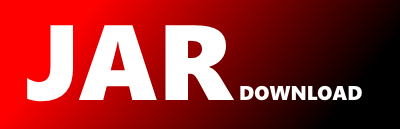
com.sun.xml.ws.db.sdo.SAX2DOMContentHandler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdo-eclipselink-plugin Show documentation
Show all versions of sdo-eclipselink-plugin Show documentation
Pluggable databinding module employing Eclipselink SDO
package com.sun.xml.ws.db.sdo;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.w3c.dom.Node;
import org.w3c.dom.ProcessingInstruction;
import org.w3c.dom.Text;
import org.xml.sax.Attributes;
import org.xml.sax.ContentHandler;
import org.xml.sax.Locator;
import org.xml.sax.SAXException;
import javax.xml.parsers.DocumentBuilder;
import javax.xml.parsers.DocumentBuilderFactory;
import java.util.Stack;
import java.util.Map;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Set;
/**
* Created by IntelliJ IDEA.
* User: giglee
* Date: Jun 5, 2009
* Time: 4:20:01 PM
* To change this template use File | Settings | File Templates.
*/
public class SAX2DOMContentHandler implements ContentHandler {
private Document doc;
private Stack stack;
private Map prefixMappings;
public SAX2DOMContentHandler() {
DocumentBuilderFactory dbf = DocumentBuilderFactory.newInstance();
dbf.setValidating(false);
dbf.setNamespaceAware(true);
dbf.setIgnoringElementContentWhitespace(true);
dbf.setIgnoringComments(true);
try {
DocumentBuilder documentBuilder = dbf.newDocumentBuilder();
doc = documentBuilder.newDocument();
stack = new Stack();
stack.push(doc);
}
catch (Exception e) {
throw new RuntimeException(e);
}
prefixMappings = new HashMap();
}
public SAX2DOMContentHandler(Document d) {
doc = d;
stack = new Stack();
stack.push(d);
prefixMappings = new HashMap();
}
public void setDocumentLocator(Locator locator) {
//To change body of implemented methods use File | Settings | File Templates.
}
public void startDocument() throws SAXException {
//To change body of implemented methods use File | Settings | File Templates.
}
public void endDocument() throws SAXException {
//To change body of implemented methods use File | Settings | File Templates.
}
public void startPrefixMapping(String prefix, String uri) throws SAXException {
prefixMappings.put(prefix, uri);
}
public void endPrefixMapping(String string) throws SAXException {
//To change body of implemented methods use File | Settings | File Templates.
}
public void startElement(String ns, String local, String qname, Attributes attributes) throws SAXException {
if (stack.isEmpty()) {
throw new SAXException("invalid state");
}
Element e = doc.createElementNS(ns, qname);
if (!prefixMappings.isEmpty()) {
Set> prefixMappingSet = prefixMappings.entrySet();
String pname = null;
Iterator> i = prefixMappingSet.iterator();
while (i.hasNext()) {
Map.Entry prefixMapping = i.next();
String p = prefixMapping.getKey();
String u = prefixMapping.getValue();
if (p == null || p.length() == 0) {
pname = "xmlns";
}
else {
pname = "xmlns:" + p;
}
e.setAttributeNS("http://www.w3.org/2000/xmlns/",pname, u);
}
prefixMappings.clear();
}
if (attributes != null) {
for (int i=0; i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy