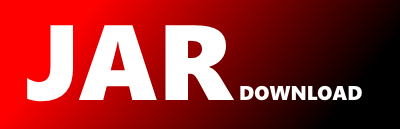
com.sun.jsftemplating.util.fileStreamer.Context Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://jsftemplating.dev.java.net/cddl1.html or
* jsftemplating/cddl1.txt.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at jsftemplating/cddl1.txt.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2006 Sun Microsystems, Inc. All rights reserved.
*/
package com.sun.jsftemplating.util.fileStreamer;
import java.io.IOException;
import java.io.OutputStream;
import java.util.Set;
/**
* This interface provides API's to encapsulate environment specific
* objects so that the FileStreamer class is not specific to a specific
* environment (like a Servlet environment).
*/
public interface Context {
/**
* Accessor to get the {@link FileStreamer} instance.
*/
public FileStreamer getFileStreamer();
/**
* This method locates the appropriate {@link ContentSource} for this
* Context
.
*/
public ContentSource getContentSource();
/**
* This method allows the Context to restrict access to resources.
* It returns true
if the user is allowed to view the
* resource. It returns false
if the user should not
* be allowed access to the resource.
*/
public boolean hasPermission(ContentSource src);
/**
* This method is responsible for setting the response header
* information.
*/
public void writeHeader(ContentSource source) throws IOException;
/**
* This method is responsible for sending an error.
*/
public void sendError(int code, String msg) throws IOException;
/**
* This method is responsible for returning an
* OutputStream
suitable for writing content.
*/
public OutputStream getOutputStream() throws IOException;
/**
* This method may be used to manage arbitrary information between the
* code invoking the {@link FileStreamer} and the
* ContentSource
. This method retrieves an attribute.
*
* See individual {@link ContentSource} implementations for more
* details on supported / required attributes.
*/
public Object getAttribute(String name);
/**
* This provides access to all attributes in this Context.
*
* See individual {@link ContentSource} implementations for more
* details on supported / required attributes.
*/
public Set getAttributeKeys();
/**
* This method may be used to manage arbitrary information between the
* code invoking the {@link FileStreamer} and the
* ContentSource
. This method sets an attribute.
*
* See individual {@link ContentSource} implementations for more
* details on supported / required attributes.
*/
public void setAttribute(String name, Object value);
/**
* This method may be used to manage arbitrary information between the
* coding invoking the {@link FileStreamer} and the
* ContentSource
. This method removes an attribute.
*
* See individual {@link ContentSource} implementations for more
* details on supported / required attributes.
*/
public void removeAttribute(String name);
/**
* This is the number of milliseconds into the future until the
* content should expire. This constant is currently set at about 7
* years.
*/
public static final long EXPIRY_TIME = 1000 * 60 * 60 * 24 * 365 * 7;
/**
* This is the {@link Context} attribute name used to
* specify the filename extension of the content. It is the
* responsibility of the {@link ContentSource} to set
* this value. The value should represent the filename extension of
* the content if it were saved to a filesystem.
*/
public static final String EXTENSION = "extension";
/**
* The Content-type ("ContentType"). This is the {@link Context}
* attribute used to specify an explicit "Content-type". It may be
* set by the {@link ContentSource}. If not specified, the
* {@link #EXTENSION} will typically be used (if possible). If that
* fails, the {@link FileStreamer#getDefaultMimeType} is used.
*/
public static final String CONTENT_TYPE = "ContentType";
/**
* The value for the "Content-Disposition" ("disposition"). This is
* the {@link Context} attribute used to specify the content
* disposition. The content disposition tells the browser how to
* handle the content. The two standard values for this are:
*
* - inline
* - attachment
*
* See RFC 2183 for more information. This value may be set by the
* {@link ContentSource}. If not specified, nothing will be set.
* This value may be used in conjunction with the
* {@link #CONTENT_FILENAME} attribute, or the entire content
* disposition may be specified with this attribute.
*/
public static final String CONTENT_DISPOSITION = "disposition";
/**
* The value for the "Content-Disposition" ("filename"). This is the
* {@link Context} attribute used to specify an explicit "filename".
* It may be set by the {@link ContentSource}. If not specified,
* nothing will be set. If {@link #CONTENT_DISPOSITION} is also set,
* this method will append the file name. If not set, it will set
* the contentDisposition to "attachment".
*/
public static final String CONTENT_FILENAME = "filename";
/**
* This is the path of the requested file ("filename"). It is the
* responsibility of the {@link Context} implementation to provide
* this information as an attribute.
*/
public static final String FILE_PATH = "filePath";
/**
* This is the parameter that may be provided to identify the
* {@link ContentSource} implementation to be used. This value must
* match the value returned by the ContentSource
* implementation's getId()
method. It is typical for
* {@link Context} implementations to allow this to be specified by a
* HttpServletRequest
parameter.
*/
public static final String CONTENT_SOURCE_ID = "contentSourceId";
/**
* This String ("ContentSources") is the name of the
* Servlet
init parameter or context param (depending
* on environment implementation) that should be used to register
* all available {@link ContentSource} implementations. This should
* be a list of full classnames of the {@link ContentSource}s.
*/
public static final String CONTENT_SOURCES = "ContentSources";
/**
* This String ("com.sun.jsftemplating.FS_ALLOW_PATHS") is the name of
* the Servlet
init parameter or context param (depending
* on environment implementation) that should be used to register all
* valid paths for resources to be streamed by {@link FileStreamer}.
* This should be specified as a comma (or semi-colon) separated list
* of path prefixes. Paths are relative to the context root of the
* application. Any path starting with one of the paths in this list
* may be served using FileStreamer (provided it is not explicitly
* excluded via {@link #DENY_PATHS}). Leading "/" characters maybe
* specified, but will be removed when doing a comparison. A value of
* "/" will provide access to the entire application, which is the
* default value if not specified.
*/
public static final String ALLOW_PATHS = "com.sun.jsftemplating.FS_ALLOW_PATHS";
/**
* This String ("com.sun.jsftemplating.FS_DENY_PATHS") is the name of
* the Servlet
init parameter or context param (depending
* on environment implementation) that should be used to register all
* valid paths for resources to be streamed by {@link FileStreamer}.
* This should be specified as a comma (or semi-colon) separated list
* of path prefixes. Paths are relative to the context root of the
* application. Any path starting with one of the paths in this list
* may NOT be served using FileStreamer. Leading '/'
* characters maybe specified, but will be removed when doing a
* comparison. The default value is:
* "META-INF/,WEB-INF/
".
*/
public static final String DENY_PATHS = "com.sun.jsftemplating.FS_DENY_PATHS";
/**
* This is the id of the default {@link ContentSource}. This is set
* to the id of the {@link ResourceContentSource}.
*/
public static final String DEFAULT_CONTENT_SOURCE_ID =
ResourceContentSource.ID;
}