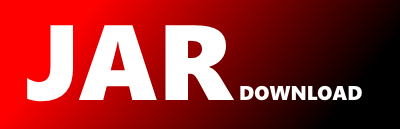
main.com.stytch.java.b2b.api.discoveryintermediatesessions.DiscoveryIntermediateSessions.kt Maven / Gradle / Ivy
package com.stytch.java.b2b.api.discoveryintermediatesessions
// !!!
// WARNING: This file is autogenerated
// Only modify code within MANUAL() sections
// or your changes may be overwritten later!
// !!!
import com.squareup.moshi.Moshi
import com.stytch.java.b2b.models.discoveryintermediatesessions.ExchangeRequest
import com.stytch.java.b2b.models.discoveryintermediatesessions.ExchangeResponse
import com.stytch.java.common.InstantAdapter
import com.stytch.java.common.StytchResult
import com.stytch.java.http.HttpClient
import kotlinx.coroutines.CoroutineScope
import kotlinx.coroutines.Dispatchers
import kotlinx.coroutines.async
import kotlinx.coroutines.future.asCompletableFuture
import kotlinx.coroutines.launch
import kotlinx.coroutines.withContext
import java.util.concurrent.CompletableFuture
public interface IntermediateSessions {
/**
* Exchange an Intermediate Session for a fully realized [Member Session](https://stytch.com/docs/b2b/api/session-object)
* in a desired [Organization](https://stytch.com/docs/b2b/api/organization-object).
* This operation consumes the Intermediate Session.
*
* This endpoint can be used to accept invites and create new members via domain matching.
*
* If the Member is required to complete MFA to log in to the Organization, the returned value of `member_authenticated`
* will be `false`.
* The `intermediate_session_token` will not be consumed and instead will be returned in the response.
* The `intermediate_session_token` can be passed into the
* [OTP SMS Authenticate endpoint](https://stytch.com/docs/b2b/api/authenticate-otp-sms) to complete the MFA step and
* acquire a full member session.
* The `intermediate_session_token` can also be used with the
* [Exchange Intermediate Session endpoint](https://stytch.com/docs/b2b/api/exchange-intermediate-session) or the
* [Create Organization via Discovery endpoint](https://stytch.com/docs/b2b/api/create-organization-via-discovery) to join
* a different Organization or create a new one.
* The `session_duration_minutes` and `session_custom_claims` parameters will be ignored.
*/
public suspend fun exchange(data: ExchangeRequest): StytchResult
/**
* Exchange an Intermediate Session for a fully realized [Member Session](https://stytch.com/docs/b2b/api/session-object)
* in a desired [Organization](https://stytch.com/docs/b2b/api/organization-object).
* This operation consumes the Intermediate Session.
*
* This endpoint can be used to accept invites and create new members via domain matching.
*
* If the Member is required to complete MFA to log in to the Organization, the returned value of `member_authenticated`
* will be `false`.
* The `intermediate_session_token` will not be consumed and instead will be returned in the response.
* The `intermediate_session_token` can be passed into the
* [OTP SMS Authenticate endpoint](https://stytch.com/docs/b2b/api/authenticate-otp-sms) to complete the MFA step and
* acquire a full member session.
* The `intermediate_session_token` can also be used with the
* [Exchange Intermediate Session endpoint](https://stytch.com/docs/b2b/api/exchange-intermediate-session) or the
* [Create Organization via Discovery endpoint](https://stytch.com/docs/b2b/api/create-organization-via-discovery) to join
* a different Organization or create a new one.
* The `session_duration_minutes` and `session_custom_claims` parameters will be ignored.
*/
public fun exchange(
data: ExchangeRequest,
callback: (StytchResult) -> Unit,
)
/**
* Exchange an Intermediate Session for a fully realized [Member Session](https://stytch.com/docs/b2b/api/session-object)
* in a desired [Organization](https://stytch.com/docs/b2b/api/organization-object).
* This operation consumes the Intermediate Session.
*
* This endpoint can be used to accept invites and create new members via domain matching.
*
* If the Member is required to complete MFA to log in to the Organization, the returned value of `member_authenticated`
* will be `false`.
* The `intermediate_session_token` will not be consumed and instead will be returned in the response.
* The `intermediate_session_token` can be passed into the
* [OTP SMS Authenticate endpoint](https://stytch.com/docs/b2b/api/authenticate-otp-sms) to complete the MFA step and
* acquire a full member session.
* The `intermediate_session_token` can also be used with the
* [Exchange Intermediate Session endpoint](https://stytch.com/docs/b2b/api/exchange-intermediate-session) or the
* [Create Organization via Discovery endpoint](https://stytch.com/docs/b2b/api/create-organization-via-discovery) to join
* a different Organization or create a new one.
* The `session_duration_minutes` and `session_custom_claims` parameters will be ignored.
*/
public fun exchangeCompletable(data: ExchangeRequest): CompletableFuture>
}
internal class IntermediateSessionsImpl(
private val httpClient: HttpClient,
private val coroutineScope: CoroutineScope,
) : IntermediateSessions {
private val moshi = Moshi.Builder().add(InstantAdapter()).build()
override suspend fun exchange(data: ExchangeRequest): StytchResult =
withContext(Dispatchers.IO) {
var headers = emptyMap()
val asJson = moshi.adapter(ExchangeRequest::class.java).toJson(data)
httpClient.post("/v1/b2b/discovery/intermediate_sessions/exchange", asJson, headers)
}
override fun exchange(
data: ExchangeRequest,
callback: (StytchResult) -> Unit,
) {
coroutineScope.launch {
callback(exchange(data))
}
}
override fun exchangeCompletable(data: ExchangeRequest): CompletableFuture> =
coroutineScope.async {
exchange(data)
}.asCompletableFuture()
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy