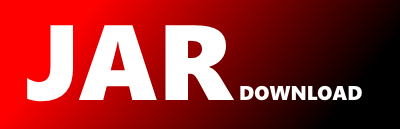
com.sun.webui.jsf.renderkit.html.RadioButtonGroupRenderer Maven / Gradle / Ivy
/*
* The contents of this file are subject to the terms
* of the Common Development and Distribution License
* (the License). You may not use this file except in
* compliance with the License.
*
* You can obtain a copy of the license at
* https://woodstock.dev.java.net/public/CDDLv1.0.html.
* See the License for the specific language governing
* permissions and limitations under the License.
*
* When distributing Covered Code, include this CDDL
* Header Notice in each file and include the License file
* at https://woodstock.dev.java.net/public/CDDLv1.0.html.
* If applicable, add the following below the CDDL Header,
* with the fields enclosed by brackets [] replaced by
* you own identifying information:
* "Portions Copyrighted [year] [name of copyright owner]"
*
* Copyright 2007 Sun Microsystems, Inc. All rights reserved.
*/
package com.sun.webui.jsf.renderkit.html;
import com.sun.faces.annotation.Renderer;
import java.io.IOException;
import javax.faces.component.UIComponent;
import javax.faces.context.FacesContext;
import javax.faces.context.ResponseWriter;
import com.sun.webui.jsf.component.RadioButton;
import com.sun.webui.jsf.component.RadioButtonGroup;
import com.sun.webui.jsf.model.Option;
import com.sun.webui.theme.Theme;
import com.sun.webui.jsf.theme.ThemeStyles;
import com.sun.webui.jsf.util.ConversionUtilities;
import com.sun.webui.jsf.util.ThemeUtilities;
/**
* The RadioButtonRenderer
renders a RadioButtonGroup
* component as set of radio buttons. The RadioButtonGroupRenderer
* creates an instance of RadioButton
for each
* Option
instance in the Array
, Map
, or
* Collection
returned by the RadioButtonGroup
* getItems()
method and renders them. It also
* creates a Label
component and renders it as the label for the
* group.
*
* Only one radio button may be selected at any time and one radio button
* must always be selected. The value of the RadioButtonGroup
* will determine which radio button shall be initially selected.
* Subsequently, the RadioButtonGroup
's value holds the
* currently selected radio button value.
*
*
* The radio buttons are rendered as a single column or some number of
* rows and columns. The rows and columns are rendered as a table as
* defined by the {@link com.sun.webui.jsf.renderkit.html.RowColumnRenderer} superclass.
* The elements
* that make up the radio button occupy a cell in the table.
* The style class selector for the group elements is identified by a java
* constants defined in the {@link com.sun.webui.jsf.theme.ThemeStyles} class.
*
*
* - RADIOBUTTON_GROUP for the TABLE element.
* - RADIOBUTTON_GROUP_CAPTION for the TD element containing the group
* label
* - RADIOBUTTON_GROUP_LABEL for the LABEL element used as the CAPTION
* - RADIOBUTTON_GROUP_LABEL_DISABLED for the LABEL used as the CAPTION if
* the group is disabled
* - RADIOBUTTON_GROUP_ROW_EVEN for even TR elements
* - RADIOBUTTON_GROUP_ROW_ODD for odd TR elements
* - RADIOBUTTON_GROUP_CELL_EVEN for even TD elements
* - RADIOBUTTON_GROUP_CELL_ODD for odd TD elements
* - RADIOBUTTON for the INPUT element
* - RADIOBUTTON_DISABLED for the INPUT element for disabled radio
* button
* - RADIOBUTTON_LABEL for a LABEL element of a radio button
* - RADIOBUTTON_LABEL_DISABLED for a LABEL element of a disabled radio
* button
* - RADIOBUTTON_IMAGE for an IMG element of a radio button
* - RADIOBUTTON_IMAGE_DISABLED for an IMG element of a disabled radio
* button
*
*
* The name
property of each radio button is the component id of
* the RadioButtonGroup
instance. The id of a
* RadioButton
component is rbgrpid_N where
* rbgrpid is the id of the
* RadioButtonGroup
instance and _N is the nth
* radio button.
*
*
* The RadioButtonGroup
is decoded by identifying the
* RadioButtonGroup
instance component id which is
* returned as a request parameter. It represents the name attribute
* of the selected radio button's <input> element. The value of
* the identified request parameter is assigned as the submitted value of the
* RadioButtonGroup
component.
*
*
* If the items property of the RadioButtonGroup
is null or
* zero length no output is produced.
*
*
*/
@Renderer(@Renderer.Renders(componentFamily = "com.sun.webui.jsf.RadioButtonGroup"))
//FIXME check about making SelectGroupRenderer a public abstract class
public class RadioButtonGroupRenderer extends SelectorGroupRenderer {
private final String MSG_COMPONENT_NOT_RADIOBUTTONGROUP =
"RadioButtonGroupRender only renders RadioButtonGroup components."; //NOI18N
/**
* Creates a new instance of RadioButtonGroupRenderer
*/
public RadioButtonGroupRenderer() {
super();
}
/**
* Ensure that the component to be rendered is a RadioButtonGroup instance.
