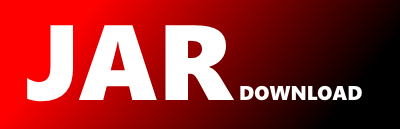
com.sun.tools.jxc.apt.SchemaGenerator Maven / Gradle / Ivy
Go to download
Old JAXB Binding Compiler. Contains source code needed for binding customization files into java sources.
In other words: the *tool* to generate java classes for the given xml representation.
package com.sun.tools.jxc.apt;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.xml.bind.SchemaOutputResolver;
import javax.xml.namespace.QName;
import javax.xml.transform.Result;
import javax.xml.transform.stream.StreamResult;
import com.sun.mirror.apt.AnnotationProcessor;
import com.sun.mirror.apt.AnnotationProcessorEnvironment;
import com.sun.mirror.apt.AnnotationProcessorFactory;
import com.sun.mirror.apt.Filer;
import com.sun.mirror.declaration.AnnotationTypeDeclaration;
import com.sun.mirror.declaration.TypeDeclaration;
import com.sun.tools.xjc.api.J2SJAXBModel;
import com.sun.tools.xjc.api.Reference;
import com.sun.tools.xjc.api.XJC;
/**
* {@link AnnotationProcessorFactory} that implements the schema generator
* command line tool.
*
* @author Kohsuke Kawaguchi
*/
public class SchemaGenerator implements AnnotationProcessorFactory {
/**
* User-specified schema locations, if any.
*/
private final Map schemaLocations = new HashMap();
public SchemaGenerator() {
}
public SchemaGenerator( Map m ) {
schemaLocations.putAll(m);
}
public Collection supportedOptions() {
return Collections.emptyList();
}
public Collection supportedAnnotationTypes() {
return Arrays.asList("*");
}
public AnnotationProcessor getProcessorFor(Set atds, final AnnotationProcessorEnvironment env) {
return new AnnotationProcessor() {
final ErrorReceiverImpl errorListener = new ErrorReceiverImpl(env);
public void process() {
List decls = new ArrayList();
for(TypeDeclaration d : env.getTypeDeclarations())
decls.add(new Reference(d,env));
J2SJAXBModel model = XJC.createJavaCompiler().bind(decls,Collections.emptyMap(),null,env);
if(model==null)
return; // error
try {
model.generateSchema(
new SchemaOutputResolver() {
public Result createOutput(String namespaceUri, String suggestedFileName) throws IOException {
File file;
OutputStream out;
if(schemaLocations.containsKey(namespaceUri)) {
file = schemaLocations.get(namespaceUri);
if(file==null) return null; // don't generate
out = new FileOutputStream(file);
} else {
// use the default
file = new File(suggestedFileName);
out = env.getFiler().createBinaryFile(Filer.Location.CLASS_TREE,"",file);
}
StreamResult ss = new StreamResult(out);
env.getMessager().printNotice("Writing "+file);
ss.setSystemId(file.getPath());
return ss;
}
}, errorListener);
} catch (IOException e) {
errorListener.error(e.getMessage(),e);
}
}
};
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy