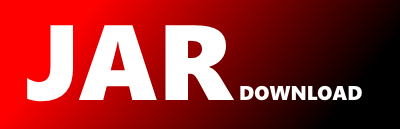
com.sun.xml.rpc.streaming.StAXReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jaxrpc-impl Show documentation
Show all versions of jaxrpc-impl Show documentation
JAXRPC Reference Implementation
/*
* Copyright (c) 1997, 2018 Oracle and/or its affiliates. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v. 2.0, which is available at
* http://www.eclipse.org/legal/epl-2.0.
*
* This Source Code may also be made available under the following Secondary
* Licenses when the conditions for such availability set forth in the
* Eclipse Public License v. 2.0 are satisfied: GNU General Public License,
* version 2 with the GNU Classpath Exception, which is available at
* https://www.gnu.org/software/classpath/license.html.
*
* SPDX-License-Identifier: EPL-2.0 OR GPL-2.0 WITH Classpath-exception-2.0
*/
package com.sun.xml.rpc.streaming;
import org.xml.sax.InputSource;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.Enumeration;
import java.util.HashSet;
import java.util.Iterator;
import javax.xml.namespace.NamespaceContext;
import javax.xml.namespace.QName;
import javax.xml.stream.*;
import javax.xml.transform.Source;
import com.sun.xml.rpc.sp.NamespaceSupport;
import com.sun.xml.rpc.util.exception.LocalizableExceptionAdapter;
import com.sun.xml.rpc.util.xml.CDATA;
// needed for stax workaround for bug 5045462
import javax.xml.transform.Transformer;
import javax.xml.transform.TransformerException;
import javax.xml.transform.stream.StreamResult;
import com.sun.xml.messaging.saaj.util.ByteInputStream;
import com.sun.xml.messaging.saaj.util.ByteOutputStream;
import com.sun.xml.rpc.util.xml.XmlUtil;
import java.io.InputStream;
// end of stax workaround
/**
* An implementation of XMLReader that uses StAX
*/
public class StAXReader extends XMLReaderBase {
static final String XMLNS_NAMESPACE_URI = "http://www.w3.org/2000/xmlns/";
// the stream reader that does the work
private XMLStreamReader reader;
// current state of reader
private int state;
// used for XMLStreamReader states that do not map to XMLReader
private static final int CONTINUE = -10;
// map of StAX xml events to XMLReaderEvents states
private static final int [] stax2XMLReader = new int [16];
static {
for (int i=0; i<16; i++) {
stax2XMLReader[i] = CONTINUE;
}
stax2XMLReader[XMLStreamConstants.START_DOCUMENT] = XMLReader.BOF;
stax2XMLReader[XMLStreamConstants.START_ELEMENT] = XMLReader.START;
stax2XMLReader[XMLStreamConstants.END_ELEMENT] = XMLReader.END;
stax2XMLReader[XMLStreamConstants.CHARACTERS] = XMLReader.CHARS;
stax2XMLReader[XMLStreamConstants.PROCESSING_INSTRUCTION] = XMLReader.PI;
stax2XMLReader[XMLStreamConstants.END_DOCUMENT] = XMLReader.EOF;
}
private QName currentName;
private AttributesImpl currentAttributes;
private ArrayList allPrefixes;
private ElementIdStack elementIds;
private int elementId;
//private static XMLInputFactory inputFactory;
public StAXReader(InputSource source, boolean rejectDTDs) {
try {
reader = getInputFactory().createXMLStreamReader(
source.getByteStream(), source.getEncoding());
finishSetup();
} catch (XMLStreamException e) {
throw new XMLReaderException("staxreader.xmlstreamexception",
new LocalizableExceptionAdapter(e));
}
}
public StAXReader(InputSource source, boolean rejectDTDs,
XMLStreamReader reader)
{
this.reader = reader;
finishSetup();
}
public StAXReader(Source source, boolean rejectDTDs) {
try {
/* still getting exception when trying this, so add workaround
* for now. will ask zephyr team again.
*/
boolean workingYet = false;
if (workingYet) {
reader = getInputFactory().createXMLStreamReader(source);
} else {
Transformer transformer = XmlUtil.newTransformer();
ByteOutputStream bos = new ByteOutputStream();
transformer.transform(source, new StreamResult(bos));
InputStream istream =
new ByteInputStream(bos.getBytes(), bos.getCount());
InputSource iSource = new InputSource(istream);
reader = getInputFactory().createXMLStreamReader(
iSource.getByteStream(), iSource.getEncoding());
}
finishSetup();
} catch (XMLStreamException e) {
throw new XMLReaderException("staxreader.xmlstreamexception",
new LocalizableExceptionAdapter(e));
} catch (TransformerException te) {
// thrown by the workaround code. todo (bobby) remove later
throw new XMLReaderException("staxreader.xmlstreamexception",
new LocalizableExceptionAdapter(te));
}
}
public StAXReader(StringReader stringReader, boolean rejectDTDs) {
try {
reader = getInputFactory().createXMLStreamReader(stringReader);
finishSetup();
} catch (XMLStreamException e) {
throw new XMLReaderException("staxreader.xmlstreamexception",
new LocalizableExceptionAdapter(e));
}
}
/*
* Used by the constructors to get the input factory
*/
private XMLInputFactory getInputFactory() throws XMLStreamException
{
//if (inputFactory == null) {
XMLInputFactory inputFactory = XMLInputFactory.newInstance();
inputFactory.setProperty("javax.xml.stream.isNamespaceAware",
Boolean.TRUE);
inputFactory.setProperty("javax.xml.stream.isCoalescing",
Boolean.TRUE);
//}
return inputFactory;
}
/*
* Used by the constructors to finish setup
*/
private void finishSetup() {
state = stax2XMLReader[reader.getEventType()];
// this will store all parsed prefixes for getPrefixes()
allPrefixes = new ArrayList();
elementIds = new ElementIdStack();
}
/**
* Returns the StAX XMLStreamReader that is being used. If this is
* called, the code must call StAXReader.synchronize() after the
* stream reader has been used so that the StAXReader has correct
* information.
*
* @return The actual XMLStreamReader being used. The StAXReader object
* will not contain valid information once the underlying reader is
* used separately.
*
* @see com.sun.xml.rpc.streaming.StAXReader#synchronizeReader
*/
public XMLStreamReader getXMLStreamReader() {
return reader;
}
/**
* Used to resync the StAXReader with its underlying XMLStreamReader.
* The element id stack may not be valid.
*
* @see com.sun.xml.rpc.streaming.StAXReader#getXMLStreamReader
*/
public void synchronizeReader() {
currentName = null;
currentAttributes = null;
state = stax2XMLReader[reader.getEventType()];
}
public int next() {
if (state == EOF) {
return EOF;
}
currentName = null;
currentAttributes = null;
try {
do {
state = stax2XMLReader[reader.next()];
} while (state == CONTINUE);
if (state == START) {
collectPrefixes();
elementId = elementIds.pushNext();
} else if (state == END) {
elementId = elementIds.pop();
}
} catch (XMLStreamException e) {
throw new XMLReaderException(
"staxreader.xmlstreamexception",
new LocalizableExceptionAdapter(e));
}
return state;
}
public int getState() {
return state;
}
public QName getName() {
if (currentName == null) {
currentName = reader.getName();
}
return currentName;
}
public String getLocalName() {
return reader.getLocalName();
}
public String getURI() {
return reader.getNamespaceURI();
}
public Attributes getAttributes() {
if (currentAttributes == null) {
if (reader.getEventType() == reader.START_ELEMENT ||
reader.getEventType() == reader.ATTRIBUTE) {
currentAttributes = new AttributesImpl(reader);
} else {
// create empty Attributes object when reader in illegal state
currentAttributes = new AttributesImpl(null);
}
}
return currentAttributes;
}
public String getURI(String prefix) {
return reader.getNamespaceURI(prefix);
}
public String getValue() {
return reader.getText();
}
public int getLineNumber() {
return reader.getLocation().getLineNumber();
}
/*
* The goal is to make this method as quick as possible to
* not slow down normal parsing. See getPrefixes() for more info.
*/
private void collectPrefixes() {
for (int i=0; i= 0 && index < atInfos.length) {
return atInfos[index].getLocalName();
}
return null;
}
public QName getName(int index) {
if (index >= 0 && index < atInfos.length) {
return atInfos[index].getName();
}
return null;
}
public String getPrefix(int index) {
if (index >= 0 && index < atInfos.length) {
return atInfos[index].getName().getPrefix();
}
return null;
}
public String getURI(int index) {
if (index >= 0 && index < atInfos.length) {
return atInfos[index].getName().getNamespaceURI();
}
return null;
}
public String getValue(int index) {
if (index >= 0 && index < atInfos.length) {
return atInfos[index].getValue();
}
return null;
}
public String getValue(QName name) {
int index = getIndex(name);
if (index != -1) {
return atInfos[index].getValue();
}
return null;
}
public String getValue(String localName) {
int index = getIndex(localName);
if (index != -1) {
return atInfos[index].getValue();
}
return null;
}
public String getValue(String uri, String localName) {
int index = getIndex(uri, localName);
if (index != -1) {
return atInfos[index].getValue();
}
return null;
}
public boolean isNamespaceDeclaration(int index) {
if (index >= 0 && index < atInfos.length) {
return atInfos[index].isNamespaceDeclaration();
}
return false;
}
public int getIndex(QName name) {
for (int i=0; i -- stax returns null
this.value = "";
} else {
this.value = value;
}
}
QName getName() {
return name;
}
String getValue() {
return value;
}
/*
* Return "xmlns:" as part of name if namespace.
*/
String getLocalName() {
if (isNamespaceDeclaration()) {
if (name.getLocalPart().equals("")) {
return "xmlns";
}
return "xmlns:" + name.getLocalPart();
}
return name.getLocalPart();
}
boolean isNamespaceDeclaration() {
return (name.getNamespaceURI() == XMLNS_NAMESPACE_URI);
}
}
/* This class is used to make a NamespaceContext object act as a
* NamespaceSupport object to pass to RecordedXMLReader. Am hoping
* the context issues will take care of themselves as reader.next()
* is called.
*/
static class NamespaceContextWrapper extends NamespaceSupport {
private NamespaceContext context;
public NamespaceContextWrapper(NamespaceContext context) {
this.context = context;
}
public String getPrefix(String uri) {
return context.getPrefix(uri);
}
public String getURI(String prefix) {
return context.getNamespaceURI(prefix);
}
public Iterator getPrefixes(String uri) {
return context.getPrefixes(uri);
}
public Iterator getPrefixes() {
if (true) { throw new UnsupportedOperationException(); }
return null; // todo
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy