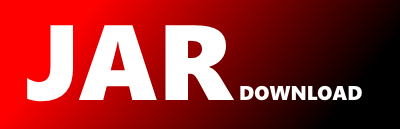
com.sun.jsftemplating.resource.ResourceBundleManager Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://jsftemplating.dev.java.net/cddl1.html or
* jsftemplating/cddl1.txt.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at jsftemplating/cddl1.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
package com.sun.jsftemplating.resource;
import com.sun.jsftemplating.util.Util;
import java.util.HashMap;
import java.util.Locale;
import java.util.Map;
import java.util.MissingResourceException;
import java.util.ResourceBundle;
import javax.faces.context.FacesContext;
/**
* This class caches ResourceBundle
objects per locale.
*
* @author Ken Paulsen ([email protected])
*/
public class ResourceBundleManager {
/**
* Use {@link #getInstance()} to obtain an instance.
*/
protected ResourceBundleManager() {
}
/**
* Use this method to get the instance of this class.
*
* @deprecated Use ResourceBundleManager#getInstance(FacesContext).
*/
public static ResourceBundleManager getInstance() {
return getInstance(null);
}
/**
* Use this method to get the instance of this class.
*/
public static ResourceBundleManager getInstance(FacesContext ctx) {
if (ctx == null) {
ctx = FacesContext.getCurrentInstance();
}
ResourceBundleManager mgr = null;
if (ctx != null) {
// Look in application scope for it...
mgr = (ResourceBundleManager) ctx.getExternalContext().
getApplicationMap().get(RB_MGR);
}
if (mgr == null) {
// 1st time... create / initialize it
mgr = new ResourceBundleManager();
if (ctx != null) {
ctx.getExternalContext().getApplicationMap().put(RB_MGR, mgr);
}
}
// Return the map...
return mgr;
}
/**
* This method checks the cache for the requested
* ResourceBundle
.
*
* @param baseName Name of the bundle.
* @param locale The Locale
.
*
* @return The requested ResourceBundle
in the most
* appropriate Locale
.
*/
protected ResourceBundle getCachedBundle(String baseName, Locale locale) {
return (ResourceBundle) _cache.get(getCacheKey(baseName, locale));
}
/**
* This method generates a unique key for setting / getting
* ResourceBundle
s from the cache. It is important to
* have different keys per locale (obviously).
*/
protected String getCacheKey(String baseName, Locale locale) {
return baseName + "__" + locale.toString();
}
/**
* This method adds a ResourceBundle
to the cache.
*/
protected void addCachedBundle(String baseName, Locale locale, ResourceBundle bundle) {
// Copy the old Map to prevent changing a Map while someone is
// accessing it.
Map map = new HashMap(_cache);
// Add the new bundle
map.put(getCacheKey(baseName, locale), bundle);
// Set this new Map as the shared cache Map
_cache = map;
}
/**
* This method obtains the requested ResourceBundle
as
* specified by the given basename
and
* locale
.
*
* @param baseName The base name for the ResourceBundle
.
* @param locale The desired Locale
.
*/
public ResourceBundle getBundle(String baseName, Locale locale) {
ResourceBundle bundle = getCachedBundle(baseName, locale);
if (bundle == null) {
try {
bundle = ResourceBundle.getBundle(baseName, locale,
Util.getClassLoader(baseName));
} catch (MissingResourceException ex) {
// Use System.out.println b/c we don't want infinite loop and
// we're too lazy to do more...
System.out.println("Can't find bundle: " + baseName);
ex.printStackTrace();
}
if (bundle != null) {
addCachedBundle(baseName, locale, bundle);
}
}
return bundle;
}
/**
* This method obtains the requested ResourceBundle as
* specified by the given basename, locale, and classloader.
*
* @param baseName The base name for the ResourceBundle
.
* @param locale The desired Locale
.
* @param loader The ClassLoader
that should be used.
*/
public ResourceBundle getBundle(String baseName, Locale locale, ClassLoader loader) {
ResourceBundle bundle = getCachedBundle(baseName, locale);
if (bundle == null) {
bundle = ResourceBundle.getBundle(baseName, locale, loader);
if (bundle != null) {
addCachedBundle(baseName, locale, bundle);
}
}
return bundle;
}
/**
* Application scope key which stores the
* ResourceBundleManager
instance for this
* application.
*/
private static final String RB_MGR = "__jsft_ResourceBundleMgr";
/**
* The cache of ResourceBundle
s.
*/
private Map _cache = new HashMap();
}