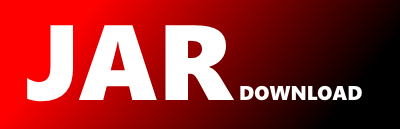
com.sun.msv.relaxns.verifier.IslandSchemaImpl Maven / Gradle / Ivy
/*
* Copyright (c) 2001-2013 Oracle and/or its affiliates. All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions
* are met:
*
* - Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
*
* - Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
*
* - Neither the name of Oracle nor the names of its
* contributors may be used to endorse or promote products derived
* from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS
* IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO,
* THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR
* PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT OWNER OR
* CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL,
* EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO,
* PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR
* PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF
* LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING
* NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package com.sun.msv.relaxns.verifier;
import java.util.Iterator;
import java.util.Map;
import java.util.Set;
import org.iso_relax.dispatcher.AttributesDecl;
import org.iso_relax.dispatcher.AttributesVerifier;
import org.iso_relax.dispatcher.ElementDecl;
import org.iso_relax.dispatcher.IslandSchema;
import org.iso_relax.dispatcher.IslandVerifier;
import org.iso_relax.dispatcher.SchemaProvider;
import org.xml.sax.ErrorHandler;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import com.sun.msv.grammar.AttributeExp;
import com.sun.msv.grammar.ElementExp;
import com.sun.msv.grammar.Expression;
import com.sun.msv.grammar.ExpressionCloner;
import com.sun.msv.grammar.ExpressionPool;
import com.sun.msv.grammar.Grammar;
import com.sun.msv.grammar.OtherExp;
import com.sun.msv.grammar.ReferenceContainer;
import com.sun.msv.grammar.ReferenceExp;
import com.sun.msv.relaxns.grammar.DeclImpl;
import com.sun.msv.relaxns.grammar.ExternalAttributeExp;
import com.sun.msv.relaxns.grammar.ExternalElementExp;
import com.sun.msv.verifier.regexp.REDocumentDeclaration;
/**
* base implementation of IslandSchema for MSV.
*
* the iso_relax package doesn't have the distinction between AGM and VGM.
* For the safety, the implementation of the createNewVerifier method creates
* a new VGM everytime it is called.
*
* Fortunately, when all island schemas are from MSV, the application can simply
* treat RELAXGrammar as a normal Grammar object; there is no need to use
* Dispatcher nor any divide-and-validate framework.
*
* So createNewVerifier method is called only when
*
* -
* MSV is used by other RELAX Namespace
* implementation or
*
-
* other IslandSchema implemntations are used by MSV's RELAXNSReader.
*
*
* In that case, the current createNewVerifier method causes a performance problem.
*
* @author Kohsuke KAWAGUCHI
*/
public abstract class IslandSchemaImpl
implements IslandSchema, java.io.Serializable {
/** map from name to DeclImpl. */
protected final Map elementDecls = new java.util.HashMap();
/** map from name to DeclImpl. */
protected final Map attributesDecls = new java.util.HashMap();
public IslandVerifier createNewVerifier( String namespace, ElementDecl[] rules ) {
// see the class comment.
// this method is invoked only under certain limited situations.
DeclImpl[] ri = new DeclImpl[rules.length];
System.arraycopy( rules,0, ri,0, rules.length );
return new TREXIslandVerifier(
new RulesAcceptor(
new REDocumentDeclaration( getGrammar() ), ri ) );
}
/** get the grammar object that represents this island. */
protected abstract Grammar getGrammar();
public ElementDecl getElementDeclByName( String name ) {
return (ElementDecl)elementDecls.get(name);
}
public Iterator iterateElementDecls() {
return elementDecls.values().iterator();
}
public ElementDecl[] getElementDecls() {
ElementDecl[] r = new DeclImpl[elementDecls.size()];
elementDecls.values().toArray(r);
return r;
}
public AttributesDecl getAttributesDeclByName( String name ) {
return (AttributesDecl)attributesDecls.get(name);
}
public Iterator iterateAttributesDecls() {
return attributesDecls.values().iterator();
}
public AttributesDecl[] getAttributesDecls() {
AttributesDecl[] r = new DeclImpl[attributesDecls.size()];
attributesDecls.values().toArray(r);
return r;
}
public AttributesVerifier createNewAttributesVerifier(
String namespaceURI, AttributesDecl[] decls ) {
throw new Error("not implemented");
}
protected void bind( ReferenceContainer con, Binder binder ) {
ReferenceExp[] exps = con.getAll();
for( int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy