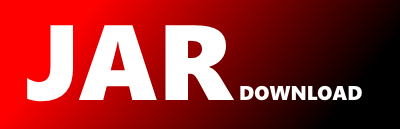
com.sun.xml.ws.db.sdo.SDOWrapperAccessor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sdo-eclipselink-plugin Show documentation
Show all versions of sdo-eclipselink-plugin Show documentation
Pluggable databinding module employing Eclipselink SDO
package com.sun.xml.ws.db.sdo;
import commonj.sdo.DataObject;
import commonj.sdo.Property;
import commonj.sdo.Type;
import commonj.sdo.helper.TypeHelper;
import com.sun.xml.ws.spi.db.PropertyGetter;
import com.sun.xml.ws.spi.db.PropertySetter;
import javax.xml.namespace.QName;
import com.sun.xml.ws.spi.db.PropertyAccessor;
import com.sun.xml.ws.spi.db.WrapperAccessor;
import java.util.HashMap;
import java.util.HashSet;
import java.util.List;
/**
* Created by IntelliJ IDEA.
* User: giglee
* Date: May 19, 2009
* Time: 10:40:31 AM
* To change this template use File | Settings | File Templates.
*/
public class SDOWrapperAccessor extends WrapperAccessor {
private SDOContextWrapper contextWrapper = null;
protected Class> contentClass;
public SDOWrapperAccessor(SDOContextWrapper contextWrapper, Class> wrapperBean) {
this.contextWrapper = contextWrapper;
contentClass = wrapperBean;
initBuilders();
}
protected void initBuilders() {
HashMap
© 2015 - 2025 Weber Informatics LLC | Privacy Policy