* Actual rendering occurs during renderEnd
*
* @param context FacesContext for the request we are processing.
* @param component UIComponent to be decoded.
*/
@Override
public void renderStart(FacesContext context, UIComponent component,
ResponseWriter writer)
throws IOException {
// Bail out if the component is not a RadioButtonGroup component.
if (!(component instanceof RadioButtonGroup)) {
throw new IllegalArgumentException(MSG_COMPONENT_NOT_RADIOBUTTONGROUP);
}
}
/**
* RadioButtonGroupRenderer renders the entire RadioButtonGroup
* component within the renderEnd method.
*
* @param context FacesContext for the request we are processing.
* @param component UIComponent to be decoded.
*/
@Override
public void renderEnd(FacesContext context, UIComponent component,
ResponseWriter writer)
throws IOException {
// Use only the cols value. If not valid render a single column.
// If there are more items than columns, render additional rows.
//
RadioButtonGroup rbgrp = (RadioButtonGroup) component;
Theme theme = ThemeUtilities.getTheme(context);
renderSelectorGroup(context, component, theme,
writer, rbgrp.getColumns());
}
/**
* Return a RadioButton component to render.
*
* @param context FacesContext
for the current request
* @param component CheckboxGroup
component rendered
* @param theme Theme
for the component
* @param option the Option
being rendered.
*/
protected UIComponent getSelectorComponent(FacesContext context,
UIComponent component, Theme theme, String id, Option option) {
RadioButtonGroup rbgrp = (RadioButtonGroup) component;
RadioButton rb = new RadioButton();
rb.setId(id);
rb.setParent(component);
rb.setName(rbgrp.getClientId(context));
rb.setToolTip(option.getTooltip());
rb.setImageURL(option.getImage());
rb.setSelectedValue(option.getValue());
rb.setLabel(option.getLabel());
rb.setDisabled(rbgrp.isDisabled());
rb.setReadOnly(rbgrp.isReadOnly());
rb.setTabIndex(rbgrp.getTabIndex());
// mbohm 6300361,6300362
// transfer event attributes from cbgrp to cb
// see RowColumnRenderer.renderRowColumnLayout
transferEventAttributes(rbgrp, rb);
// Default to not selected
//
rb.setSelected(null);
// Need to check the submittedValue for immediate condition
//
String[] subValue = (String[]) rbgrp.getSubmittedValue();
if (subValue == null) {
if (isSelected(option, rbgrp.getSelected())) {
rb.setSelected(rb.getSelectedValue());
}
} else if (subValue.length != 0) {
Object selectedValue = rb.getSelectedValue();
String selectedValueAsString =
ConversionUtilities.convertValueToString(component,
selectedValue);
if (subValue[0] != null &&
subValue[0].equals(selectedValueAsString)) {
rb.setSelected(rb.getSelectedValue());
}
}
return rb;
}
/**
* Return true if the item argument is the currently
* selected radio button. Equality is determined by the equals
* method of the object instance stored as the value
of
* item
. Return false otherwise.
*
* @param item the current radio button being rendered.
* @param currentValue the value of the current selected radio button.
*/
private boolean isSelected(Option item, Object currentValue) {
return currentValue != null && item.getValue() != null &&
item.getValue().equals(currentValue);
}
protected String[] styles = {
ThemeStyles.RADIOBUTTON_GROUP, /* GRP */
ThemeStyles.RADIOBUTTON_GROUP_CAPTION, /* GRP_CAPTION */
ThemeStyles.RADIOBUTTON_GROUP_LABEL, /* GRP_LABEL */
ThemeStyles.RADIOBUTTON_GROUP_LABEL_DISABLED, /* GRP_CAPTION_DIS */
ThemeStyles.RADIOBUTTON_GROUP_ROW_EVEN, /* GRP_ROW_EVEN */
ThemeStyles.RADIOBUTTON_GROUP_ROW_ODD, /* GRP_ROW_EVEN */
ThemeStyles.RADIOBUTTON_GROUP_CELL_EVEN,/* GRP_CELL_EVEN */
ThemeStyles.RADIOBUTTON_GROUP_CELL_ODD, /* GRP_CELL_ODD */
ThemeStyles.RADIOBUTTON, /* INPUT */
ThemeStyles.RADIOBUTTON_DISABLED, /* INPUT_DIS */
ThemeStyles.RADIOBUTTON_LABEL, /* LABEL */
ThemeStyles.RADIOBUTTON_LABEL_DISABLED, /* LABEL_DIS */
ThemeStyles.RADIOBUTTON_IMAGE, /* IMAGE */
ThemeStyles.RADIOBUTTON_IMAGE_DISABLED, /* IMAGE_DIS */
ThemeStyles.LABEL_LEVEL_ONE_TEXT, /* LABEL_LVL1 */
ThemeStyles.LABEL_LEVEL_TWO_TEXT, /* LABEL_LVL2 */
ThemeStyles.LABEL_LEVEL_THREE_TEXT /* LABLE_LVL3 */};
/**
* Return style constants for a RadioButton
component.
*/
protected String[] getStyles() {
return styles;
}
}