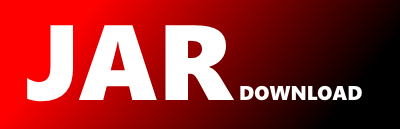
com.sun.jsftemplating.util.IncludeInputStream Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://jsftemplating.dev.java.net/cddl1.html or
* jsftemplating/cddl1.txt.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at jsftemplating/cddl1.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
package com.sun.jsftemplating.util;
import java.io.BufferedInputStream;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FilterInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URL;
/**
* This InputStream
looks for lines beginning with
* "#include 'filename'" where filename is the name of a file to
* include. It replaces the "#include" line with contents of the
* specified file. Any other line beginning with '#' is illegal.
*
* @author Ken Paulsen ([email protected])
*/
public class IncludeInputStream extends FilterInputStream {
/**
* Constructor.
*/
public IncludeInputStream(InputStream input) {
super(input);
}
/**
* This overriden method implements the include feature.
*
* @return The next character.
*/
public int read() throws IOException {
int intChar = -1;
if (redirStream != null) {
// We are already redirecting, delegate
intChar = redirStream.read();
if (intChar != -1) {
return intChar;
}
// Found end of redirect file, stop delegating
redirStream = null;
}
// Read next character
intChar = super.read();
char ch = (char) intChar;
// If we were at the end of the line, check for new line w/ #
if (eol) {
// Check to see if we have a '#'
if (ch == '#') {
intChar = startInclude();
} else {
eol = false;
}
}
// Flag EOL if we're at the end of a line
if ((ch == 0x0A) || (ch == 0x0D)) {
eol = true;
}
return intChar;
}
public int available() throws IOException {
return 0;
}
public boolean markSupported() {
return false;
}
public int read(byte[] bytes, int off, int len) throws IOException {
if (bytes == null) {
throw new NullPointerException();
} else if ((off < 0) || (off > bytes.length) || (len < 0)
|| ((off + len) > bytes.length) || ((off + len) < 0)) {
throw new IndexOutOfBoundsException();
} else if (len == 0) {
return 0;
}
int c = read();
if (c == -1) {
return -1;
}
bytes[off] = (byte) c;
int i = 1;
try {
for (; i < len; i++) {
c = read();
if (c == -1) {
break;
}
if (bytes != null) {
bytes[off + i] = (byte) c;
}
}
} catch (IOException ee) {
ee.printStackTrace();
}
return i;
}
/**
*
*/
private int startInclude() throws IOException {
// Mark this spot in case #include doesn't match
mark(MARK_LIMIT);
// We have a line beginning w/ '#', verify we have "#include"
int ch;
for (int count = 0; count < INCLUDE_LEN; count++) {
// look for include
ch = super.read();
if (Character.toLowerCase((char) ch) != INCLUDE.charAt(count)) {
reset(); // Restore stream position
return '#';
}
}
// Skip whitespace...
ch = super.read();
while ((ch == ' ') || (ch == '\t')) {
ch = super.read();
}
// Skip '"' or '\''
if ((ch == '"') || (ch == '\'')) {
ch = super.read();
}
// Read the file name
StringBuffer buf = new StringBuffer("");
while ((ch != '"')
&& (ch != '\'')
&& (ch != 0x0A)
&& (ch != 0x0D)
&& (ch != -1)) {
buf.append((char ) ch);
ch = super.read();
}
// Skip ending '"' or '\'', if any
if ((ch == '"') || (ch == '\'')) {
ch = super.read();
}
// Get the file name...
String filename = buf.toString();
// Look for the file
URL url = FileUtil.searchForFile(filename, null);
if (url != null) {
redirStream = new IncludeInputStream(
new BufferedInputStream(url.openStream()));
} else {
// Throw a FnF exception...
throw new FileNotFoundException(filename);
}
// Read the first character from the file to return
return redirStream.read();
}
/**
* Simple test case (requires a test file).
*/
public static void main(String[] args) {
try {
IncludeInputStream stream =
new IncludeInputStream(new FileInputStream(args[0]));
int ch = '\n';
while (ch != -1) {
System.out.print((char) ch);
ch = stream.read();
}
} catch (Exception ex) {
ex.printStackTrace();
}
}
private boolean eol = true;
private IncludeInputStream redirStream = null;
private static final String INCLUDE = "include";
private static final int INCLUDE_LEN = INCLUDE.length();
private static final int MARK_LIMIT = 128;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy