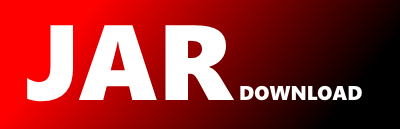
com.sun.jsftemplating.component.factory.tree.TreeAdaptorBase Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://jsftemplating.dev.java.net/cddl1.html or
* jsftemplating/cddl1.txt.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at jsftemplating/cddl1.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
package com.sun.jsftemplating.component.factory.tree;
import java.util.List;
import java.util.Map;
import javax.faces.component.UIComponent;
import com.sun.jsftemplating.layout.descriptors.LayoutComponent;
import com.sun.jsftemplating.layout.descriptors.handler.Handler;
/**
* This class provides some of the implemenation for the methods required
* by the TreeAdaptor interface. This class may be extended to assist in
* implementing a TreeAdaptor implementation.
*
* The TreeAdaptor
implementation must have a public
* static TreeAdaptor getInstance(FacesContext, LayoutComponent,
* UIComponent)
method in order to get access to an instance of the
* TreeAdaptor
instance.
*
* @see TreeAdaptor
*
* @author Ken Paulsen ([email protected])
*/
public abstract class TreeAdaptorBase implements TreeAdaptor {
/**
* This Constructor does nothing. If you need to store a reference
* to the LayoutComponent
or UIComponent
* associated with this TreeAdaptor
, it may be more
* convenient to use a different constructor.
*/
protected TreeAdaptorBase() {
}
/**
* This Constructor save the LayoutComponent
and the
* UIComponent
for easy use later.
*/
protected TreeAdaptorBase(LayoutComponent desc, UIComponent parent) {
setLayoutComponent(desc);
setParentUIComponent(parent);
}
/**
* This method retrieves the LayoutComponent
associated
* with this TreeAdaptor
.
*/
public LayoutComponent getLayoutComponent() {
return _layoutComponent;
}
/**
* This method sets the LayoutComponent
associated
* with this TreeAdaptor
.
*/
public void setLayoutComponent(LayoutComponent comp) {
_layoutComponent = comp;
}
/**
* This method retrieves the UIComponent
associated
* with this TreeAdaptor
.
*/
public UIComponent getParentUIComponent() {
return _parent;
}
/**
* This method sets the UIComponent
associated with this
* TreeAdaptor
.
*/
public void setParentUIComponent(UIComponent comp) {
_parent = comp;
}
/**
* This method is called shortly after
* getInstance(FacesContext, LayoutComponent, UIComponent). It
* provides a place for post-creation initialization to take occur.
*
* This implemenation does nothing.
*/
public void init() {
}
/**
* Returns the model object for the top TreeNode
, this
* may contain sub TreeNode
s.
*
* This implementation returns the value that was supplied by
* {@link #setTreeNodeObject(Object)}. If that method is not explicitly
* called, then this implementation will return null.
*/
public Object getTreeNodeObject() {
return _topNodeObject;
}
/**
* This method stores the top tree node model object.
*/
public void setTreeNodeObject(Object nodeObject) {
_topNodeObject = nodeObject;
}
/**
* This method returns the UIComponent
factory class
* implementation that should be used to create a
* TreeNode
for the given tree node model object.
*/
public String getFactoryClass(Object nodeObject) {
return "com.sun.jsftemplating.component.factory.sun.TreeNodeFactory";
}
/**
* This method returns any facets that should be applied to the
* TreeNode (comp)
. Useful facets for the sun
* TreeNode
component are: "content" and "image".
*
* Facets that already exist on comp
, or facets that
* are directly added to comp
do not need to be returned
* from this method.
*
* @param nodeObject The (model) object representing the tree node.
*/
public Map getFacets(Object nodeObject) {
return null;
}
/**
* Advanced framework feature which provides better handling for
* things such as expanding TreeNodes, beforeEncode, and other
* events.
*
* This method should return a Map
of List
* of Handler
objects. Each List
in the
* Map
should be registered under a key that cooresponds
* to to the "event" in which the Handler
s should be
* invoked.
*
* This implementation returns null. This method must be overriden
* to take advantage of this feature.
*/
public Map> getHandlersByType(UIComponent comp, Object nodeObject) {
return null;
}
private Object _topNodeObject = null;
private LayoutComponent _layoutComponent = null;
private UIComponent _parent = null;
}