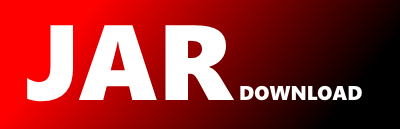
com.sun.jsftemplating.el.PageSessionResolver Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://jsftemplating.dev.java.net/cddl1.html or
* jsftemplating/cddl1.txt.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at jsftemplating/cddl1.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
package com.sun.jsftemplating.el;
import java.io.Serializable;
import java.util.HashMap;
import java.util.Map;
import javax.faces.component.UIViewRoot;
import javax.faces.context.FacesContext;
import javax.faces.el.EvaluationException;
import javax.faces.el.VariableResolver;
/**
* This VariableResolver
exists to resolve "page session"
* attributes. This concept, borrowed from NetDynamics / JATO, stores
* data w/ the page so that it is available throughout the life of the
* page. This is longer than request scope, but usually shorter than
* session. This implementation stores the attributes on the
* UIViewRoot
.
*
* @author Ken Paulsen ([email protected])
*/
public class PageSessionResolver extends VariableResolver {
/**
* Constructor.
*/
public PageSessionResolver(VariableResolver orig) {
super();
_origVariableResolver = orig;
}
/**
* This first delegates to the original VariableResolver
,
* it then checks "page session" to see if the value exists.
*/
public Object resolveVariable(FacesContext context, String name) throws EvaluationException {
Object result = null;
// Check to see if expression explicitly asks for PAGE_SESSION
if (name.equals(PAGE_SESSION)) {
// It does, return the Map
UIViewRoot root = context.getViewRoot();
result = getPageSession(context, root);
if (result == null) {
// No Map! That's ok, create one...
result = createPageSession(context, root);
}
} else {
if (_origVariableResolver != null) {
// Not explicit, let original resolver do its thing first...
result = _origVariableResolver.resolveVariable(context, name);
}
if (result == null) {
// Original resolver couldn't find anything, check page session
Map map =
getPageSession(context, (UIViewRoot) null);
if (map != null) {
result = map.get(name);
}
}
}
return result;
}
/**
* This method provides access to the "page session"
* Map
. If it doesn't exist, it returns
* null
. If the given UIViewRoot
is null,
* then the current UIViewRoot
will be used.
*/
public static Map getPageSession(FacesContext ctx, UIViewRoot root) {
if (root == null) {
root = ctx.getViewRoot();
}
return (Map)
root.getAttributes().get(PAGE_SESSION_KEY);
}
/**
* This method will create a new "page session" Map
. It
* will overwrite any existing "page session" Map
, so be
* careful.
*/
public static Map createPageSession(FacesContext ctx, UIViewRoot root) {
if (root == null) {
root = ctx.getViewRoot();
}
// Create it...
Map map = new HashMap(4);
// Store it...
root.getAttributes().put(PAGE_SESSION_KEY, map);
// Return it...
return map;
}
/**
* The original VariableResolver
.
*/
private VariableResolver _origVariableResolver = null;
/**
* The attribute key in which to store the "page" session Map.
*/
private static final String PAGE_SESSION_KEY = "_ps";
/**
* The name an expression must use when it explicitly specifies page
* session. ("pageSession")
*/
public static final String PAGE_SESSION = "pageSession";
}