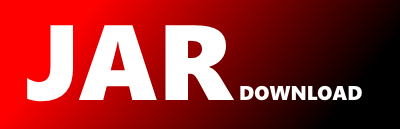
com.sun.jsftemplating.layout.descriptors.handler.HandlerDefinition Maven / Gradle / Ivy
/* * The contents of this file are subject to the terms * of the Common Development and Distribution License * (the License). You may not use this file except in * compliance with the License. * * You can obtain a copy of the license at * https://jsftemplating.dev.java.net/cddl1.html or * jsftemplating/cddl1.txt. * See the License for the specific language governing * permissions and limitations under the License. * * When distributing Covered Code, include this CDDL * Header Notice in each file and include the License file * at jsftemplating/cddl1.txt. * If applicable, add the following below the CDDL Header, * with the fields enclosed by brackets [] replaced by * you own identifying information: * "Portions Copyrighted [year] [name of copyright owner]" * * Copyright 2006 Sun Microsystems, Inc. All rights reserved. */ package com.sun.jsftemplating.layout.descriptors.handler; import com.sun.jsftemplating.util.Util; import java.lang.reflect.Method; import java.lang.reflect.Modifier; import java.util.ArrayList; import java.util.Formatter; import java.util.HashMap; import java.util.Iterator; import java.util.List; import java.util.Map; /** *
* public void beginDisplay(HandlerContext handlerCtx) * * *A HandlerDefinition defines a "handler" that may be invoked in the * process of executing an event. A HandlerDefinition has an * id, java method, input * definitions, output definitions, and * child handlers.
* *The java method to be invoked must have the * following method signature:
* *
* * @author Ken Paulsen ([email protected]) */ public class HandlerDefinition implements java.io.Serializable { /** * Constructor */ public HandlerDefinition(String id) { _id = id; } /** * This method returns the id for this handler. */ public String getId() { return _id; } /** * For future tool support */ public String getDescription() { return _description; } /** * For future tool support */ public void setDescription(String desc) { _description = desc; } /** *
void
above can return a value. Depending on the type of * event, return values may be handled differently.This method sets the event handler (method) to be invoked. The * method should be public and accept a prameter of type * "HandlerContext" Example:
* *
* public void beginDisplay(HandlerContext handlerCtx) ** * @param cls The full class name containing method * @param methodName The method name of the handler within class */ public void setHandlerMethod(String cls, String methodName) { if ((cls == null) || (methodName == null)) { throw new IllegalArgumentException( "Class name and method name must be non-null!"); } _methodClass = cls; _methodName = methodName; } /** * */ public void setHandlerMethod(Method method) { if (method != null) { _methodName = method.getName(); _methodClass = method.getDeclaringClass().getName(); } else { _methodName = null; _methodClass = null; } _method = method; } /** *This method determines if the handler is static.
*/ public boolean isStatic() { if (_static == null) { _static = Boolean.valueOf( Modifier.isStatic(getHandlerMethod().getModifiers())); } return _static.booleanValue(); } /** * */ public Method getHandlerMethod() { if (_method != null) { // return cached Method return _method; } // See if we have the info to find it if ((_methodClass != null) && (_methodName != null)) { // Find the class Class clzz = null; try { clzz = Util.loadClass(_methodClass, _methodClass); } catch (ClassNotFoundException ex) { throw new RuntimeException("'" + _methodClass + "' not found for method '" + _methodName + "'!", ex); } // Find the method on the class Method method = null; try { method = clzz.getMethod(_methodName, EVENT_ARGS); } catch (NoSuchMethodException ex) { throw new RuntimeException( "Method '" + _methodName + "' not found!", ex); } // Cache the _method _method = method; } // Return the Method if there is one return _method; } /** * This method adds an IODescriptor to the list of input descriptors. * These descriptors define the input parameters to this handler. * * @param desc The input IODescriptor to add */ public void addInputDef(IODescriptor desc) { _inputDefs.put(desc.getName(), desc); } /** * This method sets the input IODescriptors for this handler. * * @param inputDefs The Map of IODescriptors */ public void setInputDefs(MapinputDefs) { if (inputDefs == null) { throw new IllegalArgumentException( "inputDefs cannot be null!"); } _inputDefs = inputDefs; } /** * This method retrieves the Map of input IODescriptors. * * @return The Map of IODescriptors */ public Map getInputDefs() { return _inputDefs; } /** * This method returns the requested IODescriptor, null if not found. */ public IODescriptor getInputDef(String name) { return _inputDefs.get(name); } /** * This method adds an IODescriptor to the list of output descriptors. * These descriptors define the output parameters to this handler. * * @param desc The IODescriptor to add */ public void addOutputDef(IODescriptor desc) { _outputDefs.put(desc.getName(), desc); } /** * This method sets the output IODescriptors for this handler. * * @param outputDefs The Map of output IODescriptors */ public void setOutputDefs(Map outputDefs) { if (outputDefs == null) { throw new IllegalArgumentException( "outputDefs cannot be null!"); } _outputDefs = outputDefs; } /** * This method retrieves the Map of output IODescriptors. * * @return The Map of output IODescriptors */ public Map getOutputDefs() { return _outputDefs; } /** * This method returns the requested IODescriptor, null if not found. */ public IODescriptor getOutputDef(String name) { return _outputDefs.get(name); } /** * This method adds a {@link Handler} to the list of child * {@link Handler}s. Child {@link Handler}s are executed AFTER this * {@link Handler} is executed.
* * @param desc The {@link Handler} to add. */ public void addChildHandler(Handler desc) { if (_childHandlers == _emptyList) { _childHandlers = new ArrayList(); } _childHandlers.add(desc); } /** *This method sets the
List
of child {@link Handler}s * for thisHandlerDefinition
. * * @param childHandlers TheList
of child * {@link Handler}s. */ public void setChildHandlers(ListchildHandlers) { if ((childHandlers == null) || (childHandlers.size() == 0)) { childHandlers = _emptyList; } _childHandlers = childHandlers; } /** * This method retrieves the
* * @return TheList
of child * {@link Handler}s. ThisList
should not be changed * directly. Call {@link #addChildHandler(Handler)}, or make a copy * and call {@link #setChildHandlers(List)}.List
of child {@link Handler}s for this *HandlerDefinition
. */ public ListgetChildHandlers() { return _childHandlers; } /** * This toString() provides detailed information about this *
*/ public String toString() { // Print the basic info... Formatter printf = new Formatter(); printf.format("%-40s %s.%s\n", _id, _methodClass, _methodName); // Print the description if (_description != null) { printf.format("%s\n", _description); } // Print the Inputs IteratorHandlerDefinition
.it = _inputDefs.values().iterator(); while (it.hasNext()) { printf.format(" INPUT> %s\n", it.next().toString()); } // Print the Outputs it = _outputDefs.values().iterator(); while (it.hasNext()) { printf.format(" OUTPUT> %s\n", it.next().toString()); } // Print the Child Handlers (TBD...) // Return the result return printf.toString(); } public static final Class[] EVENT_ARGS = new Class[] {HandlerContext.class}; private String _id = null; private String _description = null; private String _methodClass = null; private String _methodName = null; private transient Method _method = null; private Map _inputDefs = new HashMap (5); private Map _outputDefs = new HashMap (5); private List _childHandlers = _emptyList; private transient Boolean _static = null; private static final List _emptyList = new ArrayList (0); private static final long serialVersionUID = 0xA8B7C6D5E4F30211L; }