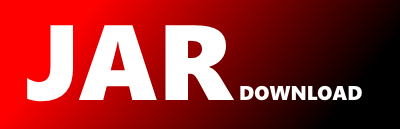
com.sun.jsftemplating.handlers.MetaDataHandlers Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://jsftemplating.dev.java.net/cddl1.html or
* jsftemplating/cddl1.txt.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at jsftemplating/cddl1.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
/*
* MetaDataHandlers.java
*
* Created on December 2, 2004, 3:06 AM
*/
package com.sun.jsftemplating.handlers;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import com.sun.jsftemplating.annotation.Handler;
import com.sun.jsftemplating.annotation.HandlerInput;
import com.sun.jsftemplating.annotation.HandlerOutput;
import com.sun.jsftemplating.layout.LayoutDefinitionManager;
import com.sun.jsftemplating.layout.ViewRootUtil;
import com.sun.jsftemplating.layout.descriptors.ComponentType;
import com.sun.jsftemplating.layout.descriptors.LayoutComponent;
import com.sun.jsftemplating.layout.descriptors.LayoutDefinition;
import com.sun.jsftemplating.layout.descriptors.handler.HandlerContext;
import com.sun.jsftemplating.layout.descriptors.handler.HandlerDefinition;
/**
* This class contains
* {@link com.sun.jsftemplating.layout.descriptors.handler.Handler}
* methods that perform common utility-type functions.
*
* @author Ken Paulsen ([email protected])
*/
public class MetaDataHandlers {
/**
* Default Constructor.
*/
public MetaDataHandlers() {
}
/**
* This handler provides information about all known (global)
* {@link com.sun.jsftemplating.layout.descriptors.handler.Handler}s.
* It allows an input value ("id") to be passed in, this is optional.
* If the value is supplied, it will return information about that
* handler only. If not supplied, it will return information about
* all handlers. Output is passed via output values "info", "ids",
* and "handler". "info" is always returned and contains a
* String
of information. "ids" is always returned and
* contains a Set
of global {@link HandlerDefinition}
* ids that may be passed into this method. "handler" is returned
* only if an id was specified and will contain the requested
* {@link HandlerDefinition}.
*/
@Handler(id="getGlobalHandlerInformation",
input={
@HandlerInput(name="id", type=String.class, required=false)
},
output={
@HandlerOutput(name="info", type=String.class),
@HandlerOutput(name="ids", type=Set.class),
@HandlerOutput(name="value", type=HandlerDefinition.class)
})
public static void getGlobalHandlerInformation(HandlerContext context) {
// Get the known global HandlerDefinitions
Map defs =
new TreeMap(LayoutDefinitionManager.getGlobalHandlerDefinitions());
// Provide a Set of ids
context.setOutputValue("ids", defs.keySet());
// If a single HandlerDefinition was requested, provide it
// Produce a String of information also
String key = (String) context.getInputValue("id");
if (key != null) {
context.setOutputValue("value", defs.get(key));
context.setOutputValue("info", defs.get(key).toString());
} else {
Iterator it = defs.values().iterator();
StringBuffer buf = new StringBuffer("");
while (it.hasNext()) {
buf.append(it.next().toString());
}
context.setOutputValue("info", buf.toString());
}
}
/**
* This handler provides information about all known (global)
* {@link ComponentType}s. It allows an input value ("id") to be
* passed in, this is optional. If the value is supplied, it will
* return information about that {@link ComponentType} only. If not
* supplied, it will return information about all
* {@link ComponentType}s. Output is passed via output values
* "info", "ids", and "value". "info" is always returned and
* contains a String
of information. "ids" is always
* returned and contains a Set
of global
* {@link ComponentType} ids that may be passed into this method.
* "value" is returned only if an id was specified and will contain
* the requested {@link ComponentType}.
*/
@Handler(id="getGlobalComponentTypeInformation",
input={
@HandlerInput(name="id", type=String.class, required=false)
},
output={
@HandlerOutput(name="info", type=String.class),
@HandlerOutput(name="ids", type=Set.class),
@HandlerOutput(name="value", type=ComponentType.class)
})
public static void getGlobalComponentTypeInformation(HandlerContext context) {
// Get the known global HandlerDefinitions
Map defs = new TreeMap(
LayoutDefinitionManager.getGlobalComponentTypes(
context.getFacesContext()));
// Provide a Set of ids
context.setOutputValue("ids", defs.keySet());
// If a single HandlerDefinition was requested, provide it
// Produce a String of information also
String key = (String) context.getInputValue("id");
if (key != null) {
// Return info for 1 ComponentType, return the type itself too
context.setOutputValue("value", defs.get(key));
context.setOutputValue("info", defs.get(key).toString());
} else {
// Return info for ALL ComponentTypes
Iterator it = defs.values().iterator();
StringBuffer buf = new StringBuffer("");
while (it.hasNext()) {
buf.append(it.next().toString());
}
context.setOutputValue("info", buf.toString());
}
}
/**
* This handler finds the (closest) requested
* {@link LayoutComponent} for the given viewId
/
* clientId
. If the viewId
is not supplied,
* the current UIViewRoot
will be used. The
* {@link LayoutComponent} is returned via the component
* output parameter. If an exact match is not found, it will return
* the last {@link LayoutComponent} found while searching the tree --
* this should be the last {@link LayoutComponent} in the hierarchy
* of the specified component.
*
* This is not an easy process since JSF components may not all be
* NamingContainer
s, so the clientId is not sufficient to
* find it. This is unfortunate, but we we have to deal with it.
*/
@Handler(id="getLayoutComponent",
input={
@HandlerInput(name="viewId", type=String.class, required=false),
@HandlerInput(name="clientId", type=String.class, required=true)},
output={
@HandlerOutput(name="component", type=LayoutComponent.class)})
public static void getLayoutComponent(HandlerContext ctx) {
// First get the clientId that we are going to attempt to walk.
String viewId = (String) ctx.getInputValue("viewId");
String clientId = (String) ctx.getInputValue("clientId");
// Next, find it
LayoutComponent result = LayoutDefinitionManager.getLayoutComponent(
ctx.getFacesContext(), viewId, clientId);
// Set the result
ctx.setOutputValue("component", result);
}
/**
* This handler provides a way to get a {@link LayoutDefinition}.
*/
@Handler(id="getLayoutDefinition",
input={
@HandlerInput(name="viewId", type=String.class, required=false)},
output={
@HandlerOutput(name="layoutDefinition", type=LayoutDefinition.class)})
public static void getLayoutDefinition(HandlerContext ctx) {
// First get the viewId...
String viewId = (String) ctx.getInputValue("viewId");
// Find the LayoutDefinition
LayoutDefinition def = ViewRootUtil.getLayoutDefinition(viewId);
// Set the result
ctx.setOutputValue("layoutDefinition", def);
}
/**
* This handler returns true if jsft is running in debug mode.
*/
@Handler(id="isDebug",
output={
@HandlerOutput(name="value", type=Boolean.class)})
public static void isDebug(HandlerContext ctx) {
// Set the result
ctx.setOutputValue("value",
LayoutDefinitionManager.isDebug(ctx.getFacesContext()));
}
}