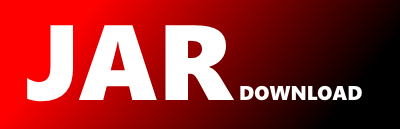
com.sun.electric.util.math.AbstractFixpPoint Maven / Gradle / Ivy
The newest version!
/* -*- tab-width: 4 -*-
*
* Electric(tm) VLSI Design System
*
* File: AbstractFixpPoint.java
* Written by: Dmitry Nadezhin, Sun Microsystems.
*
* Copyright (c) 2011, Oracle and/or its affiliates. All rights reserved.
*
* Electric(tm) is free software; you can redistribute it and/or modify
* it under the terms of the GNU General Public License as published by
* the Free Software Foundation; either version 3 of the License, or
* (at your option) any later version.
*
* Electric(tm) is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU General Public License
* along with Electric(tm); see the file COPYING. If not, write to
* the Free Software Foundation, Inc., 59 Temple Place, Suite 330,
* Boston, Mass 02111-1307, USA.
*/
package com.sun.electric.util.math;
import java.awt.geom.Point2D;
/**
*
*/
public abstract class AbstractFixpPoint extends Point2D {
public abstract long getFixpX();
public abstract long getFixpY();
public abstract void setFixpLocation(long fixpX, long fixpY);
protected abstract AbstractFixpPoint create(long fixpX, long fixpY);
@Override
public double getX() {
return FixpCoord.fixpToLambda(getFixpX());
}
@Override
public double getY() {
return FixpCoord.fixpToLambda(getFixpY());
}
@Override
public void setLocation(double x, double y) {
setFixpLocation(FixpCoord.lambdaToFixp(x), FixpCoord.lambdaToFixp(y));
}
/**
* Sets the location of this Point2D
to the same
* coordinates as the specified Point2D
object.
* @param p the specified Point2D
to which to set
* this Point2D
* @since 1.2
*/
@Override
public void setLocation(Point2D p) {
if (p instanceof AbstractFixpPoint) {
AbstractFixpPoint fp = (AbstractFixpPoint)p;
setFixpLocation(fp.getFixpX(), fp.getFixpY());
} else {
super.setLocation(p);
}
}
/**
* Returns the square of the distance from this
* Point2D
to a specified Point2D
.
*
* @param pt the specified point to be measured
* against this Point2D
* @return the square of the distance between this
* Point2D
to a specified Point2D
.
* @since 1.2
*/
@Override
public double distanceSq(Point2D pt) {
if (pt instanceof AbstractFixpPoint) {
AbstractFixpPoint fp = (AbstractFixpPoint)pt;
long fx = getFixpX() - fp.getFixpX();
long fy = getFixpY() - fp.getFixpY();
if (fx == 0) {
double dy = FixpCoord.fixpToLambda(fy);
return dy * dy;
} else if (fy == 0) {
double dx = FixpCoord.fixpToLambda(fx);
return dx * dx;
} else {
double dx = FixpCoord.fixpToLambda(fx);
double dy = FixpCoord.fixpToLambda(fy);
return dx * dx + dy * dy;
}
}
return super.distanceSq(pt);
}
/**
* Returns the square of the distance from this
* Point2D
to a specified Point2D
.
*
* @param pt the specified point to be measured
* against this Point2D
* @return the square of the distance between this
* Point2D
to a specified Point2D
.
* @since 1.2
*/
@Override
public double distance(Point2D pt) {
if (pt instanceof AbstractFixpPoint) {
AbstractFixpPoint fp = (AbstractFixpPoint)pt;
long fx = getFixpX() - fp.getFixpX();
long fy = getFixpY() - fp.getFixpY();
if (fx == 0) {
return FixpCoord.fixpToLambda(Math.abs(fy));
} else if (fy == 0) {
return FixpCoord.fixpToLambda(Math.abs(fx));
} else {
double dx = FixpCoord.fixpToLambda(fx);
double dy = FixpCoord.fixpToLambda(fy);
return Math.sqrt(dx * dx + dy * dy);
}
}
return super.distance(pt);
}
@Override
public boolean equals(Object o) {
if (o instanceof AbstractFixpPoint) {
AbstractFixpPoint that = (AbstractFixpPoint)o;
return this.getFixpX() == that.getFixpX() && this.getFixpY() == that.getFixpY();
}
return super.equals(o);
}
@Override
public String toString() {
return getClass().getSimpleName() + "["+getX()+", "+getY()+"]";
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy