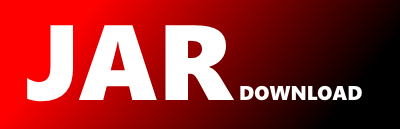
com.stytch.java.consumer.models.m2m.M2M.kt Maven / Gradle / Ivy
package com.stytch.java.consumer.models.m2m
// !!!
// WARNING: This file is autogenerated
// Only modify code within MANUAL() sections
// or your changes may be overwritten later!
// !!!
import com.squareup.moshi.Json
import com.squareup.moshi.JsonClass
@JsonClass(generateAdapter = false)
public enum class M2MSearchQueryOperator {
@Json(name = "OR")
OR,
@Json(name = "AND")
AND,
}
@JsonClass(generateAdapter = true)
public data class M2MClient
@JvmOverloads
constructor(
/**
* The ID of the client.
*/
@Json(name = "client_id")
val clientId: String,
/**
* A human-readable name for the client.
*/
@Json(name = "client_name")
val clientName: String,
/**
* A human-readable description for the client.
*/
@Json(name = "client_description")
val clientDescription: String,
/**
* The status of the client - either `active` or `inactive`.
*/
@Json(name = "status")
val status: String,
/**
* An array of scopes assigned to the client.
*/
@Json(name = "scopes")
val scopes: List,
/**
* The last four characters of the client secret.
*/
@Json(name = "client_secret_last_four")
val clientSecretLastFour: String,
/**
* An arbitrary JSON object for storing application-specific data.
*/
@Json(name = "trusted_metadata")
val trustedMetadata: Map? = emptyMap(),
/**
* The last four characters of the `next_client_secret`. Null if no `next_client_secret` exists.
*/
@Json(name = "next_client_secret_last_four")
val nextClientSecretLastFour: String? = null,
)
@JsonClass(generateAdapter = true)
public data class M2MClientWithClientSecret
@JvmOverloads
constructor(
/**
* The ID of the client.
*/
@Json(name = "client_id")
val clientId: String,
/**
* The secret of the client. **Important:** this is the only time you will be able to view the `client_secret`. Be sure to
* persist the `client_secret` in a secure location. If the `client_secret` is lost, you will need to trigger a secret
* rotation flow to receive another one.
*/
@Json(name = "client_secret")
val clientSecret: String,
/**
* A human-readable name for the client.
*/
@Json(name = "client_name")
val clientName: String,
/**
* A human-readable description for the client.
*/
@Json(name = "client_description")
val clientDescription: String,
/**
* The status of the client - either `active` or `inactive`.
*/
@Json(name = "status")
val status: String,
/**
* An array of scopes assigned to the client.
*/
@Json(name = "scopes")
val scopes: List,
/**
* The last four characters of the client secret.
*/
@Json(name = "client_secret_last_four")
val clientSecretLastFour: String,
/**
* An arbitrary JSON object for storing application-specific data.
*/
@Json(name = "trusted_metadata")
val trustedMetadata: Map? = emptyMap(),
/**
* The last four characters of the `next_client_secret`. Null if no `next_client_secret` exists.
*/
@Json(name = "next_client_secret_last_four")
val nextClientSecretLastFour: String? = null,
)
@JsonClass(generateAdapter = true)
public data class M2MClientWithNextClientSecret
@JvmOverloads
constructor(
/**
* The ID of the client.
*/
@Json(name = "client_id")
val clientId: String,
/**
* The newly created secret that's next in rotation for the client. **Important:** this is the only time you will be able
* to view the `next_client_secret`. Be sure to persist the `next_client_secret` in a secure location. If the
* `next_client_secret` is lost, you will need to trigger a secret rotation flow to receive another one.
*/
@Json(name = "next_client_secret")
val nextClientSecret: String,
/**
* A human-readable name for the client.
*/
@Json(name = "client_name")
val clientName: String,
/**
* A human-readable description for the client.
*/
@Json(name = "client_description")
val clientDescription: String,
/**
* The status of the client - either `active` or `inactive`.
*/
@Json(name = "status")
val status: String,
/**
* An array of scopes assigned to the client.
*/
@Json(name = "scopes")
val scopes: List,
/**
* The last four characters of the client secret.
*/
@Json(name = "client_secret_last_four")
val clientSecretLastFour: String,
/**
* An arbitrary JSON object for storing application-specific data.
*/
@Json(name = "trusted_metadata")
val trustedMetadata: Map? = emptyMap(),
/**
* The last four characters of the `next_client_secret`. Null if no `next_client_secret` exists.
*/
@Json(name = "next_client_secret_last_four")
val nextClientSecretLastFour: String? = null,
)
@JsonClass(generateAdapter = true)
public data class M2MSearchQuery
@JvmOverloads
constructor(
/**
* The action to perform on the operands. The accepted value are:
*
* `AND` – all the operand values provided must match.
*
* `OR` – the operator will return any matches to at least one of the operand values you supply.
*/
@Json(name = "operator")
val operator: M2MSearchQueryOperator,
/**
* An array of operand objects that contains all of the filters and values to apply to your search search query.
*/
@Json(name = "operands")
val operands: List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy