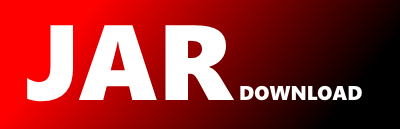
com.svix.models.StreamSinkOut Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of svix Show documentation
Show all versions of svix Show documentation
Svix webhooks API client and webhook verification library
The newest version!
/*
* Svix API
* No description provided (generated by Openapi Generator https://github.com/openapitools/openapi-generator)
*
* The version of the OpenAPI document: 1.1.1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package com.svix.models;
import java.util.Objects;
import com.google.gson.TypeAdapter;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.svix.models.BigQueryConfig;
import com.svix.models.SinkPayloadFormat;
import com.svix.models.SinkStatus;
import com.svix.models.StreamSinkInOneOf;
import com.svix.models.StreamSinkInOneOf1;
import com.svix.models.StreamSinkInOneOf2;
import com.svix.models.StreamSinkInOneOf3;
import com.svix.models.StreamSinkInOneOf4;
import com.svix.models.StreamSinkInOneOf5;
import com.svix.models.StreamSinkInOneOf6;
import com.svix.models.StreamSinkInOneOf7;
import java.io.IOException;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import org.openapitools.jackson.nullable.JsonNullable;
import java.io.IOException;
import java.lang.reflect.Type;
import java.util.logging.Level;
import java.util.logging.Logger;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashSet;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonParseException;
import com.google.gson.TypeAdapter;
import com.google.gson.TypeAdapterFactory;
import com.google.gson.reflect.TypeToken;
import com.google.gson.JsonPrimitive;
import com.google.gson.annotations.JsonAdapter;
import com.google.gson.annotations.SerializedName;
import com.google.gson.stream.JsonReader;
import com.google.gson.stream.JsonWriter;
import com.google.gson.JsonDeserializationContext;
import com.google.gson.JsonDeserializer;
import com.google.gson.JsonSerializationContext;
import com.google.gson.JsonSerializer;
import com.google.gson.JsonElement;
import com.google.gson.JsonObject;
import com.google.gson.JsonArray;
import com.google.gson.JsonParseException;
import com.svix.internal.JSON;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-12-12T23:42:33.364995Z[Etc/UTC]", comments = "Generator version: 7.9.0")
public class StreamSinkOut extends AbstractOpenApiSchema {
private static final Logger log = Logger.getLogger(StreamSinkOut.class.getName());
public static class CustomTypeAdapterFactory implements TypeAdapterFactory {
@SuppressWarnings("unchecked")
@Override
public TypeAdapter create(Gson gson, TypeToken type) {
if (!StreamSinkOut.class.isAssignableFrom(type.getRawType())) {
return null; // this class only serializes 'StreamSinkOut' and its subtypes
}
final TypeAdapter elementAdapter = gson.getAdapter(JsonElement.class);
final TypeAdapter adapterStreamSinkInOneOf = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf.class));
final TypeAdapter adapterStreamSinkInOneOf1 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf1.class));
final TypeAdapter adapterStreamSinkInOneOf2 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf2.class));
final TypeAdapter adapterStreamSinkInOneOf3 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf3.class));
final TypeAdapter adapterStreamSinkInOneOf4 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf4.class));
final TypeAdapter adapterStreamSinkInOneOf5 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf5.class));
final TypeAdapter adapterStreamSinkInOneOf6 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf6.class));
final TypeAdapter adapterStreamSinkInOneOf7 = gson.getDelegateAdapter(this, TypeToken.get(StreamSinkInOneOf7.class));
return (TypeAdapter) new TypeAdapter() {
@Override
public void write(JsonWriter out, StreamSinkOut value) throws IOException {
if (value == null || value.getActualInstance() == null) {
elementAdapter.write(out, null);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf`
if (value.getActualInstance() instanceof StreamSinkInOneOf) {
JsonElement element = adapterStreamSinkInOneOf.toJsonTree((StreamSinkInOneOf)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf1`
if (value.getActualInstance() instanceof StreamSinkInOneOf1) {
JsonElement element = adapterStreamSinkInOneOf1.toJsonTree((StreamSinkInOneOf1)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf2`
if (value.getActualInstance() instanceof StreamSinkInOneOf2) {
JsonElement element = adapterStreamSinkInOneOf2.toJsonTree((StreamSinkInOneOf2)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf3`
if (value.getActualInstance() instanceof StreamSinkInOneOf3) {
JsonElement element = adapterStreamSinkInOneOf3.toJsonTree((StreamSinkInOneOf3)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf4`
if (value.getActualInstance() instanceof StreamSinkInOneOf4) {
JsonElement element = adapterStreamSinkInOneOf4.toJsonTree((StreamSinkInOneOf4)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf5`
if (value.getActualInstance() instanceof StreamSinkInOneOf5) {
JsonElement element = adapterStreamSinkInOneOf5.toJsonTree((StreamSinkInOneOf5)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf6`
if (value.getActualInstance() instanceof StreamSinkInOneOf6) {
JsonElement element = adapterStreamSinkInOneOf6.toJsonTree((StreamSinkInOneOf6)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
// check if the actual instance is of the type `StreamSinkInOneOf7`
if (value.getActualInstance() instanceof StreamSinkInOneOf7) {
JsonElement element = adapterStreamSinkInOneOf7.toJsonTree((StreamSinkInOneOf7)value.getActualInstance());
elementAdapter.write(out, element);
return;
}
throw new IOException("Failed to serialize as the type doesn't match oneOf schemas: StreamSinkInOneOf, StreamSinkInOneOf1, StreamSinkInOneOf2, StreamSinkInOneOf3, StreamSinkInOneOf4, StreamSinkInOneOf5, StreamSinkInOneOf6, StreamSinkInOneOf7");
}
@Override
public StreamSinkOut read(JsonReader in) throws IOException {
Object deserialized = null;
JsonElement jsonElement = elementAdapter.read(in);
int match = 0;
ArrayList errorMessages = new ArrayList<>();
TypeAdapter actualAdapter = elementAdapter;
// deserialize StreamSinkInOneOf
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf'", e);
}
// deserialize StreamSinkInOneOf1
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf1.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf1;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf1'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf1 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf1'", e);
}
// deserialize StreamSinkInOneOf2
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf2.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf2;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf2'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf2 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf2'", e);
}
// deserialize StreamSinkInOneOf3
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf3.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf3;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf3'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf3 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf3'", e);
}
// deserialize StreamSinkInOneOf4
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf4.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf4;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf4'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf4 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf4'", e);
}
// deserialize StreamSinkInOneOf5
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf5.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf5;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf5'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf5 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf5'", e);
}
// deserialize StreamSinkInOneOf6
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf6.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf6;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf6'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf6 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf6'", e);
}
// deserialize StreamSinkInOneOf7
try {
// validate the JSON object to see if any exception is thrown
StreamSinkInOneOf7.validateJsonElement(jsonElement);
actualAdapter = adapterStreamSinkInOneOf7;
match++;
log.log(Level.FINER, "Input data matches schema 'StreamSinkInOneOf7'");
} catch (Exception e) {
// deserialization failed, continue
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf7 failed with `%s`.", e.getMessage()));
log.log(Level.FINER, "Input data does not match schema 'StreamSinkInOneOf7'", e);
}
if (match == 1) {
StreamSinkOut ret = new StreamSinkOut();
ret.setActualInstance(actualAdapter.fromJsonTree(jsonElement));
return ret;
}
throw new IOException(String.format("Failed deserialization for StreamSinkOut: %d classes match result, expected 1. Detailed failure message for oneOf schemas: %s. JSON: %s", match, errorMessages, jsonElement.toString()));
}
}.nullSafe();
}
}
// store a list of schema names defined in oneOf
public static final Map> schemas = new HashMap>();
public StreamSinkOut() {
super("oneOf", Boolean.FALSE);
}
public StreamSinkOut(Object o) {
super("oneOf", Boolean.FALSE);
setActualInstance(o);
}
static {
schemas.put("StreamSinkInOneOf", StreamSinkInOneOf.class);
schemas.put("StreamSinkInOneOf1", StreamSinkInOneOf1.class);
schemas.put("StreamSinkInOneOf2", StreamSinkInOneOf2.class);
schemas.put("StreamSinkInOneOf3", StreamSinkInOneOf3.class);
schemas.put("StreamSinkInOneOf4", StreamSinkInOneOf4.class);
schemas.put("StreamSinkInOneOf5", StreamSinkInOneOf5.class);
schemas.put("StreamSinkInOneOf6", StreamSinkInOneOf6.class);
schemas.put("StreamSinkInOneOf7", StreamSinkInOneOf7.class);
}
@Override
public Map> getSchemas() {
return StreamSinkOut.schemas;
}
/**
* Set the instance that matches the oneOf child schema, check
* the instance parameter is valid against the oneOf child schemas:
* StreamSinkInOneOf, StreamSinkInOneOf1, StreamSinkInOneOf2, StreamSinkInOneOf3, StreamSinkInOneOf4, StreamSinkInOneOf5, StreamSinkInOneOf6, StreamSinkInOneOf7
*
* It could be an instance of the 'oneOf' schemas.
*/
@Override
public void setActualInstance(Object instance) {
if (instance instanceof StreamSinkInOneOf) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf1) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf2) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf3) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf4) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf5) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf6) {
super.setActualInstance(instance);
return;
}
if (instance instanceof StreamSinkInOneOf7) {
super.setActualInstance(instance);
return;
}
throw new RuntimeException("Invalid instance type. Must be StreamSinkInOneOf, StreamSinkInOneOf1, StreamSinkInOneOf2, StreamSinkInOneOf3, StreamSinkInOneOf4, StreamSinkInOneOf5, StreamSinkInOneOf6, StreamSinkInOneOf7");
}
/**
* Get the actual instance, which can be the following:
* StreamSinkInOneOf, StreamSinkInOneOf1, StreamSinkInOneOf2, StreamSinkInOneOf3, StreamSinkInOneOf4, StreamSinkInOneOf5, StreamSinkInOneOf6, StreamSinkInOneOf7
*
* @return The actual instance (StreamSinkInOneOf, StreamSinkInOneOf1, StreamSinkInOneOf2, StreamSinkInOneOf3, StreamSinkInOneOf4, StreamSinkInOneOf5, StreamSinkInOneOf6, StreamSinkInOneOf7)
*/
@SuppressWarnings("unchecked")
@Override
public Object getActualInstance() {
return super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf`. If the actual instance is not `StreamSinkInOneOf`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf`
*/
public StreamSinkInOneOf getStreamSinkInOneOf() throws ClassCastException {
return (StreamSinkInOneOf)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf1`. If the actual instance is not `StreamSinkInOneOf1`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf1`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf1`
*/
public StreamSinkInOneOf1 getStreamSinkInOneOf1() throws ClassCastException {
return (StreamSinkInOneOf1)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf2`. If the actual instance is not `StreamSinkInOneOf2`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf2`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf2`
*/
public StreamSinkInOneOf2 getStreamSinkInOneOf2() throws ClassCastException {
return (StreamSinkInOneOf2)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf3`. If the actual instance is not `StreamSinkInOneOf3`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf3`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf3`
*/
public StreamSinkInOneOf3 getStreamSinkInOneOf3() throws ClassCastException {
return (StreamSinkInOneOf3)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf4`. If the actual instance is not `StreamSinkInOneOf4`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf4`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf4`
*/
public StreamSinkInOneOf4 getStreamSinkInOneOf4() throws ClassCastException {
return (StreamSinkInOneOf4)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf5`. If the actual instance is not `StreamSinkInOneOf5`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf5`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf5`
*/
public StreamSinkInOneOf5 getStreamSinkInOneOf5() throws ClassCastException {
return (StreamSinkInOneOf5)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf6`. If the actual instance is not `StreamSinkInOneOf6`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf6`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf6`
*/
public StreamSinkInOneOf6 getStreamSinkInOneOf6() throws ClassCastException {
return (StreamSinkInOneOf6)super.getActualInstance();
}
/**
* Get the actual instance of `StreamSinkInOneOf7`. If the actual instance is not `StreamSinkInOneOf7`,
* the ClassCastException will be thrown.
*
* @return The actual instance of `StreamSinkInOneOf7`
* @throws ClassCastException if the instance is not `StreamSinkInOneOf7`
*/
public StreamSinkInOneOf7 getStreamSinkInOneOf7() throws ClassCastException {
return (StreamSinkInOneOf7)super.getActualInstance();
}
/**
* Validates the JSON Element and throws an exception if issues found
*
* @param jsonElement JSON Element
* @throws IOException if the JSON Element is invalid with respect to StreamSinkOut
*/
public static void validateJsonElement(JsonElement jsonElement) throws IOException {
// validate oneOf schemas one by one
int validCount = 0;
ArrayList errorMessages = new ArrayList<>();
// validate the json string with StreamSinkInOneOf
try {
StreamSinkInOneOf.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf1
try {
StreamSinkInOneOf1.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf1 failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf2
try {
StreamSinkInOneOf2.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf2 failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf3
try {
StreamSinkInOneOf3.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf3 failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf4
try {
StreamSinkInOneOf4.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf4 failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf5
try {
StreamSinkInOneOf5.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf5 failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf6
try {
StreamSinkInOneOf6.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf6 failed with `%s`.", e.getMessage()));
// continue to the next one
}
// validate the json string with StreamSinkInOneOf7
try {
StreamSinkInOneOf7.validateJsonElement(jsonElement);
validCount++;
} catch (Exception e) {
errorMessages.add(String.format("Deserialization for StreamSinkInOneOf7 failed with `%s`.", e.getMessage()));
// continue to the next one
}
if (validCount != 1) {
throw new IOException(String.format("The JSON string is invalid for StreamSinkOut with oneOf schemas: StreamSinkInOneOf, StreamSinkInOneOf1, StreamSinkInOneOf2, StreamSinkInOneOf3, StreamSinkInOneOf4, StreamSinkInOneOf5, StreamSinkInOneOf6, StreamSinkInOneOf7. %d class(es) match the result, expected 1. Detailed failure message for oneOf schemas: %s. JSON: %s", validCount, errorMessages, jsonElement.toString()));
}
}
/**
* Create an instance of StreamSinkOut given an JSON string
*
* @param jsonString JSON string
* @return An instance of StreamSinkOut
* @throws IOException if the JSON string is invalid with respect to StreamSinkOut
*/
public static StreamSinkOut fromJson(String jsonString) throws IOException {
return JSON.getGson().fromJson(jsonString, StreamSinkOut.class);
}
/**
* Convert an instance of StreamSinkOut to an JSON string
*
* @return JSON string
*/
public String toJson() {
return JSON.getGson().toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy