like to create a user and send them a passcode with one
* request, use our [log in or create](https://stytch.com/docs/api/whatsapp-login-or-create) endpoint.
*
* Note that sending another OTP code before the first has expired will invalidate the first code.
*
* ### Cost to send SMS OTP
* Before configuring SMS or WhatsApp OTPs, please review how Stytch
* [bills the costs of international OTPs](https://stytch.com/pricing) and understand how to protect your app against
* [toll fraud](https://stytch.com/docs/guides/passcodes/toll-fraud/overview).
*
* ### Add a phone number to an existing user
*
* This endpoint also allows you to add a new phone number to an existing Stytch User. Including a `user_id`,
* `session_token`, or `session_jwt` in your Send one-time passcode by WhatsApp request will add the new, unverified phone
* number to the existing Stytch User. If the user successfully authenticates within 5 minutes, the new phone number will
* be marked as verified and remain permanently on the existing Stytch User. Otherwise, it will be removed from the User
* object, and any subsequent login requests using that phone number will create a new User.
*
* ### Next steps
*
* Collect the OTP which was delivered to the user. Call [Authenticate OTP](https://stytch.com/docs/api/authenticate-otp)
* using the OTP `code` along with the `phone_id` found in the response as the `method_id`.
*/
public fun send(
data: SendRequest,
callback: (StytchResult) -> Unit,
)
/**
* Send a One-Time Passcode (OTP) to a User's WhatsApp. If you'd like to create a user and send them a passcode with one
* request, use our [log in or create](https://stytch.com/docs/api/whatsapp-login-or-create) endpoint.
*
* Note that sending another OTP code before the first has expired will invalidate the first code.
*
* ### Cost to send SMS OTP
* Before configuring SMS or WhatsApp OTPs, please review how Stytch
* [bills the costs of international OTPs](https://stytch.com/pricing) and understand how to protect your app against
* [toll fraud](https://stytch.com/docs/guides/passcodes/toll-fraud/overview).
*
* ### Add a phone number to an existing user
*
* This endpoint also allows you to add a new phone number to an existing Stytch User. Including a `user_id`,
* `session_token`, or `session_jwt` in your Send one-time passcode by WhatsApp request will add the new, unverified phone
* number to the existing Stytch User. If the user successfully authenticates within 5 minutes, the new phone number will
* be marked as verified and remain permanently on the existing Stytch User. Otherwise, it will be removed from the User
* object, and any subsequent login requests using that phone number will create a new User.
*
* ### Next steps
*
* Collect the OTP which was delivered to the user. Call [Authenticate OTP](https://stytch.com/docs/api/authenticate-otp)
* using the OTP `code` along with the `phone_id` found in the response as the `method_id`.
*/
public fun sendCompletable(data: SendRequest): CompletableFuture>
/**
* Send a one-time passcode (OTP) to a User's WhatsApp using their phone number. If the phone number is not associated
* with a User already, a User will be created.
*
* ### Cost to send SMS OTP
* Before configuring SMS or WhatsApp OTPs, please review how Stytch
* [bills the costs of international OTPs](https://stytch.com/pricing) and understand how to protect your app against
* [toll fraud](https://stytch.com/docs/guides/passcodes/toll-fraud/overview).
*
* ### Next steps
*
* Collect the OTP which was delivered to the User. Call [Authenticate OTP](https://stytch.com/docs/api/authenticate-otp)
* using the OTP `code` along with the `phone_id` found in the response as the `method_id`.
*/
public suspend fun loginOrCreate(data: LoginOrCreateRequest): StytchResult
/**
* Send a one-time passcode (OTP) to a User's WhatsApp using their phone number. If the phone number is not associated
* with a User already, a User will be created.
*
* ### Cost to send SMS OTP
* Before configuring SMS or WhatsApp OTPs, please review how Stytch
* [bills the costs of international OTPs](https://stytch.com/pricing) and understand how to protect your app against
* [toll fraud](https://stytch.com/docs/guides/passcodes/toll-fraud/overview).
*
* ### Next steps
*
* Collect the OTP which was delivered to the User. Call [Authenticate OTP](https://stytch.com/docs/api/authenticate-otp)
* using the OTP `code` along with the `phone_id` found in the response as the `method_id`.
*/
public fun loginOrCreate(
data: LoginOrCreateRequest,
callback: (StytchResult) -> Unit,
)
/**
* Send a one-time passcode (OTP) to a User's WhatsApp using their phone number. If the phone number is not associated
* with a User already, a User will be created.
*
* ### Cost to send SMS OTP
* Before configuring SMS or WhatsApp OTPs, please review how Stytch
* [bills the costs of international OTPs](https://stytch.com/pricing) and understand how to protect your app against
* [toll fraud](https://stytch.com/docs/guides/passcodes/toll-fraud/overview).
*
* ### Next steps
*
* Collect the OTP which was delivered to the User. Call [Authenticate OTP](https://stytch.com/docs/api/authenticate-otp)
* using the OTP `code` along with the `phone_id` found in the response as the `method_id`.
*/
public fun loginOrCreateCompletable(data: LoginOrCreateRequest): CompletableFuture>
}
internal class WhatsappImpl(
private val httpClient: HttpClient,
private val coroutineScope: CoroutineScope,
) : Whatsapp {
private val moshi = Moshi.Builder().add(InstantAdapter()).build()
override suspend fun send(data: SendRequest): StytchResult =
withContext(Dispatchers.IO) {
var headers = emptyMap()
val asJson = moshi.adapter(SendRequest::class.java).toJson(data)
httpClient.post("/v1/otps/whatsapp/send", asJson, headers)
}
override fun send(
data: SendRequest,
callback: (StytchResult) -> Unit,
) {
coroutineScope.launch {
callback(send(data))
}
}
override fun sendCompletable(data: SendRequest): CompletableFuture> =
coroutineScope.async {
send(data)
}.asCompletableFuture()
override suspend fun loginOrCreate(data: LoginOrCreateRequest): StytchResult =
withContext(Dispatchers.IO) {
var headers = emptyMap()
val asJson = moshi.adapter(LoginOrCreateRequest::class.java).toJson(data)
httpClient.post("/v1/otps/whatsapp/login_or_create", asJson, headers)
}
override fun loginOrCreate(
data: LoginOrCreateRequest,
callback: (StytchResult) -> Unit,
) {
coroutineScope.launch {
callback(loginOrCreate(data))
}
}
override fun loginOrCreateCompletable(data: LoginOrCreateRequest): CompletableFuture> =
coroutineScope.async {
loginOrCreate(data)
}.asCompletableFuture()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy