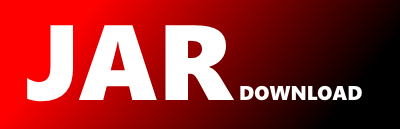
msv.tahiti.src.com.sun.tahiti.compiler.ll.Rules Maven / Gradle / Ivy
/*
* @(#)$Id: Rules.java 1087 2001-08-18 01:37:50Z Bear $
*
* Copyright 2001 Sun Microsystems, Inc. All Rights Reserved.
*
* This software is the proprietary information of Sun Microsystems, Inc.
* Use is subject to license terms.
*
*/
package com.sun.tahiti.compiler.ll;
import com.sun.msv.grammar.Expression;
import com.sun.msv.grammar.NameClassAndExpression;
import com.sun.tahiti.compiler.XMLWriter;
import com.sun.tahiti.compiler.Symbolizer;
import java.util.Iterator;
import java.util.Set;
import java.util.Map;
/**
* a set of rules.
*
* @author
* Kohsuke KAWAGUCHI
*/
public final class Rules {
/**
* set of all distinctive rules.
* This map can possibly contain unreachable object.
*/
private final Map distinctiveRules = new java.util.HashMap();
/**
* a map from left side symbol (Object) to the set of Rules.
*/
private final Map rules = new java.util.HashMap();
/** gets a Set that stores the values associated with the specified key. */
public Set get( Expression symbol ) {
Set s = (Set)rules.get(symbol);
if(s==null)
rules.put(symbol,s=new java.util.HashSet());
return s;
}
/** gets all values associated with the specified symbol. */
public Rule[] getAll( Expression symbol ) {
return (Rule[])get(symbol).toArray(new Rule[0]);
}
/** returns true if this container has a rule that expands the specified symbol
*/
public boolean contains( Expression symbol ) {
Set s = (Set)rules.get(symbol);
if(s==null) return false;
return s.size()!=0;
}
/** iterates non-terminal symbols in this rules. */
public Iterator iterateKeys() {
return rules.keySet().iterator();
}
Rule unifyRule( Rule r ) {
Rule unified = (Rule)distinctiveRules.get(r);
if(unified==null)
distinctiveRules.put( unified=r, r );
return unified;
}
/** adds a new rule. */
public void add( Expression left, Rule right ) {
get(left).add(unifyRule(right));
}
/** adds a new rule. */
public void add( Expression left, Expression[] right, boolean isInterleave ) {
add(left, new Rule(right,isInterleave));
}
/** adds a new rule. */
public void add( Expression left, Expression right ) {
add(left, new Expression[]{right}, false );
}
/** adds all rules. */
public void addAll( Expression left, Rule[] rules ) {
for( int i=0; i
* A rule which is unreachable from the specified rootSymbol
* will be removed.
*
* @param recurseElement
*
* if true, this method recursively visits element and attribute and
* discover all reachable rules. This is useful for global optimization.
*
*
* if set to false, this method treats element and attribute as terminal
* symbols. This is useful to extract per-element/per-attribute rules.
*/
public Rules removeUnreachableRules( Expression rootSymbol, boolean recurseElement ) {
Rules result = new Rules();
Set workQueue = new java.util.HashSet();
workQueue.add(rootSymbol);
while(!workQueue.isEmpty()) {
// get the first one in the queue.
Expression symbol = (Expression)workQueue.iterator().next();
workQueue.remove(symbol);
Rule[] rs = this.getAll(symbol);
assert(rs!=null && rs.length!=0);
result.addAll(symbol,rs);
for( int i=0; i